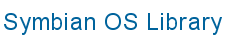
![]() |
![]() |
|
The Video player utility is used to open, play, and obtain information
from sampled video data. This functionality is implemented by the
CVideoPlayerUtility
class. The video data can be supplied
either in a file, a descriptor or a URL.
The sequence diagram below explains the different functionalities of the Video Player Utility:
Using a video player utility involves the following tasks:
The MMF provides a simple application level API called
CVideoPlayerUtility
. Using this interface, you can open,
play, and obtain information from sample video data. The source of the video
data can be files, descriptors, or URLs. You can create an object of this
utility class using the CVideoPlayerUtility::NewL
function. Unlike CMdaAudioPlayerUtility
, the video clips
cannot be opened during instantiation of the
CVideoPlayerUtility
class. Hence all the video clips must
be opened using one of the following open functions:
From a file
OpenFileL(const TDesC& aFileName, TUid aControllerUid=KNullUid);
This method opens the video clip from a file. It uses an optionally specified plugin in the argument to load a controller. If no controller plugin is specified, it searches through the list of available plugins and attempts to use each one until successful or the end of the list is reached.
Note: There is also another method to open a video clip from a file. It is strongly recommended to use this method.
OpenFileL(const TMMSource& aSource, TUid aControllerUid);
Where aSource
is a filename or an open handle to a
file containing the video clip and aControllerUid
is an optionally
specified plugin. If specified, it will force the video player to use the
controller with the given UID. If no controller plugin is specified, this
function searches through a list of all available plugins and attempts to use
each one until successful or the end of the list is reached.
From a descriptor
OpenDesL(const TDesC8& aDescriptor, TUid aControllerUid=KNullUid);
This method opens the video clip from a descriptor. It opens the video clip contained as binary data in a descriptor using an optionally specified plugin in the argument to load a controller. If no controller is specified, it searches through the list of available plugins and attempts to use each one until successful or the end of the list is reached.
From an URL
OpenUrlL(const TDesC& aUrl, TInt aIapId = KUseDefaultIap, const TDesC8& aMimeType=KNullDesC8, TUid aControllerUid=KNullUid);
This method opens the video clip from the specified URL and identified by the MIME type. In addition a plugin can be specified if necessary. If no controller plugin is specified, this function searches through a list of all available plugins and attempts to use each one until successful or the end of the list is reached.
As the opening of the video clip is complete, successfully or
otherwise, the callback function
MVideoPlayerUtilityObserver::MvpuoOpenComplete()
is
called. This notifies the client whether the video clip was successfully opened
or not.
Once the video data is ready, call the
CVideoPlayerUtility::Prepare()
function. when the client
receives the
MVideoPlayerUtilityObserver::MvpuoPrepareComplete()
callback, the video player utility will be in configured state.
To open and automatically play the video clips use the following open functions:
OpenAndPlayFileL(const TDesC& aFileName, TUid aControllerUid=KNullUid);
OpenAndPlayDesL(const TDesC8& aDescriptor, TUid aControllerUid=KNullUid);
OpenAndPlayUrlL(const TDesC& aUrl, TInt aIapId = KUseDefaultIap, const TDesC8& aMimeType=KNullDesC8, TUid aControllerUid=KNullUid);
This section lists different configuration and control related functions. Once the video clip is open and ready to play, configuration adjustments can be made using the following functions:
CVideoPlayerUtility::SetDisplayWindowL
: This
is used to provide the video controller with an area of display to render the
current video frame. It can be represented pictorially as shown below:
The screeenRect specifies the area within the window provided for displaying the video image. This rectangle is in screen coordinates. The video frame will be fitted to this rectangle as best possible, while maintaining the original aspect ratio.
The ClipRect specifies the region to draw. The drawn region will be the intersection between the ClipRect and the ScreenRect.
A crop region is defined as a region in the source video frame. The cropped region is displayed in the center of the ScreenRect with the same scaling as if it was displayed as part of the whole image. This could be used to zoom in on a region by setting the crop region, and setting the scale factor to fill the WindowRect.
void CPlayVideo::SetDisplayWindowL(RWsSession &aWs, CWsScreenDevice &aScreenDevice, RWindowBase &aWindow, const TRect &aWindowRect, const TRect &aClipRect)
{
iVideoUtility->SetDisplayWindowL(aWs, aScreenDevice, aWindow, aWindowRect, aClipRect);
}
The current volume settings can be reported and set appropriately with the help of the following functions: :
CVideoPlayerUtility::Volume()
: Returns
the current playback volume for the audio track of the video clip.
TInt CPlayVideo::Volume()
{
return iVideoUtility->Volume();
}
CVideoPlayerUtility::MaxVolume()
:
Returns the maximum volume that the audio track can support.
CVideoPlayerUtility::SetVolumeL()
: This
function allows the volume of the audio track of the video clip to be set. The
volume can be changed before or during playback and comes to effect
immediately. The volume can be set to a value anywhere between zero and the
maximum permissible volume.
TInt volume;
volume = iVideoPlayer.MaxVolume(); \\Returns maximum volume
void CPlayVideo::SetVolumeL(TInt aVolume) \\Set the audio volume
{
iVideoUtility->SetVolumeL(aVolume);
}
//The volume must be a value between 0 and the value returned by the MaxVolume function.
The audio playback balance settings can be done using the following functions:
CVideoPlayerUtility::SetBalanceL()
:
This function sets the current playback balance for the audio track of the
video clip. It can be any value between KMMFBalanceMaxLeft and
KMMFBalanceMaxRight.
void CPlayVideo::SetBalanceL(TInt aBalance)
{
iVideoUtility->SetBalanceL(aBalance);
}
CVideoPlayerUtility::Balance()
: This
function returns the current playback balance settings for the audio track of
the video clip.
TInt CPlayVideo::Balance()
{
return iVideoUtility->Balance();
}
To set the priority for the playback to access the sound hardware, use the following methods:
PriorityL()
: This function returns the
current playback priority. This is used to arbitrate between multiple objects
simultaneously trying to accesses the sound hardware.
SetPriorityL()
: This function sets the
playback priority.
void CPlayVideo::SetPriorityL(TInt aPriority, TMdaPriorityPreference aPref)
{
iVideoUtility->SetPriorityL(aPriority, aPref);
}
This section lists functions used to query and set different properties of the video clip. Using these functions you can query or set properties of the video clip such as bit and frame rate, current playback position, MIME type, and codecs as shown below:
CVideoPlayerUtility::VideoBitRateL()
: This
function returns the bit rate of the video clip. This returns the video clip's
bit rate in bits per second.
TInt CPlayVideo::VideoBitRateL()
{
return iVideoUtility->VideoBitRateL();
}
CVideoPlayerUtility::VideoFrameRateL()
: This
function returns the video frame rate. This returns the video frame rate in
frames per second.
TReal32 CPlayVideo::VideoFrameRateL()
{
return iVideoUtility->VideoFrameRateL();
}
CVideoPlayerUtility::SetVideoFrameRateL()
:
This function is used to set the number of video frames to be displayed per
second.
void CPlayVideo::SetVideoFrameRateL(TReal32 aFramesPerSecond)
{
iVideoUtility->SetVideoFrameRateL(aFramesPerSecond);
}
Note: The presence of an audio track within a video clip
can be determined using AudioEnabledL()
.
The current playback position can be queried and set using the following functions:
CVideoPlayerUtility::PositionL()
: This
function returns the current playback position. It returns the current position
from the start of the clip in microseconds.
CVideoPlayerUtility::SetPositionL()
: This
function sets the position for the playback within the video clip to start.
TTimeIntervalMicroSeconds pos;
TTimeIntervalMicroSeconds CPlayVideo::PositionL() \\ retrieves the current playback position within the video clip
{
return iVideoUtility->PositionL();
}
This section explains the functions that reports and sets the MIME type and codecs for video and audio data that is already open. The functions are as stated below:
CVideoPlayerUtility::VideoFormatMimeType()
:
This returns the current MIME type of the video clip currently open.
const TDesC8 & CPlayVideo::VideoFormatMimeType()
{
return iVideoUtility->VideoFormatMimeType();
}
The datatype of the audio track used by the video clip can be
retrieved using and AudioTypeL()
.
TFourCC CPlayVideo::AudioTypeL() \\retrieves the codecs used by audio track
{
return iVideoUtility->AudioTypeL();
}
This section explains various functions involved in the video play
process. After configuring the properties, the
CVideoPlayerUtility::play()
function is called for the
video clip to be played. The play can be paused for a small duration using
CVideoPlayerUtility::pause()
and later resumed by calling
CVideoPlayerUtility::play()
function once again. To halt
the video play CVideoPlayerUtility::Stop()
is called. In
order to unload all related controllers and return, use
CVideoPlayerUtility::close()
void CPlayVideo::Play() //Starts playback of the video clip
{
iVideoUtility->Play();
}
void CPlayVideo::PauseL() //Call to this function will maintain the current position
//playback can be resumed by calling Play function again
{
TRAPD(err,iVideoUtility->PauseL());
}
TInt CPlayVideo::Stop() //Stops playing
{
TInt err = iVideoUtility->Stop();
return err;
}
Note: You must call the
CVideoPlayerUtility::Prepare()
function, before calling
any of the functions used in the above given code.
To get the video frame currently being played, use the
CVideoPlayerUtility::GetFrameL()
function.
void CPlayVideo::GetFrameL(TDisplayMode aDisplayMode) //Returns the current frame
{
iVideoUtility->GetFrameL(aDisplayMode);
}
The current frame is sent to the client asynchronously via the
MVideoPlayerUtilityObserver::MvpuoFrameReady()
callback
function.
This section lists functions relevant to controlling the video controller plug-in. Using these functions you can query the progress of the video clip being loaded or rebuffered, and send custom commands to the plug-in directly without using the API.
To get the progress of video clip loading/rebuffering, use the
CVideoPlayerUtility::GetVideoLoadingProgressL()
function.
TInt percentage;
percentage = iVideoPlayer->GetVideoLoadingProgressL() \\Returns the progress, as a percentage of video loading.
Custom commands are used to pass the message directly to the controller plug-in instead of going through APIs such as Play(), Record(), and so on.
To send a synchronous custom command to the controller, use the
CVideoPlayerUtility::CustomCommandSync()
function.
To send a asynchronous custom command to the controller, use the
CVideoPlayerUtility::CustomCommandAsync()
function.