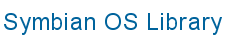
![]() |
![]() |
|
The steps required to write a rasterizer DLL are:
Obtain or write code that can read and process font files of the desired format. It is usually more practical to get either freeware or paid-for code, than to write it in-house. It may take over a year to write a rasterizer, even for an expert programmer with the right domain knowledge.
Implement an exported NewL()
factory function to
create rasterizer objects (which you derive from
COpenFontRasterizer
). You may choose to implement this as part of
your rasterizer object.
Write a class derived from COpenFontRasterizer
. This
will need to store any context associated with the rasterization process rather
than with individual typefaces. It also implements a function to create your
COpenFontFile
derived objects.
Write a class derived from COpenFontFile
. This class
manages a single file representing a typeface.
Write a class derived from COpenFont
.
Place all the code into a DLL of the right type and provide an exported function to create the rasterizer object.
Deriving from COpenFontRasterizer
,
COpenFontFile
and COpenFont
are discussed in the "See
also" topics below. This section describes the operations required to package
the code as a polymorphic DLL.
Note that only one Open Font System rasterizer has so far been released: FreeType. It uses freeware code from the FreeType project (see http://www.freetype.org) to provide a TrueType rasterizer. Fragments of code given in this document are taken from this implementation.
How to derive from COpenFontRasterizer
How to derive from COpenFontFile
How to derive from COpenFontRasterizerContext
A rasterizer DLL is an ordinary Symbian polymorphic DLL. It exports just one function and has no unusual behaviour.
In particular, it must have no writable static data. This is an important constraint on the use of code from outside sources.
The exported function is a factory function that creates objects of a
class derived from COpenFontRasterizer
. It will be called just
once — when the Font and Bitmap Server loads the DLL.
Although the name of the function does not matter because it is loaded
by ordinal, implementers should follow the practice of making it a static
member of the rasterizer class, called NewL()
. Thus, if the
rasterizer class is CExampleRasterizer
(derived from
COpenFontRasterizer
), the exported function is:
static COpenFontRasterizer* CExampleRasterizer::NewL()
The function must take no arguments. It must create a
COpenFontRasterizer
derived object on the heap, returning it to
the caller. The caller is responsible for deleting the object. As the name of
the example function suggests, it is allowed to leave.
The exported function used in the FreeType rasterizer is given below:
EXPORT_C COpenFontRasterizer* CFreeTypeRasterizer::NewL()
{
CFreeTypeRasterizer* r = new(ELeave) CFreeTypeRasterizer;
CleanupStack::PushL(r);
r->iContext = CFreeTypeContext::NewL();
CleanupStack::Pop();
return r;
}
The name of the rasterizer DLL, its type, and its UIDs are all specified in the makmake project file. Note that:
The target output file extension must be
.dll
. Only rasterizer DLLs with this extenstion are loaded during
Font and Bitmap Server startup.
The targetpath is \resources\fonts\
. This
is the location from which rasterizers are automatically loaded during Font and
Bitmap Server startup.
The targettype must be dll. This sets the
project's UID1 value, identifying it as a DLL. The value of this UID is
KDynamicLibraryUidValue
(0x10000079).
The UID line identifies the project's UID2 and UID3
values. UID2 must identify the file as an Open Font System rasterizer, i.e. be
equal to KUidOpenFontRasterizerValue
(0x10003B1F).
UID3 identifies the font format that is supported. Standard values
are KUidTrueTypeRasterizerValue
(0x100012A7) for TrueType fonts,
KUidTrueDocRasterizerValue
(0x100012A8) for the TrueDoc format
invented by BitStream, Inc., and KUidAdobeType1RasterizerValue
(0x100012A9) for the Adobe Type 1 format invented by Adobe, Inc.
If the DLL is for some other font format a new UID3 must be obtained from Symbian.
A fragment of the project file for the freetype DLL is given below.
target freetype.dll
targetpath \system\fonts
targettype dll
UID 0x10003B1F 0x100012A7
Rasterizer DLLs must #include
the following header files,
which are located in the standard include directory
\epoc32\include\
:
openfont.h
for the Open Font System classes
e32uid.h
for the UID value for a dynamic library
f32file.h
for the file handling classes