csArray< T, ElementHandler, MemoryAllocator, CapacityHandler > Class Template Reference
[Containers]
A templated array class.
More...
#include <csutil/array.h>
Inheritance diagram for csArray< T, ElementHandler, MemoryAllocator, CapacityHandler >:
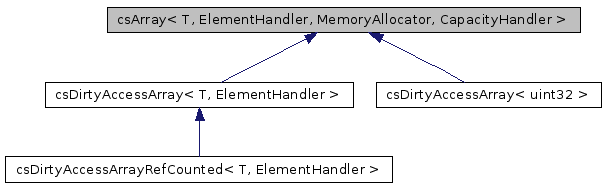
Public Types | |
typedef MemoryAllocator | AllocatorType |
typedef CapacityHandler | CapacityHandlerType |
typedef ElementHandler | ElementHandlerType |
typedef csArray< T, ElementHandler, MemoryAllocator, CapacityHandler > | ThisType |
typedef T | ValueType |
Public Member Functions | |
size_t | Capacity () const |
Query vector capacity. Note that you should rarely need to do this. | |
size_t | Contains (T const &which) const |
An alias for Find() which may be considered more idiomatic by some. | |
csArray (const csArray &source) | |
Copy constructor. | |
csArray (size_t in_capacity, const MemoryAllocator &alloc, const CapacityHandler &ch) | |
Initialize object to have initial capacity of in_capacity elements and with specific memory allocator and capacity handler initializations. | |
csArray (size_t in_capacity=0, const CapacityHandler &ch=CapacityHandler()) | |
Initialize object to have initial capacity of in_capacity elements. | |
bool | Delete (T const &item) |
Delete the given element from the array. | |
void | DeleteAll () |
Clear entire array, releasing all allocated memory. | |
bool | DeleteFast (T const &item) |
Delete the given element from the array. | |
bool | DeleteIndex (size_t n) |
Delete an element from the array. | |
bool | DeleteIndexFast (size_t n) |
Delete an element from the array in constant-time, regardless of the array's size. | |
bool | DeleteRange (size_t start, size_t end) |
Delete a given range (inclusive). | |
void | Empty () |
Remove all elements. | |
size_t | Find (T const &which) const |
Find an element in array. | |
template<class K> | |
size_t | FindKey (csArrayCmp< T, K > comparekey) const |
Find an element based upon some arbitrary key, which may be embedded within an element, or otherwise derived from it. | |
template<class K> | |
size_t | FindSortedKey (csArrayCmp< T, K > comparekey, size_t *candidate=0) const |
Find an element based on some key, using a comparison function. | |
T const & | Get (size_t n) const |
Get an element (const). | |
T & | Get (size_t n) |
Get an element (non-const). | |
const MemoryAllocator & | GetAllocator () const |
Return a reference to the allocator of this array. | |
T & | GetExtend (size_t n) |
Get an item from the array. | |
size_t | GetIndex (const T *which) const |
Given a pointer to an element in the array this function will return the index. | |
ConstIterator | GetIterator () const |
Returns an Iterator which traverses the array. | |
Iterator | GetIterator () |
Returns an Iterator which traverses the array. | |
size_t | GetSize () const |
Return the number of elements in the array. | |
bool | Insert (size_t n, T const &item) |
Insert element item before element n . | |
size_t | InsertSorted (const T &item, int(*compare)(T const &, T const &)=DefaultCompare, size_t *equal_index=0) |
Insert an element at a sorted position, using an element comparison function. | |
bool | IsEmpty () const |
Return true if the array is empty. | |
size_t | Length () const |
Return the number of elements in the array. | |
bool | operator!= (const csArray &other) const |
csArray< T, ElementHandler, MemoryAllocator, CapacityHandler > & | operator= (const csArray &other) |
Assignment operator. | |
bool | operator== (const csArray &other) const |
Check if this array has the exact same contents as other. | |
T const & | operator[] (size_t n) const |
Get a const reference. | |
T & | operator[] (size_t n) |
Get an element (non-const). | |
T | Pop () |
Pop an element from tail end of array. | |
size_t | Push (T const &what) |
Push a copy of an element onto the tail end of the array. | |
size_t | PushSmart (T const &what) |
Push a element onto the tail end of the array if not already present. | |
void | Put (size_t n, T const &what) |
Insert a copy of element at the indicated position. | |
csArray< T > | Section (size_t low, size_t high) const |
Get the portion of the array between low and high inclusive. | |
void | SetCapacity (size_t n) |
Set vector capacity to approximately n elements. | |
void | SetMinimalCapacity (size_t n) |
Set vector capacity to at least n elements. | |
void | SetSize (size_t n) |
Set the actual number of items in this array. | |
void | SetSize (size_t n, T const &what) |
Set the actual number of items in this array. | |
void | ShrinkBestFit () |
Make the array just as big as it needs to be. | |
void | Sort (int(*compare)(T const &, T const &)=DefaultCompare) |
Sort array using a comparison function. | |
T & | Top () |
Return the top element but do not remove it. (non-const). | |
T const & | Top () const |
Return the top element but do not remove it. (const). | |
void | TransferTo (csArray &destination) |
Transfer the entire contents of one array to the other. | |
void | Truncate (size_t n) |
Truncate array to specified number of elements. | |
~csArray () | |
Destroy array and all contained elements. | |
void | SetLength (size_t n) |
void | SetLength (size_t n, T const &what) |
Set the actual number of items in this array. | |
Static Public Member Functions | |
static int | DefaultCompare (T const &r1, T const &r2) |
Compare two objects of the same type. | |
Protected Member Functions | |
void | InitRegion (size_t start, size_t count) |
Initialize a region. | |
Classes | |
class | ConstIterator |
Iterator for the Array<> class. More... | |
class | Iterator |
Iterator for the Array<> class. More... |
Detailed Description
template<class T, class ElementHandler = csArrayElementHandler<T>, class MemoryAllocator = CS::Memory::AllocatorMalloc, class CapacityHandler = csArrayCapacityDefault>
class csArray< T, ElementHandler, MemoryAllocator, CapacityHandler >
A templated array class.
The objects in this class are constructed via copy-constructor and are destroyed when they are removed from the array or the array is destroyed.
- Note:
- If you want to store reference-counted object pointers, such as iFoo*, then you should consider csRefArray<>, which is more idiomatic than csArray<csRef<iFoo> >.
Definition at line 350 of file array.h.
Constructor & Destructor Documentation
csArray< T, ElementHandler, MemoryAllocator, CapacityHandler >::csArray | ( | size_t | in_capacity = 0 , |
|
const CapacityHandler & | ch = CapacityHandler() | |||
) | [inline] |
Initialize object to have initial capacity of in_capacity
elements.
The storage increase depends on the specified capacity handler. The default capacity handler accepts a threshold parameter by which the storage is increased each time the upper bound is exceeded.
csArray< T, ElementHandler, MemoryAllocator, CapacityHandler >::csArray | ( | size_t | in_capacity, | |
const MemoryAllocator & | alloc, | |||
const CapacityHandler & | ch | |||
) | [inline] |
csArray< T, ElementHandler, MemoryAllocator, CapacityHandler >::csArray | ( | const csArray< T, ElementHandler, MemoryAllocator, CapacityHandler > & | source | ) | [inline] |
Member Function Documentation
size_t csArray< T, ElementHandler, MemoryAllocator, CapacityHandler >::Capacity | ( | ) | const [inline] |
Query vector capacity. Note that you should rarely need to do this.
Definition at line 545 of file array.h.
Referenced by csArray< unsigned int, csArrayElementHandler< unsigned int > >::SetMinimalCapacity().
size_t csArray< T, ElementHandler, MemoryAllocator, CapacityHandler >::Contains | ( | T const & | which | ) | const [inline] |
static int csArray< T, ElementHandler, MemoryAllocator, CapacityHandler >::DefaultCompare | ( | T const & | r1, | |
T const & | r2 | |||
) | [inline, static] |
Compare two objects of the same type.
- Parameters:
-
r1 Reference to first object. r2 Reference to second object.
- Returns:
- Zero if the objects are equal; less-than-zero if the first object is less than the second; or greater-than-zero if the first object is greater than the second.
- Remarks:
- Assumes the existence of T::operator<(T). This is the default comparison function used by csArray for sorting if the client does not provide a custom function.
bool csArray< T, ElementHandler, MemoryAllocator, CapacityHandler >::Delete | ( | T const & | item | ) | [inline] |
Delete the given element from the array.
- Remarks:
- Performs a linear search of the array to locate
item
, thus it may be slow for large arrays.
Definition at line 1067 of file array.h.
Referenced by csFIFO< T, ElementHandler, MemoryAllocator >::Delete(), and csObjectModel::RemoveListener().
void csArray< T, ElementHandler, MemoryAllocator, CapacityHandler >::DeleteAll | ( | ) | [inline] |
Clear entire array, releasing all allocated memory.
Definition at line 888 of file array.h.
Referenced by csTreeNode::BSF(), csFrameDataHolder< csParticleSystem::PerFrameData >::Clear(), csDirtyAccessArrayRefCounted< T, ElementHandler >::DecRef(), csHash< csEventCord *, csStringID >::DeleteAll(), csFIFO< T, ElementHandler, MemoryAllocator >::DeleteAll(), csArray< unsigned int, csArrayElementHandler< unsigned int > >::operator=(), csArray< unsigned int, csArrayElementHandler< unsigned int > >::ShrinkBestFit(), csArray< unsigned int, csArrayElementHandler< unsigned int > >::TransferTo(), and csArray< unsigned int, csArrayElementHandler< unsigned int > >::~csArray().
bool csArray< T, ElementHandler, MemoryAllocator, CapacityHandler >::DeleteFast | ( | T const & | item | ) | [inline] |
Delete the given element from the array.
- Remarks:
- This is a special version of Delete() which does not preserve the order of the remaining elements. This characteristic allows deletions to be performed somewhat more quickly than by Delete(), however the speed gain is largely mitigated by the fact that a linear search is performed in order to locate
item
, thus this optimization is mostly illusory.
- Deprecated:
- The speed gain promised by this method is mostly illusory on account of the linear search for the item. In many cases, it will be faster to keep the array sorted, search for
item
using FindSortedKey(), and then remove it using the plain DeleteIndex().
bool csArray< T, ElementHandler, MemoryAllocator, CapacityHandler >::DeleteIndex | ( | size_t | n | ) | [inline] |
Delete an element from the array.
return True if the indicated item index was valid, else false.
- Remarks:
- Deletion speed is proportional to the size of the array and the location of the element being deleted. If the order of the elements in the array is not important, then you can instead use DeleteIndexFast() for constant-time deletion.
Definition at line 995 of file array.h.
Referenced by csTreeNode::BSF(), csArray< unsigned int, csArrayElementHandler< unsigned int > >::Delete(), scfStringArray::DeleteIndex(), and csTreeNode::RemoveChild().
bool csArray< T, ElementHandler, MemoryAllocator, CapacityHandler >::DeleteIndexFast | ( | size_t | n | ) | [inline] |
Delete an element from the array in constant-time, regardless of the array's size.
return True if the indicated item index was valid, else false.
- Remarks:
- This is a special version of DeleteIndex() which does not preserve the order of the remaining elements. This characteristic allows deletions to be performed in constant-time, regardless of the size of the array.
Definition at line 1020 of file array.h.
Referenced by csHash< csEventCord *, csStringID >::Delete(), csHash< csEventCord *, csStringID >::DeleteAll(), and csArray< unsigned int, csArrayElementHandler< unsigned int > >::DeleteFast().
bool csArray< T, ElementHandler, MemoryAllocator, CapacityHandler >::DeleteRange | ( | size_t | start, | |
size_t | end | |||
) | [inline] |
void csArray< T, ElementHandler, MemoryAllocator, CapacityHandler >::Empty | ( | ) | [inline] |
Remove all elements.
Similar to DeleteAll(), but does not release memory used by the array itself, thus making it more efficient for cases when the number of contained elements will fluctuate.
Definition at line 928 of file array.h.
Referenced by scfStringArray::Empty(), and csFIFO< T, ElementHandler, MemoryAllocator >::PopTop().
size_t csArray< T, ElementHandler, MemoryAllocator, CapacityHandler >::Find | ( | T const & | which | ) | const [inline] |
Find an element in array.
- Returns:
- csArrayItemNotFound if not found, else the item index.
- Warning:
- Performs a slow linear search. For faster searching, sort the array and then use FindSortedKey().
Definition at line 851 of file array.h.
Referenced by csArray< unsigned int, csArrayElementHandler< unsigned int > >::Contains(), csArray< unsigned int, csArrayElementHandler< unsigned int > >::Delete(), csArray< unsigned int, csArrayElementHandler< unsigned int > >::DeleteFast(), csArray< unsigned int, csArrayElementHandler< unsigned int > >::PushSmart(), and csTreeNode::RemoveChild().
size_t csArray< T, ElementHandler, MemoryAllocator, CapacityHandler >::FindKey | ( | csArrayCmp< T, K > | comparekey | ) | const [inline] |
Find an element based upon some arbitrary key, which may be embedded within an element, or otherwise derived from it.
The incoming key functor defines the relationship between the key and the array's element type.
- Returns:
- csArrayItemNotFound if not found, else item index.
size_t csArray< T, ElementHandler, MemoryAllocator, CapacityHandler >::FindSortedKey | ( | csArrayCmp< T, K > | comparekey, | |
size_t * | candidate = 0 | |||
) | const [inline] |
T const& csArray< T, ElementHandler, MemoryAllocator, CapacityHandler >::Get | ( | size_t | n | ) | const [inline] |
T& csArray< T, ElementHandler, MemoryAllocator, CapacityHandler >::Get | ( | size_t | n | ) | [inline] |
Get an element (non-const).
Definition at line 627 of file array.h.
Referenced by scfStringArray::Get(), csArray< T, ElementHandler, MemoryAllocator, CapacityHandler >::ConstIterator::Next(), csArray< T, ElementHandler, MemoryAllocator, CapacityHandler >::Iterator::Next(), csArray< unsigned int, csArrayElementHandler< unsigned int > >::operator==(), csArray< unsigned int, csArrayElementHandler< unsigned int > >::operator[](), and csTreeNode::~csTreeNode().
const MemoryAllocator& csArray< T, ElementHandler, MemoryAllocator, CapacityHandler >::GetAllocator | ( | ) | const [inline] |
T& csArray< T, ElementHandler, MemoryAllocator, CapacityHandler >::GetExtend | ( | size_t | n | ) | [inline] |
size_t csArray< T, ElementHandler, MemoryAllocator, CapacityHandler >::GetIndex | ( | const T * | which | ) | const [inline] |
Given a pointer to an element in the array this function will return the index.
Note that this function does not check if the returned index is actually valid. The caller of this function is responsible for testing if the returned index is within the bounds of the array.
ConstIterator csArray< T, ElementHandler, MemoryAllocator, CapacityHandler >::GetIterator | ( | ) | const [inline] |
size_t csArray< T, ElementHandler, MemoryAllocator, CapacityHandler >::GetSize | ( | ) | const [inline] |
Return the number of elements in the array.
Definition at line 529 of file array.h.
Referenced by csFrameDataHolder< csParticleSystem::PerFrameData >::GetUnusedData(), csArray< unsigned int, csArrayElementHandler< unsigned int > >::IsEmpty(), csArray< unsigned int, csArrayElementHandler< unsigned int > >::Length(), and csArray< unsigned int, csArrayElementHandler< unsigned int > >::operator==().
void csArray< T, ElementHandler, MemoryAllocator, CapacityHandler >::InitRegion | ( | size_t | start, | |
size_t | count | |||
) | [inline, protected] |
bool csArray< T, ElementHandler, MemoryAllocator, CapacityHandler >::Insert | ( | size_t | n, | |
T const & | item | |||
) | [inline] |
Insert element item
before element n
.
Definition at line 751 of file array.h.
Referenced by scfStringArray::Insert(), and csArray< unsigned int, csArrayElementHandler< unsigned int > >::InsertSorted().
size_t csArray< T, ElementHandler, MemoryAllocator, CapacityHandler >::InsertSorted | ( | const T & | item, | |
int(*)(T const &, T const &) | compare = DefaultCompare , |
|||
size_t * | equal_index = 0 | |||
) | [inline] |
Insert an element at a sorted position, using an element comparison function.
- Parameters:
-
item The item to insert. compare [optional] Pointer to a function to compare two elements. equal_index [optional] Returns the index of an element equal to the one to be inserted, if one is found. csArrayItemNotFound otherwise.
- Returns:
- The index of the inserted item.
- Remarks:
- The array must be sorted.
bool csArray< T, ElementHandler, MemoryAllocator, CapacityHandler >::IsEmpty | ( | ) | const [inline] |
Return true if the array is empty.
- Remarks:
- Rigidly equivalent to
return GetSize() == 0
, but more idiomatic.
Definition at line 938 of file array.h.
Referenced by scfStringArray::IsEmpty().
size_t csArray< T, ElementHandler, MemoryAllocator, CapacityHandler >::Length | ( | ) | const [inline] |
Return the number of elements in the array.
- Deprecated:
- Use GetSize() instead.
Definition at line 539 of file array.h.
Referenced by csTreeNode::BSF(), csHash< csEventCord *, csStringID >::Contains(), csGetShaderVariableFromStack(), csHash< csEventCord *, csStringID >::Delete(), csHash< csEventCord *, csStringID >::DeleteAll(), csTreeNode::DSF(), csArray< unsigned int, csArrayElementHandler< unsigned int > >::Find(), csArray< unsigned int, csArrayElementHandler< unsigned int > >::FindKey(), csArray< unsigned int, csArrayElementHandler< unsigned int > >::FindSortedKey(), csObjectModel::FireListeners(), csHash< csEventCord *, csStringID >::Get(), csHash< csEventCord *, csStringID >::GetAll(), csHash< csEventCord *, csStringID >::GetElementPointer(), scfStringArray::GetSize(), csArray< T, ElementHandler, MemoryAllocator, CapacityHandler >::ConstIterator::HasNext(), csArray< T, ElementHandler, MemoryAllocator, CapacityHandler >::Iterator::HasNext(), csArray< unsigned int, csArrayElementHandler< unsigned int > >::InsertSorted(), csTreeNode::IsLeaf(), csFIFO< T, ElementHandler, MemoryAllocator >::Length(), csFIFO< T, ElementHandler, MemoryAllocator >::PopTop(), csArray< unsigned int, csArrayElementHandler< unsigned int > >::Push(), csHash< csEventCord *, csStringID >::Put(), csHash< csEventCord *, csStringID >::PutUnique(), csArray< unsigned int, csArrayElementHandler< unsigned int > >::SetCapacity(), csArray< unsigned int, csArrayElementHandler< unsigned int > >::SetMinimalCapacity(), csArray< unsigned int, csArrayElementHandler< unsigned int > >::SetSize(), csArray< unsigned int, csArrayElementHandler< unsigned int > >::Sort(), and csTreeNode::~csTreeNode().
csArray<T,ElementHandler,MemoryAllocator,CapacityHandler>& csArray< T, ElementHandler, MemoryAllocator, CapacityHandler >::operator= | ( | const csArray< T, ElementHandler, MemoryAllocator, CapacityHandler > & | other | ) | [inline] |
bool csArray< T, ElementHandler, MemoryAllocator, CapacityHandler >::operator== | ( | const csArray< T, ElementHandler, MemoryAllocator, CapacityHandler > & | other | ) | const [inline] |
T const& csArray< T, ElementHandler, MemoryAllocator, CapacityHandler >::operator[] | ( | size_t | n | ) | const [inline] |
T& csArray< T, ElementHandler, MemoryAllocator, CapacityHandler >::operator[] | ( | size_t | n | ) | [inline] |
T csArray< T, ElementHandler, MemoryAllocator, CapacityHandler >::Pop | ( | ) | [inline] |
Pop an element from tail end of array.
Reimplemented in csPDelArray< T >, csRefArray< T, Allocator >, csStringArray, csPDelArray< csPluginManager::csPlugin >, csPDelArray< csPixmap >, csPDelArray< csPluginLoadRec >, csPDelArray< csArchive::ArchiveEntry >, csPDelArray< csPluginManager::csPluginOption >, csPDelArray< csRenderMeshList::renderMeshListInfo >, csRefArray< iImage >, csRefArray< csShaderVariable >, csRefArray< iEventHandler >, csRefArray< iSndSysStreamCallback >, csRefArray< iParticle >, csRefArray< iObjectNameChangeListener >, csRefArray< iSprite2DState >, csRefArray< T >, csRefArray< iTextureHandle >, csRefArray< iObjectModelListener >, csRefArray< iMeshObject >, and csRefArray< iConfigFile >.
Definition at line 727 of file array.h.
Referenced by csFIFO< T, ElementHandler, MemoryAllocator >::PopTop().
size_t csArray< T, ElementHandler, MemoryAllocator, CapacityHandler >::Push | ( | T const & | what | ) | [inline] |
Push a copy of an element onto the tail end of the array.
- Returns:
- Index of newly added element.
Definition at line 693 of file array.h.
Referenced by csTreeNode::AddChild(), csObjectModel::AddListener(), csTreeNode::BSF(), csTreeNode::csTreeNode(), csHash< csEventCord *, csStringID >::GetAll(), csFIFO< T, ElementHandler, MemoryAllocator >::PopTop(), scfStringArray::Push(), csFIFO< T, ElementHandler, MemoryAllocator >::Push(), csArray< unsigned int, csArrayElementHandler< unsigned int > >::PushSmart(), csHash< csEventCord *, csStringID >::Put(), and csArray< unsigned int, csArrayElementHandler< unsigned int > >::Section().
size_t csArray< T, ElementHandler, MemoryAllocator, CapacityHandler >::PushSmart | ( | T const & | what | ) | [inline] |
void csArray< T, ElementHandler, MemoryAllocator, CapacityHandler >::Put | ( | size_t | n, | |
T const & | what | |||
) | [inline] |
void csArray< T, ElementHandler, MemoryAllocator, CapacityHandler >::SetCapacity | ( | size_t | n | ) | [inline] |
Set vector capacity to approximately n
elements.
- Remarks:
- Never sets the capacity to fewer than the current number of elements in the array. See Truncate() or SetSize() if you need to adjust the number of actual array elements.
void csArray< T, ElementHandler, MemoryAllocator, CapacityHandler >::SetLength | ( | size_t | n, | |
T const & | what | |||
) | [inline] |
Set the actual number of items in this array.
- Deprecated:
- Use SetSize() instead.
Reimplemented in csPDelArray< T >, csPDelArray< csPluginManager::csPlugin >, csPDelArray< csPixmap >, csPDelArray< csPluginLoadRec >, csPDelArray< csArchive::ArchiveEntry >, csPDelArray< csPluginManager::csPluginOption >, and csPDelArray< csRenderMeshList::renderMeshListInfo >.
void csArray< T, ElementHandler, MemoryAllocator, CapacityHandler >::SetMinimalCapacity | ( | size_t | n | ) | [inline] |
Set vector capacity to at least n
elements.
- Remarks:
- Never sets the capacity to fewer than the current number of elements in the array. See Truncate() or SetSize() if you need to adjust the number of actual array elements. This function will also never shrink the current capacity.
void csArray< T, ElementHandler, MemoryAllocator, CapacityHandler >::SetSize | ( | size_t | n | ) | [inline] |
Set the actual number of items in this array.
This can be used to shrink an array (like Truncate()) or to enlarge an array, in which case it will properly construct all new items using their default (zero-argument) constructor.
- Parameters:
-
n New array length.
void csArray< T, ElementHandler, MemoryAllocator, CapacityHandler >::SetSize | ( | size_t | n, | |
T const & | what | |||
) | [inline] |
Set the actual number of items in this array.
This can be used to shrink an array (like Truncate()) or to enlarge an array, in which case it will properly construct all new items based on the given item.
- Parameters:
-
n New array length. what Object used as template to construct each newly added object using the object's copy constructor when the array size is increased by this method.
Definition at line 579 of file array.h.
Referenced by csArray< unsigned int, csArrayElementHandler< unsigned int > >::GetExtend(), csFrameDataHolder< csParticleSystem::PerFrameData >::GetUnusedData(), csArray< unsigned int, csArrayElementHandler< unsigned int > >::Put(), and csArray< unsigned int, csArrayElementHandler< unsigned int > >::SetLength().
void csArray< T, ElementHandler, MemoryAllocator, CapacityHandler >::ShrinkBestFit | ( | ) | [inline] |
void csArray< T, ElementHandler, MemoryAllocator, CapacityHandler >::Sort | ( | int(*)(T const &, T const &) | compare = DefaultCompare |
) | [inline] |
T& csArray< T, ElementHandler, MemoryAllocator, CapacityHandler >::Top | ( | ) | [inline] |
T const& csArray< T, ElementHandler, MemoryAllocator, CapacityHandler >::Top | ( | ) | const [inline] |
void csArray< T, ElementHandler, MemoryAllocator, CapacityHandler >::TransferTo | ( | csArray< T, ElementHandler, MemoryAllocator, CapacityHandler > & | destination | ) | [inline] |
void csArray< T, ElementHandler, MemoryAllocator, CapacityHandler >::Truncate | ( | size_t | n | ) | [inline] |
Truncate array to specified number of elements.
The new number of elements cannot exceed the current number of elements.
- Remarks:
- Does not reclaim memory used by the array itself, though the removed objects are destroyed. To reclaim the array's memory invoke ShrinkBestFit(), or DeleteAll() if you want to release all allocated resources.
The more general-purpose SetSize() method can also enlarge the array.
Definition at line 912 of file array.h.
Referenced by csArray< unsigned int, csArrayElementHandler< unsigned int > >::Empty(), and csArray< unsigned int, csArrayElementHandler< unsigned int > >::SetSize().
The documentation for this class was generated from the following file:
- csutil/array.h
Generated for Crystal Space by doxygen 1.4.7