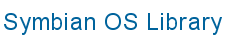
![]() |
![]() |
|
Both an RHTTPSession
and an
RHTTPTransaction
can have associated with them a set of
properties. Properties take the form of name-value pairs, where the name is an
RStringF
and the value is a
THTTPHdrVal
, allowing integers, strings, URIs and dates to
be stored.
Session properties are called Connection Information in the API since
they mainly relate to the type of connection that is made between the client
and an HTTP server. They are accessed using the
RHTTPSession::ConnectionInfo()
method, which returns an
RHTTPConnectionInfo
handle.
Note that in general, session properties should be defined before the first transction is created; filters are not obliged to check with the connection info if the values have changed after this point. However, some exceptions are allowable with HTTP version and proxy configuration.
By default, HTTP sessions will use HTTP/1.1. To use HTTP/1.0 the
connection info property HTTP::EHTTPVersion
must be set to
HTTP::EHttp10
.
From HTTPEXAMPLECLIENT
:
switch(cmd)
{
case EVersion10:
SetHttpVersion(HTTP::EHttp10);
break;
case EVersion11:
SetHttpVersion(HTTP::EHttp11);
break;
...
void CHttpClient::SetHttpVersion(HTTP::TStrings aHttpVersion)
{
RHTTPConnectionInfo connInfo = iSess.ConnectionInfo();
RStringPool p=iSess.StringPool();
connInfo.SetPropertyL(p.StringF(HTTP::EHTTPVersion,RHTTPSession::GetTable()),THTTPHdrVal(p.StringF(aHttpVersion)));
}
The version chosen will apply to all new transactions following the property change, but will not affect any transactions currently in progress.
Since HTTP/1.1 recommends the use of a persistent connection for requests made in series to a single origin server, the default behaviour of HTTP Client is to set up persistent connections for each transaction. This can be overridden by specifing a Connection header in the client request.
By default, a new RHTTPSession
will be set to make
direct connections to an origin server. To switch it to use a proxy, two
properties must be set: HTTP::EProxyUsage
and
HTTP::EProxyAddress
.
HTTP::EProxyUsage
can take the values
HTTP::EDoNotUseProxy
(the default) or
HTTP::EUseProxy
.
HTTP::EProxyAddress
must be set using an
RStringF
value that contains the proxy address as an IP
Address and port number separated by a colon, e.g. "10.158.7.4:9003"
void CHttpClient::SetProxyL(RStringF aProxyAddr)
{
RHTTPConnectionInfo connInfo = iSess.ConnectionInfo();
THTTPHdrVal proxyUsage(iSess.StringPool().StringF(HTTP::EUseProxy,RHTTPSession::GetTable()));
connInfo.SetPropertyL(iSess.StringPool().StringF(HTTP::EProxyUsage,RHTTPSession::GetTable()), proxyUsage);
THTTPHdrVal proxyAddr(aProxyAddr);
connInfo.SetPropertyL(iSess.StringPool().StringF(HTTP::EProxyAddress,RHTTPSession::GetTable()), aProxyAddr);
Printf(_L("Proxy set to:%S\n\n"), &iProxyName);
}
Again, changes made to the proxy settings will affect only new transactions opened on the session, not any currently in progress.
A proxy setting can also be set for an individual transaction by setting the proxy properties on the transaction. Proxy properties set on the transaction override any proxy properties set on the session.
Some tuning can be attempted to optimise HTTP comms performance. The
session properties HTTP::EMaxNumTransportHandlers
and
HTTP::EMaxNumTransPerTranspHndlr
determine how the
internal queueing works.
HTTP::EMaxNumTransportHandlers
determines the maximum
number of sockets that may be open at a time. Its default value is 4.
HTTP::EMaxNumTransPerTranspHndlr
determines how many
transactions may be allocated to a given transport handler (i.e. socket) before
being backed up in a pending queue. Its default value is 5.
Transaction properties are accessed using the
RHTTPTransaction::PropertySet()
method, which returns an
RHTTPTransactionPropertySet
handle. They are used as a
means of storing information related to a transaction that is required to
persist during the transaction lifetime. Such information is not transmitted,
the mechanism merely provides a useful association, mainly used by filter
developers.
Two pre-defined properties that may be set by the client are
HTTP::EUsername
and HTTP::EPassword
.
Their use is described in Validation filter.
An example of properties in use is taken from the Redirection filter, where a count is maintained of the number of redirections made in response to 300-series HTTP status codes:
// see if this transaction has been redirected before
THTTPHdrVal redirectCountProperty;
if (aTransaction.PropertySet().Property(p.StringF(HTTP::ERedirectCount,RHTTPSession::GetTable()),
redirectCountProperty))
{
__ASSERT_DEBUG(redirectCountProperty.Type() == THTTPHdrVal::KTIntVal, HTTPPanic::Panic(HTTPPanic::EHeaderInvalidType));
redirectCount = redirectCountProperty.Int();
__ASSERT_DEBUG(redirectCount > 0, HTTPPanic::Panic(HTTPPanic::EHeaderInvalidType));
}
// Only redirect a certain number of times, and update the redirect count property of the transaction
if (++redirectCount < KRedirectLimit)
aTransaction.PropertySet().SetPropertyL(p.StringF(HTTP::ERedirectCount,RHTTPSession::GetTable()), redirectCount);