csPoly3D Class Reference
[Geometry utilities]
The following class represents a general 3D polygon.
More...
#include <csgeom/poly3d.h>
Inheritance diagram for csPoly3D:
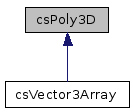
Public Member Functions | |
size_t | AddVertex (float x, float y, float z) |
Add a vertex (3D) to the polygon. | |
size_t | AddVertex (const csVector3 &v) |
Add a vertex (3D) to the polygon. | |
int | Classify (const csPlane3 &pl) const |
Classify this polygon with regards to a plane. | |
int | ClassifyAxis (int axis, float where) const |
Same as Classify() but for a given axis plane. | |
int | ClassifyX (float x) const |
Same as Classify() but for X plane only. | |
int | ClassifyY (float y) const |
Same as Classify() but for Y plane only. | |
int | ClassifyZ (float z) const |
Same as Classify() but for Z plane only. | |
int | ComputeMainNormalAxis () const |
Calculate the main axis of the normal. | |
csVector3 | ComputeNormal () const |
Compute the normal of this polygon. | |
csPlane3 | ComputePlane () const |
Compute the plane of this polygon. | |
csPoly3D (const csPoly3D ©) | |
Copy constructor. | |
csPoly3D (size_t start_size=10) | |
Make a new empty polygon. | |
void | CutToPlane (const csPlane3 &split_plane) |
Cut this polygon with a plane and only keep the front side. | |
float | GetArea () const |
Calculate the area of this polygon. | |
csVector3 | GetCenter () const |
Compute and get the central vertex of this polygon. | |
const csVector3 * | GetFirst () const |
Get the first vertex. | |
const csVector3 * | GetLast () const |
Get the last vertex. | |
const csVector3 * | GetVertex (size_t i) const |
Get the specified vertex. | |
size_t | GetVertexCount () const |
Get the number of vertices. | |
csVector3 * | GetVertices () |
Get the array with all vertices. | |
const csVector3 * | GetVertices () const |
Get the array with all vertices. | |
bool | In (const csVector3 &v) const |
Test if this vector is inside the polygon. | |
int | IsAxisAligned (float &where, float epsilon=SMALL_EPSILON) const |
Test if this polygon is axis aligned and return the axis (one of CS_AXIS_ constants). | |
void | MakeEmpty () |
Initialize the polygon to empty. | |
void | MakeRoom (size_t new_max) |
Make room for at least the specified number of vertices. | |
const csVector3 & | operator[] (size_t i) const |
Get the specified vertex. | |
csVector3 & | operator[] (size_t i) |
Get the specified vertex. | |
bool | ProjectAxisPlane (const csVector3 &point, int plane_nr, float plane_pos, csPoly2D *poly2d) const |
Project this polygon onto an axis-aligned plane as seen from some point in space. | |
bool | ProjectXPlane (const csVector3 &point, float plane_x, csPoly2D *poly2d) const |
Project this polygon onto a X plane as seen from some point in space. | |
bool | ProjectYPlane (const csVector3 &point, float plane_y, csPoly2D *poly2d) const |
Project this polygon onto a Y plane as seen from some point in space. | |
bool | ProjectZPlane (const csVector3 &point, float plane_z, csPoly2D *poly2d) const |
Project this polygon onto a Z plane as seen from some point in space. | |
void | SetVertexCount (size_t n) |
Set the number of vertices. | |
void | SetVertices (csVector3 const *v, size_t num) |
Set all polygon vertices at once. | |
void | SplitWithPlane (csPoly3D &front, csPoly3D &back, const csPlane3 &split_plane) const |
Split this polygon with the given plane (A,B,C,D). | |
void | SplitWithPlaneX (csPoly3D &front, csPoly3D &back, float x) const |
Split this polygon to the x-plane. | |
void | SplitWithPlaneY (csPoly3D &front, csPoly3D &back, float y) const |
Split this polygon to the y-plane. | |
void | SplitWithPlaneZ (csPoly3D &front, csPoly3D &back, float z) const |
Split this polygon to the z-plane. | |
virtual | ~csPoly3D () |
Destructor. | |
Static Public Member Functions | |
static int | Classify (const csPlane3 &pl, const csVector3 *vertices, size_t num_vertices) |
Static function to classify a polygon with regards to a plane. | |
static csVector3 | ComputeNormal (int *poly, size_t num, csVector3 *vertices) |
Compute the normal of an indexed polygon. | |
static csVector3 | ComputeNormal (const csArray< csVector3 > &poly) |
Compute the normal of a polygon. | |
static csVector3 | ComputeNormal (const csVector3 *vertices, size_t num) |
Compute the normal of a polygon. | |
static csPlane3 | ComputePlane (int *poly, size_t num, csVector3 *vertices) |
Compute the plane of an indexed polygon. | |
static csPlane3 | ComputePlane (const csArray< csVector3 > &poly) |
Compute the plane of a polygon. | |
static csPlane3 | ComputePlane (const csVector3 *vertices, size_t num) |
Compute the plane of a polygon. | |
static bool | In (csVector3 *poly, size_t num_poly, const csVector3 &v) |
Test if a vector is inside the given polygon. | |
Protected Attributes | |
csDirtyAccessArray< csVector3 > | vertices |
The 3D vertices. |
Detailed Description
The following class represents a general 3D polygon.
Definition at line 53 of file poly3d.h.
Constructor & Destructor Documentation
csPoly3D::csPoly3D | ( | size_t | start_size = 10 |
) |
Make a new empty polygon.
csPoly3D::csPoly3D | ( | const csPoly3D & | copy | ) |
Copy constructor.
virtual csPoly3D::~csPoly3D | ( | ) | [virtual] |
Destructor.
Member Function Documentation
size_t csPoly3D::AddVertex | ( | float | x, | |
float | y, | |||
float | z | |||
) |
Add a vertex (3D) to the polygon.
Return index of added vertex.
size_t csPoly3D::AddVertex | ( | const csVector3 & | v | ) | [inline] |
Add a vertex (3D) to the polygon.
Return index of added vertex.
Definition at line 163 of file poly3d.h.
References csVector3::x, csVector3::y, and csVector3::z.
int csPoly3D::Classify | ( | const csPlane3 & | pl | ) | const [inline] |
Classify this polygon with regards to a plane.
If this poly is on same plane it returns CS_POL_SAME_PLANE. If this poly is completely in front of the given plane it returnes CS_POL_FRONT. If this poly is completely back of the given plane it returnes CS_POL_BACK. Otherwise it returns CS_POL_SPLIT_NEEDED.
static int csPoly3D::Classify | ( | const csPlane3 & | pl, | |
const csVector3 * | vertices, | |||
size_t | num_vertices | |||
) | [static] |
Static function to classify a polygon with regards to a plane.
If this poly is on same plane it returns CS_POL_SAME_PLANE. If this poly is completely in front of the given plane it returnes CS_POL_FRONT. If this poly is completely back of the given plane it returnes CS_POL_BACK. Otherwise it returns CS_POL_SPLIT_NEEDED.
int csPoly3D::ClassifyAxis | ( | int | axis, | |
float | where | |||
) | const [inline] |
int csPoly3D::ClassifyX | ( | float | x | ) | const |
Same as Classify() but for X plane only.
int csPoly3D::ClassifyY | ( | float | y | ) | const |
Same as Classify() but for Y plane only.
int csPoly3D::ClassifyZ | ( | float | z | ) | const |
Same as Classify() but for Z plane only.
int csPoly3D::ComputeMainNormalAxis | ( | ) | const |
Calculate the main axis of the normal.
Returns one of the CS_AXIS_ constants;
csVector3 csPoly3D::ComputeNormal | ( | ) | const [inline] |
Compute the normal of an indexed polygon.
Compute the normal of a polygon.
Compute the normal of a polygon.
csPlane3 csPoly3D::ComputePlane | ( | ) | const [inline] |
Compute the plane of an indexed polygon.
Compute the plane of a polygon.
Compute the plane of a polygon.
void csPoly3D::CutToPlane | ( | const csPlane3 & | split_plane | ) |
Cut this polygon with a plane and only keep the front side.
float csPoly3D::GetArea | ( | ) | const |
Calculate the area of this polygon.
csVector3 csPoly3D::GetCenter | ( | ) | const |
Compute and get the central vertex of this polygon.
const csVector3* csPoly3D::GetFirst | ( | ) | const [inline] |
const csVector3* csPoly3D::GetLast | ( | ) | const [inline] |
const csVector3* csPoly3D::GetVertex | ( | size_t | i | ) | const [inline] |
size_t csPoly3D::GetVertexCount | ( | ) | const [inline] |
csVector3* csPoly3D::GetVertices | ( | ) | [inline] |
const csVector3* csPoly3D::GetVertices | ( | ) | const [inline] |
Test if a vector is inside the given polygon.
bool csPoly3D::In | ( | const csVector3 & | v | ) | const |
Test if this vector is inside the polygon.
int csPoly3D::IsAxisAligned | ( | float & | where, | |
float | epsilon = SMALL_EPSILON | |||
) | const |
Test if this polygon is axis aligned and return the axis (one of CS_AXIS_ constants).
The location of the axis is returned in 'where'. Returns CS_AXIS_NONE if the polygon is not axis aligned. The epsilon will be used to test if two coordinates are close.
void csPoly3D::MakeEmpty | ( | ) |
Initialize the polygon to empty.
void csPoly3D::MakeRoom | ( | size_t | new_max | ) |
Make room for at least the specified number of vertices.
const csVector3& csPoly3D::operator[] | ( | size_t | i | ) | const [inline] |
csVector3& csPoly3D::operator[] | ( | size_t | i | ) | [inline] |
bool csPoly3D::ProjectAxisPlane | ( | const csVector3 & | point, | |
int | plane_nr, | |||
float | plane_pos, | |||
csPoly2D * | poly2d | |||
) | const [inline] |
Project this polygon onto an axis-aligned plane as seen from some point in space.
Fills the given 2D polygon with the projection on the plane. This function assumes that there actually is a projection. Plane_nr is 0 for the X plane, 1 for Y, and 2 for Z. Or one of the CS_AXIX_ constants.
Project this polygon onto a X plane as seen from some point in space.
Fills the given 2D polygon with the projection on the plane. This function assumes that there actually is a projection. If the polygon to project comes on the same plane as 'point' then it will return false (no valid projection).
Project this polygon onto a Y plane as seen from some point in space.
Fills the given 2D polygon with the projection on the plane. This function assumes that there actually is a projection. If the polygon to project comes on the same plane as 'point' then it will return false (no valid projection).
Project this polygon onto a Z plane as seen from some point in space.
Fills the given 2D polygon with the projection on the plane. This function assumes that there actually is a projection. If the polygon to project comes on the same plane as 'point' then it will return false (no valid projection).
void csPoly3D::SetVertexCount | ( | size_t | n | ) | [inline] |
void csPoly3D::SetVertices | ( | csVector3 const * | v, | |
size_t | num | |||
) | [inline] |
void csPoly3D::SplitWithPlane | ( | csPoly3D & | front, | |
csPoly3D & | back, | |||
const csPlane3 & | split_plane | |||
) | const |
Split this polygon with the given plane (A,B,C,D).
Split this polygon to the x-plane.
Split this polygon to the y-plane.
Split this polygon to the z-plane.
Member Data Documentation
csDirtyAccessArray<csVector3> csPoly3D::vertices [protected] |
The documentation for this class was generated from the following file:
- csgeom/poly3d.h
Generated for Crystal Space by doxygen 1.4.7