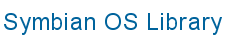
![]() |
![]() |
|
SIP (Session Initiation Protocol) is the application layer signalling protocol for creating, modifying and terminating session(s) with participant(s). SIP extensions are used for messaging, subscribing to events, receiving event notifications and publishing presence information. SIP has been adopted by the 3GPP and is used in IMS Release 5. For more information, see:
SIP can run on top of several different transport protocols.
The SIP Client API provides access to the basic services of the SIP stack. The most essential services are the sending and receiving of SIP messages, creating registrations, forming and tearing down dialogs initiated by INVITE, REFER and SUBSCRIBE requests.
The SIP Client API is intended for use by applications that want to manage multimedia sessions, subscribe to events and send stand-alone SIP messages.
Interaction with the SIP stack is mediated through a client-server mechanism. This means that more than one client can use the SIP stack concurrently.
Calls to the SIP Client API are synchronous. Clients need to implement a set of callback functions, through which the SIP Client API can pass events back to the application.
The following list describes the capabilities you need, and the specific circumstances in which they are needed:
|
An application needs to create a single instance of the
CSIP
class, and implement the callback functions defined
by MSIPObserver
. The MSIPObserver
functions
are used by CSIP
.
The CSIP
class allows you to query the supported
security mechanisms, and to find out whether a
CSIPConnection
object exists for a given IAP.
Once the CSIP
object has been created, it is
possible to create an instance of CSIPConnection
. This
class allows you to send stand-alone SIP requests and ask for the state of the
network connection. Responses are received through the
MSIPConnectionObserver
interface. This class defines a set
of callback functions that an application must implement.
Note that an application can have multiple
CSIPConnection
objects, but each of them has to have a
different IAP.
The CSIPRegistrationBinding
class provides the
services for registering, updating the registration and deregistering. It is
also possible to instruct the SIP stack to automatically refresh the
registration, and to query and set the outbound proxy used by the registration.
Creating an instance of CSIPRegistrationBinding
requires an
existing CSIPConnection
.
CSIPDialogAssocBase
is the base class for SIP
dialog associations, specifically for
CSIPInviteDialogAssoc
,
CSIPSubscribeDialogAssoc
,
CSIPReferDialogAssoc
and
CSIPNotifyDialogAssoc
.
CSIPDialogAssocBase
provides the services for
getting the associated SIP dialog, i.e. the associated
CSIPDialog
instance, and for sending a non-target Refresh
request within the dialog.
Note that CSIPDialog
is instantiated by the SIP
Client API; it cannot be created by the application.
If any of the CSIPInviteDialogAssoc
,
CSIPSubscribeDialogAssoc
,
CSIPReferDialogAssoc
and
CSIPNotifyDialogAssoc
objects are associated, they can be
bound to an existing CSIPDialog
object, or they can be
used to create a new CSIPDialog
object.
Note:
CSIPInviteDialogAssoc
allows an application
to initiate a dialog and to send INVITE
, PRACK
,
ACK
, UPDATE
and BYE
requests related to
the dialog.
CSIPSubscribeDialogAssoc
allows an
application to subscribe to different events by sending SUBSCRIBE and
(un)SUBSCRIBE requests. It is also possible to order the SIP stack to
automatically refresh the SUBSCRIBE request.
CSIPReferDialogAssoc
allows an application
to define and send REFER requests.
CSIPNotifyDialogAssoc
allows an application
to define and send NOTIFY requests.
When an application invokes a function that causes a SIP request to
be sent to the network, the SIP Client API implementation creates
a new CSIPClientTransaction
object and returns it to the
application. A CSIPClientTransaction
object represents the SIP
transaction and consists of the SIP request and the SIP response. Once a
response has been received, the application can get
CSIPResponseElements
from the
CSIPClientTransaction
object. Note that some client transactions
can also be cancelled or refreshed.
When a SIP request is received from the network, it is
passed to the application as a CSIPServerTransaction
object. The application can get CSIPRequestElements
from
the CSIPServerTransaction
object. A
CSIPRequestElements
object describes the kind of SIP request
received. Once the application has decided what kind of response(s) it is going
to send back, it uses the CSIPServerTransaction
to do that.
CSIPTransactionBase is the base class for
CSIPClientTransaction
and
CSIPServerTransaction
; it provides functions that get the
current state of the transaction and the transaction type. Some operations are
only allowed when the transaction is in a certain state.
Note that CSIPClientTransaction
and
CSIPServerTransaction
are instantiated by the SIP Client
API; they cannot be created by the application.
When an application invokes a function that causes a SIP request to
be sent to the network, the SIP Client API implementation creates
a new CSIPClientTransaction
object and returns it to the
application. A CSIPClientTransaction
object represents the SIP
transaction and consists of the SIP request and the SIP response. Once a
response has been received, the application can get
CSIPResponseElements
from the
CSIPClientTransaction
object. Note that some client transactions
can also be cancelled or refreshed.
When a SIP request is received from the network, it is
passed to the application as a CSIPServerTransaction
object. The application can get CSIPRequestElements
from
the CSIPServerTransaction
object. A
CSIPRequestElements
object describes the kind of SIP request
received. Once the application has decided what kind of response(s) it is going
to send back, it uses the CSIPServerTransaction
to do that.
CSIPTransactionBase is the base class for
CSIPClientTransaction
and
CSIPServerTransaction
; it provides functions that get the
current state of the transaction and the transaction type. Some operations are
only allowed when the transaction is in a certain state.
Note that CSIPClientTransaction
and
CSIPServerTransaction
are instantiated by the SIP Client
API; they cannot be created by the application.
Use a CSIPRefresh
object to refresh a SIP
request automatically by the SIP stack. REGISTER
,
SUBSCRIBE
and a request outside of a dialog can be refreshed. Once
a refreshed request has been sent, the application can query the state of the
refresh from CSIPRefresh
. For requests outside of a dialog,
CSIPRefresh
provides functions for updating and terminating the
refresh. Updating and terminating a refreshed REGISTER
or
SUBSCRIBE
is done using the
CSIPRegistrationBinding
and
CSIPSubscribeDialogAssoc
classes instead of using
CSIPRefresh
.
The CSIPHttpDigest
class contains only static
functions. It provides ways to manage HTTP digest security settings. You need
an instance of the CSIP
class before you can use
CSIPHttpDigest
.
After the CSIP
object and the required number
of CSIPConnection
objects have been created, most of the
memory consumption is incurred by the CSIPMessageElements
,
CSIPRequestElements
and
CSIPResponseElements
classes.
CSIPTransactionBase
holds the most recent SIP
response in a CSIPResponseElements
object. When another
response is received, the previous CSIPResponseElements
object is deleted and a new one created.
CSIPClientTransaction
contains a
CSIPRequestElements
object for the length of time it takes
to transfer the SIP request to the server side of the SIP stack.
CSIPServerTransaction
contains the SIP request as
CSIPRequestElements
.
As the application owns the CSIPClientTransaction
and CSIPServerTransaction
objects, it is recommended that
the application delete them as soon as the transaction is no longer needed -
this acts to conserve memory. A non-INVITE
CSIPClientTransaction
can be deleted when it has either received a
final response or the ErrorOccured()
callback has been called. A
non-INVITE
CSIPServerTransaction
can be deleted after
the application has sent a final response.
The INVITE
related transactions behave differently because
of the presence of an ACK
. They should be deleted if the
ErrorOccured()
callback function is called. When the application
sends a 2xx response to an INVITE
related
CSIPServerTransaction
, it will enter the state
CSIPTransactionBase::ETerminated
and can be deleted at
this point. The ACK
will be received with a new
CSIPServerTransaction
object. However, when the application has
sent an INVITE
request and the returned
CSIPClientTransaction
has received a 2xx response, the transaction
enters the state CSIPTransactionBase::ETerminated
. The associated
CSIPClientTransaction
should not be deleted yet since it will be
needed for sending the ACK
and also to receive the
MSIPConnectionObserver::InviteCompleted()
callback. So, an
INVITE
related CSIPClientTransaction
should be
deleted only after a MSIPConnectionObserver::InviteCompleted
or
MSIPConnectionObserver ::ErrorOccured
event has been
received, or if the state of the associated CSIPConnection
goes to CSIPConnection::EInactive
or
CSIPConnection::EUnavailable
.