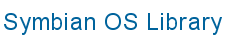
![]() |
![]() |
|
Location:
e32cmn.h
class RPointerArray : private RPointerArrayBase;
A simple and efficient array of pointers to objects.
The elements of the array are pointers to instances of a class; this class is specified as the template parameter T.
The class offers standard array behaviour which includes insertion, appending and sorting of pointers.
Derivation from RPointerArrayBase
is private.
RPointerArrayBase
- No description.
RPointerArray
- A simple and efficient array of pointers to objects
Defined in RPointerArray
:
Append()
, AppendL()
, Array()
, Close()
, Compress()
, Count()
, Find()
, Find()
, Find()
, FindInAddressOrder()
, FindInAddressOrder()
, FindInAddressOrderL()
, FindInAddressOrderL()
, FindInOrder()
, FindInOrder()
, FindInOrder()
, FindInOrderL()
, FindInOrderL()
, FindL()
, FindL()
, FindReverse()
, FindReverse()
, FindReverse()
, FindReverseL()
, FindReverseL()
, GranularCompress()
, Insert()
, InsertInAddressOrder()
, InsertInAddressOrderAllowRepeats()
, InsertInAddressOrderAllowRepeatsL()
, InsertInAddressOrderL()
, InsertInOrder()
, InsertInOrderAllowRepeats()
, InsertInOrderAllowRepeatsL()
, InsertInOrderL()
, InsertL()
, RPointerArray()
, RPointerArray()
, RPointerArray()
, RPointerArray()
, Remove()
, Reserve()
, ReserveL()
, Reset()
, ResetAndDestroy()
, Sort()
, SortIntoAddressOrder()
, SpecificFindInAddressOrder()
, SpecificFindInAddressOrder()
, SpecificFindInAddressOrderL()
, SpecificFindInAddressOrderL()
, SpecificFindInOrder()
, SpecificFindInOrder()
, SpecificFindInOrderL()
, SpecificFindInOrderL()
, operator[]()
, operator[]()
inline RPointerArray();
Default C++ constructor.
This constructs an array object for an array of pointers with default granularity, which is 8.
inline RPointerArray(TInt aGranularity);
C++ constructor with granularity.
This constructs an array object for an array of pointers with the specified granularity.
|
|
inline RPointerArray(TInt aMinGrowBy, TInt aFactor);
C++ constructor with minimum growth step and exponential growth factor.
This constructs an array object for an array of pointers with the specified minimum growth step and exponential growth factor.
|
|
inline RPointerArray(T **aEntries, TInt aCount);
C++ constructor with a pointer to the first array entry in a pre-existing array, and the number of entries in that array.
This constructor takes a pointer to a pre-existing set of entries of type pointer to class T, which is owned by another RPointerArray object. Ownership of the set of entries still resides with the original RPointerArray object.
|
|
inline void Close();
Closes the array and frees all memory allocated to it.
The function must be called before this array object goes out of scope.
Note that the function does not delete the objects whose pointers are contained in the array.
inline TInt Count() const;
Gets the number of object pointers in the array.
|
inline T *const &operator[](TInt anIndex) const;
Gets a reference to the object pointer located at the specified position within the array.
The compiler chooses this option if the returned reference is used in an expression where the reference cannot be modified.
|
|
|
inline T *&operator[](TInt anIndex);
Gets a reference to the object pointer located at the specified position within the array.
The compiler chooses this option if the returned reference is used in an expression where the reference can be modified.
|
|
|
inline TInt Append(const T *anEntry);
Appends an object pointer onto the array.
|
|
inline TInt Insert(const T *anEntry, TInt aPos);
Inserts an object pointer into the array at the specified position.
|
|
|
inline void Remove(TInt anIndex);
Removes the object pointer at the specified position from the array.
Note that the function does not delete the object whose pointer is removed.
|
|
inline void Compress();
Compresses the array down to a minimum.
After a call to this function, the memory allocated to the array is just sufficient for its contained object pointers. Subsequently adding a new object pointer to the array always results in a re-allocation of memory.
inline void Reset();
Empties the array.
It frees all memory allocated to the array and resets the internal state so that it is ready to be reused.
This array object can be allowed to go out of scope after a call to this function.
Note that the function does not delete the objects whose pointers are contained in the array.
void ResetAndDestroy();
Empties the array and deletes the referenced objects.
It frees all memory allocated to the array and resets the internal state so that it is ready to be reused. The function also deletes all of the objects whose pointers are contained by the array.
This array object can be allowed to go out of scope after a call to this function.
inline TInt Find(const T *anEntry) const;
Finds the first object pointer in the array which matches the specified object pointer, using a sequential search.
Matching is based on the comparison of pointers.
The find operation always starts at the low index end of the array. There is no assumption about the order of objects in the array.
|
|
inline TInt Find(const T *anEntry, TIdentityRelation< T > anIdentity) const;
Finds the first object pointer in the array whose object matches the specified object, using a sequential search and a matching algorithm.
The algorithm for determining whether two class T objects match is provided by a function supplied by the caller.
The find operation always starts at the low index end of the array. There is no assumption about the order of objects in the array.
|
|
inline TInt Find(const K &aKey, TBool(*apfnCompare)(const K *k, const T &t)) const;
Finds the first object pointer in the array which matches aKey using the comparison algorithm provided by apfnCompare.
The find operation always starts at the low index end of the array. There is no assumption about the order of objects in the array.
|
|
inline TInt FindReverse(const T *anEntry) const;
Finds the last object pointer in the array which matches the specified object pointer, using a sequential search.
Matching is based on the comparison of pointers.
The find operation always starts at the high index end of the array. There is no assumption about the order of objects in the array.
|
|
inline TInt FindReverse(const T *anEntry, TIdentityRelation< T > anIdentity) const;
Finds the last object pointer in the array whose object matches the specified object, using a sequential search and a matching algorithm.
The algorithm for determining whether two class T objects match is provided by a function supplied by the caller.
The find operation always starts at the high index end of the array. There is no assumption about the order of objects in the array.
|
|
inline TInt FindReverse(const K &aKey, TInt(*apfnMatch)(const K *k, const T &t)) const;
Finds the first object pointer in the array which matches aKey using the comparison algorithm provided by apfnCompare.
The find operation always starts at the high index end of the array. There is no assumption about the order of objects in the array.
|
|
inline TInt FindInAddressOrder(const T *anEntry) const;
Finds the object pointer in the array that matches the specified object pointer, using a binary search technique.
The function assumes that object pointers in the array are in address order.
|
|
inline TInt FindInOrder(const T *anEntry, TLinearOrder< T > anOrder) const;
Finds the object pointer in the array whose object matches the specified object, using a binary search technique and an ordering algorithm.
The function assumes that existing object pointers in the array are ordered so that the objects themselves are in object order as determined by an algorithm supplied by the caller and packaged as a TLinearOrder<T>.
|
|
inline TInt FindInAddressOrder(const T *anEntry, TInt &anIndex) const;
Finds the object pointer in the array that matches the specified object pointer, using a binary search technique.
The function assumes that object pointers in the array are in address order.
|
|
inline TInt FindInOrder(const T *anEntry, TInt &anIndex, TLinearOrder< T > anOrder) const;
Finds the object pointer in the array whose object matches the specified object, using a binary search technique and an ordering algorithm.
The function assumes that existing object pointers in the array are ordered so that the objects themselves are in object order as determined by an algorithm supplied by the caller and packaged as a TLinearOrder<T>.
|
|
inline TInt FindInOrder(const K &aKey, TInt(*apfnCompare)(const K *k, const T &t)) const;
Finds the object pointer in the array whose object matches the specified key, (Using the relationship defined within apfnCompare) using a binary search technique and an ordering algorithm.
The function assumes that existing object pointers in the array are ordered so that the objects themselves are in object order as determined by an algorithm supplied by the caller and packaged as a TLinearOrder<T>.
|
|
inline TInt SpecificFindInAddressOrder(const T *anEntry, TInt aMode) const;
Finds the object pointer in the array that matches the specified object pointer, using a binary search technique.
Where there is more than one matching element, it finds the first, the last or any matching element as specified by the value of aMode.
The function assumes that object pointers in the array are in address order.
|
|
inline TInt SpecificFindInOrder(const T *anEntry, TLinearOrder< T > anOrder, TInt aMode) const;
Finds the object pointer in the array whose object matches the specified object, using a binary search technique and an ordering algorithm.
Where there is more than one matching element, it finds the first, the last or any matching element as specified by the value of aMode.
The function assumes that existing object pointers in the array are ordered so that the objects themselves are in object order as determined by an algorithm supplied by the caller and packaged as a TLinearOrder<T> type.
|
|
inline TInt SpecificFindInAddressOrder(const T *anEntry, TInt &anIndex, TInt aMode) const;
Finds the object pointer in the array that matches the specified object pointer, using a binary search technique.
Where there is more than one matching element, it finds the first, the last or any matching element as specified by the value of aMode.
The function assumes that object pointers in the array are in address order.
|
|
inline TInt SpecificFindInOrder(const T *anEntry, TInt &anIndex, TLinearOrder< T > anOrder, TInt aMode) const;
Finds the object pointer in the array whose object matches the specified object, using a binary search technique and an ordering algorithm.
Where there is more than one matching element, it finds the first, the last or any matching element as specified by the value of aMode.
The function assumes that existing object pointers in the array are ordered so that the objects themselves are in object order as determined by an algorithm supplied by the caller and packaged as a TLinearOrder<T> type.
|
|
inline TInt InsertInAddressOrder(const T *anEntry);
Inserts an object pointer into the array in address order.
No duplicate entries are permitted. The array remains unchanged following an attempt to insert a duplicate entry.
The function assumes that existing object pointers within the array are in address order.
|
|
inline TInt InsertInOrder(const T *anEntry, TLinearOrder< T > anOrder);
Inserts an object pointer into the array so that the object itself is in object order.
The algorithm for determining the order of two class T objects is provided by a function supplied by the caller.
No duplicate entries are permitted. The array remains unchanged following an attempt to insert a duplicate entry.
The function assumes that the array is ordered so that the referenced objects are in object order.
|
|
inline TInt InsertInAddressOrderAllowRepeats(const T *anEntry);
Inserts an object pointer into the array in address order, allowing duplicates.
If the new object pointer is a duplicate of an existing object pointer in the array, then the new pointer is inserted after the existing one. If more than one duplicate object pointer already exists in the array, then any new duplicate pointer is inserted after the last one.
The function assumes that existing object pointers within the array are in address order.
|
|
inline TInt InsertInOrderAllowRepeats(const T *anEntry, TLinearOrder< T > anOrder);
Inserts an object pointer into the array so that the object itself is in object order, allowing duplicates
The algorithm for determining the order of two class T objects is provided by a function supplied by the caller.
If the specified object is a duplicate of an existing object, then the new pointer is inserted after the pointer to the existing object. If more than one duplicate object already exists, then the new pointer is inserted after the pointer to the last one.
|
|
inline void AppendL(const T *anEntry);
Appends an object pointer onto the array.
The function leaves with one of the system wide error codes, if the operation fails.
NOTE: This function is NOT AVAILABLE to code running on the kernel side.
|
inline void InsertL(const T *anEntry, TInt aPos);
Inserts an object pointer into the array at the specified position.
The function leaves with one of the system wide error codes, if the operation fails.
NOTE: This function is NOT AVAILABLE to code running on the kernel side.
|
|
inline TInt FindL(const T *anEntry) const;
Finds the first object pointer in the array which matches the specified object pointer, using a sequential search.
Matching is based on the comparison of pointers.
The find operation always starts at the low index end of the array. There is no assumption about the order of objects in the array.
NOTE: This function is NOT AVAILABLE to code running on the kernel side.
|
|
|
inline TInt FindL(const T *anEntry, TIdentityRelation< T > anIdentity) const;
Finds the first object pointer in the array whose object matches the specified object, using a sequential search and a matching algorithm.
The algorithm for determining whether two class T objects match is provided by a function supplied by the caller.
The find operation always starts at the low index end of the array. There is no assumption about the order of objects in the array.
NOTE: This function is NOT AVAILABLE to code running on the kernel side.
|
|
|
inline TInt FindReverseL(const T *anEntry) const;
Finds the last object pointer in the array which matches the specified object pointer, using a sequential search.
Matching is based on the comparison of pointers.
The find operation always starts at the high index end of the array. There is no assumption about the order of objects in the array.
NOTE: This function is NOT AVAILABLE to code running on the kernel side.
|
|
|
inline TInt FindReverseL(const T *anEntry, TIdentityRelation< T > anIdentity) const;
Finds the last object pointer in the array whose object matches the specified object, using a sequential search and a matching algorithm.
The algorithm for determining whether two class T objects match is provided by a function supplied by the caller.
The find operation always starts at the high index end of the array. There is no assumption about the order of objects in the array.
NOTE: This function is NOT AVAILABLE to code running on the kernel side.
|
|
|
inline TInt FindInAddressOrderL(const T *anEntry) const;
Finds the object pointer in the array that matches the specified object pointer, using a binary search technique.
The function assumes that object pointers in the array are in address order.
NOTE: This function is NOT AVAILABLE to code running on the kernel side.
|
|
|
inline TInt FindInOrderL(const T *anEntry, TLinearOrder< T > anOrder) const;
Finds the object pointer in the array whose object matches the specified object, using a binary search technique and an ordering algorithm.
The function assumes that existing object pointers in the array are ordered so that the objects themselves are in object order as determined by an algorithm supplied by the caller and packaged as a TLinearOrder<T>.
NOTE: This function is NOT AVAILABLE to code running on the kernel side.
|
|
|
inline void FindInAddressOrderL(const T *anEntry, TInt &anIndex) const;
Finds the object pointer in the array that matches the specified object pointer, using a binary search technique.
The function assumes that object pointers in the array are in address order.
NOTE: This function is NOT AVAILABLE to code running on the kernel side.
|
|
inline void FindInOrderL(const T *anEntry, TInt &anIndex, TLinearOrder< T > anOrder) const;
Finds the object pointer in the array whose object matches the specified object, using a binary search technique and an ordering algorithm.
The function assumes that existing object pointers in the array are ordered so that the objects themselves are in object order as determined by an algorithm supplied by the caller and packaged as a TLinearOrder<T>.
NOTE: This function is NOT AVAILABLE to code running on the kernel side.
|
|
inline TInt SpecificFindInAddressOrderL(const T *anEntry, TInt aMode) const;
Finds the object pointer in the array that matches the specified object pointer, using a binary search technique.
Where there is more than one matching element, it finds the first, the last or any matching element as specified by the value of aMode.
The function assumes that object pointers in the array are in address order.
NOTE: This function is NOT AVAILABLE to code running on the kernel side.
|
|
|
inline TInt SpecificFindInOrderL(const T *anEntry, TLinearOrder< T > anOrder, TInt aMode) const;
Finds the object pointer in the array whose object matches the specified object, using a binary search technique and an ordering algorithm.
In the case that there is more than one matching element finds the first, last or any match as specified by the value of aMode.
The function assumes that existing object pointers in the array are ordered so that the objects themselves are in object order as determined by an algorithm supplied by the caller and packaged as a TLinearOrder<T> type.
NOTE: This function is NOT AVAILABLE to code running on the kernel side.
|
|
|
inline void SpecificFindInAddressOrderL(const T *anEntry, TInt &anIndex, TInt aMode) const;
Finds the object pointer in the array that matches the specified object pointer, using a binary search technique.
Where there is more than one matching element, it finds the first, the last or any matching element as specified by the value of aMode.
The function assumes that object pointers in the array are in address order.
NOTE: This function is NOT AVAILABLE to code running on the kernel side.
|
|
inline void SpecificFindInOrderL(const T *anEntry, TInt &anIndex, TLinearOrder< T > anOrder, TInt aMode) const;
Finds the object pointer in the array whose object matches the specified object, using a binary search technique and an ordering algorithm.
Where there is more than one matching element, it finds the first, the last or any matching element as specified by the value of aMode.
The function assumes that existing object pointers in the array are ordered so that the objects themselves are in object order as determined by an algorithm supplied by the caller and packaged as a TLinearOrder<T>.
NOTE: This function is NOT AVAILABLE to code running on the kernel side.
|
|
inline void InsertInAddressOrderL(const T *anEntry);
Inserts an object pointer into the array in address order.
No duplicate entries are permitted. The function assumes that existing object pointers within the array are in address order.
The function leaves with one of the system wide error codes, if the operation fails.
NOTE: This function is NOT AVAILABLE to code running on the kernel side.
|
inline void InsertInOrderL(const T *anEntry, TLinearOrder< T > anOrder);
Inserts an object pointer into the array so that the object itself is in object order.
The algorithm for determining the order of two class T objects is provided by a function supplied by the caller.
No duplicate entries are permitted.
The function assumes that the array is ordered so that the referenced objects are in object order.
The function leaves with one of the system wide error codes, if the operation fails.
Note that the array remains unchanged following an attempt to insert a duplicate entry.
NOTE: This function is NOT AVAILABLE to code running on the kernel side.
|
inline void InsertInAddressOrderAllowRepeatsL(const T *anEntry);
Inserts an object pointer into the array in address order, allowing duplicates.
If the new object pointer is a duplicate of an existing object pointer in the array, then the new pointer is inserted after the existing one. If more than one duplicate object pointer already exists in the array, then any new duplicate pointer is inserted after the last one.
The function assumes that existing object pointers within the array are in address order.
The function leaves with one of the system wide error codes, if the operation fails.
NOTE: This function is NOT AVAILABLE to code running on the kernel side.
|
inline void InsertInOrderAllowRepeatsL(const T *anEntry, TLinearOrder< T > anOrder);
Inserts an object pointer into the array so that the object itself is in object order, allowing duplicates
The algorithm for determining the order of two class T objects is provided by a function supplied by the caller.
If the specified object is a duplicate of an existing object, then the new pointer is inserted after the pointer to the existing object. If more than one duplicate object already exists, then the new pointer is inserted after the pointer to the last one.
The function leaves with one of the system wide error codes, if the operation fails.
NOTE: This function is NOT AVAILABLE to code running on the kernel side.
|
inline void GranularCompress();
Compresses the array down to a granular boundary.
After a call to this function, the memory allocated to the array is sufficient for its contained object pointers. Adding new object pointers to the array does not result in a re-allocation of memory until the the total number of pointers reaches a multiple of the granularity.
inline TInt Reserve(TInt aCount);
Reserves space for the specified number of elements.
After a call to this function, the memory allocated to the array is sufficient to hold the number of object pointers specified. Adding new object pointers to the array does not result in a re-allocation of memory until the the total number of pointers exceeds the specified count.
|
|
inline void ReserveL(TInt aCount);
Reserves space for the specified number of elements.
After a call to this function, the memory allocated to the array is sufficient to hold the number of object pointers specified. Adding new object pointers to the array does not result in a re-allocation of memory until the the total number of pointers exceeds the specified count.
|
|
inline void SortIntoAddressOrder();
Sorts the object pointers within the array into address order.
inline void Sort(TLinearOrder< T > anOrder);
Sorts the object pointers within the array.
The sort order of the pointers is based on the order of the referenced objects. The referenced object order is determined by an algorithm supplied by the caller and packaged as a TLinerOrder<T>.
|