iImage Struct Reference
[2D]
The iImage interface is used to work with image objects.
More...
#include <igraphic/image.h>
Inheritance diagram for iImage:
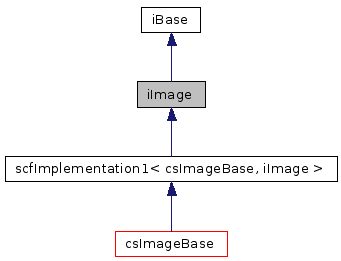
Public Member Functions | |
virtual const uint8 * | GetAlpha ()=0 |
Get alpha map for 8-bit paletted image. | |
virtual int | GetDepth () const =0 |
Query image depth (only sensible when the image type is csimg3D). | |
virtual int | GetFormat () const =0 |
Qyery image format (see CS_IMGFMT_XXX above). | |
virtual int | GetHeight () const =0 |
Query image height. | |
virtual const void * | GetImageData ()=0 |
Get image data: returns either (csRGBpixel *) or (unsigned char *) depending on format. | |
virtual csImageType | GetImageType () const =0 |
Get the type of the contained image. | |
virtual void | GetKeycolor (int &r, int &g, int &b) const =0 |
Get the keycolour stored with the image. | |
virtual void | GetKeyColor (int &r, int &g, int &b) const =0 |
Get the keycolour stored with the image. | |
virtual csRef< iImage > | GetMipmap (uint num)=0 |
Return a precomputed mipmap. | |
virtual const char * | GetName () const =0 |
Get image file name. | |
virtual const csRGBpixel * | GetPalette ()=0 |
Get image palette (or 0 if no palette). | |
virtual csRef< iDataBuffer > | GetRawData () const =0 |
Get the raw data of the image (or 0 if raw data is not provided). | |
virtual const char * | GetRawFormat () const =0 |
Get a string identifying the format of the raw data of the image (or 0 if raw data is not provided). | |
virtual csRef< iImage > | GetSubImage (uint num)=0 |
Query a sub image. | |
virtual int | GetWidth () const =0 |
Query image width. | |
virtual bool | HasKeycolor () const =0 |
Check if image has a keycolour stored with it. | |
virtual bool | HasKeyColor () const =0 |
Check if image has a keycolour stored with it. | |
virtual uint | HasMipmaps () const =0 |
Returns the number of mipmaps contained in the image (in case there exist any precalculated mipmaps), in addition to the original image. | |
virtual uint | HasSubImages () const =0 |
Returns the number of sub images, in addition to this image. | |
virtual void | SetName (const char *iName)=0 |
Set image file name. |
Detailed Description
The iImage interface is used to work with image objects.You cannot manipulate the pixel data of iImage objects directly. To do this, you need to instantiate a your own copy of the image, e.g. by creating a csImageMemory instance (which allows access to the pixel data).
Main creators of instances implementing this interface:
Definition at line 93 of file image.h.
Member Function Documentation
virtual const uint8* iImage::GetAlpha | ( | ) | [pure virtual] |
Get alpha map for 8-bit paletted image.
RGBA images contains alpha within themself. If image has no alpha map, or the image is in RGBA format, this function will return 0.
Implemented in csImageBase, csImageCubeMapMaker, csImageMemory, csImageVolumeMaker, and csCommonImageFile.
virtual int iImage::GetDepth | ( | ) | const [pure virtual] |
Query image depth (only sensible when the image type is csimg3D).
Implemented in csImageBase, csImageMemory, and csImageVolumeMaker.
Referenced by csImageTools::ComputeDataSize().
virtual int iImage::GetFormat | ( | ) | const [pure virtual] |
Qyery image format (see CS_IMGFMT_XXX above).
Implemented in csImageCubeMapMaker, csImageMemory, csImageVolumeMaker, csScreenShot, and csGLScreenShot.
Referenced by csImageTools::ComputeDataSize(), and CS::ImageAutoConvert::ImageAutoConvert().
virtual int iImage::GetHeight | ( | ) | const [pure virtual] |
Query image height.
Implemented in csImageCubeMapMaker, csImageMemory, csImageVolumeMaker, csScreenShot, and csGLScreenShot.
Referenced by csImageTools::ComputeDataSize().
virtual const void* iImage::GetImageData | ( | ) | [pure virtual] |
Get image data: returns either (csRGBpixel *) or (unsigned char *) depending on format.
Note that for RGBA images the csRGBpixel structure contains the alpha channel as well, so GetAlpha (see below) method will return 0 (because alpha is not stored separately, as for paletted images).
Implemented in csImageCubeMapMaker, csImageMemory, csImageVolumeMaker, csScreenShot, csCommonImageFile, and csGLScreenShot.
virtual csImageType iImage::GetImageType | ( | ) | const [pure virtual] |
Get the type of the contained image.
Implemented in csImageBase, csImageCubeMapMaker, csImageMemory, and csImageVolumeMaker.
virtual void iImage::GetKeycolor | ( | int & | r, | |
int & | g, | |||
int & | b | |||
) | const [pure virtual] |
Get the keycolour stored with the image.
- Deprecated:
- Use GetKeyColor() instead.
Implemented in csImageBase.
virtual void iImage::GetKeyColor | ( | int & | r, | |
int & | g, | |||
int & | b | |||
) | const [pure virtual] |
Get the keycolour stored with the image.
Implemented in csImageBase, csImageCubeMapMaker, csImageMemory, and csCommonImageFile.
Return a precomputed mipmap.
num specifies which mipmap to return; 0 returns the original image, num <= the return value of HasMipmaps() returns that mipmap.
Implemented in csImageBase, csImageCubeMapMaker, and csImageMemory.
virtual const char* iImage::GetName | ( | ) | const [pure virtual] |
virtual const csRGBpixel* iImage::GetPalette | ( | ) | [pure virtual] |
Get image palette (or 0 if no palette).
Implemented in csImageBase, csImageCubeMapMaker, csImageMemory, csImageVolumeMaker, csScreenShot, and csCommonImageFile.
virtual csRef<iDataBuffer> iImage::GetRawData | ( | ) | const [pure virtual] |
Get the raw data of the image (or 0 if raw data is not provided).
Implemented in csImageBase, csImageCubeMapMaker, csImageVolumeMaker, and csCommonImageFile.
virtual const char* iImage::GetRawFormat | ( | ) | const [pure virtual] |
Get a string identifying the format of the raw data of the image (or 0 if raw data is not provided).
Implemented in csImageBase, csImageCubeMapMaker, csImageVolumeMaker, and csCommonImageFile.
Query a sub image.
A value of 0 for num returns the original image, a value larger or equal than the return value of HasSubImages() returns that sub image, any other value returns 0.
Implemented in csImageBase, and csImageCubeMapMaker.
virtual int iImage::GetWidth | ( | ) | const [pure virtual] |
Query image width.
Implemented in csImageCubeMapMaker, csImageMemory, csImageVolumeMaker, csScreenShot, and csGLScreenShot.
Referenced by csImageTools::ComputeDataSize().
virtual bool iImage::HasKeycolor | ( | ) | const [pure virtual] |
Check if image has a keycolour stored with it.
- Deprecated:
- Use HasKeyColor() instead.
Implemented in csImageBase.
virtual bool iImage::HasKeyColor | ( | ) | const [pure virtual] |
Check if image has a keycolour stored with it.
Implemented in csImageBase, csImageCubeMapMaker, csImageMemory, and csCommonImageFile.
virtual uint iImage::HasMipmaps | ( | ) | const [pure virtual] |
Returns the number of mipmaps contained in the image (in case there exist any precalculated mipmaps), in addition to the original image.
0 means there are no precomputed mipmaps.
Implemented in csImageBase, csImageCubeMapMaker, and csImageMemory.
virtual uint iImage::HasSubImages | ( | ) | const [pure virtual] |
Returns the number of sub images, in addition to this image.
Subimages are usually used for cube map faces.
Implemented in csImageBase, and csImageCubeMapMaker.
virtual void iImage::SetName | ( | const char * | iName | ) | [pure virtual] |
The documentation for this struct was generated from the following file:
- igraphic/image.h
Generated for Crystal Space by doxygen 1.4.7