iODEGeneralJointState Struct Reference
General joint state. More...
#include <ivaria/ode.h>
Inheritance diagram for iODEGeneralJointState:
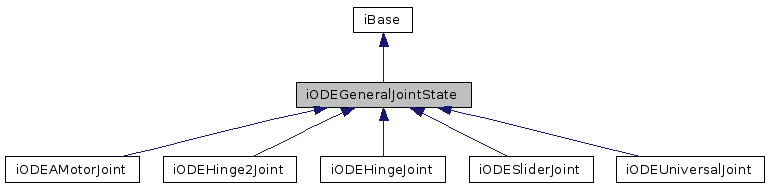
Public Member Functions | |
virtual void | Attach (iRigidBody *body1, iRigidBody *body2)=0 |
Attach the joint to some new bodies. | |
virtual csRef< iRigidBody > | GetAttachedBody (int body)=0 |
Get an attached body (valid values for body are 0 and 1). | |
virtual float | GetBounce (int axis)=0 |
Get the bouncyness of the stops. | |
virtual float | GetCFM (int axis)=0 |
Get the constraint force mixing (CFM) value for joint used when not at a stop. | |
virtual csVector3 | GetFeedbackForce1 ()=0 |
Get force that joint applies to body 1. | |
virtual csVector3 | GetFeedbackForce2 ()=0 |
Get force that joint applies to body 2. | |
virtual csVector3 | GetFeedbackTorque1 ()=0 |
Get torque that joint applies to body 1. | |
virtual csVector3 | GetFeedbackTorque2 ()=0 |
Get torque that joint applies to body 2. | |
virtual float | GetFMax (int axis)=0 |
Get the maximum force or torque that the motor will use to achieve the desired velocity. | |
virtual float | GetFudgeFactor (int axis)=0 |
Get the fudge factor. | |
virtual float | GetHiStop (int axis)=0 |
Get high stop angle or position. | |
virtual float | GetLoStop (int axis)=0 |
Get low stop angle or position. | |
virtual float | GetStopCFM (int axis)=0 |
Get the constraint force mixing (CFM) value for joint used by the stops. | |
virtual float | GetStopERP (int axis)=0 |
Get the error reduction parameter (ERP) used by the stops. | |
virtual float | GetSuspensionCFM (int axis)=0 |
Get suspension constraint force mixing (CFM) value. | |
virtual float | GetSuspensionERP (int axis)=0 |
Get suspension error reduction parameter (ERP). | |
virtual float | GetVel (int axis)=0 |
Get desired motor velocity (this will be an angular or linear velocity). | |
virtual void | SetBounce (float value, int axis)=0 |
Set the bouncyness of the stops. | |
virtual void | SetCFM (float value, int axis)=0 |
Set the constraint force mixing (CFM) value for joint used when not at a stop. | |
virtual void | SetFMax (float value, int axis)=0 |
Set the maximum force or torque that the motor will use to achieve the desired velocity. | |
virtual void | SetFudgeFactor (float value, int axis)=0 |
Set the fudge factor. | |
virtual void | SetHiStop (float value, int axis)=0 |
Set high stop angle or position. | |
virtual void | SetLoStop (float value, int axis)=0 |
Set low stop angle or position. | |
virtual void | SetStopCFM (float value, int axis)=0 |
Set the constraint force mixing (CFM) value for joint used by the stops. | |
virtual void | SetStopERP (float value, int axis)=0 |
Set the error reduction parameter (ERP) used by the stops. | |
virtual void | SetSuspensionCFM (float value, int axis)=0 |
Set suspension constraint force mixing (CFM) value. | |
virtual void | SetSuspensionERP (float value, int axis)=0 |
Set suspension error reduction parameter (ERP). | |
virtual void | SetVel (float value, int axis)=0 |
Set desired motor velocity (this will be an angular or linear velocity). |
Detailed Description
General joint state.
Definition at line 440 of file ode.h.
Member Function Documentation
virtual void iODEGeneralJointState::Attach | ( | iRigidBody * | body1, | |
iRigidBody * | body2 | |||
) | [pure virtual] |
Attach the joint to some new bodies.
If the joint is already attached, it will be detached from the old bodies first. To attach this joint to only one body, set body1 or body2 to zero - a zero body refers to the static environment. Setting both bodies to zero puts the joint into "limbo", i.e. it will have no effect on the simulation.
virtual csRef<iRigidBody> iODEGeneralJointState::GetAttachedBody | ( | int | body | ) | [pure virtual] |
Get an attached body (valid values for body are 0 and 1).
virtual float iODEGeneralJointState::GetBounce | ( | int | axis | ) | [pure virtual] |
Get the bouncyness of the stops.
virtual float iODEGeneralJointState::GetCFM | ( | int | axis | ) | [pure virtual] |
Get the constraint force mixing (CFM) value for joint used when not at a stop.
virtual csVector3 iODEGeneralJointState::GetFeedbackForce1 | ( | ) | [pure virtual] |
Get force that joint applies to body 1.
virtual csVector3 iODEGeneralJointState::GetFeedbackForce2 | ( | ) | [pure virtual] |
Get force that joint applies to body 2.
virtual csVector3 iODEGeneralJointState::GetFeedbackTorque1 | ( | ) | [pure virtual] |
Get torque that joint applies to body 1.
virtual csVector3 iODEGeneralJointState::GetFeedbackTorque2 | ( | ) | [pure virtual] |
Get torque that joint applies to body 2.
virtual float iODEGeneralJointState::GetFMax | ( | int | axis | ) | [pure virtual] |
Get the maximum force or torque that the motor will use to achieve the desired velocity.
virtual float iODEGeneralJointState::GetFudgeFactor | ( | int | axis | ) | [pure virtual] |
Get the fudge factor.
virtual float iODEGeneralJointState::GetHiStop | ( | int | axis | ) | [pure virtual] |
Get high stop angle or position.
virtual float iODEGeneralJointState::GetLoStop | ( | int | axis | ) | [pure virtual] |
Get low stop angle or position.
virtual float iODEGeneralJointState::GetStopCFM | ( | int | axis | ) | [pure virtual] |
Get the constraint force mixing (CFM) value for joint used by the stops.
virtual float iODEGeneralJointState::GetStopERP | ( | int | axis | ) | [pure virtual] |
Get the error reduction parameter (ERP) used by the stops.
virtual float iODEGeneralJointState::GetSuspensionCFM | ( | int | axis | ) | [pure virtual] |
Get suspension constraint force mixing (CFM) value.
virtual float iODEGeneralJointState::GetSuspensionERP | ( | int | axis | ) | [pure virtual] |
Get suspension error reduction parameter (ERP).
virtual float iODEGeneralJointState::GetVel | ( | int | axis | ) | [pure virtual] |
Get desired motor velocity (this will be an angular or linear velocity).
virtual void iODEGeneralJointState::SetBounce | ( | float | value, | |
int | axis | |||
) | [pure virtual] |
Set the bouncyness of the stops.
This is a restitution parameter in the range 0..1. 0 means the stops are not bouncy at all, 1 means maximum bouncyness.
virtual void iODEGeneralJointState::SetCFM | ( | float | value, | |
int | axis | |||
) | [pure virtual] |
Set the constraint force mixing (CFM) value for joint used when not at a stop.
virtual void iODEGeneralJointState::SetFMax | ( | float | value, | |
int | axis | |||
) | [pure virtual] |
Set the maximum force or torque that the motor will use to achieve the desired velocity.
This must always be greater than or equal to zero. Setting this to zero turns off the motor.
virtual void iODEGeneralJointState::SetFudgeFactor | ( | float | value, | |
int | axis | |||
) | [pure virtual] |
Set the fudge factor.
The current joint stop/motor implementation has a small problem: when the joint is at one stop and the motor is set to move it away from the stop, too much force may be applied for one time step, causing a ``jumping'' motion. This fudge factor is used to scale this excess force. It should have a value between zero and one (the default value). If the jumping motion is too visible in a joint, the value can be reduced. Making this value too small can prevent the motor from being able to move the joint away from a stop.
virtual void iODEGeneralJointState::SetHiStop | ( | float | value, | |
int | axis | |||
) | [pure virtual] |
Set high stop angle or position.
For rotational joints, this stop must be less than pi to be effective. If the high stop is less than the low stop then both stops will be ineffective.
virtual void iODEGeneralJointState::SetLoStop | ( | float | value, | |
int | axis | |||
) | [pure virtual] |
Set low stop angle or position.
For rotational joints, this stop must be greater than - pi to be effective.
virtual void iODEGeneralJointState::SetStopCFM | ( | float | value, | |
int | axis | |||
) | [pure virtual] |
Set the constraint force mixing (CFM) value for joint used by the stops.
Together with the ERP value this can be used to get spongy or soft stops. Note that this is intended for unpowered joints, it does not really work as expected when a powered joint reaches its limit.
virtual void iODEGeneralJointState::SetStopERP | ( | float | value, | |
int | axis | |||
) | [pure virtual] |
Set the error reduction parameter (ERP) used by the stops.
virtual void iODEGeneralJointState::SetSuspensionCFM | ( | float | value, | |
int | axis | |||
) | [pure virtual] |
Set suspension constraint force mixing (CFM) value.
virtual void iODEGeneralJointState::SetSuspensionERP | ( | float | value, | |
int | axis | |||
) | [pure virtual] |
Set suspension error reduction parameter (ERP).
virtual void iODEGeneralJointState::SetVel | ( | float | value, | |
int | axis | |||
) | [pure virtual] |
Set desired motor velocity (this will be an angular or linear velocity).
The documentation for this struct was generated from the following file:
- ivaria/ode.h
Generated for Crystal Space by doxygen 1.4.7