iRigidBody Struct Reference
This is the interface for a rigid body. More...
#include <ivaria/dynamics.h>
Inheritance diagram for iRigidBody:
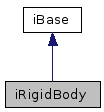
Public Member Functions | |
virtual void | AddForce (const csVector3 &force)=0 |
Add a force (world space) (active for one timestep). | |
virtual void | AddForceAtPos (const csVector3 &force, const csVector3 &pos)=0 |
Add a force (world space) at a specific position (world space) (active for one timestep). | |
virtual void | AddForceAtRelPos (const csVector3 &force, const csVector3 &pos)=0 |
Add a force (world space) at a specific position (local space) (active for one timestep). | |
virtual void | AddRelForce (const csVector3 &force)=0 |
Add a force (local space) (active for one timestep). | |
virtual void | AddRelForceAtPos (const csVector3 &force, const csVector3 &pos)=0 |
Add a force (local space) at a specific position (world space) (active for one timestep). | |
virtual void | AddRelForceAtRelPos (const csVector3 &force, const csVector3 &pos)=0 |
Add a force (local space) at a specific position (loacl space) (active for one timestep). | |
virtual void | AddRelTorque (const csVector3 &force)=0 |
Add a torque (local space) (active for one timestep). | |
virtual void | AddTorque (const csVector3 &force)=0 |
Add a torque (world space) (active for one timestep). | |
virtual void | AdjustTotalMass (float targetmass)=0 |
Set total mass to targetmass, and adjust properties. | |
virtual void | AttachCamera (iCamera *camera)=0 |
Attach an iCamera to this body. | |
virtual void | AttachCollider (iDynamicsSystemCollider *collider)=0 |
Attach collider to rigid body. | |
virtual bool | AttachColliderBox (const csVector3 &size, const csOrthoTransform &trans, float friction, float density, float elasticity, float softness=0.01f)=0 |
Add a collider box with given properties. | |
virtual bool | AttachColliderCylinder (float length, float radius, const csOrthoTransform &trans, float friction, float density, float elasticity, float softness=0.01f)=0 |
Cylinder orientated along its local z axis. | |
virtual bool | AttachColliderMesh (iMeshWrapper *mesh, const csOrthoTransform &trans, float friction, float density, float elasticity, float softness=0.01f)=0 |
Add a collider with a associated friction coefficient. | |
virtual bool | AttachColliderPlane (const csPlane3 &plane, float friction, float density, float elasticity, float softness=0.01f)=0 |
Add a collider plane with given properties. | |
virtual bool | AttachColliderSphere (float radius, const csVector3 &offset, float friction, float density, float elasticity, float softness=0.01f)=0 |
Add a collider sphere with given properties. | |
virtual void | AttachLight (iLight *light)=0 |
Attach an iLight to this body. | |
virtual void | AttachMesh (iMeshWrapper *mesh)=0 |
Attach an iMeshWrapper to this body. | |
virtual void | Collision (iRigidBody *other, const csVector3 &pos, const csVector3 &normal, float depth)=0 |
If there's a collision callback with this body, execute it. | |
virtual void | DestroyCollider (iDynamicsSystemCollider *collider)=0 |
Destroy body collider. | |
virtual void | DestroyColliders ()=0 |
Destroy body colliders. | |
virtual bool | Disable (void)=0 |
Temporarily ignores the body until something collides with it. | |
virtual bool | Enable (void)=0 |
Re-enables a body after calling Disable, or by being auto disabled. | |
virtual const csVector3 | GetAngularVelocity () const =0 |
Get the angular velocity (rotation). | |
virtual iCamera * | GetAttachedCamera ()=0 |
Returns the attached camera. | |
virtual iLight * | GetAttachedLight ()=0 |
Returns the attached light. | |
virtual iMeshWrapper * | GetAttachedMesh ()=0 |
Returns the attached MeshWrapper. | |
virtual csVector3 | GetCenter ()=0 |
Get center. | |
virtual csRef< iDynamicsSystemCollider > | GetCollider (unsigned int index)=0 |
Get body collider by its index. | |
virtual int | GetColliderCount ()=0 |
Get body colliders count. | |
virtual const csVector3 | GetForce () const =0 |
Get total force (world space). | |
virtual csRef< iBodyGroup > | GetGroup (void)=0 |
Returns which group a body belongs to. | |
virtual csMatrix3 | GetInertia ()=0 |
Get inertia. | |
virtual const csVector3 | GetLinearVelocity () const =0 |
Get the linear velocity (movement). | |
virtual float | GetMass ()=0 |
Get mass. | |
virtual const csMatrix3 | GetOrientation () const =0 |
Get the orientation. | |
virtual const csVector3 | GetPosition () const =0 |
Get the position. | |
virtual void | GetProperties (float *mass, csVector3 *center, csMatrix3 *inertia)=0 |
Get the physic properties. 0 parameters are ignored. | |
virtual const csVector3 | GetTorque () const =0 |
Get total torque (world space). | |
virtual const csOrthoTransform | GetTransform () const =0 |
Get the transform. | |
virtual bool | IsEnabled (void)=0 |
Returns true if a body is enabled. | |
virtual bool | IsStatic (void)=0 |
Tells whether a body has been made static or not. | |
virtual bool | MakeDynamic (void)=0 |
Returns a static body to a dynamic state. | |
virtual bool | MakeStatic (void)=0 |
Makes a body stop reacting dynamically. | |
virtual iObject * | QueryObject (void)=0 |
returns the underlying object | |
virtual void | SetAngularVelocity (const csVector3 &vel)=0 |
Set the angular velocity (rotation). | |
virtual void | SetCollisionCallback (iDynamicsCollisionCallback *cb)=0 |
Set a callback to be executed when this body collides with another If 0, no callback is executed. | |
virtual void | SetLinearVelocity (const csVector3 &vel)=0 |
Set the linear velocity (movement). | |
virtual void | SetMoveCallback (iDynamicsMoveCallback *cb)=0 |
Set a callback to be executed when this body moves. | |
virtual void | SetOrientation (const csMatrix3 &trans)=0 |
Set the orientation. | |
virtual void | SetPosition (const csVector3 &trans)=0 |
Set the position. | |
virtual void | SetProperties (float mass, const csVector3 ¢er, const csMatrix3 &inertia)=0 |
Set the physic properties. | |
virtual void | SetTransform (const csOrthoTransform &trans)=0 |
Set the transform. | |
virtual void | Update ()=0 |
Update transforms for mesh and/or bone. |
Detailed Description
This is the interface for a rigid body.It keeps all properties for the body. It can also be attached to a movable or a bone, to automatically update it.
Main creators of instances implementing this interface:
Main ways to get pointers to this interface:
Main users of this interface:
Definition at line 360 of file dynamics.h.
Member Function Documentation
virtual void iRigidBody::AddForce | ( | const csVector3 & | force | ) | [pure virtual] |
Add a force (world space) (active for one timestep).
virtual void iRigidBody::AddForceAtPos | ( | const csVector3 & | force, | |
const csVector3 & | pos | |||
) | [pure virtual] |
Add a force (world space) at a specific position (world space) (active for one timestep).
virtual void iRigidBody::AddForceAtRelPos | ( | const csVector3 & | force, | |
const csVector3 & | pos | |||
) | [pure virtual] |
Add a force (world space) at a specific position (local space) (active for one timestep).
virtual void iRigidBody::AddRelForce | ( | const csVector3 & | force | ) | [pure virtual] |
Add a force (local space) (active for one timestep).
virtual void iRigidBody::AddRelForceAtPos | ( | const csVector3 & | force, | |
const csVector3 & | pos | |||
) | [pure virtual] |
Add a force (local space) at a specific position (world space) (active for one timestep).
virtual void iRigidBody::AddRelForceAtRelPos | ( | const csVector3 & | force, | |
const csVector3 & | pos | |||
) | [pure virtual] |
Add a force (local space) at a specific position (loacl space) (active for one timestep).
virtual void iRigidBody::AddRelTorque | ( | const csVector3 & | force | ) | [pure virtual] |
Add a torque (local space) (active for one timestep).
virtual void iRigidBody::AddTorque | ( | const csVector3 & | force | ) | [pure virtual] |
Add a torque (world space) (active for one timestep).
virtual void iRigidBody::AdjustTotalMass | ( | float | targetmass | ) | [pure virtual] |
Set total mass to targetmass, and adjust properties.
virtual void iRigidBody::AttachCamera | ( | iCamera * | camera | ) | [pure virtual] |
Attach an iCamera to this body.
virtual void iRigidBody::AttachCollider | ( | iDynamicsSystemCollider * | collider | ) | [pure virtual] |
Attach collider to rigid body.
If you have set colliders transform before then it will be considered as relative to attached body (but still if you will use colliders "GetTransform ()" it will be in the world coordinates. Colider become dynamic (which means that it will follow rigid body).
virtual bool iRigidBody::AttachColliderBox | ( | const csVector3 & | size, | |
const csOrthoTransform & | trans, | |||
float | friction, | |||
float | density, | |||
float | elasticity, | |||
float | softness = 0.01f | |||
) | [pure virtual] |
Add a collider box with given properties.
- Parameters:
-
size the box's dimensions trans a hard transform to apply to the mesh friction how much friction this body has, ranges from 0 (no friction) to infinity (perfect friction) density the density of this rigid body (used to calculate mass based on collider volume) elasticity the "bouncyness" of this object, from 0 (no bounce) to 1 (maximum bouncyness) softness how "squishy" this object is, in the range 0...1; small values (range of 0.00001 to 0.01) give reasonably stiff collision contacts, larger values are more "mushy"
virtual bool iRigidBody::AttachColliderCylinder | ( | float | length, | |
float | radius, | |||
const csOrthoTransform & | trans, | |||
float | friction, | |||
float | density, | |||
float | elasticity, | |||
float | softness = 0.01f | |||
) | [pure virtual] |
Cylinder orientated along its local z axis.
- Parameters:
-
length length of the cylinder radius radius of the cylinder trans a hard transform to apply to the mesh friction how much friction this body has, ranges from 0 (no friction) to infinity (perfect friction) density the density of this rigid body (used to calculate mass based on collider volume) elasticity the "bouncyness" of this object, from 0 (no bounce) to 1 (maximum bouncyness) softness how "squishy" this object is, in the range 0...1; small values (range of 0.00001 to 0.01) give reasonably stiff collision contacts, larger values are more "mushy"
virtual bool iRigidBody::AttachColliderMesh | ( | iMeshWrapper * | mesh, | |
const csOrthoTransform & | trans, | |||
float | friction, | |||
float | density, | |||
float | elasticity, | |||
float | softness = 0.01f | |||
) | [pure virtual] |
Add a collider with a associated friction coefficient.
- Parameters:
-
mesh the mesh object which will act as collider trans a hard transform to apply to the mesh friction how much friction this body has, ranges from 0 (no friction) to infinity (perfect friction) density the density of this rigid body (used to calculate mass based on collider volume) elasticity the "bouncyness" of this object, from 0 (no bounce) to 1 (maximum bouncyness) softness how "squishy" this object is, in the range 0...1; small values (range of 0.00001 to 0.01) give reasonably stiff collision contacts, larger values are more "mushy"
virtual bool iRigidBody::AttachColliderPlane | ( | const csPlane3 & | plane, | |
float | friction, | |||
float | density, | |||
float | elasticity, | |||
float | softness = 0.01f | |||
) | [pure virtual] |
Add a collider plane with given properties.
- Parameters:
-
plane the plane which will act as collider friction how much friction this body has, ranges from 0 (no friction) to infinity (perfect friction) density the density of this rigid body (used to calculate mass based on collider volume) elasticity the "bouncyness" of this object, from 0 (no bounce) to 1 (maximum bouncyness) softness how "squishy" this object is, in the range 0...1; small values (range of 0.00001 to 0.01) give reasonably stiff collision contacts, larger values are more "mushy"
virtual bool iRigidBody::AttachColliderSphere | ( | float | radius, | |
const csVector3 & | offset, | |||
float | friction, | |||
float | density, | |||
float | elasticity, | |||
float | softness = 0.01f | |||
) | [pure virtual] |
Add a collider sphere with given properties.
- Parameters:
-
radius radius of sphere offset position of sphere friction how much friction this body has, ranges from 0 (no friction) to infinity (perfect friction) density the density of this rigid body (used to calculate mass based on collider volume) elasticity the "bouncyness" of this object, from 0 (no bounce) to 1 (maximum bouncyness) softness how "squishy" this object is, in the range 0...1; small values (range of 0.00001 to 0.01) give reasonably stiff collision contacts, larger values are more "mushy"
virtual void iRigidBody::AttachLight | ( | iLight * | light | ) | [pure virtual] |
Attach an iLight to this body.
virtual void iRigidBody::AttachMesh | ( | iMeshWrapper * | mesh | ) | [pure virtual] |
Attach an iMeshWrapper to this body.
virtual void iRigidBody::Collision | ( | iRigidBody * | other, | |
const csVector3 & | pos, | |||
const csVector3 & | normal, | |||
float | depth | |||
) | [pure virtual] |
If there's a collision callback with this body, execute it.
- Parameters:
-
pos is the position on which the collision occured. normal is the collision normal. depth is the penetration depth.
virtual void iRigidBody::DestroyCollider | ( | iDynamicsSystemCollider * | collider | ) | [pure virtual] |
Destroy body collider.
virtual void iRigidBody::DestroyColliders | ( | ) | [pure virtual] |
Destroy body colliders.
virtual bool iRigidBody::Disable | ( | void | ) | [pure virtual] |
Temporarily ignores the body until something collides with it.
virtual bool iRigidBody::Enable | ( | void | ) | [pure virtual] |
Re-enables a body after calling Disable, or by being auto disabled.
virtual const csVector3 iRigidBody::GetAngularVelocity | ( | ) | const [pure virtual] |
Get the angular velocity (rotation).
virtual iCamera* iRigidBody::GetAttachedCamera | ( | ) | [pure virtual] |
Returns the attached camera.
virtual iLight* iRigidBody::GetAttachedLight | ( | ) | [pure virtual] |
Returns the attached light.
virtual iMeshWrapper* iRigidBody::GetAttachedMesh | ( | ) | [pure virtual] |
Returns the attached MeshWrapper.
virtual csVector3 iRigidBody::GetCenter | ( | ) | [pure virtual] |
Get center.
virtual csRef<iDynamicsSystemCollider> iRigidBody::GetCollider | ( | unsigned int | index | ) | [pure virtual] |
Get body collider by its index.
virtual int iRigidBody::GetColliderCount | ( | ) | [pure virtual] |
Get body colliders count.
virtual const csVector3 iRigidBody::GetForce | ( | ) | const [pure virtual] |
Get total force (world space).
virtual csRef<iBodyGroup> iRigidBody::GetGroup | ( | void | ) | [pure virtual] |
Returns which group a body belongs to.
virtual csMatrix3 iRigidBody::GetInertia | ( | ) | [pure virtual] |
Get inertia.
virtual const csVector3 iRigidBody::GetLinearVelocity | ( | ) | const [pure virtual] |
Get the linear velocity (movement).
virtual float iRigidBody::GetMass | ( | ) | [pure virtual] |
Get mass.
virtual const csMatrix3 iRigidBody::GetOrientation | ( | ) | const [pure virtual] |
Get the orientation.
virtual const csVector3 iRigidBody::GetPosition | ( | ) | const [pure virtual] |
Get the position.
virtual void iRigidBody::GetProperties | ( | float * | mass, | |
csVector3 * | center, | |||
csMatrix3 * | inertia | |||
) | [pure virtual] |
Get the physic properties. 0 parameters are ignored.
virtual const csVector3 iRigidBody::GetTorque | ( | ) | const [pure virtual] |
Get total torque (world space).
virtual const csOrthoTransform iRigidBody::GetTransform | ( | ) | const [pure virtual] |
Get the transform.
virtual bool iRigidBody::IsEnabled | ( | void | ) | [pure virtual] |
Returns true if a body is enabled.
virtual bool iRigidBody::IsStatic | ( | void | ) | [pure virtual] |
Tells whether a body has been made static or not.
virtual bool iRigidBody::MakeDynamic | ( | void | ) | [pure virtual] |
Returns a static body to a dynamic state.
virtual bool iRigidBody::MakeStatic | ( | void | ) | [pure virtual] |
Makes a body stop reacting dynamically.
This is especially useful for environmental objects. It will also increase speed in some cases by ignoring all physics for that body
virtual iObject* iRigidBody::QueryObject | ( | void | ) | [pure virtual] |
returns the underlying object
virtual void iRigidBody::SetAngularVelocity | ( | const csVector3 & | vel | ) | [pure virtual] |
Set the angular velocity (rotation).
virtual void iRigidBody::SetCollisionCallback | ( | iDynamicsCollisionCallback * | cb | ) | [pure virtual] |
Set a callback to be executed when this body collides with another If 0, no callback is executed.
virtual void iRigidBody::SetLinearVelocity | ( | const csVector3 & | vel | ) | [pure virtual] |
Set the linear velocity (movement).
virtual void iRigidBody::SetMoveCallback | ( | iDynamicsMoveCallback * | cb | ) | [pure virtual] |
Set a callback to be executed when this body moves.
If 0, no callback is executed.
virtual void iRigidBody::SetOrientation | ( | const csMatrix3 & | trans | ) | [pure virtual] |
Set the orientation.
virtual void iRigidBody::SetPosition | ( | const csVector3 & | trans | ) | [pure virtual] |
Set the position.
virtual void iRigidBody::SetProperties | ( | float | mass, | |
const csVector3 & | center, | |||
const csMatrix3 & | inertia | |||
) | [pure virtual] |
Set the physic properties.
virtual void iRigidBody::SetTransform | ( | const csOrthoTransform & | trans | ) | [pure virtual] |
Set the transform.
virtual void iRigidBody::Update | ( | ) | [pure virtual] |
Update transforms for mesh and/or bone.
The documentation for this struct was generated from the following file:
- ivaria/dynamics.h
Generated for Crystal Space by doxygen 1.4.7