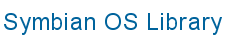
![]() |
![]() |
|
The following example code demonstrates how to:
draw a line a single pixel wide
draw a line with a pen three pixels in diameter
draw a line with a pen thirty pixels wide and 20 pixels high
draw a thin dotted line
draw a thin dot dash line
The code assumes a common pair of pre-defined points are used for each of the line drawing examples:
...
// set up a pair of points for drawing diagonal lines
TPoint startPoint(50,50);
TPoint endPoint(590,190);
...
You can draw a thin line diagonally across the screen using
DrawLine()
. This illustrates how thin a single pixel width line
is, and the visible stepping:
...
// draw a thin line from top left to bottom right
gc.DrawLine(startPoint,endPoint);
...
Use SetPenSize()
to increase the pen size to three
pixels.
Use DrawLine()
to draw a wide line diagonally across
the screen.
// Set up a "bold" size for the pen tip to (default is 1,1)
TSize penSizeBold(3,3);
...
// draw a line from top left to bottom right
gc.SetPenSize(penSizeBold);
gc.DrawLine(startPoint,endPoint);
...
Use SetPenWidth()
to set the pen width to thirty
pixels wide.
Use DrawLine()
to draw a thirty pixel wide line, (x
dimension), diagonally across the screen.
// Set up a "fat" size for the pen tip
TSize penSizeFat(30,30);
...
// draw a rather wide line from top left to bottom right,
// illustrating rounded ends and their clipping
gc.SetPenWidth(penSizeFat);
gc.DrawLine(startPoint,endPoint);
...
the ends of wide lines are rounded
the effective width of the line depends on the size of the pen tip, which is elliptical
the rounded end of a wide line may be clipped to the window, even though the end point is within the window
Use SetPenStyle()
to set the style of the pen to
dotted.
Use DrawLine()
to draw a thin dotted line diagonally
across the screen.
...
// draw a dotted line from top left to bottom right
gc.SetPenStyle(CGraphicsContext::EDottedPen);
gc.DrawLine(startPoint,endPoint);
...
The pen style remains in effect until changed by another call to
SetPenStyle()
or the graphics context is reset to its default
settings.
Use SetPenStyle()
to set the style of the pen to
dot-dashed.
Use DrawLine()
to draw a thin dot-dashed line diagonally
across the screen.
...
// draw a dot dash line from top left to bottom right
gc.SetPenStyle(CGraphicsContext::EDotDashPen);
gc.DrawLine(startPoint,endPoint);
...