PingPong a RESTful GUI Application
PingPong is
a demonstration application that is available for installation from the
NetKernel module repositories. The application is an interactive 2D multi-body simulation
of a set of particles and a user movable obstruction/bat contained within a box.
PingPong is an example of a 'RESTful' Java swing application - it is not an applet.
This guide explores PingPong to highlight the architecture, design patterns and development techniques.
Install
To install, use the module management wizard
.
- Select "Install new applications and library modules".
- Select "Install new modules from a 1060 Research distribution server".
- Select a mirror site and also ensure that "Display Libraries" is selected.
- Select "Ping Pong GUI Demo" and follow the instructions to complete the download and install.
Once installed you can control the application from the PingPong Panel
Module
PingPong can be found in the module [install]/modules/pingpong-xxx.jar.
We recommend you unzip the JAR file to a convenient directory so that you can explore the source
code as you read this guide.
Model Overview
Before commencing with a detailed examination let's consider the overall model of this GUI application.
The application consists of a core set of URI addressable services for managing and updating the
state of a particle-simulation model. These services are requested through a custom transport.
The transport manages a Swing JFrame which contains a rendering surface to
display the state of the model and which presents controls for managing and updating the application. An
animation-thread in the Swing frame drives periodic state changes to the model. All events in the
GUI, including animation state changes, are mapped through the transport to URI service requests.
Logical Structure
The application is logically structured into 3-tiers:
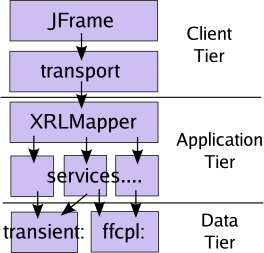
Figure 1:
PingPong Structure
The client tier is composed of a NetKernel transport which communicates with
the underlying services and starts a swing based JFrame user interface.
The service tier comprises a set of services written in both Java and Beanshell.
The services are mapped into the module address space using XRL
.
The data tier comprises two persistence address-spaces mapped to the URI schemes transient: and
ffcpl:. A custom accessor holding an in-memory map is used to implement transient:.
ffcpl:
is the standard NetKernel module resource address
space and is used to store configuration data in the two-level module filesystem.
Source Code Structure
The source code in the pingpong module can be found in /org/ten60/netkernel/pingpong/
and has the following directory structure:
Path | Description |
links.xml |
Defines the fine grained mapping
of the address space onto services within the
module using the XRL mapper .
|
model/ |
Contains the core pingpong accessors and model management services such as presets.
|
representation/ |
Contains the set of custom resource Aspects,
interfaces and implementations that are used to transfer state between the UI
and services.
|
transport/ |
Contains the transport and the set of classes and resources
that implement the client tier user interface of PingPong.
|
transrepresentation/ |
Contains a single Java class which is
registered as a Transreptor that
can serialize and parse a model-preset to and from
a properties file.
|
admin/ |
Contains a set of services
that provide resources for the application management panel.
|
doc/ |
Contains a set of resources
for the about box and dynamically discovered doc.
|
presets/ |
Contains a set of pre-defined
models serialized as property files .
|
URI Address Space
The table below shows the URI address space of the pingpong module. It contains the services and resources
of the PingPong application. The address space is managed by an XRL mapper layer with the service definitions
specified in the ffcpl:/links.xml.
Path (without /pingpong prefix) | Description |
/balls |
SOURCEd, this resource provides a representation of the current position
of all the balls in the simulation. The representation returned is an application
specific Aspect.
|
/bat |
SINKing to this resource updates the simulations position of the bat.
|
/constants/current |
SOURCEing and SINKing this resource retrieves or updates the current set of
operating parameters or constants for the simulation. We shall cover those parameters later.
|
/constants/presets |
This resource represents the set of all available presets for the operating
parameters. An XML representation of the set is returned, the structure of which
will be covered later.
|
/constants/loadPreset |
This resource acts as a function which can be SOURCEd with a name argument.
The name should be one of the available presets named in the /constants/presets
resource. If not an exception will be thrown. A void
response is returned if the preset is successfully loaded and the resource /constants/current is
updated.
|
/constants/savePreset |
This resource also acts as a function which can be SOURCEd with an argument
of preset name. When SOURCEd this function will store the current operating parameters
as a named preset. Again a void response is returned if successful.
|
/isVisible |
The resource represents the visible state of the simulations user interface.
This state is persisted across NetKernel restarts. It returns a boolean
aspect when SOURCEd and takes on when SINKing.
|
/isAudible |
This resource represents the audible state of the simulations user interface.
It returns a boolean aspect when SOURCEd.
When true the simulation "beeps" when collisions occur with a amplitude proportional to
the impact velocity.
|
/sound |
This immutable (it can only be sourced) resource is an audio sample that the user interface plays when
audible.
|
/admin/pluginpanel.xml |
This resource is the XHTML rendering of the PingPong plugin panel used on the
Manage Administration panel.
|
/admin/icon.png |
This resource is the icon image for PingPong -
|
/admin/stateVisibility |
This resource, when SOURCEd, returns a derivative of the /isVisible
resource which is an XHTML fragment containing a human/browser readable
representation.
|
/admin/stateAudibility |
This resource, when SOURCEd, returns a derivative of the /isAudible
resource which is an XHTML fragment containing a human/browser readable
representation.
|
/admin/toggleVisibility |
This resource, when SOURCEd, toggles the visibility state and then returns
/admin/stateVisibility.
|
/admin/toggleAudibility |
This resource, when SOURCEd, toggles the audibility state and then returns
/admin/stateAudbility.
|
/doc/chapter.xml |
This immutable resource when SOURCEd returns back a chapter specification
for the Dynamic Documentation .
|
/doc/readme.html |
This is the resource used for both the UI about box and the documentation exposed
in the dynamic documentation.
|
The User Interface
The PingPong application has a native
Java Swing
user interface which will be familiar to those that have developed a Swing application.
The significant difference in this application is in the way that the UI connects
with the model. Events in the UI initiate requests, using the request context of the custom transport,
to the URI address space of the pingpong module to SOURCE and SINK services and resources.
Transport
Let's consider the custom Transport transport/PingPongTransport.java through
which all URI requests are issued. This transport is registered in the pingpong module with an
entry in the module.xml.
Within an NKF transport
the getContext() method provides the INKFConvenienceHelper context object.
Just like in a scripting language or Accessor, the context object may be used to issue
URI requests to the kernel. Requests issued from a transport are made against
the internal address space of the hosting module.
The getRequestContext() is a wrapper method so that the transport's request context
can be obtained and used from the JFrame class - we shall see shortly how GUI events trigger
requests.
A transport is managed with the same lifecycle as its hosting module, therefore when NetKernel commissions the pingpong module
at boot time the PingPongTransport is started.
In order to give the GUI its own lifecycle, so that it may be started and stopped independently, the
Transport contains a controller thread which monitors the state of the ffcpl:/pingpong/isVisible resource. This resource
can be set by the Application Management Panel and the native "close" functionality on the
window itself. The state is set with a BooleanAspect SINK request. The code below shows how
the JFrame window close event updates the state of the isVisible resource. The request is issued through the transport's request context...
// listen for window closing
this.addWindowListener(new WindowAdapter()
{
public void windowClosing(WindowEvent e)
{ mThread.setStopped();
try
{ mUI.getRequestContext()
.sinkAspect("ffcpl:/pingpong/isVisible", new BooleanAspect(false));
}
catch (NKFException e2)
{ System.out.println(e2.toString());
}
}
} );
///...not shown for clarity...
}
The transport controller thread periodically polls the /isVisible and /isAudible
services to decide if the PingPongFrame should be created, destroyed or to do nothing.
JFrame
The JFrame that is managed by the transport is shown below...
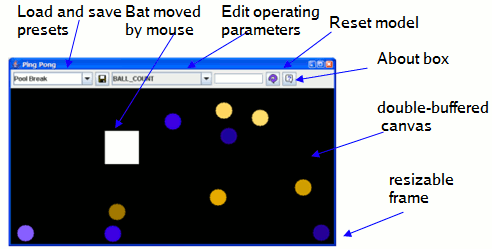
Figure 2:
PingPong UI
When the PingPongFrame is visible it runs its animation thread. This thread
drives periodic state changes to the particle simulation model and updates the
double buffered Canvas which renders the state. The thread computes a variable
delay to ensure that a constant frame rate of 50fps is achieved. In a similar way,
Mouse events cause a change in the state of the model. We shall see below how
these two types of event are mapped to URI requests to change the model.
Finally the JFrame provides a number of controls for modifying model parameters, loading
presets etc. As we shall see, in a similar way to the animation and mouse events, these GUI events
initiate URI requests to the application services.
Core Services
We have seen that events in the JFrame cause URI requests to be issued through the transport for
services in the pingpong module. Let's now consider how the animation thread and mouse events are
mapped to the core services which manage and compute the simulation model.
Let's first consider the animation thread. It periodically makes a call to updateUI() which
initiates a request to SOURCE the current state of the model...
IAspectPingPong gameState = (IAspectPingPong)getRequestContext()
.sourceAspect("ffcpl:/pingpong/balls",IAspectPingPong.class);
This is a SOURCE request to the service ffcpl:/pingpong/balls to update and return the model
with an application specific IAspectPingPong interface. The returned aspect containing the model state is then rendered to the UI.
If we examine the pingpong module definition module.xml we see that the service interface of the
module is delegated to the XRL mapper using a rewrite rule...
<mapping>
<rewrite>
<match>(ffcpl:/pingpong/.*)</match>
<to>active:mapper+operator@ffcpl:/org/ten60/netkernel/pingpong/links.xml+operand@$1</to>
</rewrite>
</mapping>
If we examine the mapper's links.xml we see that ffcpl:/pingpong/balls is mapped to the URI "active:pingpong". So a request to
ffcpl:/pingpong/balls ultimately results in a SOURCE request to active:pingpong.
<links basepath="ffcpl:/pingpong/">
<link>
<ext>/balls</ext>
<int>active:pingpong</int>
</link>
</links>
The active:pingpong accessor declaration is given by the following entry in module.xml:
<ura>
<match>active:pingpong.*</match>
<class>org.ten60.netkernel.pingpong.model.PingPongAccessor</class>
</ura>
Therefore the PingPongAccessor class is called for each source request for ffcpl:/pingpong/balls/ .
The PingPong accessor computes the particle simulation and is written in Java
to
give maximum performance. Other auxiliary services are implemented in BeanShell since
performance is not a factor and the convenience of interactive development and maintenance have priority.
PingPongAccessor
The ffcpl:/pingpong/balls service is treated as a blackbox by the JFrame GUI but let's now look at the internals
of the PingPongAccessor.
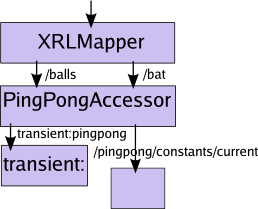
Figure 3:
PingPong Structure
When a request invokes the accessor it first determines the type of request it is receiving - the accessor
handles SOURCE requests to update and retrieve the model state and, as we shall see
in a moment, it also services SINK requests triggered by mouse events.
A SOURCE request causes the getGameState() method to be called which
performs the computation of the particle simulation. Since the Accessor is
RESTful, and therefore stateless, the first task is to request the model parameter resource
ffcpl:/pingpong/constants/current. This is requested with a custom IAspectConstants
which provides an easy Java interface to obtain the computational constants for the model. We shall see later that this resource
is persisted as a Java properties file which may be independently changed by events on the GUI interface. This simple decoupling means
that the physics model is always instantaneously reflected in the computation of the model state.
Next we try to SOURCE the current model which is persisted at the URI transient:pingpong.
If we fail to get the state (because this is the first iteration)
or if the number of balls has changed we create a new simulation state based on
the initial state parameters.
Next the simulation is computed - this calculation has been omitted from the code below to simplify the presentation.
Once the model has been computed it is persisted with a SINK request to transient:pingpong. Finally the state is
put into a custom PingPongAspect (an immutable 'value-object' with a readonly interface). A response is constructed from the aspect and
the response is marked as expired so that it will not be cached. Finally the response is returned to be rendered in the GUI.
private void getGameState(INKFConvenienceHelper context) throws Exception
{
// source the constants
IAspectConstants constants = (IAspectConstants)context
.sourceAspect("ffcpl:/pingpong/constants/current",IAspectConstants.class);
// move constants into local variables, e.g.
int cWidth=constants.getValue(IAspectConstants.WALL_WIDTH).intValue();
// source the simulation state or generate new state based on initial conditions
BallsAspect balls=null;
try
{ balls = (BallsAspect)context
.sourceAspect("transient:pingpong",BallsAspect.class);
if (balls.getBalls().size()!=cCount)
{ balls=null;
}
}
catch (NKFException e) {;}
if (balls==null)
{ balls = new BallsAspect(cCount,
cWidth/2,
cHeight/2,
cInitialSpread,
cInitialVelocity,
cInitialRadians);
}
// update simulation by 1 time unit
// (lots of code here)
// persist state
context.sinkAspect("transient:pingpong",balls);
IURAspect aspect = new PingPongAspect(balls, mi );
INKFResponse response = context.createResponseFrom(aspect);
response.setExpired();
context.setResponse(response);
}
transient:
We have seen above that the state of the model is persisted (SINK) and retrieved (SOURCE) from the URI address space transient:
You will see from the module.xml that the transient: address space is mapped to TransientDataAccessor
which is a data accessor
that provides an
in-memory persistence service for resources.
Granularity
It is worth noting the granularity of the address space for the balls in the simulation.
The whole state of the simulation is SOURCEd with a single URI. We could have chosen
to SOURCE each ball as an independent resource. This technique would work but
would lower the performance and significantly increase the complexity. It is a
general observation when developing NetKernel applications that the performance/complexity
tradeoff is optimal when working with sets of data.
Bat movement
Let's now return to the GUI to consider how mouse events cause bat movement in the simulation.
This is an example of how an asynchronous event in the UI is issued into
the pingpong address space to update state. The JFrame implements java.awt.MouseMotionListener and attaches itself as a
listener.
public void mouseMoved(MouseEvent e)
{ mActualMouseX=e.getX();
mActualMouseY=e.getY();
//some math to limit the velocity of
//bat so it can't move through balls
IAspectPoint bat = new PointAspect(mMouseX,mMouseY);
try
{ getRequestContext().sinkAspect("ffcpl:/pingpong/bat", bat);
}
catch (NKFException ex)
{ System.out.println(ex.toString());
}
}
For each event a custom PointAspect is constructed and SINKed to the ffcpl:/pingpong/bat resource. If you trace the path through
the mapper links.xml you will see that this SINK request also invokes the PingPongAccessor. In this case the accessor recognizes this is a
SINK request and calls the setBatPosition() method to update the model with a new bat position.
Miscellaneous Features
We have covered the core features of the application. This section highlights further interesting features and techniques.
Loading Resources
Rather than issuing requests into the address space, the JFrame's Control Panel
loads its icons using the module classloader:
URL url = getClass().getClassLoader()
.getResource("org/ten60/netkernel/pingpong/transport/save.gif");
Icon c = new ImageIcon(url);
This approach is direct and 'traditional' Java but it ultimately leads to less forward flexibility because it isn't possible
to provide a URI interception of this resource. For example
we could have used the following request-based approach:
IAspectBinaryStream iabs=(IAspectBinaryStream)aFrame.getRequestContext()
.sourceAspect("ffcpl:/org/ten60/netkernel/pingpong/transport/save.gif",
IAspectBinaryStream.class);
ByteArrayOutputStream baos = new ByteArrayOutputStream();
iabs.write(baos);
Icon c = new ImageIcon(baos.toByteArray());
In which case the implementation of the resource URI can be modified - like for example, mapping to dynamic generation by an SVG service - without
the consumer of the resource being aware.
Property and Presets Services
In the main discussion we showed that the physics model may be modified dynamically and that the simulation is computed
using a set of constants. If you examine the links.xml you can see that the preset services are in the URI path /constants/.
These services are called by initiating events in the GUI to save, load and select preset physics models.
If you look in the directory presets/ you will find a set of pre-defined models serialized as Java properties files. In the model/
directory the loadPreset.bsh issues a request to SOURCE these presets with the following code:
constants = (IAspectConstants)context.sourceAspect(resource,IAspectConstants.class);
By asking the kernel for the resource with an interface IAspectConstants - the script is indirectly invoking the PresetTransreptor
which transrepts the serialized Java properties file. Equally the savePreset.bsh works with IAspectConstants but in this case it
SINKs the constants to the ffcpl: space for persistence in the two-level module filesystem. However ffcpl: SINK is
implemented to require a binary stream resource, therefore the kernel must again locate the PresetTransreptor, which additionally provides a serialization mechanism to a
Java properties stream. This is an example of the state transreption pattern.
Management Panel
PingPong demonstrates the use of an application management panel which is accessible through the backend
application panel
.
A management panel is a service which is declared with a special entrypoint with category "plugin":
<entrypoint>
<name>urn:org:ten60:netkernel:pingpong:plugin:panel</name>
<uri>ffcpl:/pingpong/admin/pluginpanel.xml</uri>
<indexable>
<title>Ping Pong</title>
<desc>Plugin panel for Ping Pong</desc>
<keywords />
</indexable>
<categories>app plugin hidden</categories>
<group>ep</group>
<icon>ffcpl:/pingpong/admin/icon.png</icon>
</entrypoint>
This service is dynamically discovered by the NetKernel administration tools so that it is registered and can be called through
the application panel.
If you examine the links.xml mapping you will see that ffcpl:/pingpong/admin/pluginpanel.xml resolves to a the pluginpanel.xml
definition in the admin/ directory. This definition provides a list of administration entrypoints and widgets (XHTML controls) for the application.
Here the widget ffcpl:/pingpong/admin provides toggle controls for visibility and audibility of the UI - you can see that this service
is ultimately implemented by index.bsh which uses XRL template recursion
to implement a miniature AJAX application.