csSpline Class Reference
[Geometry utilities]
A spline superclass.
More...
#include <csgeom/spline.h>
Inheritance diagram for csSpline:
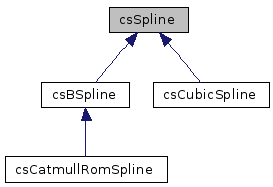
Public Member Functions | |
virtual void | Calculate (float time)=0 |
Calculate internal values for this spline given some time value. | |
csSpline (int d, int p) | |
Create a spline with d dimensions and p points. | |
int | GetCurrentIndex () const |
Get the index of the current point we are in (valid after Calculate()). | |
int | GetDimensionCount () const |
Get the number of dimensions. | |
float | GetDimensionValue (int dim, int idx) const |
Get the value for some dimension. | |
float const * | GetDimensionValues (int dim) const |
Get the values for some dimension. | |
float * | GetIndexValues (int idx) const |
Gets the values of the control point at index idx. | |
virtual float | GetInterpolatedDimension (int dim) const =0 |
After calling Calculate() you can use this to fetch the value of some dimension. | |
int | GetPointCount () const |
Get the number of points. | |
float | GetTimeValue (int idx) const |
Get one time point. | |
float const * | GetTimeValues () const |
Get the time values. | |
void | InsertPoint (int idx) |
Insert a point after some index. | |
void | RemovePoint (int idx) |
Remove a point at the index. | |
void | SetDimensionValue (int dim, int idx, float d) |
Set a value for some dimension. | |
void | SetDimensionValues (int dim, float const *d) |
Set the values for some dimension. | |
void | SetIndexValues (int idx, float const *d) |
Sets the values of the control point at index idx. | |
void | SetTimeValue (int idx, float t) |
Set one time point. | |
void | SetTimeValues (float const *t) |
Set the time values. | |
virtual | ~csSpline () |
Destroy the spline. | |
Protected Attributes | |
int | dimensions |
int | idx |
int | num_points |
float * | points |
bool | precalculation_valid |
float * | time_points |
Detailed Description
A spline superclass.This spline can control several dimensions at once.
Definition at line 35 of file spline.h.
Constructor & Destructor Documentation
csSpline::csSpline | ( | int | d, | |
int | p | |||
) |
Create a spline with d dimensions and p points.
virtual csSpline::~csSpline | ( | ) | [virtual] |
Destroy the spline.
Member Function Documentation
virtual void csSpline::Calculate | ( | float | time | ) | [pure virtual] |
Calculate internal values for this spline given some time value.
Implemented in csCubicSpline, and csBSpline.
int csSpline::GetCurrentIndex | ( | ) | const [inline] |
Get the index of the current point we are in (valid after Calculate()).
int csSpline::GetDimensionCount | ( | ) | const [inline] |
float csSpline::GetDimensionValue | ( | int | dim, | |
int | idx | |||
) | const [inline] |
float const* csSpline::GetDimensionValues | ( | int | dim | ) | const [inline] |
float* csSpline::GetIndexValues | ( | int | idx | ) | const |
Gets the values of the control point at index idx.
Caller is responsible for invoking `delete[]' on returned array when no longer needed.
virtual float csSpline::GetInterpolatedDimension | ( | int | dim | ) | const [pure virtual] |
After calling Calculate() you can use this to fetch the value of some dimension.
Implemented in csCubicSpline, and csBSpline.
int csSpline::GetPointCount | ( | ) | const [inline] |
float csSpline::GetTimeValue | ( | int | idx | ) | const [inline] |
float const* csSpline::GetTimeValues | ( | ) | const [inline] |
void csSpline::InsertPoint | ( | int | idx | ) |
Insert a point after some index.
If index == -1 add a point before all others.
void csSpline::RemovePoint | ( | int | idx | ) |
Remove a point at the index.
void csSpline::SetDimensionValue | ( | int | dim, | |
int | idx, | |||
float | d | |||
) |
Set a value for some dimension.
void csSpline::SetDimensionValues | ( | int | dim, | |
float const * | d | |||
) |
Set the values for some dimension.
'd' should point to an array containing 'num_points' values. These are the values that will be interpolated. The given array is copied.
void csSpline::SetIndexValues | ( | int | idx, | |
float const * | d | |||
) |
Sets the values of the control point at index idx.
void csSpline::SetTimeValue | ( | int | idx, | |
float | t | |||
) |
Set one time point.
void csSpline::SetTimeValues | ( | float const * | t | ) |
Set the time values.
't' should point to an array containing 'num_points' values. These values typically start with 0 and end with 1. Other values are also possible the but the values should rise. The given array is copied.
The documentation for this class was generated from the following file:
- csgeom/spline.h
Generated for Crystal Space by doxygen 1.4.7