csBitArrayTweakable< InlinedBits, Allocator > Class Template Reference
A one-dimensional array of bits, similar to STL bitset. More...
#include <csutil/bitarray.h>
Inheritance diagram for csBitArrayTweakable< InlinedBits, Allocator >:
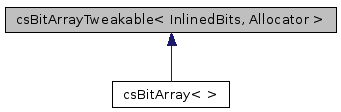
Public Types | |
typedef Allocator | AllocatorType |
typedef csBitArrayTweakable< InlinedBits, Allocator > | ThisType |
Public Member Functions | |
bool | AllBitsFalse () const |
Returns true if all bits are false. | |
bool | AreSomeBitsSet (size_t pos, size_t count) const |
Checks whether at least one of count bits is set starting at pos. | |
void | Clear () |
Set all bits to false. | |
void | ClearBit (size_t pos) |
Set the bit at position pos to false. | |
csBitArrayTweakable (const csBitArrayTweakable &that) | |
Construct as duplicate of that (copy constructor). | |
csBitArrayTweakable (size_t size) | |
Construct with a size of size bits. | |
csBitArrayTweakable () | |
Default constructor. | |
void | Delete (size_t pos, size_t count) |
Delete from the array count bits starting at pos, making the array shorter. | |
csBitArrayTweakable & | FlipAllBits () |
Change value of all bits. | |
void | FlipBit (size_t pos) |
Toggle the bit at position pos. | |
csBitArrayStorageType * | GetArrayBits () |
Return the full backing-store. | |
size_t | GetSize () const |
Return the number of stored bits. | |
bool | IsBitSet (size_t pos) const |
Returns true if the bit at position pos is true. | |
size_t | Length () const |
Return the number of stored bits. | |
csBitArrayTweakable & | operator &= (const csBitArrayTweakable &that) |
Bit-wise `and'. The arrays must be the same length. | |
bool | operator!= (const csBitArrayTweakable &that) const |
Not equal to other array? | |
csBitArrayTweakable & | operator= (const csBitArrayTweakable &that) |
Copy from other array. | |
bool | operator== (const csBitArrayTweakable &that) const |
Equal to other array? | |
bool | operator[] (size_t pos) const |
Return bit at position pos. | |
BitProxy | operator[] (size_t pos) |
Return bit at position pos. | |
csBitArrayTweakable | operator^= (const csBitArrayTweakable &that) |
Bit-wise `xor'. The arrays must be the same length. | |
csBitArrayTweakable | operator|= (const csBitArrayTweakable &that) |
Bit-wise `or'. The arrays must be the same length. | |
csBitArrayTweakable | operator~ () const |
Return complement bit array in which all bits are flipped from this one. | |
void | Set (size_t pos, bool val=true) |
Set the bit at position pos to the given value. | |
void | SetBit (size_t pos) |
Set the bit at position pos to true. | |
void | SetLength (size_t newSize) |
Set the number of stored bits. | |
void | SetSize (size_t newSize) |
Set the number of stored bits. | |
csBitArrayTweakable | Slice (size_t pos, size_t count) const |
Return a new bit array containing a slice count bits in length from this array starting at pos. | |
~csBitArrayTweakable () | |
Destructor. | |
Friends | |
class | BitProxy |
class | csComparatorBitArray |
class | csHashComputerBitArray |
csBitArrayTweakable | operator & (const csBitArrayTweakable &a1, const csBitArrayTweakable &a2) |
Bit-wise `and'. The arrays must be the same length. | |
csBitArrayTweakable | operator^ (const csBitArrayTweakable &a1, const csBitArrayTweakable &a2) |
Bit-wise `xor'. The arrays must be the same length. | |
csBitArrayTweakable | operator| (const csBitArrayTweakable &a1, const csBitArrayTweakable &a2) |
Bit-wise `or'. The arrays must be the same length. | |
Classes | |
class | BitProxy |
Detailed Description
template<int InlinedBits = csBitArrayDefaultInlineBits, typename Allocator = CS::Memory::AllocatorMalloc>
class csBitArrayTweakable< InlinedBits, Allocator >
A one-dimensional array of bits, similar to STL bitset.
The amount of bits is dynamic at runtime.
Internally, bits are stored in multiple values of the type csBitArrayStorageType. If the number of bits is below a certain threshold, the bits are stored in a field inside the class for more performance, above that threshold, the data is stored on the heap.
This threshold can be tweaked by changing the InlinedBits template parameter. At least InlinedBits bits will be stored inlined in the class (the actual amount is the next bigger multiple of the number of bits fitting into one csBitArrayStorageType). In scenarios where you can anticipate that the common number of stored bits is larger than the default number, you can tweak InlinedBits to gain more performance.
The Allocator template allocator allows you to override the logic to allocate bits from the heap.
Definition at line 127 of file bitarray.h.
Constructor & Destructor Documentation
csBitArrayTweakable< InlinedBits, Allocator >::csBitArrayTweakable | ( | ) | [inline] |
Default constructor.
Definition at line 254 of file bitarray.h.
Referenced by csBitArrayTweakable< >::operator~().
csBitArrayTweakable< InlinedBits, Allocator >::csBitArrayTweakable | ( | size_t | size | ) | [inline, explicit] |
csBitArrayTweakable< InlinedBits, Allocator >::csBitArrayTweakable | ( | const csBitArrayTweakable< InlinedBits, Allocator > & | that | ) | [inline] |
csBitArrayTweakable< InlinedBits, Allocator >::~csBitArrayTweakable | ( | ) | [inline] |
Member Function Documentation
bool csBitArrayTweakable< InlinedBits, Allocator >::AllBitsFalse | ( | ) | const [inline] |
bool csBitArrayTweakable< InlinedBits, Allocator >::AreSomeBitsSet | ( | size_t | pos, | |
size_t | count | |||
) | const [inline] |
Checks whether at least one of count bits is set starting at pos.
Definition at line 516 of file bitarray.h.
Referenced by csFixedSizeAllocator< sizeof(T), Allocator >::Compact().
void csBitArrayTweakable< InlinedBits, Allocator >::Clear | ( | ) | [inline] |
void csBitArrayTweakable< InlinedBits, Allocator >::ClearBit | ( | size_t | pos | ) | [inline] |
Set the bit at position pos to false.
Definition at line 485 of file bitarray.h.
Referenced by csBitArrayTweakable< >::Set().
void csBitArrayTweakable< InlinedBits, Allocator >::Delete | ( | size_t | pos, | |
size_t | count | |||
) | [inline] |
Delete from the array count bits starting at pos, making the array shorter.
Definition at line 560 of file bitarray.h.
Referenced by csFixedSizeAllocator< sizeof(T), Allocator >::Compact().
csBitArrayTweakable& csBitArrayTweakable< InlinedBits, Allocator >::FlipAllBits | ( | ) | [inline] |
Change value of all bits.
Definition at line 547 of file bitarray.h.
Referenced by csFixedSizeAllocator< sizeof(T), Allocator >::GetAllocationMap().
void csBitArrayTweakable< InlinedBits, Allocator >::FlipBit | ( | size_t | pos | ) | [inline] |
Toggle the bit at position pos.
Definition at line 492 of file bitarray.h.
Referenced by csBitArrayTweakable< InlinedBits, Allocator >::BitProxy::Flip().
csBitArrayStorageType* csBitArrayTweakable< InlinedBits, Allocator >::GetArrayBits | ( | ) | [inline] |
size_t csBitArrayTweakable< InlinedBits, Allocator >::GetSize | ( | ) | const [inline] |
Return the number of stored bits.
Definition at line 283 of file bitarray.h.
Referenced by csBitArrayTweakable< >::Length().
bool csBitArrayTweakable< InlinedBits, Allocator >::IsBitSet | ( | size_t | pos | ) | const [inline] |
Returns true if the bit at position pos is true.
Definition at line 508 of file bitarray.h.
Referenced by csFixedSizeAllocator< sizeof(T), Allocator >::Compact(), csBitArrayTweakable< >::Delete(), csFixedSizeAllocator< sizeof(T), Allocator >::DisposeAll(), csBitArrayTweakable< InlinedBits, Allocator >::BitProxy::Flip(), csBitArrayTweakable< InlinedBits, Allocator >::BitProxy::operator bool(), csBitArrayTweakable< InlinedBits, Allocator >::BitProxy::operator=(), csBitArrayTweakable< >::operator[](), and csBitArrayTweakable< >::Slice().
size_t csBitArrayTweakable< InlinedBits, Allocator >::Length | ( | ) | const [inline] |
Return the number of stored bits.
- Deprecated:
- Use GetSize() instead.
Definition at line 293 of file bitarray.h.
csBitArrayTweakable& csBitArrayTweakable< InlinedBits, Allocator >::operator &= | ( | const csBitArrayTweakable< InlinedBits, Allocator > & | that | ) | [inline] |
bool csBitArrayTweakable< InlinedBits, Allocator >::operator!= | ( | const csBitArrayTweakable< InlinedBits, Allocator > & | that | ) | const [inline] |
csBitArrayTweakable& csBitArrayTweakable< InlinedBits, Allocator >::operator= | ( | const csBitArrayTweakable< InlinedBits, Allocator > & | that | ) | [inline] |
bool csBitArrayTweakable< InlinedBits, Allocator >::operator== | ( | const csBitArrayTweakable< InlinedBits, Allocator > & | that | ) | const [inline] |
bool csBitArrayTweakable< InlinedBits, Allocator >::operator[] | ( | size_t | pos | ) | const [inline] |
BitProxy csBitArrayTweakable< InlinedBits, Allocator >::operator[] | ( | size_t | pos | ) | [inline] |
csBitArrayTweakable csBitArrayTweakable< InlinedBits, Allocator >::operator^= | ( | const csBitArrayTweakable< InlinedBits, Allocator > & | that | ) | [inline] |
csBitArrayTweakable csBitArrayTweakable< InlinedBits, Allocator >::operator|= | ( | const csBitArrayTweakable< InlinedBits, Allocator > & | that | ) | [inline] |
csBitArrayTweakable csBitArrayTweakable< InlinedBits, Allocator >::operator~ | ( | ) | const [inline] |
Return complement bit array in which all bits are flipped from this one.
Definition at line 441 of file bitarray.h.
void csBitArrayTweakable< InlinedBits, Allocator >::Set | ( | size_t | pos, | |
bool | val = true | |||
) | [inline] |
Set the bit at position pos to the given value.
Definition at line 499 of file bitarray.h.
Referenced by csBitArrayTweakable< >::Delete(), and csBitArrayTweakable< InlinedBits, Allocator >::BitProxy::operator=().
void csBitArrayTweakable< InlinedBits, Allocator >::SetBit | ( | size_t | pos | ) | [inline] |
Set the bit at position pos to true.
Definition at line 478 of file bitarray.h.
Referenced by csBitArrayTweakable< >::Set(), and csBitArrayTweakable< >::Slice().
void csBitArrayTweakable< InlinedBits, Allocator >::SetLength | ( | size_t | newSize | ) | [inline] |
Set the number of stored bits.
- Deprecated:
- Use SetSize() instead.
Definition at line 303 of file bitarray.h.
void csBitArrayTweakable< InlinedBits, Allocator >::SetSize | ( | size_t | newSize | ) | [inline] |
Set the number of stored bits.
- Remarks:
- If the new size is larger than the old size, the newly added bits are cleared.
Definition at line 313 of file bitarray.h.
Referenced by csBitArrayTweakable< >::csBitArrayTweakable(), csBitArrayTweakable< >::Delete(), csBitArrayTweakable< >::operator=(), and csBitArrayTweakable< >::SetLength().
csBitArrayTweakable csBitArrayTweakable< InlinedBits, Allocator >::Slice | ( | size_t | pos, | |
size_t | count | |||
) | const [inline] |
Return a new bit array containing a slice count bits in length from this array starting at pos.
Does not modify this array.
Definition at line 578 of file bitarray.h.
Friends And Related Function Documentation
csBitArrayTweakable operator & | ( | const csBitArrayTweakable< InlinedBits, Allocator > & | a1, | |
const csBitArrayTweakable< InlinedBits, Allocator > & | a2 | |||
) | [friend] |
csBitArrayTweakable operator^ | ( | const csBitArrayTweakable< InlinedBits, Allocator > & | a1, | |
const csBitArrayTweakable< InlinedBits, Allocator > & | a2 | |||
) | [friend] |
csBitArrayTweakable operator| | ( | const csBitArrayTweakable< InlinedBits, Allocator > & | a1, | |
const csBitArrayTweakable< InlinedBits, Allocator > & | a2 | |||
) | [friend] |
The documentation for this class was generated from the following file:
- csutil/bitarray.h
Generated for Crystal Space by doxygen 1.4.7