csBlockAllocator< T, Allocator, ObjectDispose > Class Template Reference
[Memory Management]
This class implements a memory allocator which can efficiently allocate objects that all have the same size.
More...
#include <csutil/blockallocator.h>
Inheritance diagram for csBlockAllocator< T, Allocator, ObjectDispose >:
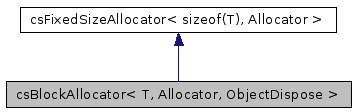
Public Types | |
typedef Allocator | AllocatorType |
typedef csBlockAllocator< T, Allocator > | ThisType |
typedef T | ValueType |
Public Member Functions | |
T * | Alloc () |
Allocate a new object. | |
csBlockAllocator (size_t nelem=32) | |
Construct a new block allocator. | |
void | Empty () |
Destroy all objects allocated by the pool. | |
void | Free (T *p) |
Deallocate an object. | |
bool | TryFree (T *p) |
Try to delete an object. | |
~csBlockAllocator () | |
Destroy all allocated objects and release memory. | |
Protected Types | |
typedef csFixedSizeAllocator< sizeof(T), Allocator > | superclass |
Detailed Description
template<class T, class Allocator = CS::Memory::AllocatorMalloc, class ObjectDispose = csBlockAllocatorDisposeDelete<T>>
class csBlockAllocator< T, Allocator, ObjectDispose >
This class implements a memory allocator which can efficiently allocate objects that all have the same size.
It has no memory overhead per allocation (unless the objects are smaller than sizeof(void*) bytes) and is extremely fast, both for Alloc() and Free(). The only restriction is that any specific allocator can be used for just one type of object (the type for which the template is instantiated).
- Remarks:
- The objects are properly constructed and destructed.
Assumes that the class
T
with which the template is instantiated has a default (zero-argument) constructor. Alloc() uses this constructor to initialize each vended object.Defining the macro CS_BLOCKALLOC_DEBUG will cause freed objects to be overwritten with '0xfb' bytes. This can be useful to track use of already freed objects, as they can be more easily recognized (as some members will be likely bogus.)
- See also:
- csArray
Definition at line 130 of file blockallocator.h.
Constructor & Destructor Documentation
csBlockAllocator< T, Allocator, ObjectDispose >::csBlockAllocator | ( | size_t | nelem = 32 |
) | [inline] |
Construct a new block allocator.
- Parameters:
-
nelem Number of elements to store in each allocation unit.
- Remarks:
- Bigger values for
nelem
will improve allocation performance, but at the cost of having some potential waste if you do not addnelem
elements to each pool. For instance, ifnelem
is 50 but you only add 3 elements to the pool, then the space for the remaining 47 elements, though allocated, will remain unused (until you add more elements).If use use csBlockAllocator as a convenient and lightweight garbage collection facility (for which it is well-suited), and expect it to dispose of allocated objects when the pool itself is destroyed, then set
warn_unfreed
to false. On the other hand, if you use csBlockAllocator only as a fast allocator but intend to manage each object's life time manually, then you may want to setwarn_unfreed
to true in order to receive diagnostics about objects which you have forgotten to release explicitly via manual invocation of Free().
Definition at line 163 of file blockallocator.h.
csBlockAllocator< T, Allocator, ObjectDispose >::~csBlockAllocator | ( | ) | [inline] |
Member Function Documentation
T* csBlockAllocator< T, Allocator, ObjectDispose >::Alloc | ( | ) | [inline] |
Allocate a new object.
The default (no-argument) constructor of T is invoked.
Reimplemented from csFixedSizeAllocator< sizeof(T), Allocator >.
Definition at line 191 of file blockallocator.h.
void csBlockAllocator< T, Allocator, ObjectDispose >::Empty | ( | ) | [inline] |
Destroy all objects allocated by the pool.
- Remarks:
- All pointers returned by Alloc() are invalidated. It is safe to perform new allocations from the pool after invoking Empty().
Reimplemented from csFixedSizeAllocator< sizeof(T), Allocator >.
Definition at line 181 of file blockallocator.h.
Referenced by csRedBlackTree< csRedBlackTreePayload< K, T > >::DeleteAll().
void csBlockAllocator< T, Allocator, ObjectDispose >::Free | ( | T * | p | ) | [inline] |
Deallocate an object.
It is safe to provide a null pointer.
- Parameters:
-
p Pointer to deallocate.
Definition at line 200 of file blockallocator.h.
Referenced by csRedBlackTree< csRedBlackTreePayload< K, T > >::DeleteNode().
bool csBlockAllocator< T, Allocator, ObjectDispose >::TryFree | ( | T * | p | ) | [inline] |
Try to delete an object.
Usage is the same as Free(), the difference being that false
is returned if the deallocation failed (the reason is most likely that the memory was not allocated by the allocator).
Definition at line 210 of file blockallocator.h.
The documentation for this class was generated from the following file:
- csutil/blockallocator.h
Generated for Crystal Space by doxygen 1.4.7