iScriptObject Struct Reference
This provides the interface to an object in an object-oriented scripting language. More...
#include <ivaria/script.h>
Inheritance diagram for iScriptObject:
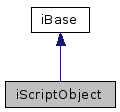
Public Member Functions | |
virtual bool | Call (const char *name, csRef< iScriptObject > &, const char *fmt,...)=0 |
Call a method in the object, with object return value. | |
virtual bool | Call (const char *name, csRef< iString > &, const char *fmt,...)=0 |
Call a method in the object, with string return value. | |
virtual bool | Call (const char *name, double &ret, const char *fmt,...)=0 |
Call a method in the object, with double return value. | |
virtual bool | Call (const char *name, float &ret, const char *fmt,...)=0 |
Call a method in the object, with float return value. | |
virtual bool | Call (const char *name, int &ret, const char *fmt,...)=0 |
Call a method in the object, with int return value. | |
virtual bool | Call (const char *name, const char *format,...)=0 |
Call a method in the object, with no return value. | |
virtual bool | Get (const char *name, csRef< iScriptObject > &) const =0 |
Get the value of an object variable in the script interpreter. | |
virtual bool | Get (const char *name, csRef< iString > &) const =0 |
Get the value of a string variable in the script interpreter. | |
virtual bool | Get (const char *name, double &data) const =0 |
Get the value of a double variable in the script interpreter. | |
virtual bool | Get (const char *name, float &data) const =0 |
Get the value of a float variable in the script interpreter. | |
virtual bool | Get (const char *name, int &data) const =0 |
Get the value of an int variable in the script interpreter. | |
virtual iBase * | GetPointer () const =0 |
If the object is an interface pointer from the cspace module, this will return its value, otherwise 0. | |
virtual bool | GetTruth (const char *name, bool &isTrue) const =0 |
Get the value of a bool variable in the script interpreter. | |
virtual bool | IsType (const char *) const =0 |
Returns a boolean specifying whether or not the object is derived from the given type. | |
virtual bool | Set (const char *name, iScriptObject *data)=0 |
Set the value of an object variable in the script interpreter. | |
virtual bool | Set (const char *name, char const *data)=0 |
Set the value of a string variable in the script interpreter. | |
virtual bool | Set (const char *name, double data)=0 |
Set the value of a double variable in the script interpreter. | |
virtual bool | Set (const char *name, float data)=0 |
Set the value of a float variable in the script interpreter. | |
virtual bool | Set (const char *name, int data)=0 |
Set the value of an int variable in the script interpreter. | |
virtual bool | SetPointer (iBase *)=0 |
If the object is an interface pointer from the cspace module, this will set its value and return true, otherwise false. | |
virtual bool | SetTruth (const char *name, bool isTrue)=0 |
Set the value of a bool variable in the script interpreter. |
Detailed Description
This provides the interface to an object in an object-oriented scripting language.Several functions here take a variable-length argument list with a printf-style format string supporting all the argument types supported by printf, except width and precision specifiers, as they have no meaning here. The type specifier "%p" signifies an iScriptObject. Remember to explicitly cast your csRef's to plain pointers in the var arg list.
Definition at line 43 of file script.h.
Member Function Documentation
virtual bool iScriptObject::Call | ( | const char * | name, | |
csRef< iScriptObject > & | , | |||
const char * | fmt, | |||
... | ||||
) | [pure virtual] |
Call a method in the object, with object return value.
Returns false if the subroutine named does not exist. Format is a printf-style format string for the arguments.
virtual bool iScriptObject::Call | ( | const char * | name, | |
csRef< iString > & | , | |||
const char * | fmt, | |||
... | ||||
) | [pure virtual] |
Call a method in the object, with string return value.
Returns false if the subroutine named does not exist. Format is a printf-style format string for the arguments.
virtual bool iScriptObject::Call | ( | const char * | name, | |
double & | ret, | |||
const char * | fmt, | |||
... | ||||
) | [pure virtual] |
Call a method in the object, with double return value.
Returns false if the subroutine named does not exist. Format is a printf-style format string for the arguments.
virtual bool iScriptObject::Call | ( | const char * | name, | |
float & | ret, | |||
const char * | fmt, | |||
... | ||||
) | [pure virtual] |
Call a method in the object, with float return value.
Returns false if the subroutine named does not exist. Format is a printf-style format string for the arguments.
virtual bool iScriptObject::Call | ( | const char * | name, | |
int & | ret, | |||
const char * | fmt, | |||
... | ||||
) | [pure virtual] |
Call a method in the object, with int return value.
Returns false if the subroutine named does not exist. Format is a printf-style format string for the arguments.
virtual bool iScriptObject::Call | ( | const char * | name, | |
const char * | format, | |||
... | ||||
) | [pure virtual] |
Call a method in the object, with no return value.
Returns false if the subroutine named does not exist. Format is a printf-style format string for the arguments.
virtual bool iScriptObject::Get | ( | const char * | name, | |
csRef< iScriptObject > & | ||||
) | const [pure virtual] |
Get the value of an object variable in the script interpreter.
Returns false if the named variable does not exist.
Get the value of a string variable in the script interpreter.
Returns false if the named property does not exist.
virtual bool iScriptObject::Get | ( | const char * | name, | |
double & | data | |||
) | const [pure virtual] |
Get the value of a double variable in the script interpreter.
Returns false if the named property does not exist.
virtual bool iScriptObject::Get | ( | const char * | name, | |
float & | data | |||
) | const [pure virtual] |
Get the value of a float variable in the script interpreter.
Returns false if the named property does not exist.
virtual bool iScriptObject::Get | ( | const char * | name, | |
int & | data | |||
) | const [pure virtual] |
Get the value of an int variable in the script interpreter.
Returns false if the named property does not exist.
virtual iBase* iScriptObject::GetPointer | ( | ) | const [pure virtual] |
If the object is an interface pointer from the cspace module, this will return its value, otherwise 0.
virtual bool iScriptObject::GetTruth | ( | const char * | name, | |
bool & | isTrue | |||
) | const [pure virtual] |
Get the value of a bool variable in the script interpreter.
Returns false if the named property does not exist.
virtual bool iScriptObject::IsType | ( | const char * | ) | const [pure virtual] |
Returns a boolean specifying whether or not the object is derived from the given type.
virtual bool iScriptObject::Set | ( | const char * | name, | |
iScriptObject * | data | |||
) | [pure virtual] |
Set the value of an object variable in the script interpreter.
Returns false if the named property does not exist.
virtual bool iScriptObject::Set | ( | const char * | name, | |
char const * | data | |||
) | [pure virtual] |
Set the value of a string variable in the script interpreter.
Returns false if the named property does not exist.
virtual bool iScriptObject::Set | ( | const char * | name, | |
double | data | |||
) | [pure virtual] |
Set the value of a double variable in the script interpreter.
Returns false if the named property does not exist.
virtual bool iScriptObject::Set | ( | const char * | name, | |
float | data | |||
) | [pure virtual] |
Set the value of a float variable in the script interpreter.
Returns false if the named property does not exist.
virtual bool iScriptObject::Set | ( | const char * | name, | |
int | data | |||
) | [pure virtual] |
Set the value of an int variable in the script interpreter.
Returns false if the named property does not exist.
virtual bool iScriptObject::SetPointer | ( | iBase * | ) | [pure virtual] |
If the object is an interface pointer from the cspace module, this will set its value and return true, otherwise false.
virtual bool iScriptObject::SetTruth | ( | const char * | name, | |
bool | isTrue | |||
) | [pure virtual] |
Set the value of a bool variable in the script interpreter.
Returns false if the named property does not exist.
The documentation for this struct was generated from the following file:
- ivaria/script.h
Generated for Crystal Space by doxygen 1.4.7