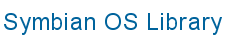
![]() |
![]() |
|
CTelephony::Hold()
puts a call on hold. Pass
it the ID of the call to hold. The ID is the
CTelephony::TCallId
returned when you dialled or answered
the call.
Before calling CTelephony::Hold()
, check that your
phone supports call holding; see Dynamic call capabilities.
CTelephony::Hold()
is asynchronous; use
CTelephony::EHoldCancel
to cancel.
For example:
#include <e32base.h>
#include <Etel3rdParty.h>
class CClientApp : public CActive
{
private:
CTelephony* iTelephony;
CTelephony::TCallId iCallId;
public:
CClientApp(CTelephony* aTelephony, CTelephony::TCallId aCallId);
TInt SomeFunction();
private:
/*
These are the pure virtual methods from CActive that
MUST be implemented by all active objects
*/
void RunL();
void DoCancel();
};
CClientApp::CClientApp(CTelephony* aTelephony, CTelephony::TCallId aCallId)
: CActive(EPriorityStandard),
iTelephony(aTelephony),
iCallId(aCallId)
{
//default constructor
}
TInt CClientApp::SomeFunction()
{
// Check that the phone supports holding calls.
CTelephony::TCallCapsV1 callCapsV1;
CTelephony::TCallCapsV1Pckg callCapsV1Pckg(callCapsV1);
iTelephony->GetCallDynamicCaps(iCallId, callCapsV1Pckg);
if( callCapsV1.iControlCaps & CTelephony::KCapsHold )
{
// The call represented by 'iCallId' can be put on hold
iTelephony->Hold(iStatus, iCallId);
SetActive();
return KErrNone;
}
else
{
// The call cannot be put on hold;
// return an error indicate this.
return KErrNotSupported;
}
}
void CClientApp::RunL()
{
if(iStatus==KErrNone)
{} // The call has been held successfully;
}
void CClientApp::DoCancel()
{
iTelephony->CancelAsync(CTelephony::EHoldCancel);
}
Remember to link to the Etel3rdParty.lib
and
euser.lib
libraries.
Once a call is on hold, you can resume the call by with
CTelephony::Resume()
. It is very similar to
CTelephony::Hold()
: check that the phone supports resuming (see
Dynamic call capabilities),
then pass CTelephony::Resume()
the ID of the call to resume.
CTelephony::Resume()
is asynchronous; use
CTelephony::EResumeCancel
to cancel.
Note that you can terminate a call while it is on hold; you do not have to resume it first.
For example:
#include <e32base.h>
#include <Etel3rdParty.h>
class CClientApp : public CActive
{
private:
CTelephony* iTelephony;
CTelephony::TCallId iCallId;
public:
CClientApp(CTelephony* aTelephony, CTelephony::TCallId aCallId);
TInt SomeFunction();
private:
/*
These are the pure virtual methods from CActive that
MUST be implemented by all active objects
*/
void RunL();
void DoCancel();
};
CClientApp::CClientApp(CTelephony* aTelephony, CTelephony::TCallId aCallId)
: CActive(EPriorityStandard),
iTelephony(aTelephony),
iCallId(aCallId)
{
//default constructor
}
TInt CClientApp::SomeFunction()
{
// Check that the phone supports Resuming calls.
CTelephony::TCallCapsV1 callCapsV1;
CTelephony::TCallCapsV1Pckg callCapsV1Pckg(callCapsV1);
iTelephony->GetCallDynamicCaps(iCallId, callCapsV1Pckg);
if( callCapsV1.iControlCaps & CTelephony::KCapsResume )
{
// The call represented by 'iCallId' can be resumed
iTelephony->Resume(iStatus, iCallId);
SetActive();
return KErrNone;
}
else
{
// The call cannot be resumed;
// return an error indicate this.
return KErrNotSupported;
}
}
void CClientApp::RunL()
{
if(iStatus==KErrNone)
{} // The call has been resumed successfully;
}
void CClientApp::DoCancel()
{
iTelephony->CancelAsync(CTelephony::EResumeCancel);
}
Remember to link to the Etel3rdParty.lib
and
euser.lib
libraries.
Call CTelephony::Swap()
when you have an
active call and a call on hold. It makes the held call active and the puts the
active call on hold. Pass it the IDs of the two calls. The IDs are the
CTelephony::TCallId
s returned when you dialled or answered
the calls.
Before calling CTelephony::Swap()
, check that your
phone supports call swapping; see Dynamic call capabilities.
CTelephony::Swap()
is asynchronous; use
CTelephony::ESwapCancel
to cancel.
For example:
#include <e32base.h>
#include <Etel3rdParty.h>
class CClientApp : public CActive
{
private:
CTelephony* iTelephony;
CTelephony::TCallId iCallIdA;
CTelephony::TCallId iCallIdB;
public:
CClientApp(CTelephony* aTelephony, CTelephony::TCallId aCallIdA, CTelephony::TCallId aCallIdB);
TInt SomeFunction();
private:
/*
These are the pure virtual methods from CActive that
MUST be implemented by all active objects
*/
void RunL();
void DoCancel();
};
CClientApp::CClientApp(CTelephony* aTelephony, CTelephony::TCallId aCallId)
: CActive(EPriorityStandard),
iTelephony(aTelephony),
iCallIdA(aCallIdA)
iCallIdB(aCallIdB)
{
//default constructor
}
TInt CClientApp::SomeFunction()
{
// Check that the phone supports Resuming calls.
CTelephony::TCallCapsV1 callCapsV1;
CTelephony::TCallCapsV1Pckg callCapsV1Pckg(callCapsV1);
iTelephony->GetCallDynamicCaps(iCallId, callCapsV1Pckg);
if( callCapsV1.iControlCaps & CTelephony::KCapsSwap )
{
// The call represented by 'iCallId' can be swapped
iTelephony->Swap(iStatus, iCallIdA, iCallIdB);
SetActive();
return KErrNone;
}
else
{
// The call cannot be swapped;
// return an error indicate this.
return KErrNotSupported;
}
}
void CClientApp::RunL()
{
if(iStatus==KErrNone)
{} // The call has been swapped successfully;
}
void CClientApp::DoCancel()
{
iTelephony->CancelAsync(CTelephony::ESwapCancel);
}
Remember to link to the Etel3rdParty.lib
and
euser.lib
libraries.