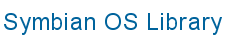
![]() |
![]() |
|
SingleRequest
: asynchronous programming without
active objects
These files are found in:
examples\Base\IPC\Async\SingleRequest
The files reproduced here are the main files contained in the examples directory. Some extra files may be needed to run the examples, and these will be found in the appropriate examples directory.
// SingleRequest.cpp
//
// Copyright (C) Symbian Software Ltd 2000-2005. All rights reserved.
//
// Shows asynchronous programming (without active objects).
// Example shows how a single request can be issued, followed
// by User::WaitForRequest()
#include "CommonFramework.h"
// Do the example
LOCAL_C void doExampleL()
{
// create and initialize heartbeat timer
RTimer heartbeat; // heartbeat timer
TRequestStatus heartbeatStatus; // request status associated with it
heartbeat.CreateLocal(); // always created for this thread
// go round timing loop
for (TInt i=0; i<10; i++)
{
// issue and wait for single request
heartbeat.After(heartbeatStatus,1000000); // wait 1 second
User::WaitForRequest(heartbeatStatus); // wait for request
// to complete
// say we're here
_LIT(KFormatString1,"Tick %d\n");
console->Printf(KFormatString1, i);
}
// close timer
heartbeat.Close(); // close timer
}
This file is found in examples\Base\CommonFramework
// EUSTD.H
//
// Copyright (C) Symbian Software Ltd 1997-2005. All rights reserved.
//
#ifndef __EUSTD_H
#define __EUSTD_H
#include <e32base.h>
#include <e32cons.h>
_LIT(KTxtEPOC32EX,"EPOC32EX");
_LIT(KTxtExampleCode,"E32 SDK Example Code");
_LIT(KFormatFailed,"failed: leave code=%d");
_LIT(KTxtOK,"ok");
_LIT(KTxtPressAnyKey," [press any key]");
// public
LOCAL_D CConsoleBase* console; // write all your messages to this
LOCAL_C void doExampleL(); // code this function for the real example
// private
LOCAL_C void callExampleL(); // initialize with cleanup stack, then do example
GLDEF_C TInt E32Main() // main function called by E32
{
__UHEAP_MARK;
CTrapCleanup* cleanup=CTrapCleanup::New(); // get clean-up stack
TRAPD(error,callExampleL()); // more initialization, then do example
__ASSERT_ALWAYS(!error,User::Panic(KTxtEPOC32EX,error));
delete cleanup; // destroy clean-up stack
__UHEAP_MARKEND;
return 0; // and return
}
LOCAL_C void callExampleL() // initialize and call example code under cleanup stack
{
console=Console::NewL(KTxtExampleCode,TSize(KConsFullScreen,KConsFullScreen));
CleanupStack::PushL(console);
TRAPD(error,doExampleL()); // perform example function
if (error)
console->Printf(KFormatFailed, error);
else
console->Printf(KTxtOK);
console->Printf(KTxtPressAnyKey);
console->Getch(); // get and ignore character
CleanupStack::PopAndDestroy(); // close console
}
#endif
// BLD.INF
// Component description file
// Copyright (C) Symbian Software Ltd 2000-2005. All rights reserved.
PRJ_MMPFILES
SingleRequest.mmp
// SingleRequest.mmp
//
// Copyright (C) Symbian Software Ltd 2000-2005. All rights reserved.
// using relative paths for source and userinclude directories
// No explicit capabilities required to run this.
TARGET SingleRequest.exe
TARGETTYPE exe
UID 0
VENDORID 0x70000001
SOURCEPATH .
SOURCE SingleRequest.cpp
USERINCLUDE .
USERINCLUDE ..\..\..\CommonFramework
SYSTEMINCLUDE \Epoc32\include
LIBRARY euser.lib
CAPABILITY None
This example shows how to issue and wait for a single request.
The example shows the general principles involved in asynchronous programming. It uses a simple wait loop and shows how the completion of asynchronous events are handled without active objects.
This example deliberately does not use active objects.
The example requires no specific capabilities in order to run - and does not demonstrate any security issues.
This example shows how a wait loop can be used to identify and handle a completed request.
It shows the general principles involved in asynchronous programming. It uses a simple wait loop and shows how the completion of asynchronous events are handled without active objects.
This example deliberately does not use active objects.
The example requires no specific capabilities in order to run - and does not demonstrate any security issues.
As with the WaitLoop example, this example shows how a wait loop can be used to identify and handle a completed request. However, this example shows how the wait loop can deal with multiple asynchronous service providers.
The example shows the general principles involved in asynchronous programming; it uses a simple wait loop and shows how the completion of asynchronous events are handled without active objects.
This example deliberately does not use active objects.
The example requires no specific capabilities in order to run - and does not demonstrate any security issues.
The example shows how active objects and an active scheduler can be used to handle asynchronous events. Compare this with the following examples; SingleRequest, WaitLoop and RealLifeWaitLoop.
It demonstrates a single CMessageTimer
active object
which runs until completion.
TRequestStatus
: asynchronous request status
CActiveScheduler
: the active scheduler
CActive
: active object abstraction
CTimer
: timer base class (used here as a source of
events)
The example requires no specific capabilities in order to run - and does not demonstrate any security issues.
These examples show how active objects and an active scheduler can be used to handle asynchronous events.
They demonstrate a single CKeyMessengerProcessor
active
object (derived from class CActiveConsole
), which accepts input
from keyboard, but does not print it. This object contains a
CMessageTimer
object which it activates if the user inputs the
character "m" and cancelled if the user inputs "c".
TRequestStatus
: asynchronous request status
CActiveScheduler
: the active scheduler
CActive
: active object abstraction
CTimer
: timer base class (used here as a source of
events)
The example requires no specific capabilities in order to run - and does not demonstrate any security issues.
These examples show how active objects and an active scheduler can be used to handle asynchronous events and long-running services to maintain system responsiveness.
TRequestStatus
: asynchronous request status
CActiveScheduler
: the active scheduler
CActive
: active object abstraction
CTimer
: timer base class (used here as a source of
events)
The example requires no specific capabilities in order to run - and does not demonstrate any security issues.