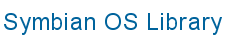
![]() |
![]() |
|
Location:
e32std.h
Link against: euser.lib
class RProcess : public RHandleBase;
A handle to a process.
The process itself is a kernel object.
RHandleBase
- A handle to an object
RProcess
- A handle to a process
Defined in RProcess
:
Create()
, Create()
, CreateWithStackOverride()
, ExitCategory()
, ExitReason()
, ExitType()
, FileName()
, GetMemoryInfo()
, HasCapability()
, HasCapability()
, Id()
, Identity()
, JustInTime()
, Kill()
, Logon()
, LogonCancel()
, Open()
, Open()
, Open()
, Panic()
, Priority()
, RProcess()
, RProcess()
, RenameMe()
, Rendezvous()
, Rendezvous()
, RendezvousCancel()
, Resume()
, SecureId()
, SetJustInTime()
, SetParameter()
, SetParameter()
, SetParameter()
, SetParameter()
, SetParameter()
, SetPriority()
, Terminate()
, Type()
, VendorId()
Inherited from RHandleBase
:
Attributes()
,
Close()
,
Duplicate()
,
FullName()
,
Handle()
,
HandleInfo()
,
Name()
,
SetHandle()
,
SetHandleNC()
,
SetReturnedHandle()
,
iHandle
inline RProcess();
Default constructor.
The constructor exists to initialise private data within this handle; it does not create the process object.
Specifically, it sets the handle-number to the value KCurrentProcessHandle. In effect, the constructor creates a default process handle.
inline RProcess(TInt aHandle);
Constructor taking a handle number.
|
IMPORT_C TInt Create(const TDesC &aFileName, const TDesC &aCommand, TOwnerType aType=EOwnerProcess);
Starts a new process, loading the specified executable.
The executable can be in ROM or RAM.
By default, ownership of this process handle is vested in the current process, but can be vested in the current thread by specifying EOwnerThread as the third parameter to this function.
|
|
IMPORT_C TInt Create(const TDesC &aFileName, const TDesC &aCommand, const TUidType &aUidType, TOwnerType aType=EOwnerProcess);
Starts a new process, loading the specified executable which matches the specified UID type.
The executable can be in ROM or RAM.
By default, ownership of this process handle is vested in the current process, but can be vested in the current thread by specifying EOwnerThread as the fourth parameter.
|
|
IMPORT_C TInt Open(const TDesC &aName, TOwnerType aType=EOwnerProcess);
Opens a handle to a specifically named process.
By default, ownership of this process handle is vested in the current process, but can be vested in the current thread by passing EOwnerThread as the second parameter to this function.
|
|
IMPORT_C TInt Open(TProcessId aId, TOwnerType aType=EOwnerProcess);
Opens a handle to the process whose process Id matches the specified process ID.
By default, ownership of this process handle is vested in the current process, but can be vested in the current thread by passing EOwnerThread as the second parameter to this function.
|
|
IMPORT_C TUidType Type() const;
Gets the Uid type associated with the process.
|
IMPORT_C TProcessId Id() const;
Gets the Id of this process.
|
static inline TInt RenameMe(const TDesC &aName);
|
|
Capability: | PowerMgmt | if the process isn't the current one. |
IMPORT_C void Kill(TInt aReason);
Ends this process, and all of its threads, specifying a reason code.
This method is intended to be used if a process is exiting under normal conditions.
If the process is system permanent, the entire system is rebooted.
|
Capability: | PowerMgmt | if the process isn't the current one. |
IMPORT_C void Terminate(TInt aReason);
Ends this process, and all of its threads, specifying a reason code.
This method is intended to be used if a process is exiting under abnormal conditions, for example if an error condition has been detected.
If the process is system critical or system permanent, the entire system is rebooted.
|
Capability: | PowerMgmt | if the process isn't the current one. |
IMPORT_C void Panic(const TDesC &aCategory, TInt aReason);
Panics the process and all of its owned threads, specifying the panic category name and reason code.
The length of the category name should be no greater than 16; any name with a length greater than 16 is truncated to 16.
If the process is system critical or system permanent, the entire system is rebooted.
|
IMPORT_C void Resume();
Makes the first thread in the process eligible for execution.
|
IMPORT_C TFileName FileName() const;
Gets a copy of the full path name of the loaded executable on which this process is based.
This is the file name which is passed to the Create()
member function of this RProcess.
|
IMPORT_C TExitType ExitType() const;
Tests whether the process has ended and, if it has ended, return how it ended.
This information allows the caller to distinguish between normal termination and a panic.
|
IMPORT_C TInt ExitReason() const;
Gets the specific reason associated with the end of this process.
The reason number together with the category name is a way of distinguishing between different causes of process termination.
If the process has panicked, this value is the panic number. If the process has ended as a result of a call to Kill()
, then the value is the one supplied by Kill()
.
If the process has not ended, then the returned value is zero.
|
IMPORT_C TExitCategoryName ExitCategory() const;
Gets the name of the category associated with the end of the process.
The category name together with the reason number is a way of distinguishing between different causes of process termination.
If the process has panicked, the category name is the panic category name; for example, E32USER-CBase or KERN-EXEC. If the
process has ended as a result of a call to Kill()
, then the category name is Kill.
If the process has not ended, then the category name is empty, i.e. the length of the category name is zero.
|
IMPORT_C TProcessPriority Priority() const;
Gets the priority of this process.
|
IMPORT_C void SetPriority(TProcessPriority aPriority) const;
Sets the priority of this process to one of the values defined by theTProcessPriority enumeration. The priority can be set to one of the four values:
EPriorityLow
EPriorityBackground
EPriorityForeground
EPriorityHigh
The absolute priority of all threads owned by the process (and all threads owned by those threads etc.) are re-calculated.
Notes:
The priority values EPriorityWindowServer, EPriorityFileServer, EPriorityRealTimeServer and EPrioritySupervisor are internal to Symbian OS and any attempt to explicitly set any of these priority values causes a KERN-EXEC 14 panic to be raised.
Any attempt to set the priority of a process which is protected and is different from the process owning the thread which invokes this function, causes a KERN-EXEC 1 panic to be raised.
A process can set its own priority whether it is protected or not.
|
IMPORT_C TBool JustInTime() const;
Tests whether "Just In Time" debugging is enabled or not for this process.
|
IMPORT_C void SetJustInTime(TBool aBoolean) const;
Enables or disables "Just In Time" debugging for this process. This will only have an effect when running on the emulator.
"Just In Time" debugging catches a thread just before it executes a panic or exception routine. Capturing a thread early, before it is terminated, allows the developer to more closely inspect what went wrong, before the kernel removes the thread. In some cases, the developer can modify context, program counter, and variables to recover the thread. This is only possible on the emulator.
By default, "Just In Time" debugging is enabled.
|
IMPORT_C void Logon(TRequestStatus &aStatus) const;
Requests notification when this process dies, normally or otherwise.
A request for notification is an asynchronous request, and completes:
when the process terminates
if the outstanding request is cancelled by a call to RProcess::LogonCancel()
.
A request for notification requires memory to be allocated; if this is unavailable, then the call to Logon()
returns, and the asynchronous request completes immediately.
|
IMPORT_C TInt LogonCancel(TRequestStatus &aStatus) const;
Cancels an outstanding request for notification of the death of this process.
A request for notification must previously have been made, otherwise the function returns KErrGeneral.
The caller passes a reference to the same request status object as was passed in the original call to Logon()
.
|
|
IMPORT_C TInt GetMemoryInfo(TModuleMemoryInfo &aInfo) const;
Gets the size and base address of the code and various data sections of the process.
The run addresses are also returned.
|
|
inline TInt Open(const TFindProcess &aFind, TOwnerType aType=EOwnerProcess);
Opens a handle to the process found by pattern matching a name.
ATFindProcess
object is used to find all processes whose full names match a specified pattern.
By default, ownership of this process handle is vested in the current process, but can be vested in the current thread by passing EOwnerThread as the second parameter to this function.
|
|
IMPORT_C void Rendezvous(TRequestStatus &aStatus) const;
Creates a Rendezvous request with the process.
The request is an asynchronous request, and completes:
when a call is made to RProcess::Rendezvous(TInt aReason)
.
if the outstanding request is cancelled by a call to RProcess::RendezvousCancel()
if the process exits
if the process panics.
Note that a request requires memory to be allocated; if this is unavailable, then this call to Rendezvous()
returns, and the asynchronous request completes immediately.
|
IMPORT_C TInt RendezvousCancel(TRequestStatus &aStatus) const;
Cancels a previously requested Rendezvous with the process.
The request completes with the value KErrCancel (if it was still outstanding).
|
|
static IMPORT_C void Rendezvous(TInt aReason);
Completes all Rendezvous' with the current process.
|
IMPORT_C TSecureId SecureId() const;
Return the Secure ID of the process.
If an intended use of this method is to check that the Secure ID is a given value, then the use of a TSecurityPolicy
object should be considered. E.g. Instead of something like:
RProcess& process;
TInt error = process.SecureId()==KRequiredSecureId ? KErrNone : KErrPermissionDenied;
this could be used;
RProcess& process;
static _LIT_SECURITY_POLICY_S0(mySidPolicy, KRequiredSecureId);
TInt error = mySidPolicy().CheckPolicy(process);
This has the benefit that the TSecurityPolicy::CheckPolicy
methods are configured by the system wide Platform Security configuration. I.e. are capable of emitting diagnostic messages
when a check fails and/or the check can be forced to always pass.
|
IMPORT_C TVendorId VendorId() const;
Return the Vendor ID of the process.
If an intended use of this method is to check that the Vendor ID is a given value, then the use of a TSecurityPolicy
object should be considered. E.g. Instead of something like:
RProcess& process;
TInt error = process.VendorId()==KRequiredVendorId ? KErrNone : KErrPermissionDenied;
this could be used;
RProcess& process;
static _LIT_SECURITY_POLICY_V0(myVidPolicy, KRequiredVendorId);
TInt error = myVidPolicy().CheckPolicy(process);
This has the benefit that the TSecurityPolicy::CheckPolicy
methods are configured by the system wide Platform Security configuration. I.e. are capable of emitting diagnostic messages
when a check fails and/or the check can be forced to always pass.
|
inline TBool HasCapability(TCapability aCapability, const char *aDiagnostic=0) const;
Check if the process has a given capability
When a check fails the action taken is determined by the system wide Platform Security configuration. If PlatSecDiagnostics is ON, then a diagnostic message is emitted. If PlatSecEnforcement is OFF, then this function will return ETrue even though the check failed.
|
|
inline TBool HasCapability(TCapability aCapability1, TCapability aCapability2, const char *aDiagnostic=0) const;
Check if the process has both of the given capabilities
When a check fails the action taken is determined by the system wide Platform Security configuration. If PlatSecDiagnostics is ON, then a diagnostic message is emitted. If PlatSecEnforcement is OFF, then this function will return ETrue even though the check failed.
|
|
IMPORT_C TInt SetParameter(TInt aIndex, RHandleBase aHandle);
Sets the specified handle into the specified environment data slot for this process.
|
|
|
IMPORT_C TInt SetParameter(TInt aSlot, const RSubSessionBase &aSession);
Sets the specfied sub-session into the specified environment data slot for this process.
|
|
|
IMPORT_C TInt SetParameter(TInt aSlot, const TDesC16 &aDes);
Sets the specified 16-bit descriptor data into the specified environment data slot for this process.
|
|
|
IMPORT_C TInt SetParameter(TInt aSlot, const TDesC8 &aDes);
Sets the specified 8-bit descriptor data into the specified environment data slot for this process.
|
|
|
IMPORT_C TInt SetParameter(TInt aSlot, TInt aData);
Sets the specfied integer value into the specified environment data slot for this process.
|
|
|
IMPORT_C TInt CreateWithStackOverride(const TDesC &aFileName, const TDesC &aCommand, const TUidType &aUidType, TInt aMinStackSize,
TOwnerType aType);
Starts a new process, loading the specified executable which matches the specified UID type and the specified minimum stack size.
The executable can be in ROM or RAM.
By default, ownership of this process handle is vested in the current process, but can be vested in the current thread by specifying EOwnerThread as the fifth parameter to this function.
|
|