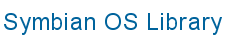
![]() |
![]() |
|
Location:
TAGMA.H
Link against: tagma.lib
class MTmCustom : public MLineBreaker;
The text customization interface. You can customize the colors, word spacing, line breaking, line height calculation, background
drawing, and text appearance, of a text object by supplying an implementation of the MTmCustom class, either directly (as
in CTmText::CustomizeL
, which takes a pointer to MTmCustom) or indirectly, making use of the fact that MTmSource
is derived from MTmCustom (as in CTmTextLayout::SetTextL
, which takes a reference to MTmSource
).
MLineBreaker
- Customizes the Unicode line-breaking algorithm
MTmCustom
- The text customization interface
Defined in MTmCustom
:
DrawBackground()
, DrawLineGraphics()
, DrawPicture()
, DrawText()
, EAiLineBreakClass
, EAlLineBreakClass
, EB2LineBreakClass
, EBaLineBreakClass
, EBbLineBreakClass
, EBkLineBreakClass
, ECbLineBreakClass
, EClLineBreakClass
, ECmLineBreakClass
, ECrLineBreakClass
, EExLineBreakClass
, EGlLineBreakClass
, EHyLineBreakClass
, EIdLineBreakClass
, EInLineBreakClass
, EIsLineBreakClass
, ELfLineBreakClass
, ELineBreakClasses
, ENsLineBreakClass
, ENuLineBreakClass
, EOpLineBreakClass
, EPoLineBreakClass
, EPrLineBreakClass
, EQuLineBreakClass
, ESaLineBreakClass
, ESgLineBreakClass
, ESpLineBreakClass
, ESyLineBreakClass
, EXxLineBreakClass
, EZwLineBreakClass
, GetLineBreakInContext()
, IsHangingCharacter()
, LineBreakClass()
, LineBreakPossible()
, Map()
, SetBrushColor()
, SetLineHeight()
, SetPenColor()
, Stretch()
, SystemColor()
, SystemColor()
, TLineHeightParam
, anonymous
Inherited from MLineBreaker
:
ExtendedInterface()
,
GetLineBreak()
,
GetLineBreakL()
virtual IMPORT_C TRgb SystemColor(TUint aColorIndex, TRgb aDefaultColor) const;
Convert a system colour index to a system colour, using or modifying the supplied default colour if desired.
|
|
virtual IMPORT_C TInt Stretch(TUint aChar) const;
Return the level of stretchability; the relative desirability of inserting space after a character when fully justifying. A value of zero means that the character cannot be stretched. Higher values mean greater stretchability.
|
|
virtual IMPORT_C TUint Map(TUint aChar) const;
The default function to map a character to the character drawn, or to 0xFFFF if the character is not to be drawn.
|
|
virtual IMPORT_C void SetLineHeight(const TLineHeightParam &aParam, TInt &aAscent, TInt &aDescent) const;
The default function to set the line height.
|
virtual IMPORT_C void DrawBackground(CGraphicsContext &aGc, const TPoint &aTextLayoutTopLeft, const TRect &aRect, const TLogicalRgb
&aBackground, TRect &aRectDrawn) const;
The default function to drawn the background. It fills the supplied rectangle with the background colour.
|
virtual IMPORT_C void DrawLineGraphics(CGraphicsContext &aGc, const TPoint &aTextLayoutTopLeft, const TRect &aRect, const
TTmLineInfo &aLineInfo) const;
By default no line graphics are drawn.
|
virtual IMPORT_C void DrawText(CGraphicsContext &aGc, const TPoint &aTopLeft, const TRect &aRect, const TTmLineInfo &aLineInfo,
const TTmCharFormat &aFormat, const TDesC &aText, const TPoint &aTextOrigin, TInt aExtraPixels) const;
Draw text and its highlit background if any. The text should be drawn with its origin at aTextOrigin after optionally drawing the background in aRect; and the text should be expanded in width by aExtraPixels, normally by using letterspacing. The default function draws the text with no special effects and supports standard and rounded highlighting only. The font, colour, and text style, which are specified in aFormat, have already been selected into the graphics context.
|
virtual IMPORT_C void DrawPicture(CGraphicsContext &aGc, const TPoint &aTextLayoutTopLeft, const TRect &aRect, MGraphicsDeviceMap
&aDevice, const CPicture &aPicture) const;
Draw the picture onto the graphics context.
|
virtual IMPORT_C TUint LineBreakClass(TUint aCode, TUint &aRangeStart, TUint &aRangeEnd) const;
Converts Unicode character into line breaking class.
|
|
virtual IMPORT_C TBool LineBreakPossible(TUint aPrevClass, TUint aNextClass, TBool aHaveSpaces) const;
Returns whether line break is possible between two characters.
|
|
virtual IMPORT_C TBool GetLineBreakInContext(const TDesC &aText, TInt aMinBreakPos, TInt aMaxBreakPos, TBool aForwards, TInt
&aBreakPos) const;
Gets the position of the first or last possible line break position in a text string. This function is called instead of LineBreakPossible()
for runs of characters of class ESaLineBreakClass. It is used for languages like Thai, Lao and Khmer that have no spaces
between words, so that line breaks must be calculated using dictionary lookup or a linguistic algorithm. Only the Thai language
is supported in this implementation, i.e. this function will return false for other languages.
|
|
virtual IMPORT_C TBool IsHangingCharacter(TUint aChar) const;
Tests whether a character may be positioned outside the right margin. This function is provided to allow customisation of the line breaking behaviour for closing punctuation in Japanese. Any characters for which this function returns ETrue are allowed to overhang the right margin. The rest will be moved to the next line.
The default implementation of this function just returns false.
|
|
IMPORT_C void SetPenColor(CGraphicsContext &aGc, TLogicalRgb aColor) const;
Sets the pen colour. The pen is used to draw lines, the outlines of filled shapes, and text.
|
IMPORT_C void SetBrushColor(CGraphicsContext &aGc, TLogicalRgb aColor) const;
Sets the brush colour. The brush is used for filling shapes and the background of text boxes. The brush has colour, style, pattern and pattern origin parameters. If no brush colour has been set, it defaults to white. However the default brush style is null, so when drawing to a window the default appears to be the window's background colour.
|
IMPORT_C TRgb SystemColor(TLogicalRgb aColor) const;
This function translates logical colours specified in FORM objects into real colours. It extracts the logical colour index
stored in TLogicalRgb
and invokes MtmCustom::SystemColor(TUint, TRgb)
to convert the logical colour to real colour.
|
|
class TLineHeightParam;
TLineHeightParam
structure is used in MTmCustom::SetLineHeight()
method to set text line height related parameters such as max character height, max ascent and descent, height and depth
of the tallest pictures (top-aligned, bottom-aligned or centered).
Defined in MTmCustom::TLineHeightParam
:
TLineHeightParam()
, iDesiredLineHeight
, iExactLineHeight
, iFontMaxAscent
, iFontMaxCharDepth
, iFontMaxCharHeight
, iFontMaxDescent
, iMaxBottomPictureHeight
, iMaxCenterPictureHeight
, iMaxCharDepth
, iMaxCharHeight
, iMaxTopPictureHeight
TLineHeightParam()
inline TLineHeightParam();
Constructs a line height parameter structure, setting all the data members to zero.
iMaxCharHeight
TInt iMaxCharHeight;
Height of the highest character in the line.
iMaxCharDepth
TInt iMaxCharDepth;
Depth of the deepest character in the line.
iMaxTopPictureHeight
TInt iMaxTopPictureHeight;
Height plus depth of the tallest top-aligned picture.
iMaxBottomPictureHeight
TInt iMaxBottomPictureHeight;
Height plus depth of the tallest bottom-aligned picture.
iMaxCenterPictureHeight
TInt iMaxCenterPictureHeight;
Height plus depth of the tallest centred picture.
iFontMaxCharHeight
TInt iFontMaxCharHeight;
Height of the tallest character of any in the fonts in the line.
iFontMaxCharDepth
TInt iFontMaxCharDepth;
Depth of the deepest character of any in the fonts in the line.
iFontMaxAscent
TInt iFontMaxAscent;
Maximum ascent of the fonts in the line.
iFontMaxDescent
TInt iFontMaxDescent;
Maximum descent of the fonts in the line.
iDesiredLineHeight
TInt iDesiredLineHeight;
Desired precise or minimum line height.
iExactLineHeight
TBool iExactLineHeight;
True if the line height must be precise.
n/a
The Unicode line breaking classes; see Unicode Technical Report 14. Not a named enumerated type, so that overriding applications can add new line breaking classes freely. The description of each constant gives the name of the line-breaking class, an example and a brief, imprecise description of the default behaviour of characters of that class.
|