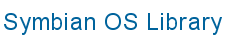
![]() |
![]() |
|
Location:
GDI.H
Link against: gdi.lib
class TZoomFactor : public MGraphicsDeviceMap;
The interface for mapping between twips and device-specific units enriched with facilities to allow zooming.
The class is recursive, because a TZoomFactor object can have a MGraphicsDeviceMap
(which could itself be a TZoomFactor) in its member data. This allows a zoom factor object to contain another zoom factor
object, and is used to allow objects with different zoom factors to be embedded in each other to an arbitrary depth by the
application architecture.
MGraphicsDeviceMap
- Interface class for mapping between twips and device-specific units (pixels)
TZoomFactor
- The interface for mapping between twips and device-specific units enriched with facilities to allow zooming
Defined in TZoomFactor
:
EZoomOneToOne
, GetNearestFontToDesignHeightInTwips()
, GetNearestFontToMaxHeightInTwips()
, GraphicsDeviceMap()
, HorizontalPixelsToTwips()
, HorizontalTwipsToPixels()
, ReleaseFont()
, SetGraphicsDeviceMap()
, SetTwipToPixelMapping()
, SetZoomFactor()
, TZoomFactor()
, TZoomFactor()
, TZoomFactor()
, VerticalPixelsToTwips()
, VerticalTwipsToPixels()
, ZoomFactor()
, anonymous
, ~TZoomFactor()
Inherited from MGraphicsDeviceMap
:
GetNearestFontInTwips()
,
PixelsToTwips()
,
TwipsToPixels()
IMPORT_C TZoomFactor();
Constructs a default zoom factor object.
Note that a TZoomFactor object cannot be used until a CGraphicsDevice
to which it is associated is specified (by SetGraphicsDeviceMap()
). Therefore the other constructor is normally used for constructing TZoomFactors. The default constructor function is provided
for use in TZoomFactor-derived classes.
IMPORT_C ~TZoomFactor();
Destructor.
Frees resources owned by the object, prior to its destruction.
inline TZoomFactor(const MGraphicsDeviceMap *aDevice);
Constructs a zoom factor object for a specific graphics device map.
The graphics map is either directly associated with a particular graphics device itself, or is associated with a hierarchy of device maps whose root map is associated with a particular graphics device.
|
IMPORT_C TInt ZoomFactor() const;
Gets the zoom factor.
|
IMPORT_C void SetZoomFactor(TInt aZoomFactor);
Sets the zoom factor.
|
inline void SetGraphicsDeviceMap(const MGraphicsDeviceMap *aDevice);
Sets the graphics device map for this zoom factor object.
|
inline const MGraphicsDeviceMap *GraphicsDeviceMap() const;
Gets the graphics device map of this zoom factor object.
|
IMPORT_C void SetTwipToPixelMapping(const TSize &aSizeInPixels, const TSize &aSizeInTwips);
Sets the twips to pixels mapping for the graphics device with which the zoom factor is associated.
This setting is used by all the twips to pixels and pixels to twips conversion functions.
|
virtual IMPORT_C TInt HorizontalTwipsToPixels(TInt aTwipWidth) const;
Converts a horizontal dimension from twips to pixels on the graphics device.
This function implements the pure virtual function defined in MGraphicsDeviceMap::HorizontalTwipsToPixels()
|
|
virtual IMPORT_C TInt VerticalTwipsToPixels(TInt aTwipHeight) const;
Converts a vertical dimension from twips to pixels on the graphics device.
This function implements the pure virtual function defined in MGraphicsDeviceMap::VerticalTwipsToPixels()
|
|
virtual IMPORT_C TInt HorizontalPixelsToTwips(TInt aPixelWidth) const;
Converts a horizontal dimension from pixels to twips on the graphics device.
This function implements the pure virtual function defined in MGraphicsDeviceMap::HorizontalPixelsToTwips()
|
|
virtual IMPORT_C TInt VerticalPixelsToTwips(TInt aPixelHeight) const;
Converts a vertical dimension from pixels to twips on the graphics device.
This function implements the pure virtual function defined in MGraphicsDeviceMap::VerticalPixelsToTwips()
|
|
virtual IMPORT_C TInt GetNearestFontToDesignHeightInTwips(CFont *&aFont, const TFontSpec &aFontSpec);
Gets the font which is the nearest to the given font specification. Matching to design height gives no guarantees on the actual physical size of the font.
|
|
virtual IMPORT_C TInt GetNearestFontToMaxHeightInTwips(CFont *&aFont, const TFontSpec &aFontSpec, TInt aMaxHeight);
Gets the font which is the nearest to the given font specification. Matching to maximum height returns a font that will fit within the height specified.
|
|
virtual IMPORT_C void ReleaseFont(CFont *aFont);
Releases the specified font.
This function implements the pure virtual function defined in MGraphicsDeviceMap::ReleaseFont()
|