iTextureHandle Struct Reference
[3D]
A texture handle as returned by iTextureManager.
More...
#include <ivideo/texture.h>
Inheritance diagram for iTextureHandle:
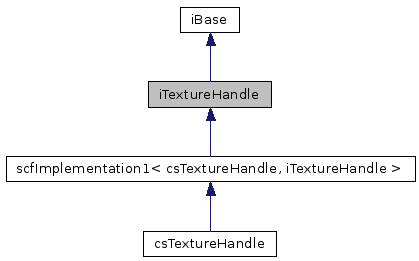
Public Types | |
enum | |
enum | |
Texture Depth Indices are used for Cubemap interface. More... | |
BGRA8888 | |
BGRA, 8 bits per pixel. | |
RGBA8888 = 0 | |
RGBA, 8 bits per pixel. | |
enum | TextureBlitDataFormat { RGBA8888 = 0, BGRA8888 } |
Format of the pixel data that is passed to iTextureHandle->Blit(). More... | |
Public Member Functions | |
virtual void | Blit (int x, int y, int width, int height, unsigned char const *data, TextureBlitDataFormat format=RGBA8888)=0 |
Blit a memory block to this texture. | |
virtual bool | GetAlphaMap ()=0 |
Query if the texture has an alpha channel. | |
virtual csAlphaMode::AlphaType | GetAlphaType () const =0 |
Get the type of alpha associated with the texture. | |
virtual int | GetFlags () const =0 |
Retrieve the flags set for this texture. | |
virtual const char * | GetImageName () const =0 |
Get the original image name. | |
virtual void | GetKeyColor (uint8 &red, uint8 &green, uint8 &blue) const =0 |
Get the key color. | |
virtual bool | GetKeyColor () const =0 |
Get the key color status (false if disabled, true if enabled). | |
virtual void | GetOriginalDimensions (int &mw, int &mh, int &md)=0 |
Return the original dimensions of the image used to create this texture. | |
virtual void | GetOriginalDimensions (int &mw, int &mh)=0 |
Return the original dimensions of the image used to create this texture. | |
virtual void * | GetPrivateObject ()=0 |
Query the private object associated with this handle. | |
virtual bool | GetRendererDimensions (int &mw, int &mh, int &md)=0 |
Get the dimensions the renderer uses for this texture. | |
virtual bool | GetRendererDimensions (int &mw, int &mh)=0 |
Get the dimensions the renderer uses for this texture. | |
virtual const char * | GetTextureClass ()=0 |
Get the "class" of a texture. | |
virtual int | GetTextureTarget () const =0 |
Get the texture target. | |
virtual void | Precache ()=0 |
Precache this texture. | |
virtual void | SetAlphaType (csAlphaMode::AlphaType alphaType)=0 |
Set the type of alpha associated with the texture. | |
virtual void | SetKeyColor (uint8 red, uint8 green, uint8 blue)=0 |
Set the key color. | |
virtual void | SetKeyColor (bool Enable)=0 |
Enable key color. | |
virtual void | SetTextureClass (const char *className)=0 |
Set the "class" of this texture. |
Detailed Description
A texture handle as returned by iTextureManager.Main creators of instances implementing this interface:
Main ways to get pointers to this interface:
Main users of this interface:
- 3D renderer implementations (iGraphics3D).
Definition at line 51 of file texture.h.
Member Enumeration Documentation
anonymous enum |
Member Function Documentation
virtual void iTextureHandle::Blit | ( | int | x, | |
int | y, | |||
int | width, | |||
int | height, | |||
unsigned char const * | data, | |||
TextureBlitDataFormat | format = RGBA8888 | |||
) | [pure virtual] |
Blit a memory block to this texture.
Format of the image is determined by the format parameter. Row by row.
- Remarks:
- If the specified target rectangle exceeds the texture dimensions the Blit() call may have no effect. It's the responsibility of the caller to ensure that the rectangle lies completely inside the texture.
virtual bool iTextureHandle::GetAlphaMap | ( | ) | [pure virtual] |
Query if the texture has an alpha channel.
This depends both on whenever the original image had an alpha channel and of the fact whenever the renderer supports alpha maps at all.
Implemented in csTextureHandle.
virtual csAlphaMode::AlphaType iTextureHandle::GetAlphaType | ( | ) | const [pure virtual] |
virtual int iTextureHandle::GetFlags | ( | ) | const [pure virtual] |
virtual const char* iTextureHandle::GetImageName | ( | ) | const [pure virtual] |
Get the original image name.
virtual bool iTextureHandle::GetKeyColor | ( | ) | const [pure virtual] |
virtual void iTextureHandle::GetOriginalDimensions | ( | int & | mw, | |
int & | mh, | |||
int & | md | |||
) | [pure virtual] |
Return the original dimensions of the image used to create this texture.
This is most often equal to GetMipMapDimensions (0, mw, mh, md) but in some cases the texture will have been resized in order to accomodate hardware restrictions (like power of two and maximum texture size). This function returns the uncorrected coordinates.
virtual void iTextureHandle::GetOriginalDimensions | ( | int & | mw, | |
int & | mh | |||
) | [pure virtual] |
Return the original dimensions of the image used to create this texture.
This is most often equal to GetMipMapDimensions (0, mw, mh) but in some cases the texture will have been resized in order to accomodate hardware restrictions (like power of two and maximum texture size). This function returns the uncorrected coordinates.
virtual void* iTextureHandle::GetPrivateObject | ( | ) | [pure virtual] |
Query the private object associated with this handle.
For internal usage by the 3D driver.
Implemented in csTextureHandle.
virtual bool iTextureHandle::GetRendererDimensions | ( | int & | mw, | |
int & | mh, | |||
int & | md | |||
) | [pure virtual] |
Get the dimensions the renderer uses for this texture.
In most cases this corresponds to the size that was used to create this texture, but some renderers have texture size limitations (like power of two) and in that case the size returned here will be the corrected size. You can get the original image size with GetOriginalDimensions().
- Returns:
- Whether the renderer-used dimensions could be determined.
virtual bool iTextureHandle::GetRendererDimensions | ( | int & | mw, | |
int & | mh | |||
) | [pure virtual] |
Get the dimensions the renderer uses for this texture.
In most cases this corresponds to the size that was used to create this texture, but some renderers have texture size limitations (like power of two) and in that case the size returned here will be the corrected size. You can get the original image size with GetOriginalDimensions().
- Returns:
- Whether the renderer-used dimensions could be determined.
virtual const char* iTextureHandle::GetTextureClass | ( | ) | [pure virtual] |
virtual int iTextureHandle::GetTextureTarget | ( | ) | const [pure virtual] |
Get the texture target.
Note the texture target is determined by the image from which the texture was created and possibly the texture flags.
virtual void iTextureHandle::Precache | ( | ) | [pure virtual] |
Precache this texture.
This might free up temporary memory and makes later usage of the texture faster.
virtual void iTextureHandle::SetAlphaType | ( | csAlphaMode::AlphaType | alphaType | ) | [pure virtual] |
Set the type of alpha associated with the texture.
Usually, the alpha mode is auto-detected (alphaSmooth on images with alpha channels, alphaBinary on keycolored images, alphaNone otherwise), but can be overridden with this method.
Implemented in csTextureHandle.
virtual void iTextureHandle::SetKeyColor | ( | bool | Enable | ) | [pure virtual] |
virtual void iTextureHandle::SetTextureClass | ( | const char * | className | ) | [pure virtual] |
Set the "class" of this texture.
A texture class is used to set some characteristics on how a texture is handled at runtime. For example, graphics hardware usually offers texture compression, but it can cause a loss of quality and precision and thus may not be desireable for all data. In this case, a class can be set on the texture that instructs the renderer to not apply texture compression.
- Remarks:
- Not all renderers may support texture classes.
- See also:
- GetTextureClass
Implemented in csTextureHandle.
The documentation for this struct was generated from the following file:
- ivideo/texture.h
Generated for Crystal Space by doxygen 1.4.7