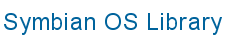
![]() |
![]() |
|
COpenFontFile
Write a class derived from COpenFontFile
to manage a file of the font format supported by the DLL. An object of this type is created by COpenFontRasterizer::NewFontFile()
.
To derive from COpenFontFile
:
Provide implementations for COpenFontFile
's two pure virtual functions: GetNearestFontInPixelsL()
and HasUnicodeCharacterL()
. These are discussed further in the following sections.
During construction call the base class constructor, passing it the arguments aUid
and aFileName
supplied to COpenFontRasterizer::NewFontFileL()
.
During construction, extract the attributes of each font in the font file into a TOpenFontFaceAttrib
. Then add the attributes for each typeface to the typeface array — using COpenFontFile::AddFaceL()
.
This function is called by the Font and Bitmap server to retrieve the font (from the font file) which most closely matches a given font specification. The freetype implementation is given below:
void CFreeTypeFontFile::GetNearestFontInPixelsL(RHeap* aHeap,
COpenFontSessionCacheList* aSessionCacheList,
const TOpenFontSpec& aFontSpec,
TInt aPixelWidth,
TInt aPixelHeight, COpenFont*& aFont,
TOpenFontSpec& aActualFontSpec)
{
// Use the standard matcher.
TInt face_index = 0;
if (GetNearestFontHelper(aFontSpec,aPixelWidth,aPixelHeight,face_index,aActualFontSpec))
{
aFont = CFreeTypeFont::NewL(aHeap,aSessionCacheList,this,face_index,
aActualFontSpec.Height(),
aActualFontSpec.WidthFactor(),
aActualFontSpec.SlantFactor());
}
}
To implement this function:
Call the helper function COpenFontFile::GetNearestFontHelper()
, passing it aDesiredFontSpec
, aPixelWidth
, aPixelHeight
, aActualFontSpec
, and the index of the typeface to search for a match. Note that a font file may contain serveral typefaces, which are accessed
by this index.
This helper function searches the typeface array for the closest matching font and returns ETrue
if a match is found. It also retrieves the matching font's specification into aActualFontSpec
.
Note that the typeface array must be populated from the font file during construction of the COpenFontFile
derived object.
If a valid match was obtained from the helper function (i.e. if it returned ETrue
) create a new COpenFont
object using the specification aActualFontSpec
. Pass the two arguments, aHeap
and aSessionCacheList
to the COpenFont
constructor.
The new object should be returned in aFont
.
If no match is found, aFont
should be set to NULL.
HasUnicodeCharacterL()
HasUnicodeCharacterL()
is called by the Font and Bitmap Server to test whether a font contains a particular Unicode character. The prototype is:
virtual TBool HasUnicodeCharacterL(TInt aFaceIndex,TInt aCode) const = 0;
Implementations must return ETrue
if the character aCode
, which is always a Unicode value, exists in the typeface. There are no other constraints on the way this function is implemented.