csPen Class Reference
A pen specialized for CS. More...
#include <cstool/pen.h>
Inheritance diagram for csPen:
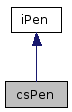
Public Member Functions | |
virtual void | ClearFlag (uint flag) |
Clears the given flag. | |
virtual void | ClearTransform () |
Clears the current transform, resets to identity. | |
virtual void | DrawArc (uint x1, uint y1, uint x2, uint y2, float start_angle=0, float end_angle=6.2831853) |
Draws an elliptical arc from start angle to end angle. | |
virtual void | DrawLine (uint x1, uint y1, uint x2, uint y2) |
Draws a single line. | |
virtual void | DrawMiteredRect (uint x1, uint y1, uint x2, uint y2, uint miter) |
Draws a mitered rectangle. | |
virtual void | DrawPoint (uint x1, uint y2) |
Draws a single point. | |
virtual void | DrawRect (uint x1, uint y1, uint x2, uint y2) |
Draws a rectangle. | |
virtual void | DrawRoundedRect (uint x1, uint y1, uint x2, uint y2, uint roundness) |
Draws a rounded rectangle. | |
void | DrawThickLine (uint x1, uint y1, uint x2, uint y2) |
Draws a thick line. | |
virtual void | DrawTriangle (uint x1, uint y1, uint x2, uint y2, uint x3, uint y3) |
Draws a triangle around the given vertices. | |
virtual void | PopTransform () |
Pops the transform stack. | |
virtual void | PushTransform () |
Pushes the current transform onto the stack. | |
virtual void | Rotate (const float &a) |
Rotates by the given matrix. | |
virtual void | SetColor (const csColor4 &color) |
Sets the current color. | |
virtual void | SetColor (float r, float g, float b, float a) |
Sets the current color. | |
virtual void | SetFlag (uint flag) |
Sets the given flag. | |
virtual void | SetMixMode (uint mode) |
Sets the given mix (blending) mode. | |
virtual void | SetOrigin (const csVector3 &o) |
Sets the origin of the coordinate system. | |
virtual void | SetPenWidth (float width) |
Sets the current pen width. | |
virtual void | SetTexture (csRef< iTextureHandle > tex) |
Sets the texture handle. | |
virtual void | SwapColors () |
Swaps the current color and the alternate color. | |
virtual void | Translate (const csVector3 &t) |
Translates by the given vector. | |
virtual void | Write (iFont *font, uint x1, uint y1, char *text) |
Writes text in the given font at the given location. | |
virtual void | WriteBoxed (iFont *font, uint x1, uint y1, uint x2, uint y2, uint h_align, uint v_align, char *text) |
Writes text in the given font, in the given box. | |
Protected Member Functions | |
void | AddTexCoord (float x, float y) |
Adds a texture coordinate. | |
void | AddThickPoints (float x1, float y1, float x2, float y2) |
Worker, adds thick line points. | |
void | AddVertex (float x, float y, bool force_add=false) |
Adds a vertex. | |
void | DrawMesh (csRenderMeshType mesh_type) |
Worker, draws the mesh. | |
void | SetAutoTexture (float w, float h) |
Worker, sets up the pen to do auto texturing. | |
void | SetupMesh () |
Worker, sets up the mesh with the vertices, color, and other information. | |
void | Start () |
Initializes our working objects. |
Detailed Description
A pen specialized for CS.
Definition at line 205 of file pen.h.
Member Function Documentation
void csPen::AddTexCoord | ( | float | x, | |
float | y | |||
) | [inline, protected] |
Adds a texture coordinate.
- Parameters:
-
x The texture's x coord. y The texture's y coord.
void csPen::AddThickPoints | ( | float | x1, | |
float | y1, | |||
float | x2, | |||
float | y2 | |||
) | [protected] |
Worker, adds thick line points.
A thick point is created when the pen width is greater than 1. It uses polygons to simulate thick lines.
void csPen::AddVertex | ( | float | x, | |
float | y, | |||
bool | force_add = false | |||
) | [protected] |
Adds a vertex.
- Parameters:
-
x X coord y Y coord force_add Forces the coordinate to be added as a vertex, instead of trying to be smart and make it a thick vertex.
virtual void csPen::ClearFlag | ( | uint | flag | ) | [virtual] |
virtual void csPen::ClearTransform | ( | ) | [virtual] |
virtual void csPen::DrawArc | ( | uint | x1, | |
uint | y1, | |||
uint | x2, | |||
uint | y2, | |||
float | start_angle = 0 , |
|||
float | end_angle = 6.2831853 | |||
) | [virtual] |
Draws an elliptical arc from start angle to end angle.
Angle must be specified in radians. The arc will be made to fit in the given box. If you want a circular arc, make sure the box is a square. If you want a full circle or ellipse, specify 0 as the start angle and 2*PI as the end angle.
Implements iPen.
void csPen::DrawMesh | ( | csRenderMeshType | mesh_type | ) | [protected] |
Worker, draws the mesh.
Draws a mitered rectangle.
The miter value should be between 0.0 and 1.0, and determines how much of the corner is mitered off and beveled.
Implements iPen.
virtual void csPen::DrawRoundedRect | ( | uint | x1, | |
uint | y1, | |||
uint | x2, | |||
uint | y2, | |||
uint | roundness | |||
) | [virtual] |
Draws a rounded rectangle.
The roundness value should be between 0.0 and 1.0, and determines how much of the corner is rounded off.
Implements iPen.
virtual void csPen::PopTransform | ( | ) | [virtual] |
virtual void csPen::PushTransform | ( | ) | [virtual] |
virtual void csPen::Rotate | ( | const float & | a | ) | [virtual] |
void csPen::SetAutoTexture | ( | float | w, | |
float | h | |||
) | [protected] |
Worker, sets up the pen to do auto texturing.
virtual void csPen::SetColor | ( | const csColor4 & | color | ) | [virtual] |
virtual void csPen::SetColor | ( | float | r, | |
float | g, | |||
float | b, | |||
float | a | |||
) | [virtual] |
virtual void csPen::SetFlag | ( | uint | flag | ) | [virtual] |
virtual void csPen::SetMixMode | ( | uint | mode | ) | [virtual] |
virtual void csPen::SetOrigin | ( | const csVector3 & | o | ) | [virtual] |
virtual void csPen::SetPenWidth | ( | float | width | ) | [virtual] |
virtual void csPen::SetTexture | ( | csRef< iTextureHandle > | tex | ) | [virtual] |
void csPen::SetupMesh | ( | ) | [protected] |
Worker, sets up the mesh with the vertices, color, and other information.
void csPen::Start | ( | ) | [protected] |
Initializes our working objects.
virtual void csPen::SwapColors | ( | ) | [virtual] |
virtual void csPen::Translate | ( | const csVector3 & | t | ) | [virtual] |
virtual void csPen::WriteBoxed | ( | iFont * | font, | |
uint | x1, | |||
uint | y1, | |||
uint | x2, | |||
uint | y2, | |||
uint | h_align, | |||
uint | v_align, | |||
char * | text | |||
) | [virtual] |
Writes text in the given font, in the given box.
The alignment specified in h_align and v_align determine how it should be aligned.
Implements iPen.
The documentation for this class was generated from the following file:
- cstool/pen.h
Generated for Crystal Space by doxygen 1.4.7