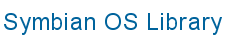
![]() |
![]() |
|
CTelephony::SendDTMFTones()
transmits a sequence
of DTMF tones across the currently active call. The sequence of tones is a
TDesC
string. It can contain one or more occurance of the
numbers 0 to 9, * and #.
SendDTMFTones()
is asynchronous; use
CTelephony::ESendDTMFTonesCancel
to cancel it.
This example sends the string 123456789
:
#include <e32base.h>
#include <Etel3rdParty.h>
_LIT(KTheTones, "123456789");
class CClientApp : public CActive
{
private:
CTelephony* iTelephony;
public:
CClientApp(CTelephony* aTelephony);
void SomeFunction();
private:
/*
These are the pure virtual methods from CActive that
MUST be implemented by all active objects
*/
void RunL();
void DoCancel();
};
CClientApp::CClientApp(CTelephony* aTelephony)
: CActive(EPriorityStandard),
iTelephony(aTelephony)
{
//default constructor
}
void CClientApp::SomeFunction()
{
iTelephony->SendDTMFTones(iStatus, KTheTones);
SetActive();
}
void CClientApp::RunL()
{
if(iStatus==KErrNone)
{} // The tones were sent successfully;
}
void CClientApp::DoCancel()
{
iTelephony->CancelAsync(CTelephony::ESendDTMFTonesCancel);
}
Remember to link to the Etel3rdParty.lib
and
euser.lib
libraries.