The 1060 NetKernel can be used as an embedded co-processor in any Java application.
The Embedded API allows requests to be made directly to a configured instance of
NetKernel running within your JVM. This is a useful pattern to use that doesn't
impose any architectural constraints on your system. The downside is that because
of the strict isolated memory model of modules running within the NetKernel it
is sometimes harder to access classes that may be required as arguments or
results of requests - this topic is covered later.
All classes related to the embedded API are in package
com.ten60.netkernel.embedded
contained within the
lib/1060netkernel-embedded-*.jar
.
Creating a NetKernel instance
A NetKernel instance is created by using the EmbeddedAPIFactory
class.
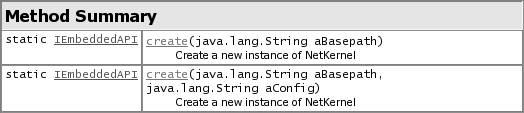
The embeddedAPI may use the standard NetKernel configuration - which will include any installed
applications and modules.
Optionally an alternative configuration can be specified allowing the embeddedAPI to use a customized
kernel system. A custom configuration is passed as a relative path from the basepath.
In either case the file URI of the NetKernel install path must be specified. This path contains the configuration and data
used during execution.
Controlling the NetKernel instance
The IEmbeddedAPI is a thread-safe NetKernel instance that can be started, stopped and have requests issued
on it.
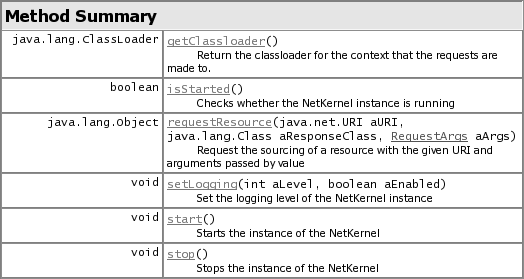
The embedded API implements a full instance of the 1060 NetKernel. All resource references are resolved
and cached. If an application wishes to minimize it's memory footprint whilst processing
is not being performed it can call stop
. When more processing is required the kernel can be restarted with
another call to start
.
For more details on the embedded API see the javadoc-ed source code supplied.
Example
Here is an example that uses the embedded API to execute the system module introspection idoc
and copy the response to System.out
package com.ten60.netkernel.embedded.examples;
import com.ten60.netkernel.embedded.*;
import com.ten60.netkernel.urii.aspect.IAspectString;
import java.io.*;
import java.lang.reflect.*;
import java.net.URI;
import org.w3c.dom.*;
/**
* Example to run the system module introspection and output
* the result to System.out
*/
public class ListModules
{
public static void main(String[] aArgs)
{ if (aArgs.length<1)
{ System.err.println("usage: ListModules <basepath>");
}
IEmbeddedAPI embedded = EmbeddedAPIFactory.create(aArgs[0]);
embedded.setLogging(IEmbeddedAPI.LOG_LEVEL_FINE, false);
embedded.setLogging(IEmbeddedAPI.LOG_LEVEL_INFO, false);
embedded.setLogging(IEmbeddedAPI.LOG_LEVEL_CONTAINER, true);
try
{ embedded.start();
URI request = URI.create("netkernel:modules");
EmbeddedToolkit.writeResource(embedded, request, null, System.out);
}
catch (EmbeddedException e)
{ StringWriter sw = new StringWriter(1024);
try
{ e.appendXML(sw);
} catch (IOException e2) {}
System.err.println(sw.toString());
}
finally
{ try
{ embedded.stop();
} catch (EmbeddedException e) { }
}
}
}
The class EmbeddedToolkit in this example is a helper class which wraps requests on
the embeddedAPI and performs some
java.lang.reflect
ion on the results
to extract
Configuration
The Embedded API uses the NetKernel infrastructure. All modules, applications and resources are
obtained from the basepath location specified in the call to EmbeddedAPIFactory
The Kernel uses a standard system.xml configuration document. This can be the standard config or can be overridden with
a customized version to suit the requirements of the application.
All requests made on the embedded API are resolved in the context of the module which defines the
Internal transport in their module.xml
file. By default this is the module
Backend Fulcrum
.
<transports>
<!---->
<transport>InternalTransport</transport>
</transports>