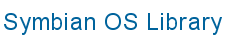
![]() |
![]() |
|
Location:
midistandardcustomcommands.h
class MMidiCustomCommandImplementor;
Mixin class to be derived from by controller plugins wishing to support the MIDI controller custom commands.
Defined in MMidiCustomCommandImplementor
:
MmcChannelVolumeL()
, MmcChannelsSupportedL()
, MmcCloseL()
, MmcCustomBankLoadedL()
, MmcDurationMicroBeatsL()
, MmcGetBalanceL()
, MmcGetBankIdL()
, MmcGetInstrumentIdL()
, MmcGetInstrumentL()
, MmcGetRepeatsL()
, MmcInstrumentNameL()
, MmcIsChannelMuteL()
, MmcIsTrackMuteL()
, MmcLoadCustomBankDataL()
, MmcLoadCustomBankL()
, MmcLoadCustomInstrumentDataL()
, MmcLoadCustomInstrumentL()
, MmcMaxChannelVolumeL()
, MmcMaxPlaybackRateL()
, MmcMaxPolyphonyL()
, MmcMaxVolumeL()
, MmcMimeTypeL()
, MmcMinPlaybackRateL()
, MmcNoteOffL()
, MmcNoteOnL()
, MmcNumTracksL()
, MmcNumberOfBanksL()
, MmcNumberOfInstrumentsL()
, MmcPercussionKeyNameL()
, MmcPitchTranspositionCentsL()
, MmcPlayNoteL()
, MmcPlayNoteL()
, MmcPlaybackRateL()
, MmcPolyphonyL()
, MmcPositionMicroBeatsL()
, MmcSendMessageL()
, MmcSendMessageL()
, MmcSendMipMessageL()
, MmcSetBalanceL()
, MmcSetBankL()
, MmcSetChannelMuteL()
, MmcSetChannelVolumeL()
, MmcSetInstrumentL()
, MmcSetMaxPolyphonyL()
, MmcSetPitchTranspositionL()
, MmcSetPlaybackRateL()
, MmcSetPositionMicroBeatsL()
, MmcSetRepeatsL()
, MmcSetStopTimeL()
, MmcSetSyncUpdateCallbackIntervalL()
, MmcSetTempoL()
, MmcSetTrackMuteL()
, MmcSetVolumeL()
, MmcSetVolumeRampL()
, MmcStopL()
, MmcStopNotesL()
, MmcStopTimeL()
, MmcTempoMicroBeatsPerMinuteL()
, MmcUnloadAllCustomBanksL()
, MmcUnloadCustomBankL()
, MmcUnloadCustomInstrumentL()
, MmcVolumeL()
virtual void MmcSetPositionMicroBeatsL(TInt64 aMicroBeats)=0;
Change the position of the currently playing MIDI resource to the given position. May be called whenever a MIDI resource is open.
|
virtual void MmcPositionMicroBeatsL(TInt64 &aMicroBeats)=0;
Gets the current metrical position of the MIDI resource being played
|
virtual void MmcPlayNoteL(TInt aChannel, TInt aNote, const TTimeIntervalMicroSeconds &aDuration, TInt aNoteOnVelocity, TInt
aNoteOffVelocity)=0;
Synchronous function to play a single note. Multiple calls to this function will be accommodated as far as the MIDI engine can manage. The same functionality could be implemented using the SendMessage function
|
virtual void MmcPlayNoteL(TInt aChannel, TInt aNote, const TTimeIntervalMicroSeconds &aStartTime, const TTimeIntervalMicroSeconds
&aDuration, TInt aNoteOnVelocity, TInt aNoteOffVelocity)=0;
Synchronous function to play a single note at a specified time. Multiple calls to this function will be accommodated as far as the MIDI engine can manage. The same functionality could be implemented using the SendMessage function
|
virtual void MmcStopNotesL(TInt aChannel)=0;
Stops the playback of all notes on the given channel, by means of an All Notes Off MIDI message
|
virtual void MmcNoteOnL(TInt aChannel, TInt aNote, TInt aVelocity)=0;
Synchronous function to commence playback of a note. Multiple calls to this function will be accommodated as far as the MIDI engine can manage
|
virtual void MmcNoteOffL(TInt aChannel, TInt aNote, TInt aVelocity)=0;
Synchronous function to terminate playback of a note. If no corresponding note is found then no error is raised
|
virtual void MmcPlaybackRateL(TInt &aPlayBackRate)=0;
Gets the current playback rate factor of the currently open MIDI resource. The playback rate is independent from tempo, i.e., it can be used to give an overall speed factor for playback.
|
virtual void MmcSetPlaybackRateL(TInt aPlayBackRate)=0;
Sets the playback rate for the playback of the current MIDI resource. The playback rate is independent from tempo, i.e., it can be used to give an overall speed factor for playback. May be called whether playback is in progress or not.
|
virtual void MmcMaxPlaybackRateL(TInt &aMaxRate)=0;
Gets the maximum playback rate in milli-percentage from the MIDI engine.
|
virtual void MmcMinPlaybackRateL(TInt &aMinRate)=0;
Gets the minimum playback rate in milli-percentage from the MIDI engine.
|
virtual void MmcTempoMicroBeatsPerMinuteL(TInt &aMicroBeatsPerMinute)=0;
Gets the current tempo of the currently open MIDI resource. The tempo is independent from the playback rate, i.e., the resulting playback speed will be affected by both.
|
virtual void MmcSetTempoL(TInt aMicroBeatsPerMinute)=0;
Sets the tempo at which the current MIDI resource should be played. May be called whether playback is in progress or not. The tempo is independent from the playback rate, i.e., the resulting playback speed will be affected by both.
|
virtual void MmcPitchTranspositionCentsL(TInt &aPitch)=0;
Gets the pitch shift in use for the currently open MIDI resource.
|
virtual void MmcSetPitchTranspositionL(TInt aCents, TInt &aCentsApplied)=0;
Sets the pitch shift to apply to the currently open MIDI resource. May be called during playback.
|
virtual void MmcDurationMicroBeatsL(TInt64 &aDuration)=0;
Gets the length of the currently open MIDI resource in micro-beats
|
virtual void MmcNumTracksL(TInt &aTracks)=0;
Gets the number of tracks present in the currently open MIDI resource.
|
virtual void MmcSetTrackMuteL(TInt aTrack, TBool aMuted)=0;
Mutes or unmutes a particular track.
|
virtual void MmcMimeTypeL(TDes8 &aMimeType)=0;
Gets the MIME type of the MIDI resource currently open.
|
virtual void MmcSetSyncUpdateCallbackIntervalL(const TTimeIntervalMicroSeconds &aMicroSeconds, TInt64 aMicroBeats=0)=0;
Sets the frequency at which MMIDIClientUtilityObserver::MmcuoSyncUpdateL() is called to allow other components to synchronise with playback of this MIDI resource.
|
virtual void MmcSendMessageL(const TDesC8 &aMidiMessage, TInt &aBytes)=0;
Sends a single MIDI message to the MIDI engine.
|
virtual void MmcSendMessageL(const TDesC8 &aMidiMessage, const TTimeIntervalMicroSeconds &aTime, TInt &aBytes)=0;
Sends a single MIDI message, with time stamp, to the MIDI engine.
|
virtual void MmcSendMipMessageL(const TArray< TMipMessageEntry > &aEntry)=0;
Sends a mip message to the MIDI engine. This is a convenience function, because the same functionality could be achieved with the SendMessage() function.
|
virtual void MmcNumberOfBanksL(TBool aCustom, TInt &aNumBanks)=0;
Gets the number of standard or custom sound banks currently available.
|
virtual void MmcGetBankIdL(TBool aCustom, TInt aBankIndex, TInt &aBankId)=0;
Gets the identifier of a sound bank. Bank identifier (aka bank number) is a 14-bit value consisting of MIDI bank MSB and LSB values.
|
virtual void MmcLoadCustomBankL(const TDesC &aFileName, TInt &aBankId)=0;
Loads one or more custom sound banks from a file into memory for use. If several banks are loaded with consequent LoadCustomBanksL() function calls, the banks are combined if the bank sets have conflicting bank numbers.
|
virtual void MmcLoadCustomBankDataL(const TDesC8 &aBankData, TInt &aBankId)=0;
Loads one or more custom sound banks from a descriptor into memory for use. If several banks are loaded with consequent LoadCustomBanksL() function calls, the banks are combined if the bank sets have conflicting bank numbers.
|
virtual void MmcUnloadCustomBankL(TInt aBankId)=0;
Removes a custom sound bank from memory. Only valid for sound banks previously loaded from file. Once unloaded the custom sound bank is no longer available for use.
|
virtual void MmcCustomBankLoadedL(TInt aBankId, TBool &aBankLoaded)=0;
Query if a bank has been loaded to the memory.
|
virtual void MmcNumberOfInstrumentsL(TInt aBankId, TBool aCustom, TInt &aNumInstruments)=0;
Gets the number of instruments available in a given sound bank.
|
virtual void MmcGetInstrumentIdL(TInt aBankId, TBool aCustom, TInt aInstrumentIndex, TInt &aInstrumentId)=0;
Gets the identifier of an instrument.
|
virtual const TDesC &MmcInstrumentNameL(TInt aBankId, TBool aCustom, TInt aInstrumentId)=0;
Gets the name of the given instrument.
|
|
virtual void MmcSetInstrumentL(TInt aChannel, TInt aBankId, TInt aInstrumentId)=0;
Sets a logical channel to use the given instrument.
|
virtual void MmcLoadCustomInstrumentL(const TDesC &aFileName, TInt aFileBankId, TInt aFileInstrumentId, TInt aMemoryBankId,
TInt aMemoryInstrumentId)=0;
Loads an individual instrument from file into custom sound bank memory for use. The bank and instrument ids given in the file can be mapped into different bank and instrument ids in memory.
|
virtual void MmcLoadCustomInstrumentDataL(const TDesC8 &aInstrumentData, TInt aBankDataId, TInt aInstrumentDataId, TInt aMemoryBankId,
TInt aMemoryInstrumentId)=0;
Loads an individual instrument from descriptor into custom sound bank memory for use. The bank and instrument ids given in the descriptor can be mapped into different bank and instrument ids in memory.
|
virtual void MmcUnloadCustomInstrumentL(TInt aCustomBankId, TInt aInstrumentId)=0;
Removes an instrument from custom sound bank memory. Only valid for instruments previously loaded from file. Once unloaded the instrument is no longer available for use.
|
virtual const TDesC &MmcPercussionKeyNameL(TInt aNote, TInt aBankId, TBool aCustom, TInt aInstrumentId)=0;
Gets the name of a particular percussion key corresponding to a given note.
|
|
virtual void MmcStopTimeL(TTimeIntervalMicroSeconds &aStopTime)=0;
Get the stop time currently set for the MIDI resource.
|
virtual void MmcSetStopTimeL(const TTimeIntervalMicroSeconds &aStopTime)=0;
Sets the stop time to use for the currently open MIDI resource
|
virtual void MmcPolyphonyL(TInt &aNumNotes)=0;
Gets the number of currently active voices.
|
virtual void MmcChannelsSupportedL(TInt &aChannels)=0;
Get the maximum number of logical channels supported by the MIDI engine.
|
virtual void MmcChannelVolumeL(TInt aChannel, TReal32 &aChannelVol)=0;
Get the current volume setting of a logical channel.
|
virtual void MmcMaxChannelVolumeL(TReal32 &aMaxVol)=0;
Gets the Maximum volume setting that may be applied to a logical channel.
|
virtual void MmcSetChannelVolumeL(TInt aChannel, TReal32 aVolume)=0;
Set the volume of a channel.
|
virtual void MmcSetChannelMuteL(TInt aChannel, TBool aMuted)=0;
Set the muting state of a channel without changing its volume setting. When unmuted the channel goes back to its previous volume setting.
|
virtual void MmcVolumeL(TInt &aVolume)=0;
Gets the overall volume of the MIDI client.
|
virtual void MmcMaxVolumeL(TInt &aMaxVolume)=0;
Maximum volume setting that may be applied overall.
|
virtual void MmcSetVolumeL(TInt aVolume)=0;
Set the overall volume of the MIDI client. This setting scales all channel volumes respectively so the actual volume that a channel is played at becomes (overall volume * channel volume / max volume).
|
virtual void MmcSetVolumeRampL(const TTimeIntervalMicroSeconds &aRampDuration)=0;
Length of time over which the volume is faded up from zero to the current settings when playback is started.
|
virtual void MmcGetBalanceL(TInt &aBalance)=0;
Get the current stereo balance value.
|
virtual void MmcSetBalanceL(TInt aBalance)=0;
Set the current stereo balance value.
|
virtual void MmcSetMaxPolyphonyL(TInt aMaxNotes)=0;
Set the max polyphony level the engine can handle.
|
virtual void MmcGetRepeatsL(TInt &aNumRepeats)=0;
Gets the number of times the current opened resources have to be repeated.
|
virtual void MmcSetRepeatsL(TInt aRepeatNumberOfTimes, const TTimeIntervalMicroSeconds &aTrailingSilence)=0;
Set the number of times to repeat the current MIDI resource. After Stop() has been called, repeat number of times and the trailing silence are reset.
|
virtual void MmcSetBankL(TBool aCustom)=0;
Tell the MIDI engine to use a custom bank or a standard bank.
|
virtual void MmcIsTrackMuteL(TInt aTrack, TBool &aTrackMute)=0;
Gets the muting status of a specific track.
|
virtual void MmcIsChannelMuteL(TInt aChannel, TBool &aChannelMute)=0;
Gets the muting status of a specific channel.
|
virtual void MmcGetInstrumentL(TInt aChannel, TInt &aInstrumentId, TInt &aBankId)=0;
Gets the instrument assigned to a specified channel.
|
virtual void MmcCloseL()=0;
Closes any currently open resources, such as files, descriptors or URLs in use. Does nothing if there is nothing currently open.
virtual void MmcStopL(const TTimeIntervalMicroSeconds &aFadeOutDuration)=0;
Stops playback of a resource but does not change the current position or release any resources. Pauses the internal timer if no resource is open.
|
virtual void MmcMaxPolyphonyL(TInt &aMaxNotes)=0;
Gets the max polyphony level the engine can handle.
|