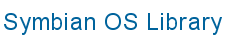
![]() |
![]() |
|
Location:
NumberConversion.h
Link against: numberconversion.lib
class NumberConversion;
Functions for converting numbers between different numbers form different scripts. The scripts supported are those given in enum TDigitType.
Defined in NumberConversion
:
AppendFormatNumber()
, ConvertDigit()
, ConvertDigits()
, ConvertFirstNumber()
, EMatchMultipleTypes
, EMatchSingleTypeOnly
, Format()
, FormatDigit()
, FormatNumber()
, LengthOfConvertedText()
, LengthOfFormattedNumber()
, PositionAndTypeOfNextNumber()
, TDigitMatchType
static IMPORT_C TInt ConvertFirstNumber(const TDesC &aText, TInt &aLength, TDigitType &aDigitType, TDigitMatchType aDigitMatchType=EMatchMultipleTypes);
Converts the descriptor aText into an integer and returns it. Ignores any minus signs: only non-negative numbers are returned.
|
|
static IMPORT_C TInt PositionAndTypeOfNextNumber(const TDesC &aText, TDigitType &aDigitType, TInt aStartFrom=0);
Finds the position and type of the next number in the descriptor. If the number has a preceeding minus sign, it will be ignored and the position of the first digit will be returned.
|
|
static IMPORT_C void FormatNumber(TDes &aText, TInt aNumber, TDigitType aDigitType);
NumberConversion::LengthOfFormattedNumber(aNumber, aDigitType) <= aText.MaxLength() && 0 <= aNumber
Converts a non-negative integer into localised text.
|
static IMPORT_C void FormatDigit(TDes &aText, TInt aNumber, TInt aLeadingZero, TDigitType aDigitType);
NumberConversion::LengthOfFormattedNumber(aNumber, aDigitType) <= aText.MaxLength() && 0 <= aNumber
Converts a non-negative integer into localised text.
|
static IMPORT_C void AppendFormatNumber(TDes &aText, TInt aNumber, TDigitType aDigitType);
NumberConversion::LengthOfFormattedNumber(aNumber, aDigitType) <= aText.MaxLength() - aText.Length() && 0 <= aNumber
Converts a non-negative integer into localised text, appending the result to a descriptor.
|
static IMPORT_C void ConvertDigits(TDes &aText, TDigitType aDigitType);
NumberConversion::LengthOfConvertedText(aText, aDigitType) <= aText.MaxLength()
Converts all of the digits in the descriptor aText into the format specified in aDigitType.
All digits in the string will either conform to one of the constants defined in enum TDigitType or will match the digit type supplied in aDigitType.
|
static IMPORT_C TInt LengthOfFormattedNumber(TInt aNumber, TDigitType aDigitType);
Returns the number of characters required to format aNumber into text.
|
|
static IMPORT_C TInt LengthOfConvertedText(const TDesC &aText, TDigitType aDigitType);
Returns the length of the descriptor required to hold text with its digits converted.
|
|
static IMPORT_C void Format(TDigitType aDigitType, TRefByValue< TDes > aFmt,...);
Formats the descriptor. Format specifiers are converted to values passed in the variable argument list. The following format specifiers are supported:
d - Interprets the argument as a TInt and formats it using the aDigitType format. Negative numbers are not supported.
S - Interprets the argument as a pointer to a TDesC and inserts it into the descriptor.
|
static IMPORT_C TChar ConvertDigit(TChar &aDigit, TDigitType aDigitType);
converts aDigit (which could be arabic, western digit etc) into the form denoted by aDigitType.
|
|