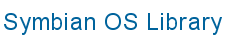
![]() |
![]() |
|
Simple
: simple window system
Found in: examples\Graphics\WS\Simple\
These are the main files contained in the examples. Some extra files may be needed to run the examples, and these will be found in the appropriate examples directory.
Note: This example is designed to work properly only with techview, there is no guarantee that it will work properly with other interfaces
// AppHolder.cpp
//
// Copyright (c) 2005 Symbian Softwares Ltd. All rights reserved.
//
#include "AppHolder.h"
#include "Simple.h"
#include <EikStart.h>
//
// EXPORTed functions
//
EXPORT_C CApaApplication* NewApplication()
{
return new CAppholderApplication;
}
// The below section is added to make the code compatible with v9.1
// This is because only exe files are compatible with v9.1
#if (defined __WINS__ && !defined EKA2) // E32Dll used only when WINS defined and EKA2 not defined
GLDEF_C TInt E32Dll(enum TDllReason)
{
return KErrNone;
}
#else // else E32Main is used
GLDEF_C TInt E32Main()
{
return EikStart::RunApplication(NewApplication);
}
#endif
////////////////////////////////////////////////////////////////
//
// Application class, CAppholderApplication
//
////////////////////////////////////////////////////////////////
TUid CAppholderApplication::AppDllUid() const
{
return KUidAppholder;
}
CApaDocument* CAppholderApplication::CreateDocumentL()
{
// Construct the document using its NewL() function, rather
// than using new(ELeave), because it requires two-phase
// construction.
return new (ELeave) CAppholderDocument(*this);
}
////////////////////////////////////////////////////////////////
//
// Document class, CAppholderDocument
//
////////////////////////////////////////////////////////////////
// C++ constructor
CAppholderDocument::CAppholderDocument(CEikApplication& aApp)
: CEikDocument(aApp)
{
}
CEikAppUi* CAppholderDocument::CreateAppUiL()
{
return new(ELeave) CAppholderAppUi;
}
CAppholderDocument::~CAppholderDocument()
{
}
////////////////////////////////////////////////////////////////
//
// App UI class, CAppholderAppUi
//
////////////////////////////////////////////////////////////////
void CAppholderAppUi::ConstructL()
{
BaseConstructL();
iClient=CExampleWsClient::NewL(ClientRect());
}
CAppholderAppUi::~CAppholderAppUi()
{
delete iClient;
}
void CAppholderAppUi::HandleCommandL(TInt aCommand)
{
switch (aCommand)
{
case EEikCmdExit:
Exit();
break;
}
}
// Base.cpp
//
// Copyright (c) 2005 Symbian Softwares Ltd. All rights reserved.
//
#include <w32std.h>
#include "Base.h"
///////////////////////////////////////////////////////////////////////////////
////////////////////////// CWindow implementation /////////////////////////////
///////////////////////////////////////////////////////////////////////////////
CWindow::CWindow(CWsClient* aClient)
: iClient(aClient)
{
}
void CWindow::ConstructL (const TRect& aRect, CWindow* aParent)
{
// If a parent window was specified, use it; if not, use the window group
// (aParent defaults to 0).
RWindowTreeNode* parent= aParent ? (RWindowTreeNode*) &(aParent->Window()) : &(iClient->iGroup);
iWindow=RWindow(iClient->iWs); // use app's session to window server
User::LeaveIfError(iWindow.Construct(*parent,(TUint32)this));
iRect = aRect;
iWindow.SetExtent(iRect.iTl, iRect.Size()); // set extent relative to group coords
iWindow.Activate(); // window is now active
}
CWindow::~CWindow()
{
iWindow.Close(); // close our window
}
RWindow& CWindow::Window()
{
return iWindow;
}
CWindowGc* CWindow::SystemGc()
{
return iClient->iGc;
}
///////////////////////////////////////////////////////////////////////////////
////////////////////////// CWsRedrawer implementation /////////////////////////
///////////////////////////////////////////////////////////////////////////////
CWsRedrawer::CWsRedrawer()
: CActive(CActive::EPriorityLow)
{
}
void CWsRedrawer::ConstructL(CWsClient* aClient)
{
iClient=aClient; // remember WsClient that owns us
CActiveScheduler::Add(this); // add ourselves to the scheduler
IssueRequest(); // issue request to draw
}
CWsRedrawer::~CWsRedrawer()
{
Cancel();
}
void CWsRedrawer::IssueRequest()
{
iClient->iWs.RedrawReady(&iStatus);
SetActive();
}
void CWsRedrawer::DoCancel()
{
iClient->iWs.RedrawReadyCancel();
}
void CWsRedrawer::RunL()
{
// find out what needs to be done
TWsRedrawEvent redrawEvent;
iClient->iWs.GetRedraw(redrawEvent); // get event
CWindow* window=(CWindow*)(redrawEvent.Handle()); // get window
if (window)
{
TRect rect=redrawEvent.Rect(); // and rectangle that needs redrawing
// now do drawing
iClient->iGc->Activate(window->Window());
window->Window().BeginRedraw(rect);
window->Draw(rect);
window->Window().EndRedraw();
iClient->iGc->Deactivate();
}
// maintain outstanding request
IssueRequest();
}
/////////////////////////////////////////////////////////////////////////////////////
/////////////////////////// CWsClient implementation ////////////////////////////////
/////////////////////////////////////////////////////////////////////////////////////
CWsClient::CWsClient()
: CActive(CActive::EPriorityHigh)
{
}
void CWsClient::ConstructL()
{
// add ourselves to active scheduler
CActiveScheduler::Add(this);
// get a session going
User::LeaveIfError(iWs.Connect());
// construct our one and only window group
iGroup=RWindowGroup(iWs);
User::LeaveIfError(iGroup.Construct(2,ETrue)); // meaningless handle; enable focus
// construct screen device and graphics context
iScreen=new (ELeave) CWsScreenDevice(iWs); // make device for this session
User::LeaveIfError(iScreen->Construct()); // and complete its construction
User::LeaveIfError(iScreen->CreateContext(iGc)); // create graphics context
// construct redrawer
iRedrawer=new (ELeave) CWsRedrawer;
iRedrawer->ConstructL(this);
// construct main window
ConstructMainWindowL();
// request first event and start scheduler
IssueRequest();
}
CWsClient::~CWsClient()
{
// neutralize us as an active object
Deque(); // cancels and removes from scheduler
// get rid of everything we allocated
delete iGc;
delete iScreen;
delete iRedrawer;
// destroy window group
iGroup.Close();
// finish with window server
iWs.Close();
}
void CWsClient::IssueRequest()
{
iWs.EventReady(&iStatus); // request an event
SetActive(); // so we're now active
}
void CWsClient::DoCancel()
{
iWs.EventReadyCancel(); // cancel event request
}
void CWsClient::ConstructMainWindowL()
{
}
// Simple.CPP
//
// Copyright (c) 2005 Symbian Softwares Ltd. All rights reserved.
//
#include <w32std.h>
#include "Base.h"
#include "Simple.h"
//////////////////////////////////////////////////////////////////////////////
// Implementation for derived window classes
//////////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////////
// CMainWindow implementation //
//////////////////////////////////////////////////////////////////////////////
/****************************************************************************\
| Function: Constructor/Destructor for CMainWindow
| Doesn't do much, as most initialisation is done by the
| CWindow base class.
| Input: aClient Client application that owns the window
\****************************************************************************/
CMainWindow::CMainWindow (CWsClient* aClient)
: CWindow (aClient)
{
}
CMainWindow::~CMainWindow ()
{
}
/****************************************************************************\
| Function: CMainWindow::Draw
| Purpose: Redraws the contents of CMainWindow within a given
| rectangle. As CMainWindow has no contents, it simply
| clears the redraw area. A blank window could be used here
| instead. The Clear() is needed because a redraw should
| always draw to every pixel in the redraw rectangle.
| Input: aRect Rectangle that needs redrawing
| Output: None
\****************************************************************************/
void CMainWindow::Draw(const TRect& aRect)
{
CWindowGc* gc=SystemGc(); // get a gc
gc->SetClippingRect(aRect); // clip outside this rect
gc->Clear(aRect); // clear
}
/****************************************************************************\
| Function: CMainWindow::HandlePointerEvent
| Purpose: Handles pointer events for CMainWindow. Doesn't do
| anything here.
| Input: aPointerEvent The pointer event
| Output: None
\****************************************************************************/
void CMainWindow::HandlePointerEvent (TPointerEvent& /*aPointerEvent*/)
{
}
//////////////////////////////////////////////////////////////////////////////
// CSmallWindow implementation
//////////////////////////////////////////////////////////////////////////////
/****************************************************************************\
| Function: Constructor/Destructor for CSmallWindow
| Doesn't do much, as most initialisation is done by the
| CWindow base class.
| Input: aClient Client application that owns the window
\****************************************************************************/
CSmallWindow::CSmallWindow (CWsClient* aClient)
: CWindow (aClient)
{
}
CSmallWindow::~CSmallWindow ()
{
iWindow.Close();
}
/****************************************************************************\
| Function: CSmallWindow::Draw
| Purpose: Redraws the contents of CSmallWindow within a given
| rectangle. CSmallWindow displays a square border around
| the edges of the window, and two diagonal lines between the
| corners.
| Input: aRect Rectangle that needs redrawing
| Output: None
\****************************************************************************/
void CSmallWindow::Draw(const TRect& aRect)
{
// Drawing to a window is done using functions supplied by
// the graphics context (CWindowGC), not the window.
CWindowGc* gc=SystemGc(); // get a gc
gc->SetClippingRect(aRect); // clip outside this rect
gc->Clear(aRect); // clear
TSize size=iWindow.Size();
TInt width=size.iWidth;
TInt height=size.iHeight;
// Draw a square border
gc->DrawLine(TPoint(0,0),TPoint(0,height-1));
gc->DrawLine (TPoint (0, height-1), TPoint (width-1, height-1));
gc->DrawLine(TPoint(width-1,height-1),TPoint(width-1,0));
gc->DrawLine (TPoint (width-1, 0), TPoint (0, 0));
// Draw a line between the corners of the window
gc->DrawLine(TPoint(0,0),TPoint(width, height));
gc->DrawLine (TPoint (0, height), TPoint (width, 0));
}
/****************************************************************************\
| Function: CSmallWindow::HandlePointerEvent
| Purpose: Handles pointer events for CSmallWindow. Doesn't do
| anything here.
| Input: aPointerEvent The pointer event
| Output: None
\****************************************************************************/
void CSmallWindow::HandlePointerEvent (TPointerEvent& /*aPointerEvent*/)
{
}
//////////////////////////////////////////////////////////////////////////////
// CExampleWsClient implementation //
//////////////////////////////////////////////////////////////////////////////
CExampleWsClient* CExampleWsClient::NewL(const TRect& aRect)
{
// make new client
CExampleWsClient* client=new (ELeave) CExampleWsClient(aRect);
CleanupStack::PushL(client); // push, just in case
client->ConstructL(); // construct and run
CleanupStack::Pop();
return client;
}
/****************************************************************************\
| Function: Constructor/Destructor for CExampleWsClient
| Destructor deletes everything that was allocated by
| ConstructMainWindowL()
\****************************************************************************/
CExampleWsClient::CExampleWsClient(const TRect& aRect)
:iRect(aRect)
{
}
CExampleWsClient::~CExampleWsClient ()
{
delete iMainWindow;
delete iSmallWindow;
}
/****************************************************************************\
| Function: CExampleWsClient::ConstructMainWindowL()
| Called by base class's ConstructL
| Purpose: Allocates and creates all the windows owned by this client
| (See list of windows in CExampleWsCLient declaration).
\****************************************************************************/
void CExampleWsClient::ConstructMainWindowL()
{
iMainWindow=new (ELeave) CMainWindow(this);
iMainWindow->ConstructL(iRect);
iSmallWindow = new (ELeave) CSmallWindow (this);
iSmallWindow->ConstructL (TRect (TPoint (100, 75), TSize (50, 50)), iMainWindow);
}
/****************************************************************************\
| Function: CExampleWsClient::RunL()
| Called by active scheduler when an even occurs
| Purpose: Processes events according to their type
| For key events: calls HandleKeyEventL() (global to client)
| For pointer event: calls HandlePointerEvent() for window
| event occurred in.
\****************************************************************************/
void CExampleWsClient::RunL()
{
// get the event
iWs.GetEvent(iWsEvent);
TInt eventType=iWsEvent.Type();
// take action on it
switch (eventType)
{
// window-group related event types
case EEventNull:
break;
case EEventKey:
{
TKeyEvent& keyEvent=*iWsEvent.Key(); // get key event
HandleKeyEventL (keyEvent);
break;
}
case EEventKeyUp:
case EEventKeyDown:
case EEventModifiersChanged:
case EEventFocusLost:
case EEventFocusGained:
case EEventSwitchOn:
case EEventPassword:
case EEventWindowGroupsChanged:
case EEventErrorMessage:
break;
// window related events
case EEventPointer:
{
CWindow* window=(CWindow*)(iWsEvent.Handle()); // get window
TPointerEvent& pointerEvent=*iWsEvent.Pointer();
window->HandlePointerEvent (pointerEvent);
break;
}
case EEventPointerEnter:
case EEventPointerExit:
case EEventPointerBufferReady:
case EEventDragDrop:
break;
default:
break;
}
IssueRequest(); // maintain outstanding request
}
void CExampleWsClient::HandleKeyEventL(struct TKeyEvent& /*aKeyEvent*/)
{
}
// AppHolder.h
//
#ifndef __APPHOLDER_H
#define __APPHOLDER_H
#include <coeccntx.h>
#include <eikenv.h>
#include <eikappui.h>
#include <eikapp.h>
#include <eikdoc.h>
#include <eikmenup.h>
#include <eikon.hrh>
const TUid KUidAppholder = { 0x100098e2 };
class CWsClient;
//
// CAppholderAppUi
//
class CAppholderAppUi : public CEikAppUi
{
public:
void ConstructL();
~CAppholderAppUi();
private: // from CEikAppUi
void HandleCommandL(TInt aCommand);
private:
CWsClient* iClient;
};
//
// CAppholderDocument
//
class CAppholderDocument : public CEikDocument
{
public:
// construct/destruct
CAppholderDocument(CEikApplication& aApp);
~CAppholderDocument();
private: // from CEikDocument
CEikAppUi* CreateAppUiL();
};
//
// CAppholderApplication
//
class CAppholderApplication : public CEikApplication
{
private: // from CApaApplication
CApaDocument* CreateDocumentL();
TUid AppDllUid() const;
};
#endif
// Base.h
//
// Copyright (c) 2005 Symbian Softwares Ltd. All rights reserved.
//
#if !defined(__SimpleWindowServerApp_H__)
#define __SimpleWindowServerApp_H__
// Forward declarations
class CWsRedrawer;
class CWindow;
/////////////////////////////////////////////////////////////////////////
////////////////////// Declaration of CWsClient /////////////////////////
/////////////////////////////////////////////////////////////////////////
// Base class for all windows
class CWsClient : public CActive
{
public:
void ConstructL();
// destruct
~CWsClient();
// main window
virtual void ConstructMainWindowL();
// terminate cleanly
void Exit();
// active object protocol
void IssueRequest(); // request an event
void DoCancel(); // cancel the request
virtual void RunL() = 0; // handle completed request
virtual void HandleKeyEventL (TKeyEvent& aKeyEvent) = 0;
protected:
//construct
CWsClient();
CWsScreenDevice* iScreen;
CWsRedrawer* iRedrawer;
RWsSession iWs;
TWsEvent iWsEvent;
private:
RWindowGroup iGroup;
CWindowGc* iGc;
friend class CWsRedrawer; // needs to get at session
friend class CWindow; // needs to get at session
};
////////////////////////////////////////////////////////////////////////////
//////////////////////// CWsRedrawer declaration ///////////////////////////
////////////////////////////////////////////////////////////////////////////
class CWsRedrawer : public CActive
{
public:
// construct/destruct
CWsRedrawer();
void ConstructL(CWsClient* aClient);
~CWsRedrawer();
// drawing
void IssueRequest();
void DoCancel();
void RunL();
protected:
CWsClient* iClient;
};
//////////////////////////////////////////////////////////////////////////////
///////////////////////// CWindow declaration ////////////////////////////////
//////////////////////////////////////////////////////////////////////////////
class CWindow : public CBase
{
public:
CWindow(CWsClient* aClient);
void ConstructL (const TRect& aRect, CWindow* aParent=0);
~CWindow();
// access
RWindow& Window(); // our own window
CWindowGc* SystemGc(); // system graphics context
// drawing
virtual void Draw(const TRect& aRect) = 0;
virtual void HandlePointerEvent (TPointerEvent& aPointerEvent) = 0;
protected:
RWindow iWindow; // window server window
TRect iRect; // rectangle re owning window
private:
CWsClient* iClient; // client including session and group
};
#endif
// Simple.h
//
// Copyright (c) 2005 Symbian Softwares Ltd. All rights reserved.
//
#if !defined(__WSWIN1_H__)
#define __WSWIN1_H__
#include "Base.h"
//////////////////////////////////////////////////////////////////////////
// Derived window classes //
//////////////////////////////////////////////////////////////////////////
class CMainWindow : public CWindow
{
public:
CMainWindow (CWsClient* aClient);
~CMainWindow ();
void Draw (const TRect& aRect);
void HandlePointerEvent (TPointerEvent& aPointerEvent);
};
class CSmallWindow : public CWindow
{
public:
CSmallWindow (CWsClient* aClient);
~CSmallWindow ();
void Draw (const TRect& aRect);
void HandlePointerEvent (TPointerEvent& aPointerEvent);
};
//////////////////////////////////////////////////////////////////////////
// Derived client class //
//////////////////////////////////////////////////////////////////////////
class CExampleWsClient : public CWsClient
{
public:
static CExampleWsClient* NewL(const TRect& aRect);
void HandleKeyEventL(struct TKeyEvent &aKeyEvent);
private:
// constructor
CExampleWsClient (const TRect& aRect);
void ConstructMainWindowL();
~CExampleWsClient ();
void RunL ();
private:
CMainWindow* iMainWindow; // main window
CMainWindow* iAnotherWindow;
CSmallWindow* iSmallWindow;
const TRect& iRect;
};
#endif
// Simple.mmp
//
// Copyright (c) 2005 Symbian Softwares Ltd. All rights reserved.
//
// From 9.1 builds supporting EKA2 with Winscw, os will treat all
// applications with the extension exe.
TARGET Simple.exe
TARGETTYPE exe
UID 0x100098e2
VENDORID 0x70000001
SOURCEPATH .
SOURCE AppHolder.cpp Base.cpp Simple.cpp
USERINCLUDE .
SYSTEMINCLUDE \epoc32\include
SYSTEMINCLUDE \epoc32\include\techview
// To support the new resource compilation practice from 9.1
// provide the following details to generate .rss files
START RESOURCE Simple.rss
TARGET Simple.rsc
TARGETPATH \Resource\Apps
HEADER
LANG 01 // Build English language versions
END
// In v8.1, both aif files and registration files are supported,
// but from v9.0 onwards, only registration files are supported.
start resource Simple_reg.rss
targetpath \private\10003a3f\apps
//lang 01
end
// To support localisation for the UI applicatons
start resource Simple_loc.rss
//targetpath \resource\apps
lang 01
end
LIBRARY euser.lib
LIBRARY apparc.lib
LIBRARY cone.lib
LIBRARY eikcore.lib
LIBRARY ws32.lib
// Simple.rss
//
NAME MEAD
#include <eikon.rh>
#include <eikcore.rsg>
RESOURCE RSS_SIGNATURE { }
RESOURCE TBUF
{
buf = "";
}
RESOURCE EIK_APP_INFO
{
menubar = r_appholder_menubar;
hotkeys = r_appholder_hotkeys;
}
RESOURCE HOTKEYS r_appholder_hotkeys
{
control =
{
HOTKEY
{
command = EEikCmdExit;
key = 'e';
}
};
}
RESOURCE MENU_BAR r_appholder_menubar
{
titles =
{
MENU_TITLE
{
menu_pane = r_appholder_file_menu;
txt = "File";
}
};
}
RESOURCE MENU_PANE r_appholder_file_menu
{
items =
{
MENU_ITEM
{
command = EEikCmdExit;
txt = "Close";
}
};
}
#include <appinfo.rh>
RESOURCE LOCALISABLE_APP_INFO
{
short_caption = "Simple";
caption_and_icon =
{
CAPTION_AND_ICON_INFO
{
caption = "Simple";
number_of_icons = 0; // each icon must be a bitmap/mask pair
}
};
}
// Simple.RSS
//
// Copyright (c) 2005 Symbian Software Ltd. All rights reserved.
//
#include <appinfo.rh>
UID2 KUidAppRegistrationResourceFile
UID3 0x100098e2
RESOURCE APP_REGISTRATION_INFO
{
app_file = Simple;
localisable_resource_file="\\resource\\apps\\Simple_loc.rss";
hidden=KAppNotHidden;
embeddability=KAppNotEmbeddable;
newfile=KAppDoesNotSupportNewFile;
launch=KAppLaunchInForeground;
}
Simple
creates two windows. a main window which has
nothing drawn to it, and a smaller window with a border and two diagonal lines.
The main window is the parent of the smaller window.
CMainWindow
: An empty window. Just draws itself.
CSmallWindow
: A small window which draws itself with a
square border around the edges of the window, and two diagonal lines between
the corners.
CExampleWsClient
: Derived from CWsClient
.
Owns the CMainWindow
and the CSmallWindow
. Processes
key and pointer events.
CWindowGc
: Window graphics context. Required when
drawing to a window.
struct TPointerEvent
: Pointer event details
struct TKeyEvent
: Key event details