csStringBase Class Reference
This is a string class with a range of useful operators and type-safe overloads. More...
#include <csutil/csstring.h>
Inheritance diagram for csStringBase:
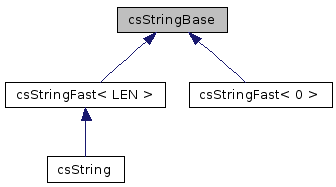
Public Member Functions | |
csStringBase & | Append (bool b) |
Append a boolean (as a number -- 1 or 0) to this string. | |
csStringBase & | Append (char c) |
Append a signed character to this string. | |
csStringBase & | Append (const csStringBase &Str, size_t Count=(size_t)-1) |
Append a string to this one. | |
csStringBase & | Append (const wchar_t *Str, size_t Count=(size_t)-1) |
Append a null-terminated wide string to this one. | |
csStringBase & | Append (const char *Str, size_t Count=(size_t)-1) |
Append a null-terminated C-string to this one. | |
csStringBase & | Clear () |
Clear the string (so that it contains only a null terminator). | |
csStringBase | Clone () const |
Get a copy of this string. | |
csStringBase & | Collapse () |
Trim leading and trailing whitespace, and collapse all internal whitespace to a single space. | |
bool | Compare (const char *iStr) const |
Check if a null-terminated C- string is equal to this string. | |
bool | Compare (const csStringBase &iStr) const |
Check if another string is equal to this one. | |
bool | CompareNoCase (const char *iStr) const |
Check if a null-terminated C- string is equal to this string. | |
bool | CompareNoCase (const csStringBase &iStr) const |
Check if another string is equal to this one. | |
csStringBase (unsigned char c) | |
Create a csStringBase object from a single unsigned character. | |
csStringBase (char c) | |
Create a csStringBase object from a single signed character. | |
csStringBase (const wchar_t *src, size_t _length) | |
Create a csStringBase object from a wide string, given the length. | |
csStringBase (const char *src, size_t _length) | |
Create a csStringBase object from a C string, given the length. | |
csStringBase (const wchar_t *src) | |
Create a csStringBase object from a null-terminated wide string. | |
csStringBase (const char *src) | |
Create a csStringBase object from a null-terminated C string. | |
csStringBase (const csStringBase ©) | |
Copy constructor. | |
csStringBase (size_t Length) | |
Create a csStringBase object and reserve space for at least Length characters. | |
csStringBase () | |
Create an empty csStringBase object. | |
csStringBase & | DeleteAt (size_t Pos, size_t Count=1) |
Delete a range of characters from the string. | |
virtual char * | Detach () |
Detach the low-level null-terminated C-string buffer from the csString object. | |
csStringBase & | Downcase () |
Convert this string to lower-case. | |
csStringBase & | Empty () |
Clear the string (so that it contains only a null terminator). | |
size_t | Find (const char *search, size_t pos=0) const |
Find the first occurrence of search in this string starting at pos . | |
size_t | FindFirst (const char *c, size_t pos=0) const |
Find the first occurrence of any of a set of characters in the string. | |
size_t | FindFirst (char c, size_t pos=0) const |
Find the first occurrence of a character in the string. | |
size_t | FindLast (const char *c, size_t pos=(size_t)-1) const |
Find the last occurrence of any of a set of characters in the string. | |
size_t | FindLast (char c, size_t pos=(size_t)-1) const |
Find the last occurrence of a character in the string. | |
void | FindReplace (const char *search, const char *replacement) |
Find all occurrences of search in this string and replace them with replacement . | |
size_t | FindStr (const char *search, size_t pos=0) const |
Find the first occurrence of search in this string starting at pos . | |
csStringBase & | Format (const char *format,...) |
Format this string using cs_snprintf()-style formatting directives. | |
csStringBase & | FormatV (const char *format, va_list args) |
Format this string using cs_snprintf() formatting directives in a va_list. | |
virtual void | Free () |
Free the memory allocated for the string. | |
char | GetAt (size_t n) const |
Get the n'th character. | |
virtual size_t | GetCapacity () const |
Return the current capacity, not including the space for the implicit null terminator. | |
virtual CS_VISIBILITY_DEFAULT char const * | GetData () const |
Get a pointer to the null-terminated character array. | |
char const * | GetDataSafe () const |
Get a pointer to the null-terminated character array. | |
size_t | GetGrowsBy () const |
Return the number of bytes by which the string grows. | |
bool | GetGrowsExponentially () const |
Returns true if exponential growth is enabled. | |
uint | GetHash () const |
GetHash() as expected by the default csHashComputer<> implementation to allow use of csStrings as hash keys. | |
csStringBase & | Insert (size_t Pos, char C) |
Insert another string into this one. | |
csStringBase & | Insert (size_t Pos, const char *Str) |
Insert another string into this one. | |
csStringBase & | Insert (size_t Pos, const csStringBase &Str) |
Insert another string into this one. | |
bool | IsEmpty () const |
Check if string is empty. | |
size_t | Length () const |
Query string length. | |
csStringBase & | LTrim () |
Trim leading whitespace. | |
operator const char * () const | |
Get a pointer to the null-terminated character array. | |
bool | operator!= (const char *Str) const |
Check if another string is not equal to this one. | |
bool | operator!= (const csStringBase &Str) const |
Check if another string is not equal to this one. | |
csStringBase | operator+ (const csStringBase &iStr) const |
Add another string to this one and return the result as a new string. | |
csStringBase & | operator+= (char const *s) |
template<typename T> | |
csStringBase & | operator+= (T const &s) |
Append a formatted value to this string. | |
bool | operator< (const char *Str) const |
Check if another string is less than this one. | |
bool | operator< (const csStringBase &Str) const |
Check if another string is less than this one. | |
csStringBase & | operator<< (char const *v) |
template<typename T> | |
csStringBase & | operator<< (T const &v) |
Shift operator. | |
const csStringBase & | operator= (const csStringBase ©) |
Assign another string to this one. | |
template<typename T> | |
const csStringBase & | operator= (T const &s) |
Assign a formatted value to this string. | |
bool | operator== (const char *Str) const |
Check if another string is equal to this one. | |
bool | operator== (const csStringBase &Str) const |
Check if another string is equal to this one. | |
bool | operator> (const char *Str) const |
Check to see if a string is greater than this one. | |
bool | operator> (const csStringBase &Str) const |
Check to see if a string is greater than this one. | |
char | operator[] (size_t n) const |
Get n'th character. | |
char & | operator[] (size_t n) |
Get a modifiable reference to n'th character. | |
csStringBase & | Overwrite (size_t Pos, const csStringBase &Str) |
Overlay another string onto a part of this string. | |
csStringBase & | PadCenter (size_t NewSize, char PadChar= ' ') |
Pad to a specified size with leading and trailing characters so as to center the string. | |
csStringBase & | PadLeft (size_t NewSize, char PadChar= ' ') |
Pad to a specified size with leading characters. | |
csStringBase & | PadRight (size_t NewSize, char PadChar= ' ') |
Pad to a specified size with trailing characters. | |
csStringBase & | Reclaim () |
Set string buffer capacity to hold exactly the current content. | |
template<typename T> | |
csStringBase & | Replace (T const &val) |
Replace contents of this string with the value in formatted form. | |
csStringBase & | Replace (const char *Str, size_t Count=(size_t)-1) |
Replace contents of this string with the contents of another. | |
csStringBase & | Replace (const csStringBase &Str, size_t Count=(size_t)-1) |
Replace contents of this string with the contents of another. | |
void | ReplaceAll (const char *search, const char *replacement) |
Find all occurrences of search in this string and replace them with replacement . | |
csStringBase & | RTrim () |
Trim trailing whitespace. | |
void | SetAt (size_t n, const char c) |
Set the n'th character. | |
void | SetCapacity (size_t NewSize) |
Ask the string to allocate enough space to hold NewSize characters. | |
void | SetGrowsBy (size_t) |
Advise the string that it should grow its allocated buffer by approximately this many bytes when more space is required. | |
void | SetGrowsExponentially (bool b) |
Tell the string to re-size its buffer exponentially as needed. | |
virtual void | ShrinkBestFit () |
Set string buffer capacity to hold exactly the current content. | |
csStringBase | Slice (size_t start, size_t len=(size_t)-1) const |
Copy and return a portion of this string. | |
bool | StartsWith (const char *iStr, bool ignore_case=false) const |
Check if this string starts with a null-terminated C- string. | |
bool | StartsWith (const csStringBase &iStr, bool ignore_case=false) const |
Check if this string starts with another one. | |
void | SubString (csStringBase &sub, size_t start, size_t len=(size_t)-1) const |
Copy a portion of this string. | |
csStringBase & | Trim () |
Trim leading and trailing whitespace. | |
csStringBase & | Truncate (size_t Len) |
Truncate the string. | |
csStringBase & | Upcase () |
Convert this string to upper-case. | |
virtual | ~csStringBase () |
Destroy the csStringBase. | |
csStringBase & | Append (ulonglong v) |
csStringBase & | Append (longlong v) |
csStringBase & | Append (double v) |
csStringBase & | Append (float v) |
csStringBase & | Append (unsigned long v) |
csStringBase & | Append (long v) |
csStringBase & | Append (unsigned int v) |
csStringBase & | Append (int v) |
csStringBase & | Append (unsigned short v) |
csStringBase & | Append (short v) |
Append the value, in formatted form, to this string. | |
csStringBase & | AppendFmt (const char *format,...) |
Append a string formatted using cs_snprintf()-style formatting directives. | |
csStringBase & | AppendFmtV (const char *format, va_list args) |
Append a string formatted using cs_snprintf()-style formatting directives. | |
Protected Types | |
enum | |
Default number of bytes by which allocation should grow. More... | |
Protected Member Functions | |
size_t | ComputeNewSize (size_t NewSize) |
Compute a new buffer size. Takes GrowBy into consideration. | |
void | ExpandIfNeeded (size_t NewSize) |
If necessary, increase the buffer capacity enough to hold NewSize bytes. | |
virtual char * | GetDataMutable () |
Get a pointer to the null-terminated character array. | |
virtual void | SetCapacityInternal (size_t NewSize, bool soft) |
Set the buffer to hold NewSize bytes. | |
Protected Attributes | |
char * | Data |
String buffer. | |
size_t | GrowBy |
Size in bytes by which allocated buffer is increased when needed. | |
size_t | MaxSize |
Size in bytes of allocated string buffer. | |
size_t | Size |
Length of string; not including null terminator. |
Detailed Description
This is a string class with a range of useful operators and type-safe overloads.Strings may contain arbitary binary data, including null bytes. It also guarantees that a null-terminator always follows the last stored character, thus you can safely use the return value from GetData() and `operator char const*()' in calls to functions expecting C strings. The implicit null terminator is not included in the character count returned by Length().
Like a typical C character string pointer, csStringBase can also represent a null pointer. This allows a non-string to be distinguished from an empty (zero-length) string. The csStringBase will represent a null-pointer in the following cases:
- When constructed with no arguments (the default constructor).
- When constructed with an explicit null-pointer.
- When assigned a null-pointer via operator=((char const*)0).
- After an invocation of Replace((char const*)0).
- After invocation of csStringBase::Free() or any method which is documented as invoking Free() as a side-effect, such as ShrinkBestFit().
- After invocation of csStringBase::Detach().
Definition at line 51 of file csstring.h.
Member Enumeration Documentation
anonymous enum [protected] |
Default number of bytes by which allocation should grow.
*** IMPORTANT *** This must be a power of two (i.e. 8, 16, 32, 64, etc.).
Definition at line 58 of file csstring.h.
Constructor & Destructor Documentation
csStringBase::csStringBase | ( | ) | [inline] |
Create an empty csStringBase object.
- Remarks:
- The newly constructed string represents a null-pointer.
Definition at line 192 of file csstring.h.
csStringBase::csStringBase | ( | size_t | Length | ) | [inline] |
Create a csStringBase object and reserve space for at least Length
characters.
- Remarks:
- The newly constructed string represents a non-null zero-length string.
Definition at line 201 of file csstring.h.
csStringBase::csStringBase | ( | const csStringBase & | copy | ) | [inline] |
Copy constructor.
- Remarks:
- The newly constructed string will represent a null-pointer if and only if the template string represented a null-pointer.
Definition at line 210 of file csstring.h.
csStringBase::csStringBase | ( | const char * | src | ) | [inline] |
Create a csStringBase object from a null-terminated C string.
- Remarks:
- The newly constructed string will represent a null-pointer if and only if the input argument is a null-pointer.
Definition at line 219 of file csstring.h.
csStringBase::csStringBase | ( | const wchar_t * | src | ) | [inline] |
Create a csStringBase object from a null-terminated wide string.
- Remarks:
- The newly constructed string will represent a null-pointer if and only if the input argument is a null-pointer.
The string will be stored as UTF-8.
Definition at line 229 of file csstring.h.
csStringBase::csStringBase | ( | const char * | src, | |
size_t | _length | |||
) | [inline] |
Create a csStringBase object from a C string, given the length.
- Remarks:
- The newly constructed string will represent a null-pointer if and only if the input argument is a null-pointer.
Definition at line 238 of file csstring.h.
csStringBase::csStringBase | ( | const wchar_t * | src, | |
size_t | _length | |||
) | [inline] |
Create a csStringBase object from a wide string, given the length.
- Remarks:
- The newly constructed string will represent a null-pointer if and only if the input argument is a null-pointer.
The string will be stored as UTF-8.
Definition at line 248 of file csstring.h.
csStringBase::csStringBase | ( | char | c | ) | [inline] |
Create a csStringBase object from a single signed character.
Definition at line 253 of file csstring.h.
csStringBase::csStringBase | ( | unsigned char | c | ) | [inline] |
Create a csStringBase object from a single unsigned character.
Definition at line 258 of file csstring.h.
virtual csStringBase::~csStringBase | ( | ) | [virtual] |
Destroy the csStringBase.
Member Function Documentation
csStringBase& csStringBase::Append | ( | short | v | ) | [inline] |
csStringBase& csStringBase::Append | ( | bool | b | ) | [inline] |
Append a boolean (as a number -- 1 or 0) to this string.
- Returns:
- Reference to itself.
Definition at line 161 of file csstring.h.
csStringBase& csStringBase::Append | ( | char | c | ) |
Append a signed character to this string.
- Returns:
- Reference to itself.
csStringBase& csStringBase::Append | ( | const csStringBase & | Str, | |
size_t | Count = (size_t)-1 | |||
) |
Append a string to this one.
- Parameters:
-
Str String which will be appended. Count Number of characters from Str to append; if -1 (the default), then all characters from Str will be appended.
- Returns:
- Reference to itself.
csStringBase& csStringBase::Append | ( | const wchar_t * | Str, | |
size_t | Count = (size_t)-1 | |||
) |
Append a null-terminated wide string to this one.
- Parameters:
-
Str String which will be appended. Count Number of characters from Str to append; if -1 (the default), then all characters from Str will be appended.
- Returns:
- Reference to itself.
- Remarks:
- The string will be appended as UTF-8.
csStringBase& csStringBase::Append | ( | const char * | Str, | |
size_t | Count = (size_t)-1 | |||
) |
Append a null-terminated C-string to this one.
- Parameters:
-
Str String which will be appended. Count Number of characters from Str to append; if -1 (the default), then all characters from Str will be appended.
- Returns:
- Reference to itself.
Referenced by csevJoystickOp(), csevMouse(), csevMouseOp(), csStringFast< LEN >::csStringFast(), operator+(), and csStringArray::SplitString().
csStringBase& csStringBase::AppendFmt | ( | const char * | format, | |
... | ||||
) |
Append a string formatted using cs_snprintf()-style formatting directives.
csStringBase& csStringBase::AppendFmtV | ( | const char * | format, | |
va_list | args | |||
) |
Append a string formatted using cs_snprintf()-style formatting directives.
csStringBase& csStringBase::Clear | ( | ) | [inline] |
Clear the string (so that it contains only a null terminator).
- Returns:
- Reference to itself.
- Remarks:
- This is rigidly equivalent to Empty(), but more idiomatic in terms of human language.
Definition at line 364 of file csstring.h.
csStringBase csStringBase::Clone | ( | ) | const [inline] |
Get a copy of this string.
- Remarks:
- The newly constructed string will represent a null-pointer if and only if this string represents a null-pointer.
Definition at line 721 of file csstring.h.
Referenced by operator+().
csStringBase& csStringBase::Collapse | ( | ) |
Trim leading and trailing whitespace, and collapse all internal whitespace to a single space.
- Returns:
- Reference to itself.
bool csStringBase::Compare | ( | const char * | iStr | ) | const [inline] |
Check if a null-terminated C- string is equal to this string.
- Parameters:
-
iStr Other string.
- Returns:
- True if they are equal; false if not.
- Remarks:
- The comparison is case-sensitive.
Definition at line 640 of file csstring.h.
bool csStringBase::Compare | ( | const csStringBase & | iStr | ) | const [inline] |
Check if another string is equal to this one.
- Parameters:
-
iStr Other string.
- Returns:
- True if they are equal; false if not.
- Remarks:
- The comparison is case-sensitive.
Definition at line 622 of file csstring.h.
References GetDataSafe(), and Length().
bool csStringBase::CompareNoCase | ( | const char * | iStr | ) | const [inline] |
Check if a null-terminated C- string is equal to this string.
- Parameters:
-
iStr Other string.
- Returns:
- True if they are equal; false if not.
- Remarks:
- The comparison is case-insensitive.
Definition at line 667 of file csstring.h.
References csStrCaseCmp().
bool csStringBase::CompareNoCase | ( | const csStringBase & | iStr | ) | const [inline] |
Check if another string is equal to this one.
- Parameters:
-
iStr Other string.
- Returns:
- True if they are equal; false if not.
- Remarks:
- The comparison is case-insensitive.
Definition at line 649 of file csstring.h.
References csStrNCaseCmp(), GetDataSafe(), and Length().
size_t csStringBase::ComputeNewSize | ( | size_t | NewSize | ) | [protected] |
Compute a new buffer size. Takes GrowBy into consideration.
Referenced by csStringFast< LEN >::SetCapacityInternal().
csStringBase& csStringBase::DeleteAt | ( | size_t | Pos, | |
size_t | Count = 1 | |||
) |
Delete a range of characters from the string.
- Parameters:
-
Pos Beginning of range to be deleted (zero-based). Count Number of characters to delete.
- Returns:
- Reference to itself.
virtual char* csStringBase::Detach | ( | ) | [inline, virtual] |
Detach the low-level null-terminated C-string buffer from the csString object.
- Returns:
- The low-level null-terminated C-string buffer, or zero if this string represents a null-pointer. See the class description for a discussion about how and when the string will represent a null-pointer.
- Remarks:
- The caller of this function becomes the owner of the returned string buffer and is responsible for destroying it via `delete[]' when no longer needed.
Reimplemented in csStringFast< LEN >.
Definition at line 934 of file csstring.h.
Referenced by csStringFast< LEN >::Detach().
csStringBase& csStringBase::Downcase | ( | ) |
Convert this string to lower-case.
- Returns:
- Reference to itself.
csStringBase& csStringBase::Empty | ( | ) | [inline] |
Clear the string (so that it contains only a null terminator).
- Returns:
- Reference to itself.
- Remarks:
- This is rigidly equivalent to Truncate(0).
Definition at line 334 of file csstring.h.
void csStringBase::ExpandIfNeeded | ( | size_t | NewSize | ) | [protected] |
If necessary, increase the buffer capacity enough to hold NewSize
bytes.
Buffer capacity is increased in GrowBy increments or exponentially depending upon configuration.
size_t csStringBase::Find | ( | const char * | search, | |
size_t | pos = 0 | |||
) | const |
Find the first occurrence of search
in this string starting at pos
.
- Parameters:
-
search String to locate. pos Start position of search (default 0).
- Returns:
- First position of
search
, or (size_t)-1 if not found.
size_t csStringBase::FindFirst | ( | const char * | c, | |
size_t | pos = 0 | |||
) | const |
Find the first occurrence of any of a set of characters in the string.
- Parameters:
-
c Characters to locate. pos Start position of search (default 0).
- Returns:
- First position of character, or (size_t)-1 if not found.
size_t csStringBase::FindFirst | ( | char | c, | |
size_t | pos = 0 | |||
) | const |
Find the first occurrence of a character in the string.
- Parameters:
-
c Character to locate. pos Start position of search (default 0).
- Returns:
- First position of character, or (size_t)-1 if not found.
size_t csStringBase::FindLast | ( | const char * | c, | |
size_t | pos = (size_t)-1 | |||
) | const |
Find the last occurrence of any of a set of characters in the string.
- Parameters:
-
c Characters to locate. pos Start position of reverse search. Specify (size_t)-1 if you want the search to begin at the very end of string.
- Returns:
- Last position of character, or (size_t)-1 if not found.
size_t csStringBase::FindLast | ( | char | c, | |
size_t | pos = (size_t)-1 | |||
) | const |
Find the last occurrence of a character in the string.
- Parameters:
-
c Character to locate. pos Start position of reverse search. Specify (size_t)-1 if you want the search to begin at the very end of string.
- Returns:
- Last position of character, or (size_t)-1 if not found.
void csStringBase::FindReplace | ( | const char * | search, | |
const char * | replacement | |||
) | [inline] |
Find all occurrences of search
in this string and replace them with replacement
.
- Deprecated:
- Use ReplaceAll() instead.
Definition at line 566 of file csstring.h.
size_t csStringBase::FindStr | ( | const char * | search, | |
size_t | pos = 0 | |||
) | const [inline] |
Find the first occurrence of search
in this string starting at pos
.
- Parameters:
-
search String to locate. pos Start position of search (default 0).
- Returns:
- First position of
search
, or (size_t)-1 if not found.
- Deprecated:
- Use Find() instead.
Definition at line 551 of file csstring.h.
csStringBase& csStringBase::Format | ( | const char * | format, | |
... | ||||
) |
Format this string using cs_snprintf()-style formatting directives.
- Returns:
- Reference to itself.
- Remarks:
- Automatically allocates sufficient memory to hold result. Newly formatted string replaces previous string value.
Referenced by csIdentStrings::StringForIdent().
csStringBase& csStringBase::FormatV | ( | const char * | format, | |
va_list | args | |||
) |
Format this string using cs_snprintf() formatting directives in a va_list.
- Returns:
- Reference to itself.
- Remarks:
- Automatically allocates sufficient memory to hold result. Newly formatted string replaces previous string value.
Referenced by csDebugImageWriter::DebugImageWrite().
virtual void csStringBase::Free | ( | ) | [virtual] |
Free the memory allocated for the string.
- Remarks:
- Following a call to this method, invocations of GetData() and 'operator char const*' will return a null pointer (until some new content is added to the string).
Reimplemented in csStringFast< LEN >.
Referenced by csStringFast< LEN >::Free(), and csStringFast< LEN >::ShrinkBestFit().
char csStringBase::GetAt | ( | size_t | n | ) | const [inline] |
virtual size_t csStringBase::GetCapacity | ( | ) | const [inline, virtual] |
Return the current capacity, not including the space for the implicit null terminator.
Reimplemented in csStringFast< LEN >.
Definition at line 116 of file csstring.h.
Referenced by csStringFast< LEN >::GetCapacity().
virtual CS_VISIBILITY_DEFAULT char const* csStringBase::GetData | ( | ) | const [inline, virtual] |
Get a pointer to the null-terminated character array.
- Returns:
- A C-string pointer to the null-terminated character array; or zero if the string represents a null-pointer.
- Remarks:
- See the class description for a discussion about how and when the string will represent a null-pointer.
Reimplemented in csStringFast< LEN >.
Definition at line 374 of file csstring.h.
virtual char* csStringBase::GetDataMutable | ( | ) | [inline, protected, virtual] |
Get a pointer to the null-terminated character array.
- Returns:
- A C-string pointer to the null-terminated character array; or zero if the string represents a null-pointer.
- Remarks:
- See the class description for a discussion about how and when the string will represent a null-pointer.
- Warning:
- This returns a non-const pointer, so use this function with care. Call this only when you need to modify the string content. External clients are never allowed to directly modify the internal string buffer, which is why this function is not public.
Reimplemented in csStringFast< LEN >.
Definition at line 99 of file csstring.h.
char const* csStringBase::GetDataSafe | ( | ) | const [inline] |
Get a pointer to the null-terminated character array.
- Returns:
- A C-string pointer to the null-terminated character array.
- Remarks:
- Unlike GetData(), this will always return a valid, non-null C-string, even if the underlying representation is that of a null-pointer (in which case, it will return a zero-length C-string. This is a handy convenience which makes it possible to use the result directly without having to perform a null check first.
Definition at line 386 of file csstring.h.
Referenced by Compare(), CompareNoCase(), operator<(), operator>(), and StartsWith().
size_t csStringBase::GetGrowsBy | ( | ) | const [inline] |
Return the number of bytes by which the string grows.
- Remarks:
- If the return value is zero, then the internal buffer grows exponentially by doubling its size, rather than by fixed increments.
Definition at line 284 of file csstring.h.
bool csStringBase::GetGrowsExponentially | ( | ) | const [inline] |
Returns true if exponential growth is enabled.
- Deprecated:
- Use GetGrowsBy() instead.
Definition at line 300 of file csstring.h.
uint csStringBase::GetHash | ( | ) | const |
GetHash() as expected by the default csHashComputer<> implementation to allow use of csStrings as hash keys.
csStringBase& csStringBase::Insert | ( | size_t | Pos, | |
char | C | |||
) |
Insert another string into this one.
- Parameters:
-
Pos Position at which to insert the other string (zero-based). C Character to insert.
- Returns:
- Reference to itself.
csStringBase& csStringBase::Insert | ( | size_t | Pos, | |
const char * | Str | |||
) |
Insert another string into this one.
- Parameters:
-
Pos Position at which to insert the other string (zero-based). Str String to insert.
- Returns:
- Reference to itself.
csStringBase& csStringBase::Insert | ( | size_t | Pos, | |
const csStringBase & | Str | |||
) |
Insert another string into this one.
- Parameters:
-
Pos Position at which to insert the other string (zero-based). Str String to insert.
- Returns:
- Reference to itself.
bool csStringBase::IsEmpty | ( | ) | const [inline] |
Check if string is empty.
- Returns:
- True if the string is empty; false if it is not.
- Remarks:
- This is rigidly equivalent to the expression 'Length() == 0'.
Definition at line 402 of file csstring.h.
size_t csStringBase::Length | ( | ) | const [inline] |
Query string length.
- Returns:
- The string length.
- Remarks:
- The returned length does not count the implicit null terminator.
Definition at line 394 of file csstring.h.
Referenced by Compare(), CompareNoCase(), and StartsWith().
csStringBase& csStringBase::LTrim | ( | ) |
Trim leading whitespace.
- Returns:
- Reference to itself.
- Remarks:
- This is equivalent to Truncate(n) where 'n' is the last non-whitespace character, or zero if the string is composed entirely of whitespace.
csStringBase::operator const char * | ( | ) | const [inline] |
Get a pointer to the null-terminated character array.
- Returns:
- A C-string pointer to the null-terminated character array; or zero if the string represents a null-pointer.
- Remarks:
- See the class description for a discussion about how and when the string will represent a null-pointer.
Definition at line 821 of file csstring.h.
bool csStringBase::operator!= | ( | const char * | Str | ) | const [inline] |
Check if another string is not equal to this one.
- Parameters:
-
Str Other string.
- Returns:
- False if they are equal; true if not.
- Remarks:
- The comparison is case-sensitive.
Definition at line 894 of file csstring.h.
bool csStringBase::operator!= | ( | const csStringBase & | Str | ) | const [inline] |
Check if another string is not equal to this one.
- Parameters:
-
Str Other string.
- Returns:
- False if they are equal; true if not.
- Remarks:
- The comparison is case-sensitive.
Definition at line 886 of file csstring.h.
csStringBase csStringBase::operator+ | ( | const csStringBase & | iStr | ) | const [inline] |
Add another string to this one and return the result as a new string.
Definition at line 811 of file csstring.h.
csStringBase& csStringBase::operator+= | ( | T const & | s | ) | [inline] |
bool csStringBase::operator< | ( | const char * | Str | ) | const [inline] |
Check if another string is less than this one.
- Parameters:
-
Str Other string.
- Returns:
- True if the string is 'lesser' than Str, false otherwise.
Definition at line 856 of file csstring.h.
bool csStringBase::operator< | ( | const csStringBase & | Str | ) | const [inline] |
Check if another string is less than this one.
- Parameters:
-
Str Other string.
- Returns:
- True if the string is 'lesser' than Str, false otherwise.
Definition at line 846 of file csstring.h.
References GetDataSafe().
csStringBase& csStringBase::operator<< | ( | T const & | v | ) | [inline] |
Shift operator.
For example:
s << "Hi " << name << ", see " << foo;
Definition at line 905 of file csstring.h.
const csStringBase& csStringBase::operator= | ( | const csStringBase & | copy | ) | [inline] |
Assign another string to this one.
Reimplemented in csStringFast< LEN >, csStringFast< 0 >, and csString.
Definition at line 796 of file csstring.h.
const csStringBase& csStringBase::operator= | ( | T const & | s | ) | [inline] |
Assign a formatted value to this string.
Reimplemented in csStringFast< LEN >.
Definition at line 793 of file csstring.h.
bool csStringBase::operator== | ( | const char * | Str | ) | const [inline] |
Check if another string is equal to this one.
- Parameters:
-
Str Other string.
- Returns:
- True if they are equal; false if not.
- Remarks:
- The comparison is case-sensitive.
Definition at line 838 of file csstring.h.
bool csStringBase::operator== | ( | const csStringBase & | Str | ) | const [inline] |
Check if another string is equal to this one.
- Parameters:
-
Str Other string.
- Returns:
- True if they are equal; false if not.
- Remarks:
- The comparison is case-sensitive.
Definition at line 830 of file csstring.h.
bool csStringBase::operator> | ( | const char * | Str | ) | const [inline] |
Check to see if a string is greater than this one.
- Parameters:
-
Str Other string.
- Returns:
- True if the string is 'greater' than Str, false otherwise.
Definition at line 876 of file csstring.h.
bool csStringBase::operator> | ( | const csStringBase & | Str | ) | const [inline] |
Check to see if a string is greater than this one.
- Parameters:
-
Str Other string.
- Returns:
- True if the string is 'greater' than Str, false otherwise.
Definition at line 866 of file csstring.h.
References GetDataSafe().
char csStringBase::operator[] | ( | size_t | n | ) | const [inline] |
char& csStringBase::operator[] | ( | size_t | n | ) | [inline] |
Get a modifiable reference to n'th character.
Definition at line 406 of file csstring.h.
References CS_ASSERT.
csStringBase& csStringBase::Overwrite | ( | size_t | Pos, | |
const csStringBase & | Str | |||
) |
Overlay another string onto a part of this string.
- Parameters:
-
Pos Position at which to insert the other string (zero-based). Str String which will be overlayed atop this string.
- Returns:
- Reference to itself.
- Remarks:
- The target string will grow as necessary to accept the new string.
csStringBase& csStringBase::PadCenter | ( | size_t | NewSize, | |
char | PadChar = ' ' | |||
) |
Pad to a specified size with leading and trailing characters so as to center the string.
- Parameters:
-
NewSize Size to which the string should grow. PadChar Character with which to pad the string (default is space).
- Returns:
- Reference to itself.
- Remarks:
- Never shortens the string. If NewSize is less than or equal to Length(), nothing happens. If the left and right sides can not be padded equally, then the right side will gain the extra one-character padding.
csStringBase& csStringBase::PadLeft | ( | size_t | NewSize, | |
char | PadChar = ' ' | |||
) |
Pad to a specified size with leading characters.
- Parameters:
-
NewSize Size to which the string should grow. PadChar Character with which to pad the string (default is space).
- Returns:
- Reference to itself.
- Remarks:
- Never shortens the string. If NewSize is less than or equal to Length(), nothing happens.
csStringBase& csStringBase::PadRight | ( | size_t | NewSize, | |
char | PadChar = ' ' | |||
) |
Pad to a specified size with trailing characters.
- Parameters:
-
NewSize Size to which the string should grow. PadChar Character with which to pad the string (default is space).
- Returns:
- Reference to itself.
- Remarks:
- Never shortens the string. If NewSize is less than or equal to Length(), nothing happens.
csStringBase& csStringBase::Reclaim | ( | ) | [inline] |
Set string buffer capacity to hold exactly the current content.
- Returns:
- Reference to itself.
- Remarks:
- If the string length is greater than zero, then the buffer's capacity will be adjusted to exactly that size. If the string length is zero, then this is equivalent to an invocation of Free(), which means that GetData() and 'operator char const*' will return a null pointer after reclamation.
Definition at line 356 of file csstring.h.
csStringBase& csStringBase::Replace | ( | T const & | val | ) | [inline] |
Replace contents of this string with the value in formatted form.
- Remarks:
- Internally uses the various flavours of Append().
Definition at line 614 of file csstring.h.
csStringBase& csStringBase::Replace | ( | const char * | Str, | |
size_t | Count = (size_t)-1 | |||
) |
Replace contents of this string with the contents of another.
- Parameters:
-
Str String from which new content of this string will be copied. Count Number of characters to copy. If (size_t)-1 is specified, then all characters will be copied.
- Returns:
- Reference to itself.
- Remarks:
- This string will represent a null-pointer after replacement if and only if Str is a null pointer.
csStringBase& csStringBase::Replace | ( | const csStringBase & | Str, | |
size_t | Count = (size_t)-1 | |||
) |
Replace contents of this string with the contents of another.
- Parameters:
-
Str String from which new content of this string will be copied. Count Number of characters to copy. If (size_t)-1 is specified, then all characters will be copied.
- Returns:
- Reference to itself.
- Remarks:
- This string will represent a null-pointer after replacement if and only if Str represents a null-pointer.
Referenced by csVfsDirectoryChanger::ChangeTo(), csStringFast< 0 >::operator=(), and csStringFast< LEN >::operator=().
void csStringBase::ReplaceAll | ( | const char * | search, | |
const char * | replacement | |||
) |
Find all occurrences of search
in this string and replace them with replacement
.
csStringBase& csStringBase::RTrim | ( | ) |
Trim trailing whitespace.
- Returns:
- Reference to itself.
- Remarks:
- This is equivalent to DeleteAt(0,n) where 'n' is the first non-whitespace character, or Lenght() if the string is composed entirely of whitespace.
void csStringBase::SetAt | ( | size_t | n, | |
const char | c | |||
) | [inline] |
Set the n'th character.
- Remarks:
- The n'th character position must be a valid position in the string. You can not expand the string by setting a character beyond the end of string.
Definition at line 425 of file csstring.h.
References CS_ASSERT.
void csStringBase::SetCapacity | ( | size_t | NewSize | ) |
Ask the string to allocate enough space to hold NewSize
characters.
- Remarks:
- After calling this method, the string's internal capacity will be at least
NewSize
+ 1 (one for the implicit null terminator). Never shrinks capacity. If you need to actually reclaim memory, then use Free() or ShrinkBestFit().
Referenced by csStringFast< LEN >::csStringFast().
virtual void csStringBase::SetCapacityInternal | ( | size_t | NewSize, | |
bool | soft | |||
) | [protected, virtual] |
Set the buffer to hold NewSize bytes.
If soft is true it means the buffer can be rounded up to reduce the number of allocations needed.
Reimplemented in csStringFast< LEN >.
Referenced by csStringFast< LEN >::SetCapacityInternal().
void csStringBase::SetGrowsBy | ( | size_t | ) |
Advise the string that it should grow its allocated buffer by approximately this many bytes when more space is required.
This is an optimization to avoid excessive memory reallocation and heap management, which can be quite slow.
- Remarks:
- This value is only a suggestion. The actual value by which it grows may be rounded up or down to an implementation-dependent allocation multiple.
If the value is zero, then the internal buffer grows exponentially by doubling its size, rather than by fixed increments.
void csStringBase::SetGrowsExponentially | ( | bool | b | ) | [inline] |
Tell the string to re-size its buffer exponentially as needed.
- Deprecated:
- Use SetGrowsBy(0) instead.
Definition at line 292 of file csstring.h.
virtual void csStringBase::ShrinkBestFit | ( | ) | [virtual] |
Set string buffer capacity to hold exactly the current content.
- Returns:
- Reference to itself.
- Remarks:
- If the string length is greater than zero, then the buffer's capacity will be adjusted to exactly that size. If the string length is zero, then this is equivalent to an invocation of Free(), which means that GetData() and 'operator char const*' will return a null pointer after reclamation.
Reimplemented in csStringFast< LEN >.
Referenced by csStringFast< LEN >::ShrinkBestFit().
csStringBase csStringBase::Slice | ( | size_t | start, | |
size_t | len = (size_t)-1 | |||
) | const |
Copy and return a portion of this string.
- Parameters:
-
start Start position of slice (zero-based). len Number of characters in slice.
- Returns:
- The indicated string slice.
bool csStringBase::StartsWith | ( | const char * | iStr, | |
bool | ignore_case = false | |||
) | const [inline] |
Check if this string starts with a null-terminated C- string.
- Parameters:
-
iStr Other string. ignore_case Causes the comparison to be case insensitive if true.
- Returns:
- True if they are equal up to the length of iStr; false if not.
Definition at line 699 of file csstring.h.
References CS_ASSERT, and csStrNCaseCmp().
bool csStringBase::StartsWith | ( | const csStringBase & | iStr, | |
bool | ignore_case = false | |||
) | const [inline] |
Check if this string starts with another one.
- Parameters:
-
iStr Other string. ignore_case Causes the comparison to be case insensitive if true.
- Returns:
- True if they are equal up to the length of iStr; false if not.
Definition at line 676 of file csstring.h.
References CS_ASSERT, csStrNCaseCmp(), GetDataSafe(), and Length().
void csStringBase::SubString | ( | csStringBase & | sub, | |
size_t | start, | |||
size_t | len = (size_t)-1 | |||
) | const |
Copy a portion of this string.
- Parameters:
-
sub String which will receive the indicated substring copy. start Start position of slice (zero-based). len Number of characters in slice.
- Remarks:
- Use this method instead of Slice() for cases where you expect to extract many substrings in a tight loop, and want to avoid the overhead of allocation of a new string object for each operation. Simply re-use 'sub' for each operation.
csStringBase& csStringBase::Trim | ( | ) |
csStringBase& csStringBase::Truncate | ( | size_t | Len | ) |
Truncate the string.
- Parameters:
-
Len The number of characters to which the string should be truncated (possibly 0).
- Returns:
- Reference to itself.
- Remarks:
- Will only make a string shorter; will never extend it. This method does not reclaim memory; it merely shortens the string, which means that Truncate(0) is a handy method of clearing the string, without the overhead of slow heap management. This may be important if you want to re-use the same string many times over. If you need to reclaim memory after truncating the string, then invoke ShrinkBestFit(). GetData() and 'operator char const*' will return a non-null zero-length string if you truncate the string to 0 characters, unless the string had already represented a null-pointer, in which case it will continue to represent a null-pointer after truncation.
csStringBase& csStringBase::Upcase | ( | ) |
Convert this string to upper-case.
- Returns:
- Reference to itself.
Member Data Documentation
char* csStringBase::Data [protected] |
String buffer.
Definition at line 61 of file csstring.h.
Referenced by csStringFast< LEN >::Detach(), csStringFast< LEN >::GetCapacity(), csStringFast< LEN >::GetData(), csStringFast< LEN >::GetDataMutable(), csStringFast< LEN >::SetCapacityInternal(), and csStringFast< LEN >::ShrinkBestFit().
size_t csStringBase::GrowBy [protected] |
Size in bytes by which allocated buffer is increased when needed.
If this value is zero, then growth occurs exponentially by doubling the size.
Definition at line 70 of file csstring.h.
size_t csStringBase::MaxSize [protected] |
Size in bytes of allocated string buffer.
Definition at line 65 of file csstring.h.
Referenced by csStringFast< LEN >::Detach(), and csStringFast< LEN >::SetCapacityInternal().
size_t csStringBase::Size [protected] |
Length of string; not including null terminator.
Definition at line 63 of file csstring.h.
Referenced by csStringFast< LEN >::Detach(), csStringFast< LEN >::SetCapacityInternal(), and csStringFast< LEN >::ShrinkBestFit().
The documentation for this class was generated from the following file:
- csutil/csstring.h
Generated for Crystal Space by doxygen 1.4.7