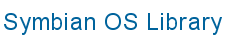
![]() |
![]() |
|
You can create a URI by one of the following 4 methods using
CUri8
and UriUtils
classes:
Create from parts (components)
You can create a URI by adding each component. This is done by calling
CUri8::SetComponentL()
repeatedly for each component.
CUri8
supports modification of URI components. It also
provides reference to a TUriC8
object to use the
non-modifying functionality that is provided by TUriC8
.
The code below constructs a CUri
object from parts
such as scheme, host and user info components.
TUriC8 pUri;
CUri8* uri = CUri8::NewL(pUri); //create a pointer to CUri8 object, pass a reference to a parsed TUriC8 object
NewL()
and NewLC()
methods have overloads that
construct an empty URI object.
// Add components to build a URI
_LIT8(KScheme0, "http");
_LIT8(KHost0, "www.mypage.com");
_LIT8(KUserInfo0, "user:pass");
CUri8* uri = CUri8::NewLC();
uri->SetComponentL(KScheme0, EUriScheme); //set the scheme component
uri->SetComponentL(KHost0, EUriHost); //set the host component
uri->SetComponentL(KUserInfo0, EUriUserinfo); //set the user info component
const TDesC8& des = uri->Uri().UriDes();/ / retrieve the created URI
The descriptor des
contains the URI
"http://user:[email protected]".
You can remove a specific component from a URI using
CUri8::RemoveComponentL()
. The code below removes the
scheme component of the URI. If the component does not exist, this function
does nothing.
uri8->RemoveComponentL(EUriScheme); //remove the scheme component
const TDesC8& des8 = uri8->Uri().UriDes(); //retrieve the URI
des
contains the remaining part of URI that is
"user:[email protected]", after removing "http", the scheme component.
Similar to a URI, authority components can be created from its
components. This is done by adding the user info, host and port by calling
CAuthority8::SetComponentL()
repeatedly.
Parse the component that needs to be added calling
TAuthorityParser8::Parse()
and create an object using
CAuthority8::NewL()
.
TAuthorityParser8 authorityParser;
_LIT8(KAuthority,"www.symbian.com");
authorityParser.Parse(KAuthority);//parse the authority component
CAuthority8* authority = CAuthority8::NewL(authorityParser);// create an authority object
// Add components to the Authority
_LIT8(KUserinfo,"user:info");
authority->SetComponentL(KUserinfo,EAuthorityUserinfo);//set the userinfo
_LIT8(KHost, "www.symbian.com");
authority->SetComponentL(KHost,EAuthorityHost);//set the host
_LIT8(KPort,"80");
authority->SetComponentL(KPort,EAuthorityPort); //set the port
const TDesC8& authorityDes = authority->Authority().AuthorityDes(); // get the authority component
authorityDes
descriptor contains the complete authority
component "user:[email protected]:80
".
CAuthority8::SetAndEscapeComponentL()
allows you
to escape encode and set the authority component in a URI. This escapes any
unsafe data before setting the component.
CAuthority8* authority = CAuthority8::NewL(authorityParser);
authority->SetAndEscapeComponentL(KUserinfo,EAuthorityUserinfo); //escape encode and set user info component
CAuthority8::RemoveComponentL()
removes a
component, specified with a TAuthorityComponent
component,
from the authority data.
//Remove the user info component from the URI
authority->RemoveComponentL(EAuthorityUserinfo);
To create a file URI object, call
CUri8::CreateFileUriL()
.
The code fragment below creates the file URI object.
//Set the physical path of the file
LIT(KFullUriName, "D:\\MyFolder\\MyDoc.doc");
//create the Uri for the path component
CUri8* pathUri8 = CUri8::CreateFileUriL(KFullUriName,0);
The code returns the URI for the specified file path. Provide the full
file name descriptor. For example, D:\\MyFolder\\MyDoc.doc
and
specify whether the drive on which the file exists is fixed or external. 0
indicates a fixed drive, otherwise specify EExtMedia
.
If the file exists on:
a fixed drive, then the URI takes the form file:///private/<drive-letter>/<filepath>
a removable drive, then the URI takes the form file:///ext-media/<filepath> or file:///private/ext-media/<filepath> .
If the file is private to the application, then use
CUri8::CreatePrivateFileUriL()
instead of
CUri8::CreateFileUriL()
.
This section explains how to create URIs from base and reference URIs.
Resolving is the process of interpreting what the string means, stripping it into some component parts and then using those to decide on the following:
How to look something up interpreting the raw content that was sent back to us.
What we want to look up for that identifier.
Once we have looked it up, what protocol is needed to acquire it.
Interpreting the raw content that was sent back to us.
The first one is called resolving. In this context, resolving is the
process of converting a relative URI reference (for example,
../../../resource.txt
) to its absolute form, for example,
http://somehost/resource.txt
.
You need to resolve the URI to know what the identifier actually points to.
The code below resolves the reference URI against the base URI and returns an absolute URI.
Create the base and reference URIs from its components using SetComponentL(). Refer to Creating a URI from parts.
_LIT8(KBase,"http://www.mypage.com/folder1/folder2/index.html");
_LIT8(KReference, "../../empdetail.html");
TUriParser8 baseUri;//base URI object
TInt error = baseUri.Parse(KBase);//parse the base URI
TUriParser8 refUri;//reference URI object
error = refUri.Parse(KReference);/parse the reference URI
CUri8* resolvedUri = NULL;
resolvedUri = CUri8::ResolveL(baseUri, refUri); //resolve the reference URI against base URI
const TDesC8& des1 = resolvedUri->Uri().UriDes(); //retrieve the resolved URI
The code above resolves the reference URI against the base URI and
returns an absolute URI, "http://www.mypage.com/empdetail.html
" in
this case.
UriUtils::CreateUriL()
creates a
CUri8
object from a Unicode descriptor.
_LIT8(KUri,"http://web.intra/Dev/Sysdoc/devlib.htm");
CUri8* uri8= UriUtils::CreateUriL(KUri);
This returns a new CUri8
object. It returns
EUriUtilsParserErrInvalidUri
if the descriptor is an invalid URI.
You can create an authority component of URI from a descriptor calling
UriUtils::CreateAuthorityL()
.