iDocumentNode Struct Reference
[Utilities]
Representation of a node in a document.
More...
#include <iutil/document.h>
Inheritance diagram for iDocumentNode:
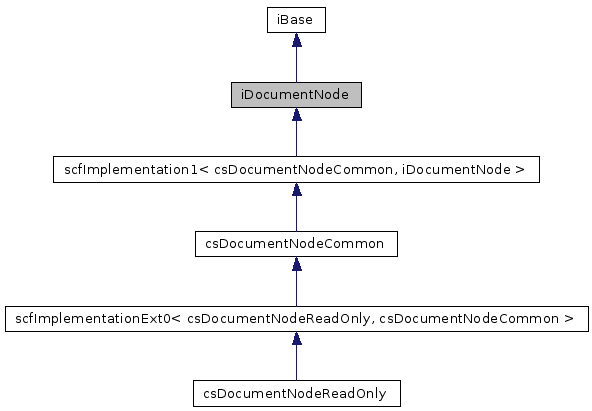
Public Member Functions | |
virtual csRef< iDocumentNode > | CreateNodeBefore (csDocumentNodeType type, iDocumentNode *before=0)=0 |
Create a new node of the given type before the given node. | |
virtual bool | Equals (iDocumentNode *other)=0 |
Compare this node with another node. | |
virtual csRef< iDocumentAttribute > | GetAttribute (const char *name)=0 |
Get an attribute by name. | |
virtual csRef< iDocumentAttributeIterator > | GetAttributes ()=0 |
Get an iterator over all attributes. | |
virtual const char * | GetAttributeValue (const char *name)=0 |
Get an attribute value by name as a string. | |
virtual bool | GetAttributeValueAsBool (const char *name, bool defaultvalue=false)=0 |
Get an attribute value by name as a bool. | |
virtual float | GetAttributeValueAsFloat (const char *name)=0 |
Get an attribute value by name as a floating point value. | |
virtual int | GetAttributeValueAsInt (const char *name)=0 |
Get an attribute value by name as an integer. | |
virtual const char * | GetContentsValue ()=0 |
Get the value of a node. | |
virtual float | GetContentsValueAsFloat ()=0 |
Get the value of a node as float. | |
virtual int | GetContentsValueAsInt ()=0 |
Get the value of a node as an integer. | |
virtual csRef< iDocumentNode > | GetNode (const char *value)=0 |
Get the first node of the given value. | |
virtual csRef< iDocumentNodeIterator > | GetNodes (const char *value)=0 |
Get an iterator over all children of the specified value. | |
virtual csRef< iDocumentNodeIterator > | GetNodes ()=0 |
Get an iterator over all children. | |
virtual csRef< iDocumentNode > | GetParent ()=0 |
Get the parent. | |
virtual csDocumentNodeType | GetType ()=0 |
Get the type of this node (one of CS_NODE_. | |
virtual const char * | GetValue ()=0 |
Get the value of this node. | |
virtual void | RemoveAttribute (const csRef< iDocumentAttribute > &attr)=0 |
Remove an attribute. | |
virtual void | RemoveAttributes ()=0 |
Remove all attributes. | |
virtual void | RemoveNode (const csRef< iDocumentNode > &child)=0 |
Remove a child. | |
virtual void | RemoveNodes ()=0 |
Remove all children. | |
virtual void | RemoveNodes (csRef< iDocumentNodeIterator > children)=0 |
Remove all children returned by iterator. | |
virtual void | SetAttribute (const char *name, const char *value)=0 |
Change or add an attribute. | |
virtual void | SetAttributeAsFloat (const char *name, float value)=0 |
Change or add an attribute to a string representation of a float. | |
virtual void | SetAttributeAsInt (const char *name, int value)=0 |
Change or add an attribute to a string representation of an integer. | |
virtual void | SetValue (const char *value)=0 |
Set the value of this node. | |
virtual void | SetValueAsFloat (float value)=0 |
Set value to the string representation of a float. | |
virtual void | SetValueAsInt (int value)=0 |
Set value to the string representation of an integer. |
Detailed Description
Representation of a node in a document.Main creators of instances implementing this interface:
Main ways to get pointers to this interface:
Definition at line 155 of file document.h.
Member Function Documentation
virtual csRef<iDocumentNode> iDocumentNode::CreateNodeBefore | ( | csDocumentNodeType | type, | |
iDocumentNode * | before = 0 | |||
) | [pure virtual] |
Create a new node of the given type before the given node.
If the given node is 0 then it will be added at the end. Returns the new node or 0 if the given type is not valid (CS_NODE_DOCUMENT is not allowed here for example).
Implemented in csDocumentNodeReadOnly.
virtual bool iDocumentNode::Equals | ( | iDocumentNode * | other | ) | [pure virtual] |
Compare this node with another node.
You have to use this function to compare document node objects as equality on the iDocumentNode pointer itself doesn't work in all cases (iDocumentNode is just a wrapper of the real node in some implementations).
- Remarks:
- Does *not* check equality of contents!
virtual csRef<iDocumentAttribute> iDocumentNode::GetAttribute | ( | const char * | name | ) | [pure virtual] |
Get an attribute by name.
Implemented in csDocumentNodeCommon.
Referenced by CS::DocumentHelper::NodeAttributeCompare::operator()().
virtual csRef<iDocumentAttributeIterator> iDocumentNode::GetAttributes | ( | ) | [pure virtual] |
virtual const char* iDocumentNode::GetAttributeValue | ( | const char * | name | ) | [pure virtual] |
Get an attribute value by name as a string.
Implemented in csDocumentNodeCommon.
Referenced by CS::DocumentHelper::NodeAttributeRegexpTest::operator()(), and CS::DocumentHelper::NodeAttributeValueTest::operator()().
virtual bool iDocumentNode::GetAttributeValueAsBool | ( | const char * | name, | |
bool | defaultvalue = false | |||
) | [pure virtual] |
Get an attribute value by name as a bool.
"yes", "true", and "1" all are returned as true.
Implemented in csDocumentNodeCommon.
virtual float iDocumentNode::GetAttributeValueAsFloat | ( | const char * | name | ) | [pure virtual] |
virtual int iDocumentNode::GetAttributeValueAsInt | ( | const char * | name | ) | [pure virtual] |
virtual const char* iDocumentNode::GetContentsValue | ( | ) | [pure virtual] |
Get the value of a node.
Scans all child nodes and looks for a node of type 'text', and returns the text of that node. WARNING! Make a copy of this text if you intend to use it later. The document parsing system does not guarantee anything about the lifetime of the returned string.
Implemented in csDocumentNodeCommon.
virtual float iDocumentNode::GetContentsValueAsFloat | ( | ) | [pure virtual] |
Get the value of a node as float.
Scans all child nodes and looks for a node of type 'text', converts the text of that node to a number and returns the represented floating point value.
Implemented in csDocumentNodeCommon.
virtual int iDocumentNode::GetContentsValueAsInt | ( | ) | [pure virtual] |
Get the value of a node as an integer.
Scans all child nodes and looks for a node of type 'text', converts the text of that node to a number and returns the represented integer.
Implemented in csDocumentNodeCommon.
virtual csRef<iDocumentNode> iDocumentNode::GetNode | ( | const char * | value | ) | [pure virtual] |
virtual csRef<iDocumentNodeIterator> iDocumentNode::GetNodes | ( | const char * | value | ) | [pure virtual] |
Get an iterator over all children of the specified value.
- Remarks:
- Never returns 0.
Implemented in csDocumentNodeCommon.
virtual csRef<iDocumentNodeIterator> iDocumentNode::GetNodes | ( | ) | [pure virtual] |
Get an iterator over all children.
- Remarks:
- Never returns 0.
Implemented in csDocumentNodeCommon.
Referenced by CS::DocumentHelper::RemoveDuplicateChildren().
virtual csRef<iDocumentNode> iDocumentNode::GetParent | ( | ) | [pure virtual] |
Get the parent.
virtual csDocumentNodeType iDocumentNode::GetType | ( | ) | [pure virtual] |
Get the type of this node (one of CS_NODE_.
..).
Referenced by CS::DocumentHelper::NodeAttributeCompare::operator()(), and CS::DocumentHelper::NodeNameCompare::operator()().
virtual const char* iDocumentNode::GetValue | ( | ) | [pure virtual] |
Get the value of this node.
What this is depends on the type of the node:
- CS_NODE_DOCUMENT: filename of the document file
- CS_NODE_ELEMENT: name of the element
- CS_NODE_COMMENT: comment text
- CS_NODE_UNKNOWN: tag contents
- CS_NODE_TEXT: text string
- CS_NODE_DECLARATION: undefined
Referenced by CS::DocumentHelper::NodeValueTest::operator()(), and CS::DocumentHelper::NodeNameCompare::operator()().
virtual void iDocumentNode::RemoveAttribute | ( | const csRef< iDocumentAttribute > & | attr | ) | [pure virtual] |
virtual void iDocumentNode::RemoveAttributes | ( | ) | [pure virtual] |
virtual void iDocumentNode::RemoveNode | ( | const csRef< iDocumentNode > & | child | ) | [pure virtual] |
Remove a child.
Implemented in csDocumentNodeReadOnly.
Referenced by CS::DocumentHelper::RemoveDuplicateChildren().
virtual void iDocumentNode::RemoveNodes | ( | ) | [pure virtual] |
virtual void iDocumentNode::RemoveNodes | ( | csRef< iDocumentNodeIterator > | children | ) | [pure virtual] |
virtual void iDocumentNode::SetAttribute | ( | const char * | name, | |
const char * | value | |||
) | [pure virtual] |
virtual void iDocumentNode::SetAttributeAsFloat | ( | const char * | name, | |
float | value | |||
) | [pure virtual] |
Change or add an attribute to a string representation of a float.
Implemented in csDocumentNodeCommon, and csDocumentNodeReadOnly.
virtual void iDocumentNode::SetAttributeAsInt | ( | const char * | name, | |
int | value | |||
) | [pure virtual] |
Change or add an attribute to a string representation of an integer.
Implemented in csDocumentNodeCommon, and csDocumentNodeReadOnly.
virtual void iDocumentNode::SetValue | ( | const char * | value | ) | [pure virtual] |
Set the value of this node.
What this is depends on the type of the node:
- CS_NODE_DOCUMENT: filename of the document file
- CS_NODE_ELEMENT: name of the element
- CS_NODE_COMMENT: comment text
- CS_NODE_UNKNOWN: tag contents
- CS_NODE_TEXT: text string
- CS_NODE_DECLARATION: undefined
Implemented in csDocumentNodeReadOnly.
virtual void iDocumentNode::SetValueAsFloat | ( | float | value | ) | [pure virtual] |
Set value to the string representation of a float.
Implemented in csDocumentNodeCommon, and csDocumentNodeReadOnly.
virtual void iDocumentNode::SetValueAsInt | ( | int | value | ) | [pure virtual] |
Set value to the string representation of an integer.
Implemented in csDocumentNodeCommon, and csDocumentNodeReadOnly.
The documentation for this struct was generated from the following file:
- iutil/document.h
Generated for Crystal Space by doxygen 1.4.7