csRedBlackTreeMap< K, T > Class Template Reference
[Containers]
Key-value-map, backed by csRedBlackTree.
More...
#include <csutil/redblacktree.h>
Inheritance diagram for csRedBlackTreeMap< K, T >:
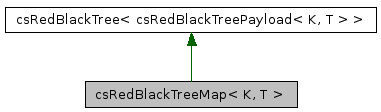
Public Member Functions | |
bool | Delete (const K &key) |
Delete element from map,. | |
void | DeleteAll () |
Delete all keys. | |
void | Empty () |
Delete all the keys. (Idiomatic alias for DeleteAll().). | |
bool | IsEmpty () const |
Returns whether this map has no nodes. | |
T * | Put (const K &key, const T &value) |
Add element to map,. | |
T & | Get (const K &key, T &fallback) |
const T & | Get (const K &key, const T &fallback) const |
Get the element matching the given key, or fallback if there is none. | |
T * | GetElementPointer (const K &key) |
const T * | GetElementPointer (const K &key) const |
Get a pointer to the element matching the given key, or 0 if there is none. | |
template<typename CB> | |
void | TraverseInOrder (CB &callback) const |
Traverse tree. |
Detailed Description
template<typename K, typename T>
class csRedBlackTreeMap< K, T >
Key-value-map, backed by csRedBlackTree.
- Remarks:
- As with csRedBlackTree, every key must be unique.
Definition at line 556 of file redblacktree.h.
Member Function Documentation
bool csRedBlackTreeMap< K, T >::Delete | ( | const K & | key | ) | [inline] |
Delete element from map,.
- Returns:
- Whether the deletion was successful. Fails if the key is not in the tree.
Definition at line 588 of file redblacktree.h.
References csRedBlackTree< csRedBlackTreePayload< K, T > >::Delete(), and csRedBlackTree< csRedBlackTreePayload< K, T > >::Find().
void csRedBlackTreeMap< K, T >::DeleteAll | ( | ) | [inline] |
Delete all keys.
Reimplemented from csRedBlackTree< csRedBlackTreePayload< K, T > >.
Definition at line 631 of file redblacktree.h.
References csRedBlackTree< csRedBlackTreePayload< K, T > >::Empty().
Referenced by csRedBlackTreeMap< K, T >::Empty().
void csRedBlackTreeMap< K, T >::Empty | ( | ) | [inline] |
Delete all the keys. (Idiomatic alias for DeleteAll().).
Reimplemented from csRedBlackTree< csRedBlackTreePayload< K, T > >.
Definition at line 633 of file redblacktree.h.
References csRedBlackTreeMap< K, T >::DeleteAll().
const T& csRedBlackTreeMap< K, T >::Get | ( | const K & | key, | |
const T & | fallback | |||
) | const [inline] |
Get the element matching the given key, or fallback
if there is none.
Definition at line 617 of file redblacktree.h.
References csRedBlackTree< csRedBlackTreePayload< K, T > >::Find(), and csRedBlackTreePayload< K, T >::GetValue().
const T* csRedBlackTreeMap< K, T >::GetElementPointer | ( | const K & | key | ) | const [inline] |
Get a pointer to the element matching the given key, or 0 if there is none.
Definition at line 599 of file redblacktree.h.
References csRedBlackTree< csRedBlackTreePayload< K, T > >::Find(), and csRedBlackTreePayload< K, T >::GetValue().
bool csRedBlackTreeMap< K, T >::IsEmpty | ( | ) | const [inline] |
Returns whether this map has no nodes.
Reimplemented from csRedBlackTree< csRedBlackTreePayload< K, T > >.
Definition at line 635 of file redblacktree.h.
References csRedBlackTree< csRedBlackTreePayload< K, T > >::IsEmpty().
T* csRedBlackTreeMap< K, T >::Put | ( | const K & | key, | |
const T & | value | |||
) | [inline] |
Add element to map,.
- Returns:
- A pointer to the copy of the value stored in the tree, or 0 if the key already exists.
Definition at line 577 of file redblacktree.h.
References csRedBlackTreePayload< K, T >::GetValue(), and csRedBlackTree< csRedBlackTreePayload< K, T > >::Insert().
void csRedBlackTreeMap< K, T >::TraverseInOrder | ( | CB & | callback | ) | const [inline] |
Traverse tree.
Reimplemented from csRedBlackTree< csRedBlackTreePayload< K, T > >.
Definition at line 640 of file redblacktree.h.
References csRedBlackTree< csRedBlackTreePayload< K, T > >::TraverseInOrder().
The documentation for this class was generated from the following file:
- csutil/redblacktree.h
Generated for Crystal Space by doxygen 1.4.7