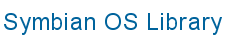
![]() |
![]() |
|
The bitmap blitting functions of the bitmapped graphics context
(CBitmapContext
) cannot be used to perform scaling of the bitmap,
but always draws the bitmap using its dimensions in pixels. The overloaded GDI
CGraphicsContext::DrawBitmap()
function, however, can be used to
draw a bitmap:
stretched or compressed to a set size in twips
stretched and/or compressed to fit a given rectangle
Note that DrawBitmap()
is slower than
BitBlt()
, so BitBlt()
should be used in preference
wherever possible.
A bitmap can have a real world size in twips set for it, and be drawn scaled that size.
Construct a TSize
with the required dimensions in
twips.
Set the size of the bitmap using
CFbsBitmap::SetSizeInTwips()
. The default size in twips of a
bitmap when loaded is (0,0)
.
Draw the bitmap using DrawBitmap()
The example assumes bitmap
is a pointer to a valid
CFbsBitmap
.
// Set twips size
TSize bmpSizeInTwips(2000,2000);
bitmap->SetSizeInTwips(bmpSizeInTwips);
gc.DrawBitmap(TPoint(50,50), bitmap);
DrawBitmap()
can also draw a bitmap by stretching it to
fit a given rectangle.
The example assumes bitmap
is a pointer to a valid
CFbsBitmap
.
// Draw bitmap stretched from the origin to 100,100
TRect rect(0,0,100,100);
gc.DrawBitmap(rect, bitmap);