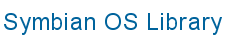
![]() |
![]() |
|
The DML statements are those which modify the data content of the
database, i.e. the INSERT
, UPDATE
and DELETE
statements. These update SQL
statements are effectively run within a transaction and are, therefore, atomic;
either they complete fully or no changes are made to the database. There is no
immediate functional equivalent provided by DBMS, though these operations can
also be performed using an RDbView
object with a suitable query
and update code.
You can use the InsertL()
function to insert a
row:
_LIT(KSelect,"SELECT SupplierName from Suppliers");
_LIT(KSymLim,"Symbian Limited");
...
RDbView view;
view.Prepare(database,KSelect,view.EInsertOnly);
view.InsertL();
view.SetColL(1,KSymLim);
view.PutL();
view.Close();
Alternatively you may use the SQL INSERT
statement:
_LIT(KSQLText,"INSERT INTO Suppliers (SupplierName) VALUES (Symbian Limited)");
...
database.Execute(KSQLText);
You can use the DeleteL()
function to delete a row:
_LIT(KSelect,"SELECT SupplierName from Suppliers WHERE SupplierName=Symbian Limited");
...
database.Begin();
RDbView view;
view.Prepare(database,KSelect);
view.EvaluateAll();
while (view.NextL())
view.DeleteL();
view.Close();
database.Commit();
Alternatively you may use the SQL DELETE
statement:
_LIT(KSQLText,"DELETE FROM Suppliers WHERE SupplierName=Symbian Limited");
...
database.Execute(KSQLText);
For UPDATE
and DELETE
statements where many rows are affected, the SQL forms can be more efficient
than the equivalent C++ functional form; however, INSERT
statements are significantly less efficient than the equivalent C++
code.