csColor Class Reference
A class used to represent a color in RGB space. More...
#include <csutil/cscolor.h>
Inheritance diagram for csColor:
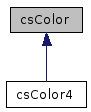
Public Member Functions | |
void | Add (float r, float g, float b) |
Add given R,G,B components to color. | |
void | Clamp (float r, float g, float b) |
Clamp color to given R,G,B values. | |
void | ClampDown () |
Make sure color components are not negative. | |
csColor (const csColor &c) | |
Initialize a color object with an existing color. | |
csColor (float r, float g, float b) | |
Initialize a color object with given R,G,B components. | |
csColor () | |
Initialize a color object (contents undefined). | |
csColor | operator * (const float f) |
Multiply this color by a scalar, return result. | |
csColor & | operator *= (const csColor &c) |
Multiply another color with this one. | |
csColor & | operator *= (float f) |
Multiply this color by a scalar value. | |
bool | operator!= (const csColor &c) const |
Compare inequality of two colors. | |
csColor & | operator+= (const csColor &c) |
Add another color to this one. | |
csColor & | operator-= (const csColor &c) |
Subtract another color to this one. | |
csColor & | operator= (const csColor &c) |
Assign one color object to another. | |
bool | operator== (const csColor &c) const |
Compare equality of two colors. | |
void | Set (const csColor &c) |
Set color to given color. | |
void | Set (float r, float g, float b) |
Set color to given R,G,B components. | |
void | Subtract (float r, float g, float b) |
Subtract given R,G,B components from color. | |
Public Attributes | |
float | blue |
Blue (0..1). | |
float | green |
Green (0..1). | |
float | red |
Red (0..1). |
Detailed Description
A class used to represent a color in RGB space.This class is similar to csRGBpixel and csRGBcolor except that it uses floating-point values to store R,G,B values.
Definition at line 33 of file cscolor.h.
Constructor & Destructor Documentation
csColor::csColor | ( | ) | [inline] |
csColor::csColor | ( | float | r, | |
float | g, | |||
float | b | |||
) | [inline] |
csColor::csColor | ( | const csColor & | c | ) | [inline] |
Member Function Documentation
void csColor::Add | ( | float | r, | |
float | g, | |||
float | b | |||
) | [inline] |
void csColor::Clamp | ( | float | r, | |
float | g, | |||
float | b | |||
) | [inline] |
void csColor::ClampDown | ( | ) | [inline] |
csColor csColor::operator * | ( | const float | f | ) | [inline] |
csColor& csColor::operator *= | ( | float | f | ) | [inline] |
bool csColor::operator!= | ( | const csColor & | c | ) | const [inline] |
bool csColor::operator== | ( | const csColor & | c | ) | const [inline] |
void csColor::Set | ( | const csColor & | c | ) | [inline] |
void csColor::Set | ( | float | r, | |
float | g, | |||
float | b | |||
) | [inline] |
void csColor::Subtract | ( | float | r, | |
float | g, | |||
float | b | |||
) | [inline] |
Member Data Documentation
float csColor::blue |
Blue (0..1).
Definition at line 41 of file cscolor.h.
Referenced by Add(), Clamp(), ClampDown(), csColor(), operator *(), operator *(), csColor4::operator *=(), operator *=(), csColor4::operator!=(), operator!=(), csColor4::operator+=(), operator+=(), csColor4::operator-=(), operator-=(), operator/(), csColor4::operator=(), operator=(), csColor4::operator==(), operator==(), csColor4::Set(), Set(), csShaderVariable::SetValue(), and Subtract().
float csColor::green |
Green (0..1).
Definition at line 39 of file cscolor.h.
Referenced by Add(), Clamp(), ClampDown(), csColor(), operator *(), operator *(), csColor4::operator *=(), operator *=(), csColor4::operator!=(), operator!=(), csColor4::operator+=(), operator+=(), csColor4::operator-=(), operator-=(), operator/(), csColor4::operator=(), operator=(), csColor4::operator==(), operator==(), csColor4::Set(), Set(), csShaderVariable::SetValue(), and Subtract().
float csColor::red |
Red (0..1).
Definition at line 37 of file cscolor.h.
Referenced by Add(), Clamp(), ClampDown(), csColor(), operator *(), operator *(), csColor4::operator *=(), operator *=(), csColor4::operator!=(), operator!=(), csColor4::operator+=(), operator+=(), csColor4::operator-=(), operator-=(), operator/(), csColor4::operator=(), operator=(), csColor4::operator==(), operator==(), csColor4::Set(), Set(), csShaderVariable::SetValue(), and Subtract().
The documentation for this class was generated from the following file:
- csutil/cscolor.h
Generated for Crystal Space by doxygen 1.4.7