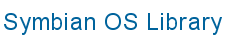
![]() |
![]() |
|
Messaging XML Support provides XML functionality suitable for use in messaging applications. Its primary purpose is to provide SMIL parsing and composing functionality for MMS. However, the component has also been designed to provide support for parsing and composing other types of XML document e.g. XHTML.
There are three libraries that are provided to parse XML and SMIL files. Two provide the more generic XML support:
XMLDOM: support for the DOM classes. This component can be used separately from the XMLParser or with a different XML parser if required.
XMLParser: parsing and composing functionality. It converts XML data to and from the DOM tree structure.
SMILDTD: contains the DTD specific information for SMIL. This component is used in conjunction with the DOM and parser/composer classes to provide the validation for SMIL data.
The end result of the XML parsing is a DOM tree structure. In the case of SMIL, the player can use this DOM tree to render the slides.
CMDXMLParser
is used to generate a DOM tree
from an XML file.
In order to parse XML data, an instance of the parser should be
created. Two types of constructors exist, one type takes a
MXMLDtd
object, while the other does not. If a DTD object
is supplied, then the parser will validate the XML according to that DTD. If no
DTD class is provided, then no validation takes place.
All of the constructors take a
MMDXMLParserObserver
parameter. This interface is usually
implemented by the owning class which then passes itself to the parser
constructor. This interface exists so that the parser can notify its owner when
parsing is complete.
The steps required to parse XML data and get the DOM tree are:
Call CMDXMLParser::ParseFile()
, passing
in the filename of the XML document to be parsed.
When the parsing is complete, the parser will call the
observer's ParseFileCompleteL()
function. This function should
initiate the handling of the DOM tree that has been generated by the
parser.
Call CMDXMLParser::DetachXMLDoc()
to
take ownership of the parsed DOM tree.
Call CMDXMLDocument::DocumentElement()
to get the root element of the DOM tree.
The way in which the DOM tree is used varies depending on the type of XML and even between different clients of the same type of XML. Different SMIL players might walk the DOM tree in slightly different ways; however several functions are likely to be used by all XML clients.
The DOM tree is made up of CMDXMLNode
objects. The document element that is returned by the final step of the
previous section is an example of a CMDXMLNode
.
Use the following functions to navigate the DOM tree:
CMDXMLNode::HasChildNodes()
CMDXMLNode::FirstChild()
CMDXMLNode::LastChild()
CMDXMLNode::NextSibling()
CMDXMLNode::PreviousSibling()
Once a child or sibling node has been located, it is necessary to
cast it to the specific type. The type can be identified by calling
CMDXMLNode::NodeType()
. The following types of node might
exist in a parsed DOM tree. Once the type has been identified, they should be
cast to one of the following:
CMDXMLComment
: Comment data.
CMDXMLProcessingInstruction
: Processing
instruction data.
CMDXMLText
: Text data.
CMDXMLElement
: Element data including
attribute name/value pairs.
CMDXMLDocumentElement
: Represents the
root element in the DOM tree. This is the first node that is accessible after
parsing is complete.
An MMS client may choose to use the SMIL composer to generate the presentation part of outgoing messages, alternatively, it may use template SMIL files. The first option results in a more flexible MMS composer, while the second may be easier to implement.
The steps required to build the DOM tree are:
Create a CMDXMLDocument
object, and pass
in a MXMLDtd
if validation is required. The DTD class can
be omitted if no validation is required.
Call CMDXMLDocument::DocumentElement()
to get the root node of the DOM tree.
Create a child node of the required type, eg.
CMDXMLElement
or
CMDXMLComment
.
Add the node using
CMDXMLNode::AppendChild()
.
The tree can be navigated as above, as more child nodes are added.
Nodes can also be manipulated by using the following functions:
CMDXMLNode::RemoveChild()
CMDXMLNode::InsertBefore()
CMDXMLNode::ReplaceChild()
Once the DOM tree has been built it can be used to generate the XML data. The following steps should be followed to compose the data:
Create an instance of CMDXMLComposer
,
passing in an observer.
Call CMDXMLComposer::ComposeFile()
,
passing in the name of the file where the XML data is to be written.
The composer will call the
ComposeFileCompleteL()
on the observer when it has completed. The
observer can then check the composer to see if the XML has been generated
successfully.
The SMIL DTD class contains the necessary logic for validating SMIL DOM trees. It can be used to validate SMIL data during parsing or composition.
To validate SMIL data as it is parsed, an instance of the SMIL DTD
class should be passed to the CMDXMLParser
on
construction.
To validate SMIL composition, the SMIL DTD class should be passed
to the CMDXMLDocument
on construction.
Messaging XML support was originally designed as a SMIL parser and composer, although it can be used for other types of XML. The only DTD class provided as standard is the SMIL DTD; however it is possible to implement others.
In order to provide support for validating other DTDs, it is
necessary to derive a class from MXMLDtd
.