iScript Struct Reference
This provides the interface to a scripting language interpreter. More...
#include <ivaria/script.h>
Inheritance diagram for iScript:
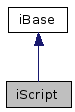
Public Member Functions | |
virtual bool | Call (const char *name, csRef< iScriptObject > &ret, const char *fmt,...)=0 |
Call a subroutine in the script, with object return value. | |
virtual bool | Call (const char *name, csRef< iString > &, const char *fmt,...)=0 |
Call a subroutine in the script, with string return value. | |
virtual bool | Call (const char *name, double &ret, const char *fmt,...)=0 |
Call a subroutine in the script, with double return value. | |
virtual bool | Call (const char *name, float &ret, const char *fmt,...)=0 |
Call a subroutine in the script, with float return value. | |
virtual bool | Call (const char *name, int &ret, const char *fmt,...)=0 |
Call a subroutine in the script, with int return value. | |
virtual bool | Call (const char *name, const char *format,...)=0 |
Call a subroutine in the script, with no return value. | |
virtual bool | GetTruth (const char *name, bool &isTrue) const =0 |
Get the value of a bool variable in the script interpreter. | |
virtual bool | Initialize (iObjectRegistry *object_reg)=0 |
This function is deprecated and should no longer be used. | |
virtual bool | LoadModule (const char *name)=0 |
Load a module in the script interpreter. | |
virtual csRef< iScriptObject > | NewObject (const char *type, const char *ctorFormat,...)=0 |
Create an object in the script. | |
virtual bool | Remove (const char *name)=0 |
Remove a variable from the script interpreter. | |
virtual bool | Retrieve (const char *name, csRef< iScriptObject > &) const =0 |
Get the value of an object variable in the script interpreter. | |
virtual bool | Retrieve (const char *name, csRef< iString > &) const =0 |
Get the value of a string variable in the script interpreter. | |
virtual bool | Retrieve (const char *name, double &data) const =0 |
Get the value of a double variable in the script interpreter. | |
virtual bool | Retrieve (const char *name, float &data) const =0 |
Get the value of a float variable in the script interpreter. | |
virtual bool | Retrieve (const char *name, int &data) const =0 |
Get the value of an int variable in the script interpreter. | |
virtual bool | RunText (const char *text)=0 |
Run some script in the scripting language. | |
virtual bool | SetTruth (const char *name, bool isTrue)=0 |
Set the value of a bool variable in the script interpreter. | |
virtual bool | Store (const char *name, iScriptObject *data)=0 |
Set the value of an object variable in the script interpreter. | |
virtual bool | Store (const char *name, char const *data)=0 |
Set the value of a string variable in the script interpreter. | |
virtual bool | Store (const char *name, double data)=0 |
Set the value of a double variable in the script interpreter. | |
virtual bool | Store (const char *name, float data)=0 |
Set the value of a float variable in the script interpreter. | |
virtual bool | Store (const char *name, int data)=0 |
Set the value of an int variable in the script interpreter. | |
virtual bool | Store (const char *name, void *data, void *tag)=0 |
This function is deprecated and should no longer be used. |
Detailed Description
This provides the interface to a scripting language interpreter.Several functions here take a variable-length argument list with a printf-style format string supporting all the argument types supported by printf, except width and precision specifiers, as they have no meaning here. The specifier "%p" signifies an iScriptObject. Remember to explicitly cast your csRef's to plain pointers in the var arg list.
Definition at line 201 of file script.h.
Member Function Documentation
virtual bool iScript::Call | ( | const char * | name, | |
csRef< iScriptObject > & | ret, | |||
const char * | fmt, | |||
... | ||||
) | [pure virtual] |
Call a subroutine in the script, with object return value.
Returns false if the subroutine named does not exist. Format is a printf-style format string for the arguments.
virtual bool iScript::Call | ( | const char * | name, | |
csRef< iString > & | , | |||
const char * | fmt, | |||
... | ||||
) | [pure virtual] |
Call a subroutine in the script, with string return value.
Returns false if the subroutine named does not exist. Format is a printf-style format string for the arguments.
virtual bool iScript::Call | ( | const char * | name, | |
double & | ret, | |||
const char * | fmt, | |||
... | ||||
) | [pure virtual] |
Call a subroutine in the script, with double return value.
Returns false if the subroutine named does not exist. Format is a printf-style format string for the arguments.
virtual bool iScript::Call | ( | const char * | name, | |
float & | ret, | |||
const char * | fmt, | |||
... | ||||
) | [pure virtual] |
Call a subroutine in the script, with float return value.
Returns false if the subroutine named does not exist. Format is a printf-style format string for the arguments.
virtual bool iScript::Call | ( | const char * | name, | |
int & | ret, | |||
const char * | fmt, | |||
... | ||||
) | [pure virtual] |
Call a subroutine in the script, with int return value.
Returns false if the subroutine named does not exist. Format is a printf-style format string for the arguments.
virtual bool iScript::Call | ( | const char * | name, | |
const char * | format, | |||
... | ||||
) | [pure virtual] |
Call a subroutine in the script, with no return value.
Returns false if the subroutine named does not exist. Format is a printf-style format string for the arguments.
virtual bool iScript::GetTruth | ( | const char * | name, | |
bool & | isTrue | |||
) | const [pure virtual] |
Get the value of a bool variable in the script interpreter.
Returns false if the named variable does not exist.
virtual bool iScript::Initialize | ( | iObjectRegistry * | object_reg | ) | [pure virtual] |
This function is deprecated and should no longer be used.
virtual bool iScript::LoadModule | ( | const char * | name | ) | [pure virtual] |
Load a module in the script interpreter.
virtual csRef<iScriptObject> iScript::NewObject | ( | const char * | type, | |
const char * | ctorFormat, | |||
... | ||||
) | [pure virtual] |
Create an object in the script.
Returns 0 if the constructor fails. CtorFormat is a printf-style format string for the arguments.
virtual bool iScript::Remove | ( | const char * | name | ) | [pure virtual] |
Remove a variable from the script interpreter.
Returns false if the named variable does not exist.
virtual bool iScript::Retrieve | ( | const char * | name, | |
csRef< iScriptObject > & | ||||
) | const [pure virtual] |
Get the value of an object variable in the script interpreter.
Returns false if the named variable does not exist.
Get the value of a string variable in the script interpreter.
Returns false if the named variable does not exist.
virtual bool iScript::Retrieve | ( | const char * | name, | |
double & | data | |||
) | const [pure virtual] |
Get the value of a double variable in the script interpreter.
Returns false if the named variable does not exist.
virtual bool iScript::Retrieve | ( | const char * | name, | |
float & | data | |||
) | const [pure virtual] |
Get the value of a float variable in the script interpreter.
Returns false if the named variable does not exist.
virtual bool iScript::Retrieve | ( | const char * | name, | |
int & | data | |||
) | const [pure virtual] |
Get the value of an int variable in the script interpreter.
Returns false if the named variable does not exist.
virtual bool iScript::RunText | ( | const char * | text | ) | [pure virtual] |
Run some script in the scripting language.
virtual bool iScript::SetTruth | ( | const char * | name, | |
bool | isTrue | |||
) | [pure virtual] |
Set the value of a bool variable in the script interpreter.
virtual bool iScript::Store | ( | const char * | name, | |
iScriptObject * | data | |||
) | [pure virtual] |
Set the value of an object variable in the script interpreter.
virtual bool iScript::Store | ( | const char * | name, | |
char const * | data | |||
) | [pure virtual] |
Set the value of a string variable in the script interpreter.
virtual bool iScript::Store | ( | const char * | name, | |
double | data | |||
) | [pure virtual] |
Set the value of a double variable in the script interpreter.
virtual bool iScript::Store | ( | const char * | name, | |
float | data | |||
) | [pure virtual] |
Set the value of a float variable in the script interpreter.
virtual bool iScript::Store | ( | const char * | name, | |
int | data | |||
) | [pure virtual] |
Set the value of an int variable in the script interpreter.
virtual bool iScript::Store | ( | const char * | name, | |
void * | data, | |||
void * | tag | |||
) | [pure virtual] |
This function is deprecated and should no longer be used.
The documentation for this struct was generated from the following file:
- ivaria/script.h
Generated for Crystal Space by doxygen 1.4.7