csPolygonMesh Class Reference
[Geometry utilities]
A convenience polygon mesh implementation that you can feed with vertices and polygons from another source.
More...
#include <csgeom/polymesh.h>
Inheritance diagram for csPolygonMesh:
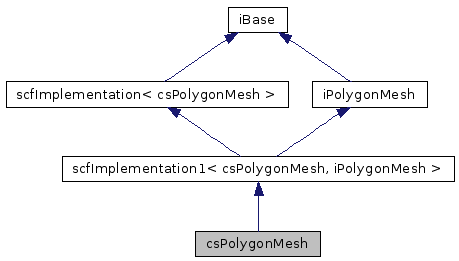
Public Member Functions | |
csPolygonMesh () | |
Construct a polygon mesh. | |
virtual uint32 | GetChangeNumber () const |
When this number changes you know the polygon mesh has changed (deformation has occured) since the last time you got another number from this function. | |
virtual csFlags & | GetFlags () |
Get flags for this polygon mesh. | |
virtual int | GetPolygonCount () |
Get the number of polygons for this mesh. | |
int * | GetPolygonIndices () |
Get the polygon index table. | |
virtual csMeshedPolygon * | GetPolygons () |
Get the pointer to the array of polygons. | |
virtual int | GetTriangleCount () |
Get the number of triangles for this mesh. | |
virtual csTriangle * | GetTriangles () |
Get the triangle table for this mesh. | |
virtual int | GetVertexCount () |
Get the number of vertices for this mesh. | |
virtual csVector3 * | GetVertices () |
Get the pointer to the array of vertices. | |
virtual void | Lock () |
Lock the polygon mesh. | |
void | SetPolygonCount (int po_count) |
Set polygon count. | |
void | SetPolygonIndexCount (int po_index_count) |
Set polygon index count. | |
void | SetPolygonIndices (int *po_indices, bool delete_po_indices) |
Set polygon indices used by SetPolygons(). | |
void | SetPolygons (csMeshedPolygon *po, int po_count, bool delete_po) |
Set the polygons to use for this polygon mesh. | |
void | SetVertexCount (int vt_count) |
Set vertex count. | |
void | SetVertices (csVector3 *vt, int vt_count, bool delete_vt) |
Set the vertices to use for this polygon mesh. | |
void | ShapeChanged () |
Indicate that the shape has changed. | |
virtual void | Unlock () |
Unlock the polygon mesh. | |
virtual | ~csPolygonMesh () |
Detailed Description
A convenience polygon mesh implementation that you can feed with vertices and polygons from another source.It will automatically calculate the triangles if requested.
Definition at line 47 of file polymesh.h.
Constructor & Destructor Documentation
csPolygonMesh::csPolygonMesh | ( | ) | [inline] |
Member Function Documentation
virtual uint32 csPolygonMesh::GetChangeNumber | ( | ) | const [inline, virtual] |
When this number changes you know the polygon mesh has changed (deformation has occured) since the last time you got another number from this function.
Implements iPolygonMesh.
Definition at line 206 of file polymesh.h.
virtual csFlags& csPolygonMesh::GetFlags | ( | ) | [inline, virtual] |
Get flags for this polygon mesh.
This is zero or a combination of the following flags:
- CS_POLYMESH_CLOSED: mesh is closed.
- CS_POLYMESH_NOTCLOSED: mesh is not closed.
- CS_POLYMESH_CONVEX: mesh is convex.
- CS_POLYMESH_NOTCONVEX: mesh is not convex.
- CS_POLYMESH_DEFORMABLE: mesh is deformable.
- CS_POLYMESH_TRIANGLEMESH: mesh prefers triangle mesh.
Note that if neither CS_POLYMESH_CLOSED nor CS_POLYMESH_NOTCLOSED are set then the closed state is not known. Setting both is illegal. Note that if neither CS_POLYMESH_CONVEX nor CS_POLYMESH_NOTCONVEX are set then the convex state is not known. Setting both is illegal.
Implements iPolygonMesh.
Definition at line 205 of file polymesh.h.
virtual int csPolygonMesh::GetPolygonCount | ( | ) | [inline, virtual] |
Get the number of polygons for this mesh.
Implements iPolygonMesh.
Definition at line 191 of file polymesh.h.
int* csPolygonMesh::GetPolygonIndices | ( | ) | [inline] |
virtual csMeshedPolygon* csPolygonMesh::GetPolygons | ( | ) | [inline, virtual] |
Get the pointer to the array of polygons.
Implements iPolygonMesh.
Definition at line 192 of file polymesh.h.
virtual int csPolygonMesh::GetTriangleCount | ( | ) | [inline, virtual] |
Get the number of triangles for this mesh.
Implements iPolygonMesh.
Definition at line 193 of file polymesh.h.
virtual csTriangle* csPolygonMesh::GetTriangles | ( | ) | [inline, virtual] |
Get the triangle table for this mesh.
Implements iPolygonMesh.
Definition at line 198 of file polymesh.h.
virtual int csPolygonMesh::GetVertexCount | ( | ) | [inline, virtual] |
Get the number of vertices for this mesh.
Implements iPolygonMesh.
Definition at line 189 of file polymesh.h.
virtual csVector3* csPolygonMesh::GetVertices | ( | ) | [inline, virtual] |
Get the pointer to the array of vertices.
Implements iPolygonMesh.
Definition at line 190 of file polymesh.h.
virtual void csPolygonMesh::Lock | ( | ) | [inline, virtual] |
Lock the polygon mesh.
This prevents the polygon and triangle data from being cleaned up.
Implements iPolygonMesh.
Definition at line 203 of file polymesh.h.
void csPolygonMesh::SetPolygonCount | ( | int | po_count | ) | [inline] |
Set polygon count.
This will make room for the specified number of polygons so that the user can update them. This class will delete the polygons itself later.
Definition at line 173 of file polymesh.h.
void csPolygonMesh::SetPolygonIndexCount | ( | int | po_index_count | ) | [inline] |
Set polygon index count.
This will make room for the specified number of polygon indices so that the user can update them. This class will delete the indices itself later.
Definition at line 142 of file polymesh.h.
void csPolygonMesh::SetPolygonIndices | ( | int * | po_indices, | |
bool | delete_po_indices | |||
) | [inline] |
void csPolygonMesh::SetPolygons | ( | csMeshedPolygon * | po, | |
int | po_count, | |||
bool | delete_po | |||
) | [inline] |
Set the polygons to use for this polygon mesh.
If 'delete_po' is true then this class will do the cleanup itself at destruction. Otherwise you have to make sure that the pointer to the polygons remains valid until this object is deleted.
Definition at line 119 of file polymesh.h.
void csPolygonMesh::SetVertexCount | ( | int | vt_count | ) | [inline] |
Set vertex count.
This will make room for the specified number of vertices so that the user can update them. This class will delete the vertices itself later.
Definition at line 160 of file polymesh.h.
void csPolygonMesh::SetVertices | ( | csVector3 * | vt, | |
int | vt_count, | |||
bool | delete_vt | |||
) | [inline] |
Set the vertices to use for this polygon mesh.
If 'delete_vt' is true then this class will do the cleanup itself at destruction. Otherwise you have to make sure that the pointer to the vertices remains valid until this object is deleted.
Definition at line 105 of file polymesh.h.
void csPolygonMesh::ShapeChanged | ( | ) | [inline] |
virtual void csPolygonMesh::Unlock | ( | ) | [inline, virtual] |
Unlock the polygon mesh.
This allows clean up again.
Implements iPolygonMesh.
Definition at line 204 of file polymesh.h.
The documentation for this class was generated from the following file:
- csgeom/polymesh.h
Generated for Crystal Space by doxygen 1.4.7