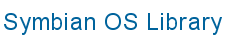
![]() |
![]() |
|
Location:
S32STOR.H
Link against: estor.lib
class RStoreReclaim;
Performs space reclamation or compaction on a permanent file store in incremental steps.
Reclaiming unused space makes it available for re-use by the store. Compacting makes unused space available for re-use by the relevant system pool — for example, the filing system in the case of file-based stores.
Once compaction is complete, the store must be committed.
Notes:
Space reclamation and compaction are only supported by the file store CPermanentFileStore
and are not supported by embedded or direct file stores.
Use active objects when implementing space reclamation or compaction asynchronously.
This class performs incremental compaction/reclamation. These operations can be performed in a possibly long running single
step using CStreamStore
functions.
Defined in RStoreReclaim
:
Available()
, Close()
, CompactL()
, CompactLC()
, Next()
, Next()
, NextL()
, NextL()
, OpenL()
, OpenLC()
, RStoreReclaim()
, Release()
, ResetL()
IMPORT_C void OpenL(CStreamStore &aStore, TInt &aCount);
Prepares the object to perform space reclamation.
|
IMPORT_C void OpenLC(CStreamStore &aStore, TInt &aCount);
Prepares the object to perform space reclamation and puts a pointer onto the cleanup stack.
Placing a cleanup item for the object onto the cleanup stack allows allocated resources to be cleaned up if a subsequent leave occurs.
|
IMPORT_C void CompactL(CStreamStore &aStore, TInt &aCount);
Prepares the object to perform compaction.
Streams must be closed before calling this function.
|
IMPORT_C void CompactLC(CStreamStore &aStore, TInt &aCount);
Prepares the object to perform compaction, putting a cleanup item onto the cleanup stack.
P lacing a cleanup item for the object onto the cleanup stack allows allocated resources to be cleaned up if a subsequent leave occurs.
Streams must be closed before calling this function.
|
inline void Close();
Stops space reclamation or compaction. All allocated resources are freed.
Notes:
If a cleanup item was placed on the cleanup stack when the RStoreReclaim object was prepared for space reclamation or compaction
(i.e. by a call to OpenLC()
or CompactLC()
), then this function need not be called explicitly; clean up is implicitly done by CleanupStack::PopAndDestroy()
.
The ResetL()
member function can be used to restart abandoned space reclamation or compaction activity.
IMPORT_C void Release();
Releases allocated resources. Any space reclamation or compaction in progress is abandoned.
Notes:
If a cleanup item was placed on the cleanup stack when the RStoreReclaim object was prepared for space reclamation or compaction
(i.e. by a call to OpenLC()
or CompactLC()
), then this function need not be called explicitly; clean up is implicitly done by CleanupStack::PopAndDestroy()
.
The ResetL()
member function can be used to restart abandoned space reclamation or compaction activity.
IMPORT_C void ResetL(TInt &aCount);
Restarts space reclamation or compaction.
The value in aCount must be:
that which was set by the most recent call to Next()
or NextL()
, if space reclamation or compaction had been started.
that which was set by OpenL()
, OpenLC()
, CompactL()
or CompactLC()
, if space reclamation or compaction had not been started.
|
IMPORT_C void NextL(TInt &aStep);
Performs the next space reclamation or compaction step synchronous, leaves. The function updates the value in aStep, and should only be called while aStep is non-zero. Once this value is zero, no further calls should be made.
The step is performed synchronously, i.e. the function does not return until the step is complete.
|
IMPORT_C void Next(TPckgBuf< TInt > &aStep, TRequestStatus &aStatus);
Initiates the next space reclamation or compaction step asynchronous, non-leaving. The function updates the value in aStep, and should only be called while aStep is non-zero. Once this value is zero, no further calls should be made.
The step itself is performed asynchronously.
Note:
The RStoreReclaim object should be made part of an active object to simplify the handling of the step completion event.
|
IMPORT_C void NextL(TPckgBuf< TInt > &aStep, TRequestStatus &aStatus);
Initiates the next space reclamation or compaction step asynchronous, leaving. The function updates the value in aStep, and should only be called while aStep is non-zero. Once this value is zero, no further calls should be made.
The step itself is performed asynchronously.
Note:
The RStoreReclaim object should be made part of an active object to simplify the handling of the step completion event.
|
IMPORT_C TInt Next(TInt &aStep);
Performs the next space reclamation or compaction step synchronous, non-leaving. The function updates the value in aStep, and should only be called while aStep is non-zero. Once this value is zero, no further calls should be made.
The step is performed synchronously, i.e. the function does not return until the step is complete.
|
|
inline TInt Available() const;
Returns the amount of free space currently available within the store. The function may be called at any time during space reclamation or compaction.
|