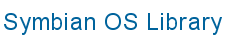
![]() |
![]() |
|
The following example demonstrates how to declare an active object window server event handling class.
Provide a CActive::RunL()
function to handle the completion of an asynchronous request, i.e. an event being received.
Provide a CActive::DoCancel()
function to implement a cancellation request.
Provide a function, here called IssueRequest()
, to issue a request to window server for events.
Create a TWsEvent
object to store events.
/* An active object that wraps a window server session.
An event being received causes RunL() to be called,
where the event is processed. */
class CExampleWsClient : public CActive
{
public:
...
// Active object protocol
void RunL ();
void DoCancel();
// Issue request to window server for events
IssueRequest();
private:
// Access to window server session
RWsSession& iWs;
// Access to screen device
CWsScreenDevice& iScreen;
// Window server general event
TWsEvent iWsEvent;
};
Once the active object has been constructed, a request can be issued. In the following code fragment, the active object provides
the function IssueRequest()
to invoke the encapsulated event request function.
Pass the a TRequestStatus
object, iStatus
, to the EventReady()
function to request window server events from the iWs
window server session.
Use the CActive::SetActive()
function, to indicate that the active object is currently active.
/* Request window server events */
void CExampleWsClient::IssueRequest()
{
iWs.EventReady(&iStatus;); // request an event
SetActive(); // so we're now active
}