scfStringArray Class Reference
This class is a thin wrapper around csStringArray with SCF capability. More...
#include <csutil/scfstringarray.h>
Inheritance diagram for scfStringArray:
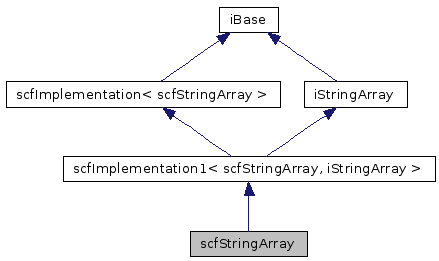
Public Member Functions | |
virtual size_t | Contains (const char *str, bool case_sensitive=true) const |
Alias for Find() and FindCaseInsensitive(). | |
virtual void | DeleteAll () |
Remove all strings from array. | |
virtual bool | DeleteIndex (size_t n) |
Delete string n from the array. | |
virtual void | Empty () |
Remove all strings from array, releasing allocated memory. | |
virtual size_t | Find (const char *value) const |
Find a string, case-sensitive. | |
virtual size_t | FindCaseInsensitive (const char *value) const |
Find a string, case-insensitive. | |
virtual size_t | FindSortedKey (const char *value) const |
Find an element based on some key, using a comparison function. | |
virtual char const * | Get (size_t n) const |
Get a particular string from the array. | |
virtual size_t | GetSize () const |
Get array length. | |
virtual bool | Insert (size_t n, char const *value) |
Insert a string before entry n in the array. | |
virtual bool | IsEmpty () const |
Return true if the array is empty. | |
virtual size_t | Length () const |
Get array length. | |
virtual char * | Pop () |
Pop an element from tail end of array. | |
virtual void | Push (char const *value) |
Push a string onto the stack. | |
scfStringArray (int limit=16, int delta=16) | |
Create a iStringArray from scratch. | |
virtual void | Sort (bool case_sensitive=true) |
Sort array. | |
virtual | ~scfStringArray () |
Destructor - nothing to do. |
Detailed Description
This class is a thin wrapper around csStringArray with SCF capability.
Definition at line 33 of file scfstringarray.h.
Constructor & Destructor Documentation
scfStringArray::scfStringArray | ( | int | limit = 16 , |
|
int | delta = 16 | |||
) | [inline] |
virtual scfStringArray::~scfStringArray | ( | ) | [inline, virtual] |
Member Function Documentation
virtual size_t scfStringArray::Contains | ( | const char * | str, | |
bool | case_sensitive = true | |||
) | const [inline, virtual] |
Alias for Find() and FindCaseInsensitive().
- Parameters:
-
str String to look for in array. case_sensitive If true, consider case when performing comparison. (default: yes)
- Returns:
- csArrayItemNotFound if not found, else item index.
- Remarks:
- Works with sorted and unsorted arrays, but FindSortedKey() is faster on sorted arrays.
Some people find Contains() more idiomatic than Find().
Implements iStringArray.
Definition at line 130 of file scfstringarray.h.
References csStringArray::Contains().
virtual void scfStringArray::DeleteAll | ( | ) | [inline, virtual] |
Remove all strings from array.
- Deprecated:
- Use Empty() instead.
Implements iStringArray.
Definition at line 168 of file scfstringarray.h.
References Empty().
virtual bool scfStringArray::DeleteIndex | ( | size_t | n | ) | [inline, virtual] |
Delete string n
from the array.
Implements iStringArray.
Definition at line 146 of file scfstringarray.h.
References csArray< T, ElementHandler, MemoryAllocator, CapacityHandler >::DeleteIndex().
virtual void scfStringArray::Empty | ( | ) | [inline, virtual] |
Remove all strings from array, releasing allocated memory.
Implements iStringArray.
Definition at line 158 of file scfstringarray.h.
References csArray< T, ElementHandler, MemoryAllocator, CapacityHandler >::Empty().
Referenced by DeleteAll().
virtual size_t scfStringArray::Find | ( | const char * | value | ) | const [inline, virtual] |
Find a string, case-sensitive.
- Returns:
- csArrayItemNotFound if not found, else item index.
- Remarks:
- Works with sorted and unsorted arrays, but FindSortedKey() is faster on sorted arrays.
Implements iStringArray.
Definition at line 93 of file scfstringarray.h.
References csStringArray::Find().
virtual size_t scfStringArray::FindCaseInsensitive | ( | const char * | value | ) | const [inline, virtual] |
Find a string, case-insensitive.
- Returns:
- csArrayItemNotFound if not found, else item index.
- Remarks:
- Works with sorted and unsorted arrays, but FindSortedKey() is faster on sorted arrays.
Implements iStringArray.
Definition at line 104 of file scfstringarray.h.
References csStringArray::FindCaseInsensitive().
virtual size_t scfStringArray::FindSortedKey | ( | const char * | value | ) | const [inline, virtual] |
Find an element based on some key, using a comparison function.
- Returns:
- csArrayItemNotFound if not found, else item index.
- Remarks:
- The array must be sorted.
Implements iStringArray.
Definition at line 114 of file scfstringarray.h.
References csStringArray::FindSortedKey().
virtual char const* scfStringArray::Get | ( | size_t | n | ) | const [inline, virtual] |
Get a particular string from the array.
Implements iStringArray.
Definition at line 82 of file scfstringarray.h.
References csArray< T, ElementHandler, MemoryAllocator, CapacityHandler >::Get().
virtual size_t scfStringArray::GetSize | ( | ) | const [inline, virtual] |
Get array length.
Implements iStringArray.
Definition at line 50 of file scfstringarray.h.
References csArray< T, ElementHandler, MemoryAllocator, CapacityHandler >::Length().
Referenced by Length().
virtual bool scfStringArray::Insert | ( | size_t | n, | |
char const * | value | |||
) | [inline, virtual] |
Insert a string before entry n
in the array.
Implements iStringArray.
Definition at line 152 of file scfstringarray.h.
References csArray< T, ElementHandler, MemoryAllocator, CapacityHandler >::Insert().
virtual bool scfStringArray::IsEmpty | ( | ) | const [inline, virtual] |
Return true if the array is empty.
- Remarks:
- Rigidly equivalent to
return GetSize() == 0
, but more idiomatic.
Implements iStringArray.
Definition at line 178 of file scfstringarray.h.
References csArray< T, ElementHandler, MemoryAllocator, CapacityHandler >::IsEmpty().
virtual size_t scfStringArray::Length | ( | ) | const [inline, virtual] |
Get array length.
- Deprecated:
- Use GetSize() instead.
Implements iStringArray.
Definition at line 60 of file scfstringarray.h.
References GetSize().
virtual char* scfStringArray::Pop | ( | ) | [inline, virtual] |
Pop an element from tail end of array.
- Remarks:
- Caller is responsible for invoking delete[] on the returned string when no longer needed.
Implements iStringArray.
Definition at line 76 of file scfstringarray.h.
References csStringArray::Pop().
virtual void scfStringArray::Push | ( | char const * | value | ) | [inline, virtual] |
Push a string onto the stack.
Implements iStringArray.
Definition at line 66 of file scfstringarray.h.
References csArray< T, ElementHandler, MemoryAllocator, CapacityHandler >::Push().
virtual void scfStringArray::Sort | ( | bool | case_sensitive = true |
) | [inline, virtual] |
Sort array.
- Parameters:
-
case_sensitive If true, consider case when performing comparison. (default: yes)
Implements iStringArray.
Definition at line 140 of file scfstringarray.h.
References csStringArray::Sort().
The documentation for this class was generated from the following file:
- csutil/scfstringarray.h
Generated for Crystal Space by doxygen 1.4.7