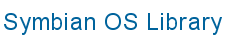
![]() |
![]() |
|
Location:
gulbordr.h
Link against: egul.lib
class TGulBorder;
Draws a border around a control.
The rectangular areas enclosed by borders are typically working areas of the graphics context so the class supplies a number of methods which describe how it occupies the area.
A distinction between descriptive and logical borders is made. For descriptive borders the appearance of the border is known and fixed. The type of border drawn is determined by flags which describe how it is to be constructed. These flags can be combined to achieve many different effects, and standard combinations have been supplied (see the TBorderType enumeration). For logical borders the use of the border is known and fixed but the appearance is not specified. The appearance and sizing functions must be supplied in custom written code.
Defined in TGulBorder
:
Adjacent()
, Draw()
, Draw()
, EAddFourPixels
, EAddFourRoundingPixels
, EAddOnePixel
, EAddOneRoundingPixel
, EAddTwoPixels
, EAddTwoRoundingPixels
, EContainerFamily
, EControlFamily
, EDeepRaised
, EDeepRaisedWithOutline
, EDeepSunken
, EDeepSunkenWithOutline
, EDottedOutline
, EFlat
, EFlatContainer
, EFlatControl
, EFocusedRaisedControl
, EFocusedSunkenControl
, EHorizontalBar
, EInvertedTwoStep
, ENone
, EOneStep
, ERaised
, ERaisedContainer
, ERaisedControl
, EShallowRaised
, EShallowSunken
, ESingleBlack
, ESingleDotted
, ESingleGray
, ESunken
, ESunkenContainer
, ESunkenControl
, EThickDeepRaisedWithOutline
, EThreeStep
, ETwoStep
, EVerticalBar
, EWindow
, EWindowFamily
, EWithInline
, EWithOutline
, HasBorder()
, InnerRect()
, Margins()
, OuterRect()
, SetAdjacent()
, SetType()
, SizeDelta()
, T3DStyle
, TBorderType
, TColors
, TConstructionStyle
, TGulBorder()
, TGulBorder()
, TGulBorder()
, TGulBorder()
, TGulBorder()
, TInlineStyle
, TLogicalFamily
, TLogicalType
, TOutlineStyle
, TRounding
, TThickness
, Type()
IMPORT_C TGulBorder();
Default constructor.
The border type is initialised to zero (i.e. no border).
IMPORT_C TGulBorder(TBorderType aType);
Constructor with a border type.
|
IMPORT_C TGulBorder(TBorderType aType, TGulAdjacent aAdjacent);
Constructor with a border type and an adjacency.
|
IMPORT_C TGulBorder(TInt aType);
Constructor with a border type, specified as a TInt.
|
IMPORT_C TGulBorder(TInt aType, TGulAdjacent aAdjacent);
Constructor with a border type, specified as a TInt, and a border adjacency.
|
IMPORT_C void Draw(CGraphicsContext &aGc, const TRect &aRect, const TColors &aBorderColors) const;
Draws the border using a graphics context, inside a containing rectangle, and using the border colours specified.
This function does nothing if the border type is ENone. If the border is a logical border, drawing is delegated to its implementation
of MGulLogicalBorder::Draw()
.
|
IMPORT_C void Draw(CGraphicsContext &aGc, const TRect &aRect) const;
Draws the border using a graphics context, inside a containing rectangle, and using default values for the border colours.
For details of the default border colours, see class TColors
.
This function does nothing if the border type is ENone. If the border is a logical border, drawing is delegated to its implementation
of MGulLogicalBorder::Draw()
.
|
IMPORT_C TRect InnerRect(const TRect &aOuterRect) const;
Returns the rectangular area enclosed by the border.
|
|
IMPORT_C TRect OuterRect(const TRect &aInnerRect) const;
Returns the rectangular area required to accommodate the inner rectangle aInnerRect and the border.
|
|
IMPORT_C void SetType(TInt aType);
Sets the border type.
Descriptive borders use one of the values from the TBorderType enum. Logical borders use one of the TLogicalType values. Custom border types can be created by selecting one value from each of the enums T3DStyle and TConstructionStyle, one or more values from each of the enums TOutlineStyle and TInlineStyle, one or more values from the enums TThickness and TRounding and ORing all these values together.
|
IMPORT_C void SetAdjacent(TInt aAdjacent);
Sets the border adjacency.
No outline is drawn for border sides that are adjacent.
|
IMPORT_C TSize SizeDelta() const;
Returns the size difference between the inner and outer rectangles of the border.
|
IMPORT_C TMargins Margins() const;
Returns the border margins.
The border margins are four integers that represent the widths in pixels of the top, bottom, left and right hand sides of the border.
If the border is a logical border, then calculating the margins is delegated to its implementation of MGulLogicalBorder::Margins()
.
Otherwise, the margins are calculated by adding together the single pixel border outline, if present (this is zero for adjacent sides), the border thickness (containing the mid-tone highlights and lowlights), and the single pixel interior outline, if present.
|
IMPORT_C TBool HasBorder() const;
Tests whether a border is present.
No border is present if its type is ENoBorder.
|
IMPORT_C TInt Adjacent() const;
Gets the border adjacency.
No outline is drawn for border sides that are adjacent.
|
IMPORT_C TInt Type() const;
Gets the border type.
|
class TColors;
Represents the colours used within the border and for the optional single pixel border outlines.
These colours are stored using TRgb
values.
An object of this type can be populated using ColorUtils::GetRgbDerivedBorderColors()
.
The colours used inside the border are derived from iBack, the border's background colour, so that border colours can be lighter or darker shades of the colour used in the main body of the control they enclose. Different combinations of light and dark shades are used to draw opposite border sides, to achieve a raised or sunken effect.
Defined in TGulBorder::TColors
:
TColors()
, iBack
, iDark
, iInternalBack
, iLight
, iLine
, iMid
, iMidlight
TColors()
IMPORT_C TColors();
Default constructor.
Initialises the border colours to blacks, whites and greys. For details, see the data members.
iLine
TRgb iLine;
The line colour.
This is the colour used to draw the outlines on either side of the border. Not all border types have an outline. By default, KRgbBlack.
iBack
TRgb iBack;
The background colour for the border.
The other colours used in the border are derived from this.
By default, KRgbWhite.
iLight
TRgb iLight;
The lightest colour.
By default, KRgbWhite.
iMidlight
TRgb iMidlight;
The mid light colour.
This colour is midway between iBack and iLight. By default, KRgbWhite.
iMid
TRgb iMid;
The mid dark colour.
This colour is midway between iBack and iDark. By default, KRgbDarkGray.
iDark
TRgb iDark;
The darkest colour.
By default, KRgbDarkGray.
iInternalBack
TRgb iInternalBack;
Not used.
TOutlineStyle
Defines the border outline style.
Note that not all border types have an outline.
|
TInlineStyle
Defines whether or not the border has a single pixel interior border.
By default it does not.
|
T3DStyle
Defines the 3D border style.
|
TConstructionStyle
Defines the border's construction style.
|
TThickness
Defines the number of pixels to add to the border thickness.
The border thickness is the central part of the border, coloured in the mid-tone highlights and lowlights.
For two step-constructed borders, the additional pixels are only added to either the top left or bottom right hand sides.
|
TRounding
Defines the number of pixels that are removed to produce rounded corners.
|
TLogicalFamily
For logical borders, defines whether the border encloses a window, a container control or a control.
|
TBorderType
Defines the descriptive border types.
|
TLogicalType
Defines the logical border types.
|