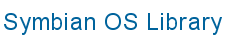
![]() |
![]() |
|
Conversion from one data type to another is performed by a class derived
from the abstract CConverterBase2
class. Conversions can
be performed between files, specified by filenames, or between objects which
support the stream interfaces, e.g. stream stores, memory streams and the
clipboard.
The key CConverterBase2
functions to implement are:
ConvertAL()
, which prepares the converter to
convert data in a file. By default, this leaves with
KErrNotSupported
.
ConvertObjectAL()
, which prepares the converter
to convert data in a stream. By default, this leaves with
KErrNotSupported
.
DoConvertL()
, which does a step in the
conversion and indicates whether the conversion is complete, or whether the
client needs to call DoConvertL()
again. By default this leaves
with KErrNotSupported
.
CancelConvert()
, which can be called at any
stage in the conversion process to cancel the conversion. By default, this does
nothing.
The approach of an initial preparatory step followed by a sequence of further incremental steps allows the client thread to stay responsive while a potentially lengthy conversion process is taking place.
CConverterBase2
also defines two functions which do the
complete conversion in one function call: ConvertL()
and
ConvertObjectL()
. CConverterBase2
supplies
default implementations of these functions which perform their tasks by calling
ConvertAL()
/ConvertObjectAL()
once and
DoConvertL()
in a loop until complete.
A converter must also implement :
Uid()
to return a UID that uniquely identifies
the converter. This should be the same value as the conv_uid
value
defined in the converter's CONARC_RESOURCE_FILE
resource.
Capabilities()
to return a flag indicating if
the converter can convert files, streams, or both.
This is an example declaration of a converter class:
class CExampleConverter : public CConverterBase2
{
public: static CConverterBase2* NewL();
CExampleConverter();
~CExampleConverter();
// from CConverterBase2
void ConvertObjectAL(RReadStream& aReadStream, RWriteStream& aWriteStream, MConverterUiObserver* aObserver=NULL);
TBool DoConvertL();
TUid Uid();
TInt Capabilities();
void CancelConvert();
private:
RReadStream* iReadStream;
RWriteStream* iWriteStream;
};
The MConverterUiObserver
observer pointer can be
NULL
, so check for this before calling its functions.