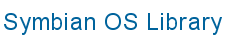
![]() |
![]() |
|
Location:
convutils.h
Link against: convutils.lib
class CnvUtilities;
Provides static character conversion utilities for complex encodings. Its functions may be called from a plug-in DLL's implementation
of ConvertFromUnicode()
and ConvertToUnicode().
These utility functions are provided for use when converting to/from complex character set encodings, including modal encodings. Modal encodings are those where the interpretation of a given byte of data is dependent on the current mode; mode changing is performed by escape sequences which occur in the byte stream. A non-modal complex encoding is one in which characters are encoded using variable numbers of bytes. The number of bytes used to encode a character depends on the value of the initial byte.
Defined in CnvUtilities
:
ConvertFromIntermediateBufferInPlace()
, ConvertFromUnicode()
, ConvertFromUnicode()
, ConvertToUnicodeFromHeterogeneousForeign()
, ConvertToUnicodeFromHeterogeneousForeign()
, ConvertToUnicodeFromModalForeign()
, ConvertToUnicodeFromModalForeign()
, FConvertFromIntermediateBufferInPlace
, FConvertToIntermediateBufferInPlace
, FNumberOfBytesAbleToConvert
, SCharacterSet
, SMethod
, SState
static IMPORT_C TInt ConvertFromUnicode(CCnvCharacterSetConverter::TEndianness aDefaultEndiannessOfForeignCharacters, const
TDesC8 &aReplacementForUnconvertibleUnicodeCharacters, TDes8 &aForeign, const TDesC16 &aUnicode, CCnvCharacterSetConverter::TArrayOfAscendingIndices
&aIndicesOfUnconvertibleCharacters, const TArray< SCharacterSet > &aArrayOfCharacterSets);
Converts Unicode text into a complex foreign character set encoding. This is an encoding which cannot be converted simply
by calling CCnvCharacterSetConverter::DoConvertFromUnicode()
. It may be modal (e.g. JIS) or non-modal (e.g. Shift-JIS).
The Unicode text specified in aUnicode is converted using the array of conversion data objects (aArrayOfCharacterSets) provided by the plug-in for the complex character set encoding, and the converted text is returned in aForeign. Any existing contents in aForeign are overwritten.
Unlike CCnvCharacterSetConverter::DoConvertFromUnicode()
, multiple character sets can be specified. aUnicode is converted using the first character conversion data object in the
array. When a character is found which cannot be converted using that data, each character set in the array is tried in turn.
If it cannot be converted using any object in the array, the index of the character is appended to aIndicesOfUnconvertibleCharacters
and the character is replaced by aReplacementForUnconvertibleUnicodeCharacters.
If it can be converted using another object in the array, that object is used to convert all subsequent characters until another unconvertible character is found.
|
|
static IMPORT_C TInt ConvertFromUnicode(CCnvCharacterSetConverter::TEndianness aDefaultEndiannessOfForeignCharacters, const
TDesC8 &aReplacementForUnconvertibleUnicodeCharacters, TDes8 &aForeign, const TDesC16 &aUnicode, CCnvCharacterSetConverter::TArrayOfAscendingIndices
&aIndicesOfUnconvertibleCharacters, const TArray< SCharacterSet > &aArrayOfCharacterSets, TUint &aOutputConversionFlags, TUint
aInputConversionFlags);
Converts Unicode text into a complex foreign character set encoding. This is an encoding which cannot be converted simply
by a call to CCnvCharacterSetConverter::DoConvertFromUnicode()
. It may be modal (e.g. JIS) or non-modal (e.g. Shift-JIS).
The Unicode text specified in aUnicode is converted using the array of conversion data objects (aArrayOfCharacterSets) provided by the plug-in for the complex character set encoding and the converted text is returned in aForeign. The function can either append to aForeign or overwrite its contents (if any).
Unlike CCnvCharacterSetConverter::DoConvertFromUnicode()
, multiple character sets can be specified. aUnicode is converted using the first character conversion data object in the
array. When a character is found which cannot be converted using that data, each character set in the array is tried in turn.
If it cannot be converted using any object in the array, the index of the character is appended to aIndicesOfUnconvertibleCharacters
and the character is replaced by aReplacementForUnconvertibleUnicodeCharacters.
If it can be converted using another object in the array, that object is used to convert all subsequent characters until another unconvertible character is found.
|
|
static IMPORT_C void ConvertFromIntermediateBufferInPlace(TInt aStartPositionInDescriptor, TDes8 &aDescriptor, TInt &aNumberOfCharactersThatDroppedOut,
const TDesC8 &aEscapeSequence, TInt aNumberOfBytesPerCharacter);
Inserts an escape sequence into the descriptor.
This function is provided to help in the implementation of ConvertFromUnicode()
for modal character set encodings. Each SCharacterSet
object in the array passed to ConvertFromUnicode()
must have its iConvertFromIntermediateBufferInPlace member assigned. To do this for a modal character set encoding, implement
a function whose signature matches that of FConvertFromIntermediateBufferInPlace and which calls this function, passing all
arguments unchanged, and specifying the character set's escape sequence and the number of bytes per character.
|
static IMPORT_C TInt ConvertToUnicodeFromModalForeign(CCnvCharacterSetConverter::TEndianness aDefaultEndiannessOfForeignCharacters,
TDes16 &aUnicode, const TDesC8 &aForeign, TInt &aState, TInt &aNumberOfUnconvertibleCharacters, TInt &aIndexOfFirstByteOfFirstUnconvertibleCharacter,
const TArray< SState > &aArrayOfStates);
Converts text from a modal foreign character set encoding into Unicode.
The non-Unicode text specified in aForeign is converted using the array of character set conversion objects (aArrayOfStates) provided by the plug-in, and the converted text is returned in aUnicode. The function can either append to aUnicode or overwrite its contents (if any), depending on the input conversion flags specified. The first element in aArrayOfStates is taken to be the default mode (i.e. the mode to assume by default if there is no preceding escape sequence).
|
|
static IMPORT_C TInt ConvertToUnicodeFromModalForeign(CCnvCharacterSetConverter::TEndianness aDefaultEndiannessOfForeignCharacters,
TDes16 &aUnicode, const TDesC8 &aForeign, TInt &aState, TInt &aNumberOfUnconvertibleCharacters, TInt &aIndexOfFirstByteOfFirstUnconvertibleCharacter,
const TArray< SState > &aArrayOfStates, TUint &aOutputConversionFlags, TUint aInputConversionFlags);
|
|
static IMPORT_C TInt ConvertToUnicodeFromHeterogeneousForeign(CCnvCharacterSetConverter::TEndianness aDefaultEndiannessOfForeignCharacters,
TDes16 &aUnicode, const TDesC8 &aForeign, TInt &aNumberOfUnconvertibleCharacters, TInt &aIndexOfFirstByteOfFirstUnconvertibleCharacter,
const TArray< SMethod > &aArrayOfMethods);
Converts text from a non-modal complex character set encoding (e.g. Shift-JIS or EUC-JP) into Unicode.The non-Unicode text specified in aForeign is converted using the array of character set conversion methods (aArrayOfMethods) provided by the plug-in, and the converted text is returned in aUnicode. Overwrites the contents, if any, of aUnicode.
|
|
static IMPORT_C TInt ConvertToUnicodeFromHeterogeneousForeign(CCnvCharacterSetConverter::TEndianness aDefaultEndiannessOfForeignCharacters,
TDes16 &aUnicode, const TDesC8 &aForeign, TInt &aNumberOfUnconvertibleCharacters, TInt &aIndexOfFirstByteOfFirstUnconvertibleCharacter,
const TArray< SMethod > &aArrayOfMethods, TUint &aOutputConversionFlags, TUint aInputConversionFlags);
|
|
struct SCharacterSet;
Stores information about a non-Unicode character set. The information is used to locate the conversion information required
by ConvertFromUnicode()
and ConvertToUnicode().
An array of these structs that contains all available character sets can be generated by CreateArrayOfCharacterSetsAvailableLC() and CreateArrayOfCharacterSetsAvailableL(), and is used by one of the overloads of PrepareToConvertToOrFromL().
Defined in CnvUtilities::SCharacterSet
:
iConversionData
, iConvertFromIntermediateBufferInPlace
, iEscapeSequence
iConversionData
const SCnvConversionData * iConversionData;
The conversion data.
iConvertFromIntermediateBufferInPlace
FConvertFromIntermediateBufferInPlace iConvertFromIntermediateBufferInPlace;
A pointer to a function which "mangles" the text in a way appropriate to the target complex character set. For instance it might insert a shifting character, escape sequence, or other special characters.
iEscapeSequence
const TDesC8 * iEscapeSequence;
The escape sequence which introduces the character set, i.e. it identifies this character set as the next one to use. Must not be NULL. If the character set is non-modal, this should be set to an empty descriptor.
struct SState;
Character conversion data for one of the character sets which is specified in a modal character set encoding. An array of
these structs is used when converting from a modal character set into Unicode, using CnvUtilities::ConvertToUnicodeFromModalForeign()
. Neither of the members may be NULL.
Defined in CnvUtilities::SState
:
iConversionData
, iEscapeSequence
iEscapeSequence
const TDesC8 * iEscapeSequence;
The escape sequence which introduces the character set, i.e. it identifies this character set as the next one to use. This must begin with KControlCharacterEscape.
iConversionData
const SCnvConversionData * iConversionData;
The conversion data.
struct SMethod;
Defined in CnvUtilities::SMethod
:
iConversionData
, iConvertToIntermediateBufferInPlace
, iNumberOfBytesAbleToConvert
, iNumberOfBytesPerCharacter
, iNumberOfCoreBytesPerCharacter
iNumberOfBytesAbleToConvert
FNumberOfBytesAbleToConvert iNumberOfBytesAbleToConvert;
A pointer to a function which calculates the number of consecutive bytes in the remainder of the foreign descriptor which
can be converted using the current character set's conversion data. It may return a negative CCnvCharacterSetConverter::TError
value to indicate an error in the encoding.
iConvertToIntermediateBufferInPlace
FConvertToIntermediateBufferInPlace iConvertToIntermediateBufferInPlace;
A pointer to a function which prepares the text for conversion into Unicode. For instance it might remove any shifting or other special characters.
iConversionData
const SCnvConversionData * iConversionData;
The conversion data.
iNumberOfBytesPerCharacter
TInt16 iNumberOfBytesPerCharacter;
The number of bytes per character.
iNumberOfCoreBytesPerCharacter
TInt16 iNumberOfCoreBytesPerCharacter;
The number of core bytes per character.
typedef void(* CnvUtilities::FConvertFromIntermediateBufferInPlace)(TInt aStartPositionInDescriptor, TDes8& aDescriptor, TInt&
aNumberOfCharactersThatDroppedOut);
A pointer to a function which "mangles" text when converting from Unicode into a complex modal or non-modal foreign character set encoding.
It might insert a shifting character, escape sequence, or other special characters.If the target character set encoding is
modal, the implementation of this function may call the CnvUtilities::ConvertFromIntermediateBufferInPlace()
utility function which is provided because many modal character sets require an identical implementation of this function.
" convutils.lib "
typedef TInt(* CnvUtilities::FNumberOfBytesAbleToConvert)(const TDesC8& aDescriptor);
A pointer to a function which calculates the number of consecutive bytes in the remainder of the foreign descriptor which can be converted using the current character set's conversion data.
Called when converting from a non-modal complex character set encoding into Unicode. It may return a negative CCnvCharacterSetConverter::TError
value to indicate an error in the encoding.
" convutils.lib "
typedef void(* CnvUtilities::FConvertToIntermediateBufferInPlace)(TDes8& aDescriptor);
A pointer to a function which prepares the text for conversion into Unicode.
For instance it might remove any shifting or other special characters. Called when converting from a non-modal complex character set encoding into Unicode.
" convutils.lib "