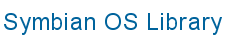
![]() |
![]() |
|
Location:
ES_SOCK.H
Link against: esock.lib
class RConnection : public RCommsSubSession;
The management interface for a network connection or subconnection.
Provides clients with the following functionality:
Opening and closing the connection
Starting a connection, which means associating it with a new underlying interface
Attaching the RConnection instance to an existing interface
Stopping the connection, which means disassociating it from the underlying interface
Obtaining progress information and notification during connection start-up
Notifying when subconnections come up and go down
Notifying when there is a service change for the connection
Notifying when a given amount of data has been sent or received on a connection or subconnection
Reading CommDB fields specific to an active connection
Collecting statistical information on the network connection and subconnections. A UI component can display the collected statistical information in order to allow the user to examine the status of connections. The information that can be gathered is the following:
All available internet access point names and internet access point 'friendly' names as appropriate for each network (GPRS/UMTS) connection
Enumerating the currently active connections and subconnections
The current status of all network connections e.g. active/suspended
The amount of data (in bytes) transferred uplink and downlink by the network connection and subconnections
The amount of time each network connection has been active (in seconds)
The current status of the connection and subconnections with respect to data transfer, i.e. active/inactive
The Quality of Service profile associated with each Packet Data Protocol (GPRS/UMTS) context, e.g. low/medium/high
Note that several of the new functions are asynchronous. It is essential for these calls that the client ensures that the parameters they pass to the RConnection API remain in scope for the duration of the asynchronous call.
RSubSessionBase
- Client-side handle to a sub-session
RCommsSubSession
- (No abstract)
RConnection
- The management interface for a network connection or subconnection
Defined in RConnection
:
AllInterfaceNotification()
, Attach()
, CancelAllInterfaceNotification()
, CancelProgressNotification()
, CancelServiceChangeNotification()
, Close()
, Control()
, EAttachTypeMonitor
, EAttachTypeNormal
, EStopAuthoritative
, EStopNormal
, EnumerateConnections()
, GetBoolSetting()
, GetConnectionInfo()
, GetDesSetting()
, GetDesSetting()
, GetIntSetting()
, GetLongDesSetting()
, GetOpt()
, LastProgressError()
, Name()
, Open()
, Open()
, Progress()
, ProgressNotification()
, RConnection()
, ServiceChangeNotification()
, SetOpt()
, Start()
, Start()
, Start()
, Start()
, Stop()
, Stop()
, TConnAttachType
, TConnStopType
, ~RConnection()
Inherited from RSubSessionBase
:
CloseSubSession()
,
CreateAutoCloseSubSession()
,
CreateSubSession()
,
Send()
,
SendReceive()
,
Session()
,
SubSessionHandle()
IMPORT_C TInt Open(RSocketServ &aSocketServer, TUint aConnectionType=KConnectionTypeDefault);
Opens a new RConnection instance.
|
|
Capability: | Dependent | on the type of connection so deferred to PRT |
IMPORT_C TInt Open(RSocketServ &aSocketServer, TName &aName);
Opens a new RConnection instance cloned from an existing RConnection instance.
|
|
IMPORT_C void Close();
Closes the connection.
The connection will not be dropped immediately: it will be dropped when there is no more data traffic on the connection. So
if a client needs to graciously shutdown the connection, Close()
, not Stop()
, needs to be used after shutting down the socket.
Capability: | Dependent | on the type of connection so deferred to PRT |
IMPORT_C void Start(TRequestStatus &aStatus);
Starts a connection asynchronously using the existing connection preferences in CommDb.
Note that this may or may not result in a dialog prompt, depending on the connection preference settings.
The request will complete once the connection is fully up (i.e. has has reached the KLinkLayerOpen stage), or if an error occurs during any stage of connection startup.
|
Capability: | Dependent | on the type of connection so deferred to PRT |
IMPORT_C void Start(TConnPref &aPref, TRequestStatus &aStatus);
Starts a connection asynchronously by overriding connection preference settings in CommDb.
The settings which can be overridden are: IAP Id, Network Id, Dialog Preference, Direction, Bearer Set.
The request will complete once the connection is fully up (i.e. has has reached the KLinkLayerOpen stage), or if an error occurs during any stage of connection startup.
|
Capability: | Dependent | on the type of connection so deferred to PRT |
IMPORT_C TInt Start();
Starts a connection synchronously using the connection preference settings in CommDb.
There is no overriding of settings (such as IAP Id, Network Id, Dialog Preference, Direction, Bearer Set).
This may or may not result in a dialog prompt, depending on the connection preference settings.
The request will complete once the connection is fully up (i.e. has has reached the KLinkLayerOpen stage), or if an error occurred during any stage of connection startup.
|
Capability: | Dependent | on the type of connection so deferred to PRT |
IMPORT_C TInt Start(TConnPref &aPref);
Starts a connection synchronously by overriding connection preference settings in CommDb.
The settings which can be overridden are: IAP Id, Network Id, Dialog Preference, Direction, Bearer Set.
The request will complete once the connection is fully up (i.e. has has reached the KLinkLayerOpen stage), or if an error occurred during any stage of connection startup.
|
|
IMPORT_C TInt Stop();
Stops the entire connection by disconnecting the underlying network interface immediately, regardless of whether other clients are using it or not.
Applications using the connection will be sent the socket error code KErrCancel. The application generally responds with clean up operations and pop-up boxes alerting the user to the termination of the application.
|
IMPORT_C TInt Stop(TConnStopType aStopType);
Stops the entire connection by disconnecting the underlying network interface immediately, regardless of whether other clients are using it or not.
If the argument is EStopNormal this is identical to calling Stop()
with no argument. If it is EStopAuthoritative then applications using the connection will be sent the socket error code KErrConnectionTerminated,
which generally results in the applications closing quietly (without pop-up boxes).
|
|
IMPORT_C void ProgressNotification(TNifProgressBuf &aProgress, TRequestStatus &aStatus, TUint aSelectedProgress=KConnProgressDefault);
Requests asynchronous progress notification for the connection.
|
IMPORT_C void CancelProgressNotification();
Cancels a request for progress notification for the connection, as issued by ProgressNotification()
.
IMPORT_C TInt Progress(TNifProgress &aProgress);
Obtains the current progress information for the connection.
|
|
IMPORT_C TInt LastProgressError(TNifProgress &aProgress);
Obtains information about the last Progress()
call which failed with an error.
|
|
IMPORT_C void ServiceChangeNotification(TUint32 &aNewISPId, TDes &aNewServiceType, TRequestStatus &aStatus);
Requests service change notification from the agent.
This call completes if the underlying service changes (i.e. ISP, GPRS APN or LAN Service).
|
IMPORT_C void CancelServiceChangeNotification();
Cancels a request for notification of change of service for the connection, as issued by ServiceChangeNotification()
.
Capability: | Dependent | on table - deferred to RDBMS |
IMPORT_C TInt GetIntSetting(const TDesC &aSettingName, TUint32 &aValue);
An attached connection: as a result of performing either a Start()
or an Attach()
Reads current CommDb settings for the active connection.
|
|
Capability: | Dependent | on table - deferred to RDBMS |
IMPORT_C TInt GetBoolSetting(const TDesC &aSettingName, TBool &aValue);
An attached connection: as a result of performing either a Start()
or an Attach()
Reads current CommDb settings for the active connection.
|
|
Capability: | Dependent | on table - deferred to RDBMS |
IMPORT_C TInt GetDesSetting(const TDesC &aSettingName, TDes8 &aValue);
An attached connection: as a result of performing either a Start()
or an Attach()
Reads current CommDb settings for the active connection.
|
|
Capability: | Dependent | on table - deferred to RDBMS |
IMPORT_C TInt GetDesSetting(const TDesC &aSettingName, TDes16 &aValue);
An attached connection: as a result of performing either a Start()
or an Attach()
Reads current CommDb settings for the active connection.
|
|
Capability: | Dependent | on table - deferred to RDBMS |
IMPORT_C TInt GetLongDesSetting(const TDesC &aSettingName, TDes &aValue);
An attached connection: as a result of performing either a Start()
or an Attach()
Reads current CommDb settings for the active connection.
|
|
IMPORT_C TInt Name(TName &aName);
Gets the unique name of an RConnection.
Used to create an RConnection which is a clone of an existing RConnection (possibly in a different process).
|
|
IMPORT_C TInt EnumerateConnections(TUint &aCount);
Enumerates the number of currently active interfaces.
Note: This does not count the number of RConnections but the number of underlying interfaces. These may be attached to by varying numbers of RConnections, RSockets etc.
|
|
IMPORT_C TInt GetConnectionInfo(TUint aIndex, TDes8 &aConnectionInfo);
Gets information about one of the currently active connections.
Note that the actual connection information is gathered on a call to EnumerateConnections()
and GetConnectionInfo()
is simply used to return the information to the client. Therefore, if the state of the connections change after the EnumerateConnections()
call, then the information returned by GetConnectionInfo()
may be out of date.
|
|
IMPORT_C void AllInterfaceNotification(TDes8 &aNotification, TRequestStatus &aStatus);
Requests asynchronous change notification for all interfaces.
This allows a client to receive a notification whenever a connection in the system goes up or down.
This allows the automatic update of the list of active network connections.
|
IMPORT_C void CancelAllInterfaceNotification();
Cancels a change notification request previously issued by a call to AllInterfaceNotification()
.
Capability: | Dependent | on the type of operation so deferred to PRT. See documentation of constant values used in aOptionName and aOptionLevel for more information |
Capability: | NetworkControl | Conditional on: KCoGetConnectionSocketInfo
|
Capability: | NetworkControl | Conditional on: KCoGetConnectionClientInfo
|
Capability: | NetworkControl | Conditional on: KConnDisableTimers
|
Capability: | NetworkControl | Conditional on: KCOLAgent
|
Capability: | NetworkControl | Conditional on: KCoEnumerateConnectionClients
|
Capability: | NetworkControl | Conditional on: KCOLInterface
|
IMPORT_C TInt Control(TUint aOptionLevel, TUint aOptionName, TDes8 &aOption);
Gets detailed information on connection clients and sockets.
More likely to be used by system control type applications.
|
|
Capability: | Dependent | on the type of operation so deferred to PRT. See documentation of constant values used in aOptionName and aOptionLevel for more information |
IMPORT_C TInt GetOpt(TUint aOptionLevel, TUint aOptionName, TInt &aOption);
Gets an option.
|
|
Capability: | Dependent | on the type of operation so deferred to PRT. See documentation of constant values used in aOptionName and aOptionLevel for more information |
IMPORT_C TInt SetOpt(TUint aOptionLevel, TUint aOptionName, TInt aOption=0);
Sets an option.
|
|
Capability: | Dependent | on the type of connection so deferred to PRT |
IMPORT_C TInt Attach(const TDesC8 &aConnectionInfo, TConnAttachType aAttachType);
Attaches the RConnection object to an existing interface.
This operation will not start an interface, as Start()
does, but attaches to an existing interface if it exists.
|
|
TConnAttachType
Identifies the intended use of the connection.
|
TConnStopType
Identifies the type of requirement for stopping the connection.
|