Web Services with JOnAS
Starting with JOnAS 3.3, Web Services can be used within
EJBs and/or servlets/JSPs. This integration conforms to the JSR 921(v1.1) specification
(public
draft 3).
Note: While JSR 921 is in a maintenance review phase, we provide a link to
the 109(v1.0)
specification.
1. Web Services
A. Some Definitions
WSDL:
WSDL (Web Service Description
Language) specifies an XML format for describing a service as a set of
endpoints that operate on messages.
SOAP:
SOAP (Simple Object Access Protocol)
v1.1 specification defines an XML-based protocol for the exchange of
information in a decentralized, distributed environment. SOAP defines a
convention for representation of remote procedure calls and responses.
JAXRPC:
JAXRPC (Java Api for XML
RPC) v1.1 is the specification that defines the programming model for XML
RPC with Java.
B. Overview of a Web Service
Strictly speaking, a Web Service is a well-defined,
modular, encapsulated function used for loosely coupled integration between
applications' or systems' components. It is based on standard technologies,
such as XML, SOAP, and UDDI.
Web Services are generally exposed and discovered
through a standard registry service. With these standards, Web Services
consumers (whether they be users or other applications) can access a broad
range of information -- personal financial data, news, weather, and
enterprise documents -- through applications that reside on servers
throughout the network.
Web Services use a WSDL Definition (refer to www.w3.org/TR/WSDL) as a contract
between client and server (also called endpoint). WSDL defines the types to
serialize through the network (described with XMLSchema), the messages to
send and receive (composition, parameters), the portTypes (abstract view of a
Port), the bindings (concrete description of PortType: SOAP, GET, POST, ...),
the services (set of Ports), and the Port (the port is associated with a
unique endpoint URL that defines the location of the Web Service).
A Web Service for J2EE is a component with some methods
exposed and accessible by HTTP (through servlet(s)). Web Services can be
implemented as Stateless Session Beans or as JAXRPC classes (a simple Java
class, no inheritance needed).
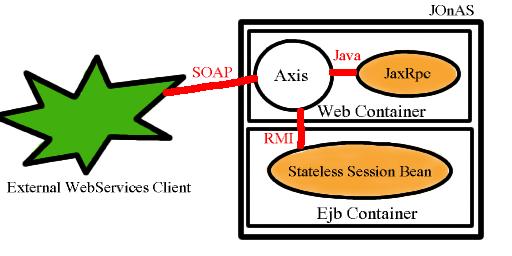
Figure 1. Web Services endpoints deployed within JOnAS (an external client
code can access the endpoint via AxisServlet)
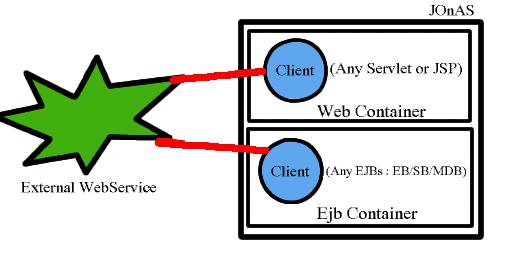
Figure 2. Web Services client deployed within JOnAS (can access external Web
Services)
The servlet is used to respond to a client request and
dispatch the call to the designated instance of servant (the SSB or JAXRPC
class exposed as Web Service). It handles the deserialization of incoming
SOAP message to transform SOAP XML into a Java Object, perform the call, and
serialize the call result (or the thrown exception) into SOAP XML before send
the response message to the client.
2. Exposing a J2EE Component as
a Web Service
There are two types of J2EE components that can be exposed
as Web Services endpoints: StateLess Session Beans and JAX-RPC classes. Web
Services' Endpoints must not contain state information.
A new standard Deployment Descriptor has been created to
describe Web Services endpoints. This Descriptor is named "webservices.xml"
and can be used in a webapp (in WEB-INF/) or in an EjbJar (in META-INF/).
This Descriptor has its JOnAS-specific Deployment Descriptor
(jonas-webservices.xml is optional).
At this time, the user must configure the Axis-specific
deployment descriptor (.wsdd). Refer to the Axis reference
guide for more information about WSDD files.
Refer to the webServices sample for example files (etc
directory).
A. JAX-RPC Endpoint
A JAX-RPC endpoint is a simple class running in the
servlet container (Tomcat or Jetty). SOAP requests are dispatched to an
instance of this class and the response is serialized and sent back to the
client.
A JAX-RPC endpoint must be in a WebApp (the war file must
contain a "WEB-INF/webservices.xml").
Note: The developer must declare the AxisServlet with only
one servlet-mapping. Additionally, a server-config.wsdd must be provided in
the WEB-INF directory. This file tells Axis which service to expose (one
service element corresponds to one port-component in webservices.xml) with
information for accessing the servant class set as parameter(s) sub
element(s) (className, allowedMethods, ...).
B. Stateless Session Bean
Endpoint
An SSB is an EJB that will be exposed (all or some of its
methods) as a Web Service endpoint.
Typically, an SSB must be in an EjbJar, and a
"META-INF/webservices.xml" is located in the EjbJar file.
Note: Because an SSB cannot be accessed directly via HTTP,
the user must create an empty webapp that declares the AxisServlet with a
server-config.wsdd file (refer to the previous). For this, the user must set
information for bean access (beanJndiName, localHomeInterfaceName,
localInterfaceName, homeInterfaceName, remoteInterfaceName) in
server-config.xml. Not all of these parameters are required, only those
specific to the user's needs (for example, no remote interfaces if an SSB is
only local). The developer must also create a JOnAS-specific deployment
descriptor for Web Services: jonas-webservices.xml. The filename of the war
created previously must be specified to identify to JOnAS the URL for
accessing the SSB. Finally, these two files (EjbJar and WebApp) must be added
into an Application archive.
C. Usage
In this Descriptor, the developer describes the
components that will be exposed as Web Services' endpoints; these are called
the port-component(s). A set of port-components defines a
webservice-description, and a webservice-description uses a WSDL Definition
file for a complete description of the Web Services' endpoints.
Each port-component is linked to the J2EE component
that will respond to the request (service-impl-bean with a servlet-link or
ejb-link child element) and to a WSDL port (wsdl-port defining the port's
QName). A list of JAX-RPC Handlers is provided for each port-component. The
optional service-endpoint-interface defines the methods of the J2EE
components that will be exposed (no inheritance needed).
A JAX-RPC Handler is a class used to read and/or modify
the SOAP Message before transmission and/or after reception (refer to the
JAX-RPC v1.1 spec. chap#12 "SOAP Message Handlers").The Session Handler is a
simple example that will read/write SOAP session information in the SOAP
Headers. Handlers are identified with a unique name (within the application
unit), are initialized with the init-param(s), and work on processing a list
of SOAP Headers defined with soap-headers child elements. The Handler is run
as the SOAP actor(s) defined in the list of soap-roles.
A webservice-description defines a set of
port-components, a WSDL Definition (describing the Web Service) and a mapping
file (WSDL-2-Java bindings). The wsdl-file element and the
jaxrpc-mapping-file element must specify a path to a file contained in the
module unit (i.e., the war/jar file). Note that a URL cannot be set here. The
specification also requires that the WSDLs be placed in a wsdl subdirectory
(i.e., WEB-INF/wsdl or META-INF/wsdl); there is no such requirement for the
jaxrpc-mapping-file. All the ports defined in the WSDL must be linked to a
port-component. This is essential because the WSDL is a contract between the
endpoint and a client (if the client use a port not implemented/linked with a
component, the client call will systematically fail).
As for all other Deployment Descriptor, a standard
DTD is used to constrain the XML. Note that DTDs will be replaced by XML
schemas in J2EE 1.4.
D. Simple Example (expose a JAX-RPC Endpoint) of webservices.xml
<?xml version="1.0"?>
<!DOCTYPE webservices PUBLIC
"-//IBM Corporation, Inc.//DTD J2EE Web services 1.0//EN"
"http://www.ibm.com/webservices/dtd/j2ee_web_services_1_0.dtd">
<webservices>
<display-name>Simple Web Service Endpoint
DeploymentDesc</display-name>
<webservice-description>
<!-- name must be unique in an Application unit
-->
<webservice-description-name>
Simple Web Service Endpoint
</webservice-description-name>
<!-- Link to the WSDL file describing the
endpoint -->
<wsdl-file>WEB-INF/wsdl/warendpoint.wsdl</wsdl-file>
<!-- Link to the mapping file describing
binding between WSDL and Java -->
<jaxrpc-mapping-file>WEB-INF/warEndpointMapping.xml</jaxrpc-mapping-file>
<!-- The list of endpoints -->
<port-component>
<!-- Unique name of
the port-component -->
<port-component-name>WebappPortComp1</port-component-name>
<!-- The QName of the WSDL Port the
J2EE port-component is linked to -->
<wsdl-port>
<namespaceURI>http://wsendpoint.servlets.ws.objectweb.org</namespaceURI>
<localpart>wsendpoint1</localpart>
</wsdl-port>
<!-- The endpoint
interface defining methods exposed
for the
endpoint -->
<service-endpoint-interface>
org.objectweb.ws.servlets.wsendpoint.WSEndpointSei
</service-endpoint-interface>
<!-- Link to the J2EE
component (servlet/EJB)
implementing methods of the SEI -->
<service-impl-bean>
<!-- name of the
servlet element defining the JAX-RPC endpoint -->
<!-- can
be ejb-link if SSB is used (only in EjbJar !) -->
<servlet-link>WSEndpoint</servlet-link>
</service-impl-bean>
<!-- The list of
optional JAX-RPC Handlers -->
<handler>
<handler-name>MyHandler</handler-name>
<handler-class>org.objectweb.ws.handlers.SimpleHandler</handler-class>
<!-- A
list of init-param for Handler configuration -->
<init-param>
<param-name>param1</param-name>
<param-value>value1</param-value>
</init-param>
<init-param>
<param-name>param2</param-name>
<param-value>value2</param-value>
</init-param>
</handler>
</port-component>
</webservice-description>
</webservices> |
E. The optionnal jonas-webservices.xml
The jonas-webservices.xml file is collocated with the
webservices.xml. It is an optional Deployment Descriptor (only required in
some case). Its role is to link a webservices.xml to the WebApp in charge of
the SOAP request dispatching. In fact, it is only needed for an EjbJar (the
only one that depends on another servlet to be accessible with HTTP/HTTPS)
that does not use the Default Naming Convention used to retrieve a webapp
name from the EjbJar name.
Convention: <ejbjar-name>.jar will have an
<ejbjar-name>.war WebApp.
Example:
<?xml version="1.0"?>
<!DOCTYPE jonas-webservices PUBLIC
"-//Objectweb//DTD JOnAS WebServices 3.3//EN"
" http://jonas.objectweb.org/dtds/ jonas-webservices_3_3.dtd">
<jonas-webservices>
<!-- the name of the webapp in the EAR (it is
the same as the one
in application.xml)
-->
<war>dispatchingWebApp.war</war>
</jonas-webservices>
|
F. Changes to jonas-web.xml
JOnAS allows the developer to fully configure an
application by setting the hostname, the context-root, and the port used to
access the Web Services. This is done in the jonas-web.xml of the dispatching
WebApp.
host: configure the hostname to use in URL (must be an
available web container host).
port: configure the port number to use in URL (must be an
available HTTP/HTTPS connector port number).
When these values are not set, JOnAS will attempt to
determine the default values for host and port.
Limitations:
- The host can only be determined when only one host is
set for the web container.
- The port can only be determined when only one connector
is used by the web container.
3. The client of a Web
Service
An EJB or a servlet that wants to use a Web Service (as
client) must declare a dependency on the Web Service with a service-ref
element (same principle as for all *-ref elements).
Note: In JOnAS 3.3, the EJB 2.1 and
servlet 2.4 XML Grammar is not yet supported. Therefore, to use service-ref
elements in an ejb-jar.xml and a web.xml, the developer must change the DOCTYPE of the Deployment Descriptors.
For web.xml:
<!DOCTYPE
web-app PUBLIC
"-//Sun Microsystems, Inc.//DTD Web Application 2.3//EN"
" http://jonas.objectweb.org/dtds/ web-app_2_3_ws.dtd">
|
For ejb-jar.xml:
<!DOCTYPE
ejb-jar PUBLIC
"-//Sun
Microsystems, Inc.//DTD Enterprise JavaBeans 2.0//EN"
"http://jonas.objectweb.org/dtds/ejb-jar_2_0_ws.dtd">
|
A. The service-ref element
The service-ref element declares reference to a Web
Service used by a J2EE component in the web and EJB Deployment Descriptor.
The component uses a logical name called a
service-ref-name to lookup the service instance. Thus, any component that
performs a lookup on a Web Service must declare a dependency (the service-ref
element) in the standard deployment descriptor (web.xml or ejb-jar.xml).
Example of service-ref:
<service-ref>
<!-- (Optional) A Web services
description that can be used
in administration
tool. -->
<description>Sample WebService
Client</description>
<!-- (Optional) The WebService
reference name. (used in Administration
tools)
-->
<display-name>WebService Client
1</display-name>
<!-- (Optional) An icon for this
WebService. -->
<icon> <!-- ... --> </icon>
<!-- The logical name for the reference
that is used in the client source
code. It is recommended, but not
required that the name begin with
'services/' -->
<service-ref-name>services/myService</service-ref-name>
<!-- Defines the class name of the
JAX-RPC Service interface that
the client depends on. In most
cases, the value will be :
javax.xml.rpc.Service but a
generated specific Service Interface
class may be specified (require WSDL
knowledge and so
the wsdl-file element).
-->
<service-interface>javax.xml.rpc.Service</service-interface>
<!-- (Optional) Contains the location
(relative to the root of
the module) of the web service WSDL
description.
- need to be in the wsdl directory.
- required if generated interface
and sei are declared. -->
<wsdl-file>WEB-INF/wsdl/stockQuote.wsdl</wsdl-file>
<!--
(Optional) A file specifying the
correlation of the WSDL definition
to the interfaces (Service Endpoint
Interface, Service Interface).
- required if generated
interface and sei (Service Endpoint
Interface) are
declared. -->
<jaxrpc-mapping-file>WEB-INF/myMapping.xml</jaxrpc-mapping-file>
<!-- (Optional) Declares the specific
WSDL service element that is being
referred to. It is not specified if
no wsdl-file is declared or if
WSDL contains only 1 service
element. A service-qname is composed
by a namespaceURI and a localpart.
It must be defined if more than 1
service is declared in
the WSDL. -->
<service-qname>
<namespaceURI>http://beans.ws.objectweb.org</namespaceURI>
<localpart>MyWSDLService</localpart>
</service-qname>
<!-- Declares a client dependency on
the container to resolving a Service
Endpoint Interface to a WSDL port.
It optionally associates the
Service Endpoint Interface with a
particular port-component. -->
<port-component-ref>
<service-endpoint-interface>
org.objectweb.ws.beans.ssbendpoint.MyService
</service-endpoint-interface>
<!-- Define a link to a port component
declared in another unit
of the
application -->
<port-component-link>ejb_module.jar#PortComponentName </port-component-link>
</port-component-ref>
<!--A list of Handler to use for this
service-ref -->
<handler>
<!-- Must be unique
within the module. -->
<handler-name>MyHandler</handler-name>
<handler-class>org.objectweb.ws.handlers.myHandler</handler-class>
<!-- A list of init-param (couple
name/value) for Handler
initialization -->
<init-param>
<param-name>param_1</param-name>
<param-value>value_1</param-value>
</init-param>
<!-- A list of QName specifying the
SOAP Headers the handler
will work on. Namespace and
locapart values must be found
inside the WSDL. -->
<soap-header>
<namespaceURI>http://ws.objectweb.org</namespaceURI>
<localpart>MyWSDLHeader</localpart>
</soap-header>
<!-- A list of SOAP actor
definition that the Handler will play
as a role. A soap-role is a
namespace URI. -->
<soap-role>http://actor.soap.objectweb.org</soap-role>
<!-- A list
of port-name element defines the WSDL port-name
that
a handler should be
associated with. If no port-name is specified,
the handler is assumed to
be associated with all ports of the
service-ref.
-->
<port-name>myWSDLPort</port-name>
</handler>
</service-ref> |
B. The jonas-service-ref element
A jonas-service-ref must be specified for each service-ref
declared in the standard Deployment Descriptor. The jonas-service-ref adds
JOnAS-specific (and Web Service engine-specific) information to service-ref
elements.
Example of jonas-service-ref:
<jonas-service-ref>
<!-- Define the service-ref
contained in the component
deployment descriptor
(web.xml or ejb-jar.xml).
used as a key to associate a
service-ref to its correspondent
jonas-service-ref-->
<service-ref-name>services/myService</service-ref-name>
<!-- Define the physical
name of the resource. -->
<jndi-name>webservice_1</jndi-name>
<!-- A list of init-param used for specific
configuration of
the service -->
<jonas-init-param>
<param-name>serviceClassName</param-name>
<param-value>org.objectweb.ws.services.myServiceImpl</param-value>
</jonas-init-param>
</jonas-service-ref> |
6. Limitations
- The jaxrpx-mapping-file is used only to retrieve XML namespace to java
package information mapping. All other information is not used at this
time (Axis limitation).
- service-endpoint-interface in port-component and port-component-ref is
read, but not used.
- port-component-link element is not supported
last update : 17 September 2003