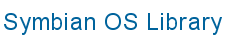
![]() |
![]() |
|
An object definition's instantiation function does not have the usual
new(ELeave)
..PushL()
...ConstructL()
...Pop()
structure. Instead, it delegates construction to a static function
REComSession::CreateImplementationL()
.
There are a number of overloads of the
CreateImplementationL()
function that offer different parameter
options:
a UID that uniquely identifies the interface is always passed. Every implementation uses the same UID in its registration resource file to declares which interface it implements.
the instance identifier key can be passed by reference, or by
passing the offset to the data member (calculated using the
_FOFF
macro).
optionally, resolution data, usually ultimately supplied by the
client, can be passed, which a resolver will use to select the implemenation to
use. This data must be wrapped in a TEComResolverParams
object.
optionally, a UID can be passed to specify a particular resolver to use, if the default resolver is not appropriate.
optionally, a data block can be given for passing to the instantiated object.
An interface definition can have more than one such instantiation function, offering different options to the client.
The following example declares a NewL()
function that
takes a string to use for resolution from the client, wraps this in a
TEComResolverParams
, and calls
REComSession::CreateImplementationL()
to get an instance.
Typically, the NewL()
function is static, and means that
you need to pass the offset to the data member that is to contain the instance
identifier key. This offset value is passed as the second parameter in the call
to REComSession::CreateImplementationL()
, which then puts the
instance identifier key value into that position in the returned object (which
is cast as a CExampleInterfaceDefinition
type).
inline CExampleInterfaceDefinition* CExampleInterfaceDefinition::NewL(const TDesC& aMatchString)
{
const TUid KExampleInterfaceDefinitionUid = {0x10009DC0};
TEComResolverParams resolverParams;
resolverParams.SetDataType(aMatchString);
TAny* ptr = REComSession::CreateImplementationL(
KExampleInterfaceUid,
_FOFF(CExampleInterfaceDefinition,iDtor_ID_Key),
resolverParams);
return REINTERPRET_CAST(CExampleInterfaceDefinition*, ptr);
}
CreateImplementationL()
returns a TAny*
(i.e. a void*
), so this must be cast to the actual pointer
type.