csImageMemory Class Reference
[Graphics]
Memory image.
More...
#include <csgfx/imagememory.h>
Inheritance diagram for csImageMemory:
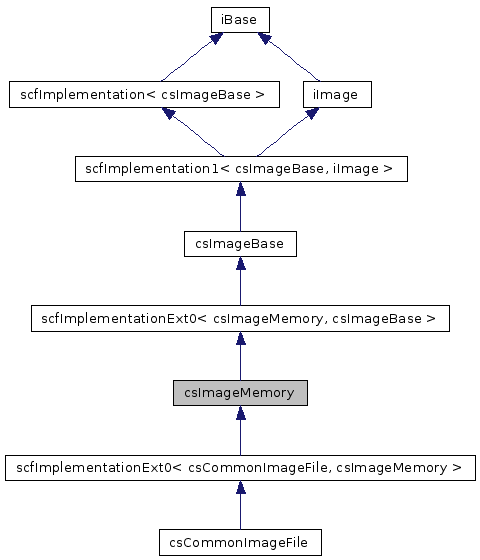
Public Member Functions | |
virtual void | ApplyKeycolor () |
virtual void | ApplyKeyColor () |
Apply the keycolor, that is, set all pixels which match the keycolor to 0. | |
void | CheckAlpha () |
Check if all alpha values are "non-transparent" and if so, discard alpha. | |
void | Clear (const csRGBpixel &colour) |
Clears image to colour. Only works for truecolor images. | |
virtual void | ClearKeycolor () |
virtual void | ClearKeyColor () |
Remove the keycolor. | |
void | ConvertFromPal8 (uint8 *iImage, uint8 *alpha, const csRGBcolor *iPalette, int nPalColors=256) |
Used to convert an 8-bit indexed image into requested format. | |
void | ConvertFromPal8 (uint8 *iImage, uint8 *alpha, csRGBpixel *iPalette, int nPalColors=256) |
Used to convert an 8-bit indexed image into requested format. | |
void | ConvertFromRGBA (csRGBpixel *iImage) |
Used to convert an truecolor RGB image into requested format. | |
bool | Copy (iImage *srcImage, int x, int y, int width, int height) |
Copy an image as subpart into this image. | |
bool | CopyScale (iImage *srcImage, int x, int y, int width, int height) |
Copy an image as subpart into this image with scaling sImage to the given size. | |
bool | CopyTile (iImage *srcImage, int x, int y, int width, int height) |
Copy an image as subpart into this image and tile and scale sImage to the given size. | |
csImageMemory (iImage *source, int newFormat) | |
Create an instance that copies the pixel data from another iImage object, and also change the data format. | |
csImageMemory (iImage *source) | |
Create an instance that copies the pixel data from another iImage object. | |
csImageMemory (int width, int height, const void *buffer, int format=CS_IMGFMT_TRUECOLOR, const csRGBpixel *palette=0) | |
Create an instance from a pixel buffer with these dimensions. | |
csImageMemory (int width, int height, void *buffer, bool destroy, int format=CS_IMGFMT_TRUECOLOR, csRGBpixel *palette=0) | |
Create an instance for this pixel buffer with these dimensions. | |
csImageMemory (int width, int height, int depth, int format) | |
Create a blank image of these dimensions and the specified format. | |
csImageMemory (int width, int height, int format=CS_IMGFMT_TRUECOLOR) | |
Create a blank image of these dimensions and the specified format. | |
virtual const uint8 * | GetAlpha () |
Get alpha map for 8-bit paletted image. | |
uint8 * | GetAlphaPtr () |
Get a pointer to the alpha data that can be changed. | |
virtual int | GetDepth () const |
Query image depth (only sensible when the image type is csimg3D). | |
virtual int | GetFormat () const |
Qyery image format (see CS_IMGFMT_XXX above). | |
virtual int | GetHeight () const |
Query image height. | |
virtual const void * | GetImageData () |
Get image data: returns either (csRGBpixel *) or (unsigned char *) depending on format. | |
void * | GetImagePtr () |
Get a pointer to the image data that can be changed. | |
virtual csImageType | GetImageType () const |
Get the type of the contained image. | |
virtual void | GetKeyColor (int &r, int &g, int &b) const |
Get the keycolour stored with the image. | |
virtual csRef< iImage > | GetMipmap (uint num) |
Return a precomputed mipmap. | |
virtual const csRGBpixel * | GetPalette () |
Get image palette (or 0 if no palette). | |
csRGBpixel * | GetPalettePtr () |
Get a pointer to the palette data that can be changed. | |
virtual int | GetWidth () const |
Query image width. | |
virtual bool | HasKeyColor () const |
Check if image has a keycolour stored with it. | |
virtual uint | HasMipmaps () const |
Returns the number of mipmaps contained in the image (in case there exist any precalculated mipmaps), in addition to the original image. | |
void | SetFormat (int iFormat) |
Convert the image to another format. | |
void | SetImageType (csImageType type) |
virtual void | SetKeycolor (int r, int g, int b) |
virtual void | SetKeyColor (int r, int g, int b) |
Set the keycolor. | |
bool | SetMipmap (uint num, iImage *mip) |
Set a mipmap of this image. | |
Protected Member Functions | |
void | AllocImage () |
Allocate the pixel data buffers. | |
csImageMemory (int iFormat) | |
csImageMemory constructor, only set a format, no dimensions. | |
void | EnsureImage () |
Allocate the pixel data buffers if they aren't already. | |
void | FreeImage () |
Free all image data: pixels and palette. | |
void | SetDimensions (int newWidth, int newHeight) |
Set the width and height. | |
Protected Attributes | |
uint8 * | Alpha |
The alpha map. | |
int | Depth |
Depth of image. | |
bool | destroy_image |
If true when these interfaces are destroyed the image is also. | |
int | Format |
Image format (see CS_IMGFMT_XXX above). | |
bool | has_keycolour |
if it has a keycolour. | |
int | Height |
Height of image. | |
void * | Image |
The image data. | |
csImageType | imageType |
Type of the contained images. | |
csRGBpixel | keycolour |
keycolour value | |
csArray< csRef< iImage > > | mipmaps |
Mip map images. | |
csRGBpixel * | Palette |
The image palette or 0. | |
int | Width |
Width of image. |
Detailed Description
Memory image.
Definition at line 40 of file imagememory.h.
Constructor & Destructor Documentation
csImageMemory::csImageMemory | ( | int | iFormat | ) | [protected] |
csImageMemory constructor, only set a format, no dimensions.
Intended to be used by loaders which later call SetDimensions().
csImageMemory::csImageMemory | ( | int | width, | |
int | height, | |||
int | format = CS_IMGFMT_TRUECOLOR | |||
) |
Create a blank image of these dimensions and the specified format.
- Parameters:
-
width Width of the image height Height of the image format Image format. Default: CS_IMGFMT_TRUECOLOR
csImageMemory::csImageMemory | ( | int | width, | |
int | height, | |||
int | depth, | |||
int | format | |||
) |
Create a blank image of these dimensions and the specified format.
- Parameters:
-
width Width of the image height Height of the image depth Height of the image format Image format.
csImageMemory::csImageMemory | ( | int | width, | |
int | height, | |||
void * | buffer, | |||
bool | destroy, | |||
int | format = CS_IMGFMT_TRUECOLOR , |
|||
csRGBpixel * | palette = 0 | |||
) |
Create an instance for this pixel buffer with these dimensions.
If destroy is set to true then the supplied buffer will be destroyed when the instance is.
- Parameters:
-
width Width of the image height Height of the image buffer Data containing initial data destroy Destroy the buffer when the Image is destroyed format Image format. Data in - buffer must be in this format. Default: CS_IMGFMT_TRUECOLOR
palette Palette for indexed images.
csImageMemory::csImageMemory | ( | int | width, | |
int | height, | |||
const void * | buffer, | |||
int | format = CS_IMGFMT_TRUECOLOR , |
|||
const csRGBpixel * | palette = 0 | |||
) |
Create an instance from a pixel buffer with these dimensions.
A copy of the pixel data is made.
- Parameters:
-
width Width of the image height Height of the image buffer Data containing initial data format Image format. Data in - buffer must be in this format. Default: CS_IMGFMT_TRUECOLOR
palette Palette for indexed images.
csImageMemory::csImageMemory | ( | iImage * | source | ) |
Create an instance that copies the pixel data from another iImage object.
csImageMemory::csImageMemory | ( | iImage * | source, | |
int | newFormat | |||
) |
Create an instance that copies the pixel data from another iImage object, and also change the data format.
Member Function Documentation
void csImageMemory::AllocImage | ( | ) | [protected] |
Allocate the pixel data buffers.
virtual void csImageMemory::ApplyKeyColor | ( | ) | [virtual] |
Apply the keycolor, that is, set all pixels which match the keycolor to 0.
void csImageMemory::CheckAlpha | ( | ) |
Check if all alpha values are "non-transparent" and if so, discard alpha.
void csImageMemory::Clear | ( | const csRGBpixel & | colour | ) |
Clears image to colour. Only works for truecolor images.
virtual void csImageMemory::ClearKeyColor | ( | ) | [virtual] |
Remove the keycolor.
void csImageMemory::ConvertFromPal8 | ( | uint8 * | iImage, | |
uint8 * | alpha, | |||
const csRGBcolor * | iPalette, | |||
int | nPalColors = 256 | |||
) |
Used to convert an 8-bit indexed image into requested format.
Pass a pointer to color indices, a pointer to the alpha mask and a pointer to the palette, and you're done. NOTE: the pointer should be allocated with new uint8 [] and you should not free it after calling this function: the function will free the buffer itself if it is appropriate (or wont if the buffer size/contents are appropriate for target format). Same about palette and alpha.
void csImageMemory::ConvertFromPal8 | ( | uint8 * | iImage, | |
uint8 * | alpha, | |||
csRGBpixel * | iPalette, | |||
int | nPalColors = 256 | |||
) |
Used to convert an 8-bit indexed image into requested format.
Pass a pointer to color indices, a pointer to the alpha mask and a pointer to the palette, and you're done. NOTE: the pointer should be allocated with new uint8 [] and you should not free it after calling this function: the function will free the buffer itself if it is appropriate (or wont if the buffer size/contents are appropriate for target format). Same about palette and alpha.
void csImageMemory::ConvertFromRGBA | ( | csRGBpixel * | iImage | ) |
Used to convert an truecolor RGB image into requested format.
If the image loader cannot handle conversion itself, and the image file is in a format that is different from the requested one, load the image in csRGBpixel format and pass the pointer to this function which will handle the RGB -> target format conversion. NOTE: the pointer should be allocated with new csRGBpixel [] and you should not free it after calling this function: the function will free the buffer itself if it is appropriate (or wont if the buffer size/contents are appropriate for target format).
bool csImageMemory::Copy | ( | iImage * | srcImage, | |
int | x, | |||
int | y, | |||
int | width, | |||
int | height | |||
) |
Copy an image as subpart into this image.
bool csImageMemory::CopyScale | ( | iImage * | srcImage, | |
int | x, | |||
int | y, | |||
int | width, | |||
int | height | |||
) |
Copy an image as subpart into this image with scaling sImage to the given size.
bool csImageMemory::CopyTile | ( | iImage * | srcImage, | |
int | x, | |||
int | y, | |||
int | width, | |||
int | height | |||
) |
Copy an image as subpart into this image and tile and scale sImage to the given size.
void csImageMemory::EnsureImage | ( | ) | [protected] |
Allocate the pixel data buffers if they aren't already.
void csImageMemory::FreeImage | ( | ) | [protected] |
Free all image data: pixels and palette.
Takes care of image data format.
virtual const uint8* csImageMemory::GetAlpha | ( | ) | [inline, virtual] |
Get alpha map for 8-bit paletted image.
RGBA images contains alpha within themself. If image has no alpha map, or the image is in RGBA format, this function will return 0.
Reimplemented from csImageBase.
Reimplemented in csCommonImageFile.
Definition at line 185 of file imagememory.h.
uint8* csImageMemory::GetAlphaPtr | ( | ) |
Get a pointer to the alpha data that can be changed.
virtual int csImageMemory::GetDepth | ( | ) | const [inline, virtual] |
Query image depth (only sensible when the image type is csimg3D).
Reimplemented from csImageBase.
Definition at line 181 of file imagememory.h.
virtual int csImageMemory::GetFormat | ( | ) | const [inline, virtual] |
Qyery image format (see CS_IMGFMT_XXX above).
Implements iImage.
Definition at line 183 of file imagememory.h.
virtual int csImageMemory::GetHeight | ( | ) | const [inline, virtual] |
virtual const void* csImageMemory::GetImageData | ( | ) | [inline, virtual] |
Get image data: returns either (csRGBpixel *) or (unsigned char *) depending on format.
Note that for RGBA images the csRGBpixel structure contains the alpha channel as well, so GetAlpha (see below) method will return 0 (because alpha is not stored separately, as for paletted images).
Implements iImage.
Reimplemented in csCommonImageFile.
Definition at line 178 of file imagememory.h.
void* csImageMemory::GetImagePtr | ( | ) |
Get a pointer to the image data that can be changed.
virtual csImageType csImageMemory::GetImageType | ( | ) | const [inline, virtual] |
Get the type of the contained image.
Reimplemented from csImageBase.
Definition at line 222 of file imagememory.h.
virtual void csImageMemory::GetKeyColor | ( | int & | r, | |
int & | g, | |||
int & | b | |||
) | const [inline, virtual] |
Get the keycolour stored with the image.
Reimplemented from csImageBase.
Reimplemented in csCommonImageFile.
Definition at line 189 of file imagememory.h.
Return a precomputed mipmap.
num specifies which mipmap to return; 0 returns the original image, num <= the return value of HasMipmaps() returns that mipmap.
Reimplemented from csImageBase.
Definition at line 231 of file imagememory.h.
virtual const csRGBpixel* csImageMemory::GetPalette | ( | ) | [inline, virtual] |
Get image palette (or 0 if no palette).
Reimplemented from csImageBase.
Reimplemented in csCommonImageFile.
Definition at line 184 of file imagememory.h.
csRGBpixel* csImageMemory::GetPalettePtr | ( | ) |
Get a pointer to the palette data that can be changed.
virtual int csImageMemory::GetWidth | ( | ) | const [inline, virtual] |
virtual bool csImageMemory::HasKeyColor | ( | ) | const [inline, virtual] |
Check if image has a keycolour stored with it.
Reimplemented from csImageBase.
Reimplemented in csCommonImageFile.
Definition at line 187 of file imagememory.h.
virtual uint csImageMemory::HasMipmaps | ( | ) | const [inline, virtual] |
Returns the number of mipmaps contained in the image (in case there exist any precalculated mipmaps), in addition to the original image.
0 means there are no precomputed mipmaps.
Reimplemented from csImageBase.
Definition at line 225 of file imagememory.h.
void csImageMemory::SetDimensions | ( | int | newWidth, | |
int | newHeight | |||
) | [protected] |
Set the width and height.
This will also free the 'image' buffer to hold the pixel data, but it will NOT allocate a new buffer (thus `image' is 0 after calling this function). You should pass an appropiate pointer to one of ConvertXXX functions below, define the image itself (or assign something to `Image' manually), or call EnsureImage().
void csImageMemory::SetFormat | ( | int | iFormat | ) |
Convert the image to another format.
This method will allocate a respective color component if it was not allocated before. For example, you can use this method to add alpha channel to paletted images, to allocate a image for CS_IMGFMT_NONE alphamaps or vice versa, to remove the image and leave alphamap alone. This routine may be used as well for removing alpha channel.
virtual void csImageMemory::SetKeyColor | ( | int | r, | |
int | g, | |||
int | b | |||
) | [virtual] |
Set the keycolor.
Set a mipmap of this image.
- Remarks:
- You can set any mipmap except number 0 to your liking, however, no sanity checking is performed! That is, you can set mipmaps to 0, leave out mipmaps and provide more mipmaps than the image really needs - this can be problematic as most clients will expect non-null mipmaps. (Note however that trailing 0 mipmaps will not be reported.)
Definition at line 245 of file imagememory.h.
Member Data Documentation
uint8* csImageMemory::Alpha [protected] |
int csImageMemory::Depth [protected] |
bool csImageMemory::destroy_image [protected] |
If true when these interfaces are destroyed the image is also.
Definition at line 78 of file imagememory.h.
int csImageMemory::Format [protected] |
bool csImageMemory::has_keycolour [protected] |
int csImageMemory::Height [protected] |
void* csImageMemory::Image [protected] |
The image data.
A value of 0 means the Image contents are "undefined". However, this is an internal state only. No operation should fail due undefined image data (although the data may stay undefined).
Definition at line 66 of file imagememory.h.
csImageType csImageMemory::imageType [protected] |
csRGBpixel csImageMemory::keycolour [protected] |
csArray<csRef<iImage> > csImageMemory::mipmaps [protected] |
csRGBpixel* csImageMemory::Palette [protected] |
int csImageMemory::Width [protected] |
The documentation for this class was generated from the following file:
- csgfx/imagememory.h
Generated for Crystal Space by doxygen 1.4.7