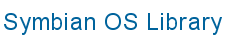
![]() |
![]() |
|
EmbeddingShell
: embedded pictures
EmbeddingShell
provides an application shell, and a
concrete control.
CGraphicExampleControl
is a control class, derived from
CCoeControl
, which links the app shell to the concrete control.
The CPictureControl
class, derived from
CGraphicExampleControl
, illustrates the drawing of pictures and
how they can be stored to and restored from a direct file store.
The source code for this example application can be found in the directory:
examples\Graphics\Embedding
The files reproduced here are the main files contained in the examples directory. Some extra files may be needed to run the examples, and these will be found in the appropriate examples directory.
// EmbeddingShell.h
//
// Copyright (c) 2000 Symbian Ltd. All rights reserved.
#ifndef __EmbeddingShell_H
#define __EmbeddingShell_H
#include <coecntrl.h>
#include <coeccntx.h>
#include <eikappui.h>
#include <eikapp.h>
#include <eikdoc.h>
#include "EmbeddingGraphicsControl.h"
// UID of app
const TUid KUidExampleShellApp={ 0x1000525c };
//
// TExampleShellModel
//
class TExampleShellModel
{
public:
TExampleShellModel();
TBool Differs(const TExampleShellModel* aCompare) const;
public:
TFileName iLibrary; // active control
};
//
// class CExampleShellContainer
//
class CExampleShellContainer : public CCoeControl,
public MCoeControlBrushContext,
public MGraphicsExampleObserver
{
public:
void ConstructL(const TRect& aRect, TExampleShellModel* aModel);
~CExampleShellContainer();
// changing view
void ResetExampleL(CGraphicExampleControl* aExample);
private: // from CCoeControl
void Draw(const TRect& /*aRect*/) const;
TKeyResponse OfferKeyEventL(const TKeyEvent& aKeyEvent,TEventCode aType);
TInt CountComponentControls() const;
CCoeControl* ComponentControl(TInt aIndex) const;
private: // from MGraphicsExampleObserver
void NotifyGraphicExampleFinished();
public: // also from MGraphicsExampleObserver
void NotifyStatus(const TDesC& aMessage);
private: // new function
void CreateLabelL();
private: // data
CGraphicExampleControl* iExampleControl; // example control
CEikLabel* iLabel; // label for status messages
// irrelevant
TExampleShellModel* iModel;
};
//
// CExampleShellDocument
//
class CExampleShellDocument : public CEikDocument
{
public:
CExampleShellDocument(CEikApplication& aApp): CEikDocument(aApp) { }
TExampleShellModel* Model() { return(&iModel); }
private: // from CEikDocument
CEikAppUi* CreateAppUiL();
private:
TExampleShellModel iModel;
};
//
// CExampleShellAppUi
//
class CExampleShellAppUi : public CEikAppUi
{
public:
void ConstructL();
~CExampleShellAppUi();
private: // from CEikAppUi
void HandleCommandL(TInt aCommand);
private: // internal use
// void PrepareToolbarButtons();
private:
CExampleShellContainer* iContainer;
TExampleShellModel* iModel;
};
//
// CExampleShellApplication
//
class CExampleShellApplication : public CEikApplication
{
private: // from CApaApplication
CApaDocument* CreateDocumentL();
TUid AppDllUid() const;
};
#endif
// EmbeddingGraphicsControl.h
//
// Copyright (c) 2000 Symbian Ltd. All rights reserved.
#ifndef __EmbeddingGraphicsControl_H
#define __EmbeddingGraphicsControl_H
#include <coecntrl.h>
#include <s32file.h>
// For Picture example
// CSmileyPicture
#include "CommonGraphicsControlFramework.h"
class CSmileyPicture : public CPicture
{
public:
enum TMood { EHappy, ENeutral, ESad }; // various moods
enum TSizeSpec { ELarge, EMedium, ESmall }; // sizes
// creating
CSmileyPicture();
static CSmileyPicture* NewL(TMood aMood, TSizeSpec aSizeSpec); // from scratch
static CSmileyPicture* NewL(const CStreamStore& aStore, TStreamId aStreamId); // from stream
TStreamId StoreL(CStreamStore& aStore) const;
void Draw(CGraphicsContext& aGc,const TPoint& aTopLeft,const TRect& aClipRect,
MGraphicsDeviceMap* aMap)const;
void SetMood(TMood aMood); // set mood
TMood Mood(); // get mood
void SetSize(TSizeSpec aSizeSpec); // set size
TSizeSpec Size(); // get size
TInt SpecToFactor() const;
void GetOriginalSizeInTwips(TSize& aSize) const;
// only needed for cropping and scaling
void SetScaleFactor(TInt aScaleFactorWidth,TInt aScaleFactorHeight);
void SetCropInTwips(const TMargins& aMargins);
TPictureCapability Capability() const;
void GetCropInTwips(TMargins& aMargins) const;
TInt ScaleFactorWidth() const;
TInt ScaleFactorHeight() const;
private:
// streaming
void ExternalizeL(RWriteStream& aStream) const; // externalize state
void InternalizeL(RReadStream& aStream); // internalize state
private:
// member data - size and details of whether happy or sad
TMood iMood;
TSizeSpec iSizeSpec;
};
// sundry derived classes
class CPictureControl : public CGraphicExampleControl
{
public:
CPictureControl();
~CPictureControl() { delete(iPicture); };
void UpdateModelL();
void Draw(const TRect& aRect) const;
private:
enum TDocStatus { EFalse, EHeader, EPicture };
TStreamId StoreHeaderL(CStreamStore& aStore) const;
void StoreHeaderComponentsL(CStoreMap& aMap,CStreamStore& aStore) const;
void RestoreHeaderL(CStreamStore& aStore, TStreamId aId);
TZoomFactor testZf;
MGraphicsDeviceMap* testMap;
TDocStatus iValidDocument; // if false, then Draw() draws gray screen
CSmileyPicture* iPicture; // if there, then draw
TPictureHeader iHeader; // if no iPicture, draw outline to specified size
TPoint iOffset; // offset of picture from top-left
CFileStore* iStore; // stream store for persistence
TStreamId iHeaderId; // root stream of store
};
#endif
// EmbeddingShell.cpp
//
// Copyright (c) 2000-2005 Symbian Software Ltd. All rights reserved.
#include <e32keys.h>
#include <coemain.h>
#include <eikenv.h>
#include <eikdef.h>
#include <eikon.hrh>
#include <eiklabel.h>
#include <eikstart.h>
#include <EmbeddingShell.rsg>
#include "EmbeddingShell.hrh"
#include "EmbeddingShell.h"
//
// TExampleShellModel
//
TExampleShellModel::TExampleShellModel()
{
iLibrary=KNullDesC;
}
TBool TExampleShellModel::Differs(const TExampleShellModel* aCompare) const
{
return((*(TInt32*)this)!=(*(TInt32*)aCompare));
}
//
// class CExampleShellContainer
//
void CExampleShellContainer::ConstructL(const TRect& aRect, TExampleShellModel* aModel)
{
iModel=aModel;
CreateWindowL();
Window().SetShadowDisabled(ETrue);
iContext=this;
iBrushStyle=CGraphicsContext::ESolidBrush;
iBrushColor=KRgbWhite;
SetRect(aRect);
CreateLabelL();
ActivateL();
}
CExampleShellContainer::~CExampleShellContainer()
{
delete iExampleControl;
delete iLabel;
}
TInt CExampleShellContainer::CountComponentControls() const
{
return 1 + (iExampleControl ? 1 : 0);
}
CCoeControl* CExampleShellContainer::ComponentControl(TInt aIndex) const
{
switch (aIndex)
{
case 0: return iLabel;
case 1: return iExampleControl;
default: return 0;
};
}
const TInt KLabelHeight=20;
void CExampleShellContainer::CreateLabelL()
{
iLabel=new (ELeave) CEikLabel;
TRect rect=Rect();
rect.iTl.iY=rect.iBr.iY-KLabelHeight; // make it bottom 20 pixels
iLabel->SetContainerWindowL(*this);
iLabel->SetRect(rect);
iLabel->SetAlignment(EHCenterVCenter); // center text
iLabel->SetBufferReserveLengthL(200); // nice long buffer
iLabel->SetFont(iEikonEnv->AnnotationFont());
iLabel->ActivateL(); // now ready
}
void CExampleShellContainer::ResetExampleL(CGraphicExampleControl* aExample)
{
// get rid of old control
delete iExampleControl;
// set up new one
iExampleControl=aExample;
// if non-zero, then carry on
if (!iExampleControl) return;
TRect rect=Rect(); // get our rect
rect.iBr.iY-=KLabelHeight; // make way for label
rect.Shrink(2,2); // shrink it a bit
iExampleControl->ConstructL(rect,this,*this); // construct, giving rect and observer
}
_LIT(KTxtFinished,"example finished");
void CExampleShellContainer::NotifyGraphicExampleFinished()
{
NotifyStatus(KTxtFinished);
}
void CExampleShellContainer::NotifyStatus(const TDesC& aMessage)
{
iLabel->SetTextL(aMessage);
if (IsActivated()) iLabel->DrawNow();
}
TKeyResponse CExampleShellContainer::OfferKeyEventL(const TKeyEvent& aKeyEvent,TEventCode aType)
{
if (iExampleControl)
return iExampleControl->OfferKeyEventL(aKeyEvent,aType);
else
return EKeyWasNotConsumed;
}
void CExampleShellContainer::Draw(const TRect& /*aRect*/) const
{
CWindowGc& gc = SystemGc();
gc.SetPenStyle(CGraphicsContext::ENullPen);
gc.SetBrushStyle(CGraphicsContext::ESolidBrush);
gc.DrawRect(Rect());
}
//
// CExampleShellAppUi
//
_LIT(KTxtInitialized,"initialized");
void CExampleShellAppUi::ConstructL()
{
BaseConstructL();
iModel=((CExampleShellDocument*)iDocument)->Model();
iContainer=new(ELeave) CExampleShellContainer;
iContainer->ConstructL(ClientRect(),iModel);
iContainer->NotifyStatus(KTxtInitialized);
// add container to stack; enables key event handling.
AddToStackL(iContainer);
}
void CExampleShellAppUi::HandleCommandL(TInt aCommand)
{
switch (aCommand)
{
case EExampleShellSelectPicture:
iContainer->ResetExampleL(new (ELeave) CPictureControl);
return;
case EEikCmdExit:
Exit();
return;
}
}
CExampleShellAppUi::~CExampleShellAppUi()
{
RemoveFromStack(iContainer);
delete iContainer;
}
//
// CExampleShellDocument
//
CEikAppUi* CExampleShellDocument::CreateAppUiL()
{
return(new(ELeave) CExampleShellAppUi);
}
//
// CExampleShellApplication
//
TUid CExampleShellApplication::AppDllUid() const
{
return KUidExampleShellApp;
}
CApaDocument* CExampleShellApplication::CreateDocumentL()
{
return new(ELeave) CExampleShellDocument(*this);
}
//
// EXPORTed functions
//
EXPORT_C CApaApplication* NewApplication()
{
return new CExampleShellApplication;
}
GLDEF_C TInt E32Main()
{
return EikStart::RunApplication(NewApplication);
}
// EmbeddingGraphicsControl.cpp
//
// Copyright (c) 2000 Symbian Ltd. All rights reserved.
#include "EmbeddingGraphicsControl.h"
#include <coemain.h>
#include <coeaui.h>
_LIT(KtxtSwiss,"Swiss");
void CGraphicExampleControl::ConstructL(const TRect& aRect, MGraphicsExampleObserver* aGraphObserver, const CCoeControl& aParent)
{
// remember the graphics observer
iGraphObserver=aGraphObserver;
// create window
CreateWindowL(&aParent);
// construct font for messages
TFontSpec spec(KtxtSwiss,213);
iMessageFont=iCoeEnv->CreateScreenFontL(spec);
// set rectangle to prescription
SetRect(aRect);
// go for it
ActivateL();
UpdateModelL(); // phase 0
}
CGraphicExampleControl::~CGraphicExampleControl()
{
iCoeEnv->ReleaseScreenFont(iMessageFont);
}
void CGraphicExampleControl::Quit()
{
iGraphObserver->NotifyGraphicExampleFinished();
}
void CGraphicExampleControl::NextPhaseL()
{
if (++iPhase >= iMaxPhases)
Quit();
else
{
UpdateModelL();
DrawNow();
}
}
void CGraphicExampleControl::HandlePointerEventL(const TPointerEvent& aPointerEvent)
{
if (aPointerEvent.iType==TPointerEvent::EButton1Down) NextPhaseL();
}
TKeyResponse CGraphicExampleControl::OfferKeyEventL(
const TKeyEvent& aKeyEvent,TEventCode aType
)
{
if (aType!=EEventKey) return EKeyWasNotConsumed;
TInt code=aKeyEvent.iCode;
switch (code)
{
case ' ':
NextPhaseL();
break;
default:
return EKeyWasNotConsumed;
}
return EKeyWasConsumed;
}
// Picture.cpp
//
// Copyright (c) 2000-2005 Symbian Software Ltd. All rights reserved.
#include "EmbeddingGraphicsControl.h"
#include <coemain.h>
// for the store
#include <s32std.h>
static const TUid KUidExampleSmileyPicture = { 0x100000E6 } ;
/*
CExamplePictureFactory
Give picture header, checks UID and only if it's KUidExampleSmileyPicture will this restore
a picture - changing the swizzle in aHeader from a stream id into a pointer.
[incidentally, what errors should result if either the picture is already restored, or the UID
isn't recognized?]
*/
class TExamplePictureFactory : public MPictureFactory
{
public:
void NewPictureL(TPictureHeader& aHeader,const CStreamStore& aDeferredPictureStore) const;
};
void TExamplePictureFactory::NewPictureL(TPictureHeader& aHeader,const CStreamStore& aDeferredPictureStore) const
{
if (aHeader.iPictureType == KUidExampleSmileyPicture)
{
// restore new picture from store into the TSwizzle
// (which changes from stream id to pointer)
// Construct a CSmileyPicture object and restore from the stream
if (aHeader.iPicture.IsId())
aHeader.iPicture = CSmileyPicture::NewL(aDeferredPictureStore,aHeader.iPicture.AsId());
}
else
{
// output error and panic
User::Leave(KErrNoMemory);
}
}
// implementation of the CSmileyPicture class
CSmileyPicture::CSmileyPicture()
{
// do nothing
}
CSmileyPicture* CSmileyPicture::NewL(TMood aMood, TSizeSpec aSizeSpec)
{
CSmileyPicture* self = new (ELeave) CSmileyPicture();
self->iMood = aMood;
self->iSizeSpec = aSizeSpec;
return self;
}
CSmileyPicture* CSmileyPicture::NewL(const CStreamStore& aStore, TStreamId aStreamId)
// create from store
{
RStoreReadStream inStream;
inStream.OpenLC(aStore,aStreamId);
CSmileyPicture* self = new (ELeave) CSmileyPicture();
self->InternalizeL(inStream);
CleanupStack::PopAndDestroy();
return self;
}
TStreamId CSmileyPicture::StoreL(CStreamStore& aStore) const
// stores the CSmileyPicture in a new stream of the specified store (using ExternalizeL())
{
RStoreWriteStream stream;
TStreamId id=stream.CreateLC(aStore);
ExternalizeL(stream);
stream.CommitL();
CleanupStack::PopAndDestroy();
return id;
}
void CSmileyPicture::ExternalizeL(RWriteStream& aStream) const
{
aStream.WriteInt8L(iMood);
aStream.WriteInt8L(iSizeSpec);
}
void CSmileyPicture::InternalizeL(RReadStream& aStream)
{
iMood = TMood(aStream.ReadInt8L());
iSizeSpec = TSizeSpec(aStream.ReadInt8L());
}
TInt CSmileyPicture::SpecToFactor() const
{
switch (iSizeSpec)
{
case ESmall:
return 1;
case EMedium:
return 2;
case ELarge:
return 3;
default:
return 0;
}
}
void CSmileyPicture::Draw(CGraphicsContext& aGc,const TPoint& aTopLeft,const TRect& aClipRect,
MGraphicsDeviceMap* /*aMap*/) const
{
aGc.SetClippingRect(aClipRect);
TInt scaleFactor = SpecToFactor();
TSize penSizeBold(3*scaleFactor,3*scaleFactor);
TSize penSizeFat(5*scaleFactor,5*scaleFactor);
aGc.SetPenSize(penSizeFat);
TInt leftOffset = 13*scaleFactor;
TInt rightOffset = 27*scaleFactor;
TInt circleSize = 40*scaleFactor;
TInt shrinkSize = 10*scaleFactor;
TInt halfCircle = 20*scaleFactor;
TInt neutralSize = 13*scaleFactor;
TPoint leftEye(aTopLeft.iX+leftOffset,aTopLeft.iY+leftOffset);
TPoint rightEye(aTopLeft.iX+rightOffset,aTopLeft.iY+leftOffset);
aGc.Plot(leftEye);
aGc.Plot(rightEye);
aGc.SetPenSize(penSizeBold);
TRect circle(aTopLeft,TPoint(aTopLeft.iX+circleSize,aTopLeft.iY+circleSize));
aGc.DrawEllipse(circle);
// draw the smile
TRect smile = circle;
switch (iMood)
{
case EHappy:
{
smile.Shrink(shrinkSize,shrinkSize);
aGc.DrawArc(smile,TPoint(aTopLeft.iX,aTopLeft.iY+circleSize-shrinkSize),TPoint(aTopLeft.iX+circleSize,aTopLeft.iY+circleSize-shrinkSize));
}
break;
case ENeutral:
{
aGc.DrawLine(TPoint(leftEye.iX,leftEye.iY+neutralSize),TPoint(rightEye.iX,rightEye.iY+neutralSize));
}
break;
case ESad:
{
smile.Shrink(shrinkSize,shrinkSize);
smile.Move(0,15*scaleFactor);
aGc.DrawArc(smile,TPoint(aTopLeft.iX+circleSize,aTopLeft.iY+halfCircle),TPoint(aTopLeft.iX,aTopLeft.iY+halfCircle));
}
break;
}
}
void CSmileyPicture::SetMood(TMood aMood)
{
iMood = aMood;
}
CSmileyPicture::TMood CSmileyPicture::Mood()
{
return iMood;
}
void CSmileyPicture::SetSize(TSizeSpec aSizeSpec)
{
iSizeSpec = aSizeSpec;
}
CSmileyPicture::TSizeSpec CSmileyPicture::Size()
{
return iSizeSpec;
}
void CSmileyPicture::GetOriginalSizeInTwips(TSize& /*aSize*/) const
{
// do nothing
}
void CSmileyPicture::SetScaleFactor(TInt /*aScaleFactorWidth*/,TInt /*aScaleFactorHeight*/)
{
// do nothing
}
TInt CSmileyPicture::ScaleFactorWidth()const
{
return 1;
}
TInt CSmileyPicture::ScaleFactorHeight()const
{
return 1;
}
void CSmileyPicture::SetCropInTwips(const TMargins& /*aMargins*/)
{
// do nothing
}
void CSmileyPicture::GetCropInTwips(TMargins& aMargins)const
{
CPicture::GetCropInTwips(aMargins); // no crop
}
TPictureCapability CSmileyPicture::Capability()const
{
return CPicture::Capability(); // no scale, no crop
}
// example really starts here
CPictureControl::CPictureControl()
{
SetMaxPhases(7);
iValidDocument = CPictureControl::EPicture;
iOffset = TPoint(50,50);
}
// The file name
_LIT(KFileName,"C:\\grpict.dat");
// Literal text
_LIT(KTxtUpdateModelCase0,"draw happy face at (50,50)");
_LIT(KTxtUpdateModelCase1,"draw sad face at (50,50)");
_LIT(KTxtUpdateModelCase2,"draw neutral face at (50,50)");
_LIT(KTxtUpdateModelCase3,"store picture in file");
_LIT(KTxtUpdateModelCase4,"delete picture from memory");
_LIT(KTxtUpdateModelCase5,"restore picture header from file");
_LIT(KTxtUpdateModelCase6,"restore picture from file");
void CPictureControl::UpdateModelL()
{
// set up zoom factor object
testZf.SetGraphicsDeviceMap(iCoeEnv->ScreenDevice());
// set the zoom factor of the object
testZf.SetZoomFactor(TZoomFactor::EZoomOneToOne);
// use graphics device maps for drawing and getting fonts
testMap=&testZf;
// the file session used in the example
RFs fsSession;
TParse filestorename;
switch (Phase())
{
case 0:
iGraphObserver->NotifyStatus(KTxtUpdateModelCase0);
iPicture = CSmileyPicture::NewL(CSmileyPicture::EHappy,CSmileyPicture::ESmall);
break;
case 1:
iGraphObserver->NotifyStatus(KTxtUpdateModelCase1);
iPicture->SetMood(CSmileyPicture::ESad);
iPicture->SetSize(CSmileyPicture::EMedium);
break;
case 2:
iGraphObserver->NotifyStatus(KTxtUpdateModelCase2);
iPicture->SetMood(CSmileyPicture::ENeutral);
iPicture->SetSize(CSmileyPicture::ELarge);
break;
case 3:
iGraphObserver->NotifyStatus(KTxtUpdateModelCase3);
// set up the permament direct file store for the picture
fsSession.Connect();
// Create (replace, if it exists) the direct file store
fsSession.Parse(KFileName,filestorename);
iStore = CDirectFileStore::ReplaceLC(fsSession,filestorename.FullName(),EFileWrite);
// Must say what kind of file store.
iStore->SetTypeL(KDirectFileStoreLayoutUid);
// create picture header
iHeader.iPicture = iPicture;
iHeader.iSize = TSize(iPicture->SpecToFactor()*40,iPicture->SpecToFactor()*40);
iHeader.iPictureType = KUidExampleSmileyPicture;
// store picture header and picture
iHeaderId = StoreHeaderL(*iStore);
// make header stream the root stream
iStore->SetRootL(iHeaderId);
// close store
CleanupStack::PopAndDestroy();
fsSession.Close();
break;
case 4:
iGraphObserver->NotifyStatus(KTxtUpdateModelCase4);
delete iPicture;
iPicture = NULL;
iValidDocument = CPictureControl::EFalse;
break;
case 5:
iGraphObserver->NotifyStatus(KTxtUpdateModelCase5);
// set up the permament direct file store for the picture
fsSession.Connect();
// Open the direct file store and read the header
fsSession.Parse(KFileName,filestorename);
iStore = CDirectFileStore::OpenLC(fsSession,filestorename.FullName(),EFileRead);
RestoreHeaderL(*iStore,iStore->Root());
// close store
CleanupStack::PopAndDestroy();
fsSession.Close();
iValidDocument = CPictureControl::EHeader;
break;
case 6:
iGraphObserver->NotifyStatus(KTxtUpdateModelCase6);
fsSession.Connect();
// Open the direct file store and read the header
fsSession.Parse(KFileName,filestorename);
iStore = CDirectFileStore::OpenLC(fsSession,filestorename.FullName(),EFileRead);
TExamplePictureFactory factory;
factory.NewPictureL(iHeader,*iStore);
iPicture = (CSmileyPicture *) iHeader.iPicture.AsPtr();
// close store
CleanupStack::PopAndDestroy();
fsSession.Close();
iValidDocument = CPictureControl::EPicture;
break;
}
}
void CPictureControl::RestoreHeaderL(CStreamStore& aStore, TStreamId aId)
{
RStoreReadStream stream;
stream.OpenLC(aStore,aId);
iHeader.InternalizeL(stream);
CleanupStack::PopAndDestroy(); // close and delete the stream
}
TStreamId CPictureControl::StoreHeaderL(CStreamStore& aStore) const
{
CStoreMap* map=CStoreMap::NewLC(aStore);
StoreHeaderComponentsL(*map,aStore);
RStoreWriteStream stream(*map);
TStreamId id = stream.CreateLC(aStore);
iHeader.ExternalizeL(stream);
stream.CommitL();
map->Reset();
CleanupStack::PopAndDestroy(2);
return id;
}
void CPictureControl::StoreHeaderComponentsL(CStoreMap& aMap,CStreamStore& aStore) const
{
TStreamId id;
id = iPicture->StoreL(aStore);
aMap.BindL(iPicture,id);
}
void CPictureControl::Draw(const TRect& /* aRect */) const
{
// draw surrounding rectangle
CWindowGc& gc=SystemGc(); // graphics context we draw to
gc.UseFont(iMessageFont); // use the system message font
gc.Clear(); // clear the area to be drawn to
SystemGc().DrawRect(Rect()); // surrounding rectangle to draw into
TRect rect=Rect(); // a centered rectangle of the default size
TRgb darkGray(85,85,85);
// decide what to do, and do it
switch (iValidDocument)
{
case CPictureControl::EFalse:
// if document is not valid then Draw() draws gray screen
gc.SetBrushColor(darkGray);
gc.Clear(rect);
break;
case CPictureControl::EPicture:
// if picture in is memory then draw it
iPicture->Draw(gc,iOffset,rect,testMap);
break;
case CPictureControl::EHeader:
// if no iPicture, draw picture outline to specified size at iOffset
TInt bottomRightX = iOffset.iX + iHeader.iSize.iWidth;
TInt bottomRightY = iOffset.iY + iHeader.iSize.iHeight;
TRect outlineBox(iOffset,TPoint(bottomRightX,bottomRightY));
gc.SetPenStyle(CGraphicsContext::EDottedPen);
gc.DrawRect(outlineBox);
break;
}
//iConEnv->ScreenDevice()->ReleaseFont(iMessageFont);
//gc.ReleaseFont(iMessageFont);
//gc.DiscardFont(); // discard font
}
// EmbeddingShell.hrh
//
// Copyright (c) 2000 Symbian Ltd. All rights reserved.
enum TExampleMenuCommands
{
EExampleShellSelectPicture=200
};
// BLD.INF
// Component description file
//
// Copyright (c) 2000 Symbian Ltd. All rights reserved.
PRJ_MMPFILES
EmbeddingShell.mmp
// EmbeddingShell.mmp
//
// Copyright (c) 2000-2005 Symbian Software Ltd. All rights reserved.
// using relative paths for source and userinclude directories
TARGET EmbeddingShell.exe
TARGETTYPE exe
UID 0x100039ce 0x1000525c
VENDORID 0x70000001
SOURCEPATH .
SOURCE EmbeddingShell.cpp EmbeddingGraphicsControl.cpp Picture.cpp
START RESOURCE EmbeddingShell.rss
TARGETPATH \resource\apps
HEADER
END
USERINCLUDE .
USERINCLUDE ..\CommonGraphicsExampleFiles
SYSTEMINCLUDE \Epoc32\include
SYSTEMINCLUDE \epoc32\include\techview
LIBRARY euser.lib efsrv.lib estor.lib gdi.lib ws32.lib
LIBRARY cone.lib apparc.lib eikcore.lib eikcoctl.lib
START RESOURCE EmbeddingShell_reg.rss
TARGETPATH \private\10003a3f\apps
END
// EmbeddingShell.rss
//
// Copyright (c) 2000 Symbian Ltd. All rights reserved.
NAME GRSH
#include <eikon.rh>
#include "EmbeddingShell.hrh"
RESOURCE RSS_SIGNATURE { }
RESOURCE TBUF { buf=""; }
RESOURCE EIK_APP_INFO
{
menubar=r_grsh_menubar;
hotkeys=r_grsh_hotkeys;
}
RESOURCE HOTKEYS r_grsh_hotkeys
{
control=
{
HOTKEY { command=EEikCmdExit; key='e'; }
};
}
RESOURCE MENU_BAR r_grsh_menubar
{
titles=
{
MENU_TITLE {menu_pane=menu1; txt="File"; },
MENU_TITLE {menu_pane=menu2; txt="List Of Programs "; }
};
}
RESOURCE MENU_PANE menu1
{
items=
{
MENU_ITEM { command=EEikCmdExit; txt="Exit"; }
};
}
RESOURCE MENU_PANE menu2
{
items=
{
MENU_ITEM { command=EExampleShellSelectPicture; txt="Picture"; }
};
}
EmbeddingShell_reg.rss
#include <appinfo.rh>
UID2 KUidAppRegistrationResourceFile
UID3 0x1000525c // application UID
RESOURCE APP_REGISTRATION_INFO
{
app_file = "EmbeddingShell";
}
The source code for this example application can be found in the directory:
examples\Graphics\Embedding
It may be in the directory in which you installed Symbian OS, or it may
be in src\common\developerlibrary\
. It includes the two project
files needed for building: bld.inf
and the .mmp
file.
The Symbian OS build process describes how to build this application. For the emulator, an
application called EmbeddingShell.exe
is created in
epoc32\release\winscw\<udeb or urel>\
.
Launch the emulator:
epoc32\release\winscw\<urel or udeb>\EPOC.EXE
.
Click on EMBEDDINGSHELL
to run the application. If
using the TechView emulator, this will be in the
Extras menu.
Start the example by choosing Picture
from the menu
List of Programs
.
Step through each phase of the example by pressing the space bar or by tapping on the window drawn by that example.
CPicture
: an abstract base class for drawing pictures
to a graphics context and the storing and restoring of those pictures
MPictureFactory
: a mixin class defining an interface
for instantiating and restoring new CPicture
-derived objects
CDirectFileStore
: a direct file store
RStoreWriteStream
: supports creation and manipulation
of a stream in a store
RStoreReadStream
: supports opening and manipulation of
a stream in a store
TStreamId
: stream identification
TSwizzle
: A device for handling the dual
representation of an object as a streamid or as a pointer
RFs
: file server session